Python 101/ A Comprehensive Step-by-Step Coding Tutorial
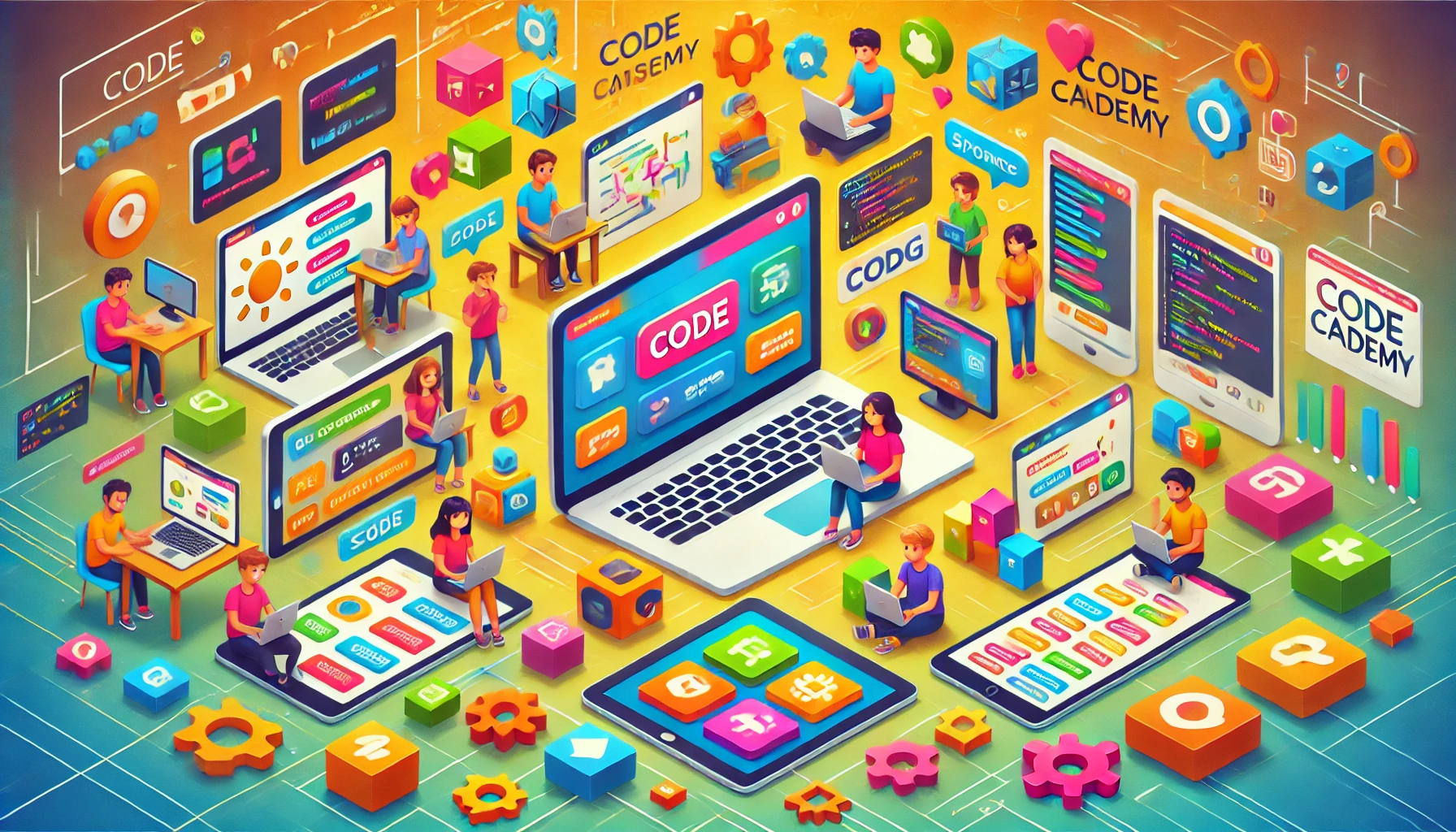
Welcome to “Python 101,” your go-to guide for learning Python from the ground up. Python is a versatile and user-friendly programming language that’s perfect for beginners and experienced coders alike. This tutorial will take you through the essentials, from setting up your Python environment to mastering advanced topics. By the end of this guide, you’ll have a solid understanding of Python and be ready to tackle real-world coding challenges.
