Coding Interview Mistakes That Cost You the Job
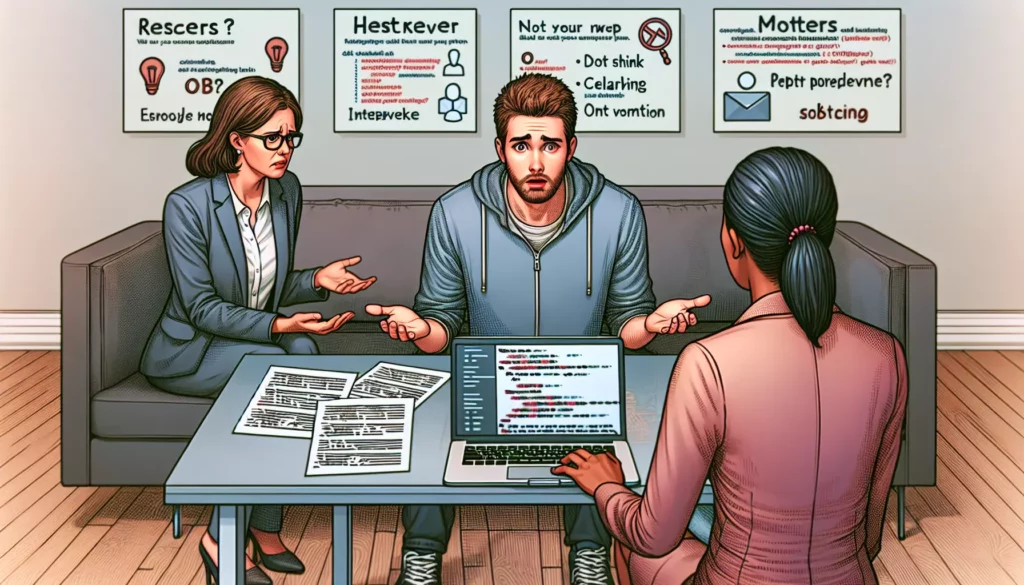
Coding interviews are a crucial step in landing your dream job in the tech industry. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or a promising startup, your performance during the technical interview can make or break your chances. As an aspiring developer, it’s essential to be aware of common pitfalls that could cost you the job. In this comprehensive guide, we’ll explore the most frequent coding interview mistakes and provide actionable tips to help you avoid them.
1. Failing to Clarify the Problem
One of the biggest mistakes candidates make is jumping into coding without fully understanding the problem at hand. This often leads to solving the wrong problem or missing crucial details.
Why it’s a problem:
- Wastes valuable interview time
- Shows poor communication skills
- May result in an incomplete or incorrect solution
How to avoid it:
- Take time to read the problem statement carefully
- Ask clarifying questions about input formats, edge cases, and expected outputs
- Discuss your understanding of the problem with the interviewer before coding
Remember, interviewers are not just evaluating your coding skills but also your ability to communicate and collaborate effectively.
2. Neglecting to Plan Before Coding
Another common mistake is diving into coding without a clear plan or strategy. This can lead to disorganized code and difficulty explaining your thought process.
Why it’s a problem:
- Results in inefficient or suboptimal solutions
- Makes it harder to explain your approach to the interviewer
- Increases the likelihood of bugs and errors
How to avoid it:
- Spend a few minutes outlining your approach on paper or a whiteboard
- Discuss your high-level strategy with the interviewer
- Break down the problem into smaller, manageable steps
A well-thought-out plan demonstrates your problem-solving skills and helps you stay organized throughout the coding process.
3. Ignoring Time and Space Complexity
Many candidates focus solely on getting a working solution without considering the efficiency of their code. This oversight can be a major red flag for interviewers.
Why it’s a problem:
- Shows a lack of understanding of algorithmic efficiency
- May result in solutions that don’t scale well for large inputs
- Misses an opportunity to demonstrate advanced problem-solving skills
How to avoid it:
- Always analyze and discuss the time and space complexity of your solution
- Consider multiple approaches and their trade-offs
- Be prepared to optimize your initial solution if asked
Understanding and discussing complexity demonstrates your ability to write efficient, scalable code – a crucial skill for any developer.
