Exploring AI Assistants: Claude, GitHub Copilot, and Visual Studio Code Integration
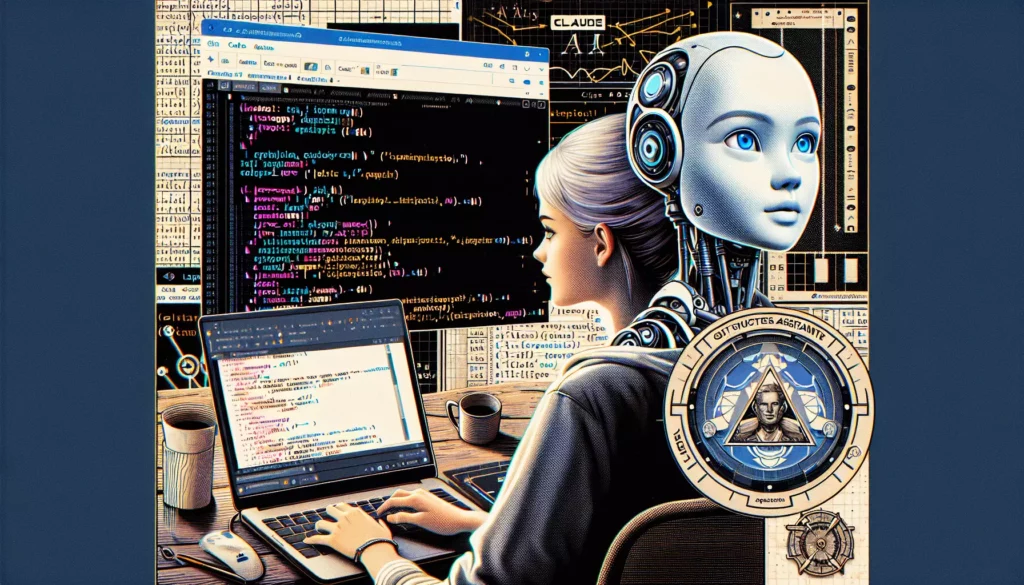
In the ever-evolving landscape of software development, artificial intelligence (AI) has emerged as a powerful ally for programmers. AI assistants are revolutionizing the way developers write code, debug issues, and even learn new programming concepts. In this comprehensive guide, we’ll delve into the world of AI coding assistants, with a particular focus on Claude, GitHub Copilot, and their integration with Visual Studio Code. We’ll explore how these tools can enhance your coding experience, boost productivity, and help you become a more efficient developer.
Understanding AI Coding Assistants
AI coding assistants are sophisticated tools that leverage machine learning and natural language processing to provide intelligent suggestions, automate repetitive tasks, and offer context-aware help to developers. These assistants aim to streamline the coding process, reduce errors, and help programmers focus on higher-level problem-solving rather than getting bogged down in syntax and boilerplate code.
Key Features of AI Coding Assistants
- Code completion and suggestion
- Automated code generation
- Context-aware documentation lookup
- Bug detection and fixing
- Natural language query support
- Code refactoring assistance
Introducing Claude: The Versatile AI Assistant
Claude is an advanced AI assistant developed by Anthropic, designed to assist with a wide range of tasks, including coding. While not exclusively a coding assistant, Claude’s natural language understanding and generation capabilities make it a powerful tool for developers seeking help with various programming-related tasks.
Key Features of Claude
- Natural language interaction for coding queries
- Explanation of complex programming concepts
- Assistance with algorithm design and problem-solving
- Code review and suggestions for improvement
- Language-agnostic support for multiple programming languages
Using Claude for Coding Assistance
To leverage Claude for coding assistance, developers can engage in a conversational manner, asking questions about programming concepts, seeking explanations for specific code snippets, or requesting help with algorithm design. Here’s an example of how you might interact with Claude:
Human: Can you explain the concept of recursion and provide a simple example in Python?
Claude: Certainly! Recursion is a programming concept where a function calls itself to solve a problem by breaking it down into smaller, similar subproblems. It's often used for tasks that have a naturally recursive structure, like traversing tree-like data structures or implementing divide-and-conquer algorithms.
Here's a simple example of recursion in Python to calculate the factorial of a number:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
# Example usage
print(factorial(5)) # Output: 120
In this example, the factorial function calls itself with a smaller argument (n - 1) until it reaches the base case (n == 0 or n == 1). The results are then combined to compute the final factorial value.
Recursion can be powerful, but it's important to ensure that your recursive function has a proper base case to avoid infinite recursion and stack overflow errors.