Key Takeaways
- Python is great for beginners due to its simple syntax and readability.
- Setting up a proper Python environment is crucial for efficient coding.
- Understanding basic data types and control flow is essential for writing effective programs.
- Functions help you organize your code and make it reusable.
- Python’s extensive libraries and frameworks make it suitable for a wide range of applications.
Setting Up Your Python Environment
Installing Python on Different Operating Systems
To start coding in Python, you first need to install it. Python is available for Windows, macOS, and Linux. Follow these steps to install Python on your operating system:
- Windows:
- Download the installer from the official Python website.
- Run the installer and follow the on-screen instructions.
- Make sure to check the box that says “Add Python to PATH”.
- macOS:
- Open the Terminal application.
- Use Homebrew to install Python by running:
brew install python
.
- Linux:
- Open your terminal.
- Use your package manager to install Python. For example, on Ubuntu, run:
sudo apt-get install python3
.
Choosing the Right IDE or Text Editor
Selecting the right Integrated Development Environment (IDE) or text editor can make coding easier. Here are some popular options:
- PyCharm: A powerful IDE with many features like code completion and debugging tools.
- Visual Studio Code: A lightweight, customizable editor with Python support.
- Jupyter Notebook: Great for data science and interactive coding.
Setting Up Virtual Environments
Virtual environments help you manage dependencies for different projects. Here’s a step-by-step guide to creating and managing a Python virtual environment:
- Install
virtualenv
:- Run:
pip install virtualenv
.
- Run:
- Create a virtual environment:
- Navigate to your project directory.
- Run:
virtualenv venv
.
- Activate the virtual environment:
- On Windows:
venv\Scripts\activate
. - On macOS/Linux:
source venv/bin/activate
.
- On Windows:
- Deactivate the virtual environment:
- Simply run:
deactivate
.
- Simply run:
Setting up a virtual environment ensures that your projects are isolated and dependencies are managed efficiently.
Understanding Python Syntax and Basics
Writing Your First Python Program
To start with Python, you need to write a simple program. Open your text editor or IDE and type the following code:
print("Hello, World!")
Save the file with a .py
extension and run it from your command line or through your IDE. Congratulations on running your first Python script!
Basic Data Types and Variables
In Python, variables are used to store data. You don’t need to declare the type of a variable explicitly. The type is inferred from the value assigned to it. Here are some common data types in Python:
- Integers: Whole numbers (e.g.,
a = 5
) - Floats: Decimal numbers (e.g.,
b = 3.14
) - Strings: Text data (e.g.,
c = "Hello, World!"
) - Lists: Ordered collections of items (e.g.,
d = [1, 2, 3, 4, 5]
) - Tuples: Ordered, immutable collections of items (e.g.,
e = (1, 2, 3)
) - Dictionaries: Unordered collections of key-value pairs (e.g.,
f = {"name": "Alice", "age": 25}
)
Comments and Documentation
Comments are used to explain code and are not executed. In Python, you can write comments using the #
symbol. For example:
# This is a comment
print("Hello, World!") # This is also a comment
For longer explanations, you can use multi-line comments with triple quotes:
"""
This is a multi-line comment.
It can span multiple lines.
"""
print("Hello, World!")
> Comments are essential for making your code understandable to others and your future self.