4. Poor Code Organization and Style
Writing messy, unreadable code is a quick way to lose points in a coding interview. Even if your solution works, poorly organized code can leave a negative impression.
Why it’s a problem:
- Makes it difficult for the interviewer to follow your logic
- Suggests poor coding practices and habits
- Can lead to bugs and errors that are hard to identify and fix
How to avoid it:
- Use meaningful variable and function names
- Maintain consistent indentation and formatting
- Break down complex logic into smaller, well-named functions
- Add comments to explain non-obvious parts of your code
Here’s an example of poorly organized code versus well-organized code:
Poorly organized:
function s(a){let r=0;for(let i=0;i<a.length;i++){if(a[i]%2==0){r+=a[i];}}return r;}
Well-organized:
function sumEvenNumbers(numbers) {
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
if (isEven(numbers[i])) {
sum += numbers[i];
}
}
return sum;
}
function isEven(number) {
return number % 2 === 0;
}
The well-organized version is much easier to read, understand, and maintain.
5. Not Testing Your Code
Submitting your solution without thoroughly testing it is a critical mistake that can cost you the job, even if your approach is correct.
Why it’s a problem:
- Leaves room for bugs and edge case failures
- Shows a lack of attention to detail
- Misses an opportunity to demonstrate your debugging skills
How to avoid it:
- Always test your code with multiple inputs, including edge cases
- Walk through your code step-by-step with a small example
- Consider writing unit tests if time allows
Here’s an example of how you might test a function that finds the maximum element in an array:
function findMax(arr) {
if (arr.length === 0) {
throw new Error("Array is empty");
}
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
// Test cases
console.log(findMax([1, 3, 2, 5, 4])); // Expected: 5
console.log(findMax([-1, -3, -2, -5, -4])); // Expected: -1
console.log(findMax([0])); // Expected: 0
try {
console.log(findMax([]));
} catch (error) {
console.log("Error caught:", error.message); // Expected: Error caught: Array is empty
}
By testing your code with various inputs, including edge cases, you demonstrate thoroughness and attention to detail.
6. Failing to Communicate During Problem-Solving
Staying silent while working on the problem is a missed opportunity to showcase your thought process and problem-solving skills.
Why it’s a problem:
- Leaves the interviewer guessing about your approach
- Misses chances for the interviewer to provide hints or guidance
- Fails to demonstrate your ability to work collaboratively
How to avoid it:
- Explain your thought process as you work through the problem
- Discuss trade-offs between different approaches
- Ask for feedback or confirmation when you’re unsure
Remember, the interview is not just about getting the right answer but also about demonstrating how you approach problems and work with others.
7. Giving Up Too Easily
Encountering a difficult problem and giving up without putting in a genuine effort is a major red flag for interviewers.
Why it’s a problem:
- Shows a lack of persistence and problem-solving skills
- Misses opportunities to demonstrate your ability to work under pressure
- Fails to show your full potential to the interviewer
How to avoid it:
- Break down the problem into smaller, manageable parts
- Start with a brute force solution and then work on optimizing it
- Ask for hints if you’re truly stuck, but show that you’ve made an effort
Even if you don’t arrive at the perfect solution, showing persistence and a willingness to tackle challenging problems can leave a positive impression.
8. Neglecting to Handle Edge Cases
Failing to consider and handle edge cases in your solution is a common mistake that can significantly impact your interview performance.