This example demonstrates how Claude can provide both conceptual explanations and practical code examples, making it a valuable resource for learning and problem-solving in programming.
GitHub Copilot: Your AI Pair Programmer
GitHub Copilot is an AI-powered code completion tool developed by GitHub in collaboration with OpenAI. It uses a large language model trained on vast amounts of public code to generate suggestions and complete code snippets based on the context of your current project.
Key Features of GitHub Copilot
- Real-time code suggestions as you type
- Whole-line and whole-function completions
- Support for multiple programming languages and frameworks
- Context-aware suggestions based on your codebase
- Integration with popular IDEs and text editors
How GitHub Copilot Works
GitHub Copilot analyzes the code you’ve written, including variable names, function signatures, and comments, to understand the context of your project. It then generates suggestions that are likely to be relevant and useful. As you continue to write code, Copilot adapts its suggestions to better match your coding style and project requirements.
Example of GitHub Copilot in Action
Let’s say you’re working on a Python script to process some data. You might start typing a function definition like this:
def process_data(data):
# Process the input data and return the result
GitHub Copilot might then suggest completing the function with something like:
def process_data(data):
# Process the input data and return the result
result = []
for item in data:
processed_item = item.strip().lower()
if processed_item:
result.append(processed_item)
return result
This suggestion demonstrates how Copilot can infer the likely implementation based on the function name and comment, saving you time and effort in writing boilerplate code.
Visual Studio Code: The Ultimate Development Environment
Visual Studio Code (VS Code) is a popular, free, and open-source code editor developed by Microsoft. Known for its speed, flexibility, and extensive ecosystem of extensions, VS Code has become a favorite among developers across various programming languages and platforms.
Key Features of Visual Studio Code
- IntelliSense code completion
- Debugging support for multiple languages
- Git integration
- Extensibility through a vast marketplace of extensions
- Customizable user interface and keybindings
- Integrated terminal
- Support for remote development
Integrating AI Assistants with Visual Studio Code
One of the strengths of VS Code is its ability to integrate seamlessly with various AI coding assistants, including GitHub Copilot. This integration allows developers to leverage the power of AI within their preferred development environment, creating a more efficient and productive coding experience.
Setting Up GitHub Copilot in VS Code
To use GitHub Copilot in Visual Studio Code, follow these steps:
- Install the GitHub Copilot extension from the VS Code marketplace.
- Sign in to your GitHub account when prompted.
- Authorize the Copilot extension to access your GitHub account.
- Once activated, Copilot will start providing suggestions as you code.
With Copilot integrated into VS Code, you’ll see suggestions appear in light gray text as you type. You can accept these suggestions by pressing Tab or continue typing to ignore them.
Using Claude with Visual Studio Code
While Claude doesn’t have a direct integration with VS Code like GitHub Copilot does, you can still leverage its capabilities alongside your development environment. Here are a few ways to use Claude in conjunction with VS Code:
- Use Claude in a separate browser window for quick queries and explanations.
- Copy and paste code snippets from VS Code to Claude for analysis or improvement suggestions.
- Use Claude to generate code snippets or algorithms, which you can then implement in VS Code.
- Leverage Claude for high-level planning and problem-solving before diving into the implementation in VS Code.
Comparing Claude and GitHub Copilot
While both Claude and GitHub Copilot are AI-powered tools that can assist with coding tasks, they have different strengths and use cases. Let’s compare these two assistants across various dimensions:
1. Scope and Functionality
- Claude: A general-purpose AI assistant capable of handling a wide range of tasks, including coding, but not limited to it. Claude excels in providing explanations, answering questions, and offering high-level guidance.
- GitHub Copilot: Specifically designed for code completion and generation, focusing on providing real-time suggestions within the coding environment.
2. Integration with Development Environments
- Claude: No direct integration with IDEs or text editors. Typically used through a chat interface or API.
- GitHub Copilot: Seamlessly integrates with popular IDEs like Visual Studio Code, providing in-editor suggestions and completions.
3. Learning and Explanation
- Claude: Excels at explaining complex concepts, providing detailed answers to programming questions, and offering step-by-step guidance for problem-solving.
- GitHub Copilot: Primarily focused on code generation rather than explanation. While it can provide working code examples, it doesn’t offer detailed explanations of concepts.
4. Code Generation
- Claude: Can generate code snippets and algorithms based on high-level descriptions or requirements, but may require more specific prompting.
- GitHub Copilot: Specializes in generating code in real-time based on the current context of your project, often providing full function implementations or complex code structures.