Control Flow in Python
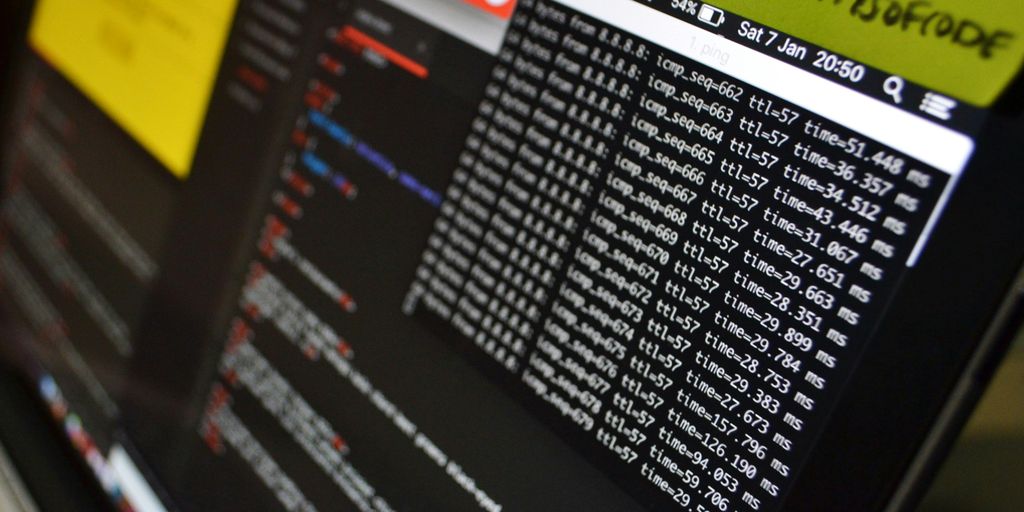
Conditional Statements
Conditional statements are used to execute code only if a certain condition is true. The main types are if
, elif
, and else
statements. Learn how if-else statements control code flow, create dynamic programs, and unlock Python’s full potential. Here’s a simple example:
X = 10
Y = 12
if X < Y:
print('X is less than Y')
elif X > Y:
print('X is greater than Y')
else:
print('X and Y are equal')
In this example, the output will be X is less than Y
because the condition X < Y
is true.
Loops and Iterations
Loops allow you to repeat a block of code multiple times. There are two main types of loops in Python: while
loops and for
loops.
- While Loop: Repeats as long as a condition is true.
count = 0
while count < 10:
print(count)
count += 1
- For Loop: Repeats a set number of times, often used to iterate over a sequence.
fruits = ['Banana', 'Apple', 'Grapes']
for fruit in fruits:
print(fruit)
Comprehensions
Comprehensions provide a concise way to create lists, dictionaries, and sets. They are more readable and often faster than traditional loops.
- List Comprehension: Creates a new list by applying an expression to each item in an existing list.
squares = [x**2 for x in range(10)]
- Dictionary Comprehension: Creates a new dictionary by applying an expression to each key-value pair in an existing dictionary.
squares_dict = {x: x**2 for x in range(10)}
Comprehensions are a powerful tool for writing clean and efficient code. They can replace many traditional loops and conditional statements, making your code more readable and concise.
Working with Functions
Defining and Calling Functions
Functions are a convenient way to divide your code into useful blocks, making it more readable and reusable. To define a function in Python, use the def
keyword followed by the function name and parentheses. For example:
def add(a, b):
return a + b
To call this function, simply use its name followed by parentheses, passing any required arguments:
result = add(10, 20)
print(result) # Output: 30
Function Arguments and Return Values
Functions can take arguments and return values. Arguments are specified within the parentheses in the function definition. You can also set default values for arguments. For example:
def greet(name, message="Hello"):
return f"{message}, {name}!"
Calling greet("Alice")
will return “Hello, Alice!”, while greet("Bob", "Hi")
will return “Hi, Bob!”.
Lambda Functions and Higher-Order Functions
Lambda functions are small anonymous functions defined using the lambda
keyword. They are often used for short, throwaway functions. For example:
add = lambda x, y: x + y
print(add(5, 3)) # Output: 8
Higher-order functions are functions that take other functions as arguments or return them as results. A common example is the map
function, which applies a given function to all items in a list:
numbers = [1, 2, 3, 4]
squares = list(map(lambda x: x**2, numbers))
print(squares) # Output: [1, 4, 9, 16]
By the end of this section, you’ll know how to define, call, and use Python’s built-in functions effectively.
Data Structures in Python
Lists and Tuples
In Python, lists and tuples are fundamental data structures. Lists are mutable, meaning you can change their content, while tuples are immutable.
- This list contains both strings and numbers.