Why it’s a problem:
- Results in incomplete or incorrect solutions
- Shows a lack of thoroughness in your problem-solving approach
- Misses an opportunity to demonstrate attention to detail
How to avoid it:
- Always consider edge cases such as empty inputs, null values, or boundary conditions
- Discuss potential edge cases with the interviewer
- Include error handling and input validation in your code
Here’s an example of how to handle edge cases in a function that calculates the average of an array of numbers:
function calculateAverage(numbers) {
if (!Array.isArray(numbers)) {
throw new Error("Input must be an array");
}
if (numbers.length === 0) {
return 0; // Or throw an error, depending on requirements
}
const sum = numbers.reduce((acc, num) => {
if (typeof num !== "number") {
throw new Error("All elements must be numbers");
}
return acc + num;
}, 0);
return sum / numbers.length;
}
// Test cases
console.log(calculateAverage([1, 2, 3, 4, 5])); // Expected: 3
console.log(calculateAverage([0])); // Expected: 0
console.log(calculateAverage([])); // Expected: 0
try {
console.log(calculateAverage("not an array"));
} catch (error) {
console.log("Error caught:", error.message); // Expected: Error caught: Input must be an array
}
try {
console.log(calculateAverage([1, 2, "3", 4, 5]));
} catch (error) {
console.log("Error caught:", error.message); // Expected: Error caught: All elements must be numbers
}
By handling these edge cases, you demonstrate a thorough understanding of the problem and attention to detail in your implementation.
9. Overlooking the Importance of Data Structures
Choosing the wrong data structure or failing to utilize appropriate data structures can lead to inefficient solutions and missed opportunities to showcase your knowledge.
Why it’s a problem:
- Results in suboptimal time or space complexity
- Misses chances to demonstrate your understanding of fundamental computer science concepts
- Can lead to unnecessarily complex code
How to avoid it:
- Review common data structures and their use cases before the interview
- Consider which data structure best fits the problem at hand
- Discuss trade-offs between different data structures with the interviewer
Here’s an example of how choosing the right data structure can significantly improve the efficiency of a solution:
Inefficient solution using an array:
function containsDuplicate(nums) {
for (let i = 0; i < nums.length; i++) {
for (let j = i + 1; j < nums.length; j++) {
if (nums[i] === nums[j]) {
return true;
}
}
}
return false;
}
// Time complexity: O(n^2)
Efficient solution using a Set:
function containsDuplicate(nums) {
const seen = new Set();
for (const num of nums) {
if (seen.has(num)) {
return true;
}
seen.add(num);
}
return false;
}
// Time complexity: O(n)
By using a Set data structure, we’ve improved the time complexity from O(n^2) to O(n), demonstrating a good understanding of data structures and their applications.
10. Not Asking Questions About the Role or Company
While this isn’t strictly a coding mistake, failing to ask thoughtful questions about the role or company at the end of the interview can leave a negative impression.
Why it’s a problem:
- Shows a lack of genuine interest in the position
- Misses an opportunity to gather important information for your decision-making
- Fails to demonstrate your enthusiasm and initiative
How to avoid it:
- Prepare a list of thoughtful questions about the role, team, and company culture
- Ask about the challenges and opportunities in the position
- Inquire about the company’s technical stack and development processes
Here are some example questions you might ask:
- “Can you tell me more about the team I’d be working with and the projects they’re currently focused on?”
- “What are the biggest challenges facing the engineering team right now?”
- “How does the company approach continuous learning and professional development for its engineers?”
- “Can you describe the typical development cycle for a feature, from ideation to deployment?”
- “What opportunities are there for mentorship or leadership within the engineering team?”
Asking insightful questions not only helps you gather valuable information but also demonstrates your genuine interest in the role and your potential as a thoughtful, engaged team member.
Conclusion
Coding interviews can be challenging, but by avoiding these common mistakes, you can significantly improve your chances of success. Remember to:
- Clarify the problem before coding
- Plan your approach
- Consider time and space complexity
- Write clean, well-organized code
- Test your solution thoroughly
- Communicate your thought process
- Persist through challenges
- Handle edge cases
- Choose appropriate data structures
- Ask thoughtful questions about the role and company
By focusing on these areas, you’ll not only perform better in coding interviews but also develop skills that will serve you well throughout your career as a software developer. Remember, practice makes perfect, so use platforms like AlgoCademy to hone your skills and prepare for technical interviews. With dedication and the right approach, you’ll be well on your way to landing your dream job in the tech industry.