5. Contextual Awareness
- Claude: Maintains context within a conversation but doesn’t have direct access to your project’s codebase.
- GitHub Copilot: Highly context-aware, considering your entire codebase, file structure, and coding patterns when generating suggestions.
6. Language and Framework Support
- Claude: Can assist with a wide range of programming languages and frameworks, leveraging its broad knowledge base.
- GitHub Copilot: Supports numerous programming languages and frameworks, with particularly strong performance in popular languages and commonly used libraries.
Best Practices for Using AI Coding Assistants
While AI coding assistants like Claude and GitHub Copilot can significantly enhance your productivity, it’s important to use them effectively and responsibly. Here are some best practices to keep in mind:
1. Understand the Generated Code
Always review and understand the code suggested by AI assistants. Don’t blindly accept suggestions without verifying their correctness and appropriateness for your specific use case.
2. Use AI as a Learning Tool
Leverage AI assistants to learn new concepts, explore alternative approaches, and improve your coding skills. Use tools like Claude to ask for explanations and clarifications on complex topics.
3. Maintain Code Quality
While AI can generate code quickly, it’s your responsibility to ensure that the final code meets your project’s quality standards, follows best practices, and is well-documented.
4. Be Mindful of Licensing and Privacy
Be aware of the licensing implications of using AI-generated code in your projects. Also, be cautious about sharing sensitive or proprietary information with AI assistants.
5. Combine AI with Human Expertise
Use AI assistants as a complement to your skills and knowledge, not a replacement. Combine AI suggestions with your own expertise and critical thinking.
6. Stay Updated
AI coding assistants are rapidly evolving. Stay informed about new features, improvements, and best practices to make the most of these tools.
The Future of AI in Coding Education
As AI coding assistants continue to evolve and improve, they are poised to play an increasingly important role in coding education and skill development. Here are some ways AI is shaping the future of programming education:
1. Personalized Learning Paths
AI can analyze a learner’s progress, strengths, and weaknesses to create tailored learning paths, focusing on areas that need improvement and adapting to individual learning styles.
2. Interactive Coding Exercises
AI-powered platforms can generate dynamic coding exercises and challenges, providing immediate feedback and guidance as learners work through problems.
3. Real-time Code Analysis and Feedback
AI assistants can analyze code in real-time, offering suggestions for improvement, identifying potential bugs, and explaining best practices.
4. Natural Language Programming
As AI becomes more adept at understanding natural language, we may see a shift towards more intuitive programming interfaces where developers can describe their intentions in plain language and have AI generate the corresponding code.
5. Enhanced Collaboration
AI can facilitate better collaboration among developers by suggesting optimal ways to merge code, resolve conflicts, and maintain consistent coding styles across teams.
6. Accelerated Skill Acquisition
With AI assistants providing instant access to knowledge and best practices, developers can potentially learn new languages, frameworks, and concepts more quickly than ever before.
Conclusion
AI coding assistants like Claude and GitHub Copilot, when integrated with powerful development environments like Visual Studio Code, are revolutionizing the way developers work and learn. These tools offer unprecedented support in code generation, problem-solving, and knowledge acquisition, allowing developers to focus on higher-level thinking and creative problem-solving.
As we’ve explored in this article, each AI assistant has its strengths and ideal use cases. Claude excels in providing detailed explanations and guidance across a wide range of topics, making it an excellent tool for learning and high-level problem-solving. GitHub Copilot, on the other hand, shines in its ability to generate context-aware code suggestions in real-time, seamlessly integrating with your development workflow.
The key to maximizing the benefits of these AI assistants lies in understanding their capabilities and limitations, and using them as complementary tools to enhance your own skills and knowledge. By following best practices and staying informed about the latest developments in AI-assisted coding, you can leverage these powerful tools to become a more efficient, knowledgeable, and productive developer.
As AI continues to advance, we can expect even more sophisticated and helpful coding assistants in the future. These tools will likely play an increasingly important role in coding education, making programming more accessible and accelerating the learning process for aspiring developers. However, it’s important to remember that AI assistants are tools to augment human creativity and problem-solving skills, not replace them. The most successful developers will be those who can effectively combine their own expertise with the capabilities of AI to create innovative and impactful solutions.
Whether you’re a seasoned professional or just starting your coding journey, embracing AI coding assistants can open up new possibilities and help you stay at the forefront of the rapidly evolving world of software development. So why not give Claude, GitHub Copilot, or other AI coding assistants a try? You might be surprised at how they can transform your coding experience and help you reach new heights in your programming skills.