- Tuples are faster than lists and are useful for fixed collections of items.
Dictionaries and Sets
Dictionaries and sets are also built-in data structures in Python.
- This dictionary stores a student’s name and age.
- Sets are useful for storing unique items.
Understanding Mutability
Mutability refers to whether an object can be changed after it is created.
- Mutable objects: These can be changed. Examples include lists and dictionaries.
- Immutable objects: These cannot be changed. Examples include tuples and strings.
Understanding the difference between mutable and immutable objects is crucial for writing efficient and bug-free code.
File Handling in Python
Reading and Writing Files
Python makes it easy to work with files. You can open, read, write, and close files using built-in functions such as open()
, read()
, write()
, and close()
. Here’s a simple example:
with open('file.txt', 'r') as file:
content = file.read()
print(content)
Working with File Paths
Handling file paths correctly is crucial for file operations. Python’s os
and pathlib
modules provide tools to work with file paths across different operating systems. For example:
from pathlib import Path
file_path = Path('folder/subfolder/file.txt')
print(file_path)
Handling Exceptions
When working with files, it’s important to handle exceptions to avoid crashes. Use try
, except
, else
, and finally
blocks to manage errors gracefully. For instance:
try:
with open('file.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print('File not found')
finally:
print('Operation completed')
In this article, we will explore Python file handling, advantages, disadvantages, and how open, write, and append functions work in Python file.
Object-Oriented Programming in Python
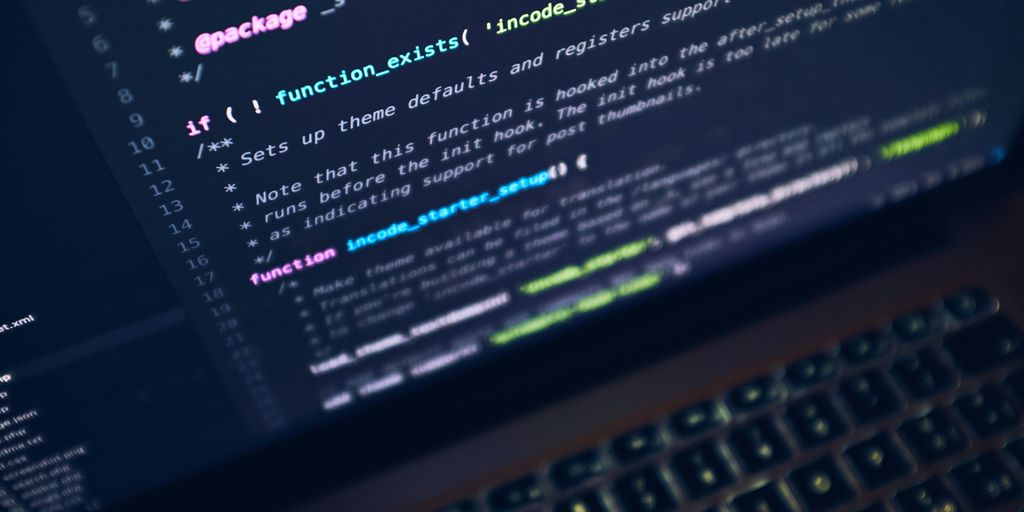
Object-oriented programming is a fundamental concept in Python, empowering developers to build modular, maintainable, and scalable applications. Let’s dive into the key aspects of OOP in Python.
Classes and Objects
In Python, classes are blueprints for creating objects. A class defines a set of attributes and methods that the created objects will have. For example:
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def start_engine(self):
print("Engine started")
An object is an instance of a class. You can create multiple objects from a single class:
my_car = Car("Toyota", "Corolla")
my_car.start_engine()
Inheritance and Polymorphism
Inheritance allows a new class to inherit attributes and methods from an existing class. This helps in reusing code and creating a hierarchical relationship between classes. For example:
class ElectricCar(Car):
def __init__(self, make, model, battery_size):
super().__init__(make, model)
self.battery_size = battery_size
def start_engine(self):
print("Electric engine started")
Polymorphism allows methods to do different things based on the object it is acting upon. This is achieved through method overriding, as shown in the start_engine
method above.
Magic Methods and Operator Overloading
Magic methods in Python are special methods that start and end with double underscores (__
). They allow you to define how objects of your class behave with built-in functions and operators. For example, the __init__
method is a magic method that initializes an object.
Operator overloading allows you to define how operators like +
, -
, *
, etc., behave for objects of your class. For example:
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
v1 = Vector(2, 3)
v2 = Vector(4, 5)
v3 = v1 + v2
print(v3.x, v3.y) # Output: 6 8
Modules and Packages
Importing Modules
In Python, you can organize your code into modules. A module is simply a file containing Python code. You can import these modules into your programs to reuse code. For example, to use the math module, you would write:
import math
print(math.sqrt(16))
Creating Your Own Modules
Creating your own modules is straightforward. Just save your Python code in a file with a .py
extension. For instance, if you have a file named my_module.py
with the following content:
def greet(name):
return f"Hello, {name}!"
You can import and use it in another script:
import my_module
print(my_module.greet("World"))
Using Third-Party Packages
Python’s strength lies in its vast array of third-party packages. These packages can be installed using the pip
tool. For example, to install the requests
package, you would run:
pip install requests
Once installed, you can use it in your code:
import requests
response = requests.get('https://api.example.com')
print(response.status_code)
Organizing your code into modules and packages makes it more manageable and reusable.
Error Handling and Debugging
Understanding Exceptions
In Python, exceptions are errors that occur during the execution of a program. They disrupt the normal flow of the program. Common exceptions include ValueError
, TypeError
, and IndexError
.
Using Try-Except Blocks
To handle exceptions, Python provides the try
, except
, else
, and finally
blocks. Here’s a simple example:
try:
# Code that might raise an exception
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
else:
print("Division successful!")
finally:
print("This will always execute.")
Debugging Techniques
Debugging is the process of finding and fixing errors in your code. Here are some common techniques:
- Print Statements: Use print statements to check the values of variables at different points in your code.
- Using a Debugger: Tools like
pdb
in Python allow you to step through your code and inspect variables. - Code Reviews: Having someone else review your code can help catch errors you might have missed.
Debugging is an essential skill for any programmer. It helps ensure your code runs smoothly and efficiently.
Introduction to Data Science with Python
Using Pandas for Data Analysis
Pandas is a powerful library for data manipulation and analysis. It provides data structures like DataFrames and Series, which make it easy to handle and analyze data. With Pandas, you can perform operations such as filtering, grouping, and merging data.
Key features of Pandas include:
- Data alignment and handling of missing data
- Label-based slicing, indexing, and subsetting of large datasets
- Merging and joining datasets
- Reshaping and pivoting datasets
Data Visualization with Matplotlib
Matplotlib is a widely-used library for creating static, animated, and interactive visualizations in Python. It is highly customizable and can generate plots, histograms, bar charts, and more. Data visualization helps in understanding data patterns and trends.
Here are some common types of plots you can create with Matplotlib:
- Line plots
- Scatter plots
- Bar charts
- Histograms
- Pie charts
Introduction to Machine Learning with Scikit-Learn
Scikit-Learn is a robust library for machine learning in Python. It provides simple and efficient tools for data mining and data analysis. With Scikit-Learn, you can implement various machine learning algorithms, including classification, regression, clustering, and dimensionality reduction.
Important features of Scikit-Learn include:
- Easy-to-use API
- Comprehensive documentation
- Wide range of algorithms
- Integration with other Python libraries like Pandas and NumPy
Learning data science with Python will help you understand the basics of Python along with different steps of data science. Master data science with Python to unlock new career opportunities and enhance your analytical skills.
Web Development with Python
Introduction to Flask
Flask is a lightweight and modular framework that provides the tools needed to build web applications. It is highly customizable and allows you to add extensions for features such as form validation, user authentication, and database integration.
Building a Simple Web Application
- Install Flask: You can install Flask using pip:
pip install Flask
- Create a Basic App: Create a new Python file and add the following code:
from flask import Flask app = Flask(__name__) @app.route('/') def home(): return "Hello, Flask!" if __name__ == '__main__': app.run(debug=True)
- Run the App: Run your app by executing the Python file:
python yourfile.py
- View in Browser: Open your web browser and go to
http://127.0.0.1:5000/
to see your app in action.
Deploying Your Application
Deploying a Flask application can be done using various services like Heroku, AWS, or even a simple VPS. Here are the basic steps to deploy on Heroku:
- Install Heroku CLI: Download and install the Heroku CLI from the official website.
- Login to Heroku: Use the command:
heroku login
- Create a Heroku App: Navigate to your project directory and create a new Heroku app:
heroku create
- Deploy Your Code: Push your code to Heroku using Git:
git push heroku main
- Open Your App: Once deployed, open your app in the browser:
heroku open
Gain practical experience through hands-on projects and real-world applications, mastering essential Django principles and techniques. Whether you’re just starting out or looking to enhance your skills, web development with Python offers endless possibilities.
Best Practices and Coding Standards
Writing Clean and Readable Code
Follow PEP 8: Adhere to the Python Enhancement Proposal 8 (PEP 8), which is the style guide for Python code. It covers naming conventions, line length, indentation, and much more, promoting a readable and uniform coding style.
- Use Descriptive Names: Choose meaningful names for variables, functions, and classes which reflect their purpose and make your code self-documenting.
- Keep Functions Short: Each function should have a single, clear purpose. If a function is performing multiple tasks, consider breaking it down into smaller functions.
Using Version Control with Git
- Use Git: Keep your projects under version control with Git. It helps track changes, revert to previous states, and collaborate with others more effectively.
- Commit Often: Small, frequent commits are preferable. They make it easier to understand changes and isolate issues.
Testing and Documentation
- Write Tests: Use Python’s built-in unittest framework or third-party libraries like pytest to write tests. Good test coverage helps prevent regressions and ensures code reliability.
- Document Your Code: Use docstrings to describe what functions, classes, and modules do. Tools like Sphinx can generate beautiful documentation from your docstrings.
- Keep Documentation Up-to-Date: As your code evolves, make sure your documentation and comments are updated to match. Outdated documentation can be misleading and detrimental.
Adopting these best practices will not only make your code more readable and maintainable but also help you collaborate more effectively with others.
Following best practices and coding standards is key to becoming a great programmer. At AlgoCademy, we offer interactive tutorials and AI-assisted learning to help you master these skills. Our step-by-step guides and video lessons make it easy to understand complex concepts. Ready to take your coding to the next level?
Conclusion
Congratulations on completing Python 101! You’ve taken your first steps into the world of programming with one of the most popular and versatile languages out there. From understanding basic syntax to exploring more complex concepts like functions and modules, you’ve built a solid foundation. Python’s simplicity and readability make it an excellent choice for beginners, while its powerful libraries and frameworks cater to advanced users. Keep practicing and exploring, and you’ll find that Python can open doors to many exciting opportunities in fields like web development, data science, and automation. Happy coding!
Frequently Asked Questions
What is Python used for?
Python is used for many things like web development, data science, automation, and making games. It’s very flexible and can be used for lots of different tasks.
How do I install Python?
To install Python, go to the official Python website and download the installer for your operating system. Follow the instructions to complete the installation.
What is an IDE, and do I need one for Python?
An IDE is a tool that helps you write code. It can make coding easier by providing features like error checking and code suggestions. While you don’t need one to code in Python, it can be very helpful.
What are some basic data types in Python?
Some basic data types in Python are integers (whole numbers), floats (decimal numbers), strings (text), and booleans (True or False).
How do I write a comment in Python?
To write a comment in Python, use the # symbol before your comment. For example: # This is a comment.
What is a virtual environment in Python?
A virtual environment is a tool that helps keep your Python projects separate. It makes sure that each project can have its own dependencies, without interfering with other projects.
How do I handle errors in Python?
You can handle errors in Python using try-except blocks. This lets your program keep running even if it encounters an error.
What are some popular Python libraries for data science?
Some popular Python libraries for data science are Pandas for data analysis, Matplotlib for data visualization, and Scikit-Learn for machine learning.