Should You Avoid High-Level Languages as a Beginner?
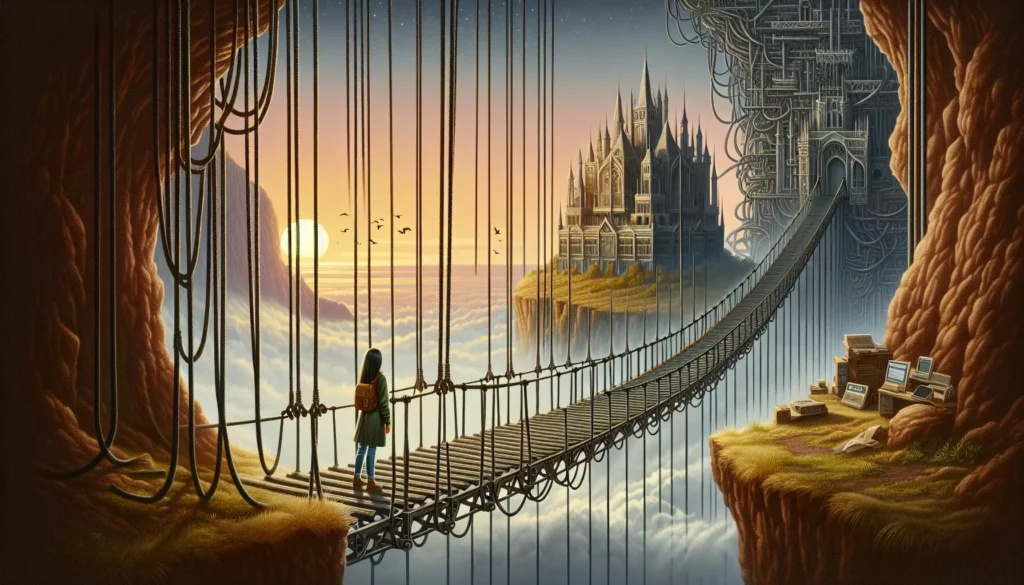
When embarking on your programming journey, one of the first decisions you’ll face is choosing which programming language to learn. This choice can be overwhelming, especially with the myriad of options available, ranging from low-level languages like Assembly to high-level languages such as Python or JavaScript. A common piece of advice floating around in coding circles is that beginners should avoid high-level languages and start with lower-level ones to build a solid foundation. But is this advice sound? Let’s dive deep into this topic and explore whether beginners should indeed steer clear of high-level languages or embrace them.
Understanding High-Level vs. Low-Level Languages
Before we can address the main question, it’s crucial to understand what we mean by high-level and low-level languages.
Low-Level Languages
Low-level languages are closer to machine code and provide more direct control over a computer’s hardware. They include:
- Assembly language
- Machine code
These languages offer fine-grained control over system resources but are more challenging to write and understand.
High-Level Languages
High-level languages are more abstracted from machine code and are designed to be more human-readable. Examples include:
- Python
- JavaScript
- Java
- C#
- Ruby
These languages are generally easier to learn and use, as they handle many low-level details automatically.
The Case for Starting with Low-Level Languages
Proponents of starting with low-level languages often argue that it provides several benefits:
1. Better Understanding of Computer Architecture
Learning a low-level language like Assembly forces you to understand how computers work at a fundamental level. You’ll learn about memory management, CPU instructions, and hardware interactions.
2. Appreciation for Abstraction
When you start with a low-level language and then move to a high-level one, you’ll have a deeper appreciation for the abstractions provided by high-level languages.
3. Efficient Code Writing
Understanding low-level operations can help you write more efficient code, even in high-level languages, as you’ll have a better grasp of what’s happening “under the hood.”
4. Debugging Skills
Low-level programming often requires meticulous attention to detail, which can foster strong debugging skills that are valuable across all programming paradigms.
The Case for Starting with High-Level Languages
On the other hand, many educators and professionals advocate for beginners to start with high-level languages. Here’s why:
1. Faster Learning Curve
High-level languages are designed to be more intuitive and closer to human language. This allows beginners to grasp programming concepts more quickly without getting bogged down in low-level details.
2. Immediate Gratification
With high-level languages, beginners can create functional programs much faster, which can be incredibly motivating. For example, you can create a simple web scraper in Python with just a few lines of code:
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title.string)
3. Focus on Problem-Solving
High-level languages allow beginners to focus on problem-solving and algorithmic thinking rather than getting caught up in the intricacies of memory management or system-level operations.
4. Relevance to Modern Development
Many of today’s most popular and in-demand programming jobs involve high-level languages. Starting with these can prepare beginners for real-world applications more quickly.
5. Abundance of Resources
High-level languages often have larger communities, more comprehensive documentation, and a wealth of learning resources, making it easier for beginners to find help and tutorials.
The Middle Ground: A Balanced Approach
While the debate between high-level and low-level languages for beginners continues, many experienced programmers and educators suggest a balanced approach:
1. Start with a High-Level Language
Begin your journey with a high-level language like Python or JavaScript. This allows you to grasp fundamental programming concepts, such as:
- Variables and data types
- Control structures (if statements, loops)
- Functions and modules
- Object-oriented programming basics
2. Build Projects
Use your high-level language skills to build real projects. This could include:
- Simple games
- Web applications
- Data analysis scripts
- Automation tools
3. Dive Deeper
As you become comfortable with programming concepts, start exploring how things work under the hood. This might involve:
- Learning about computer architecture
- Studying data structures and algorithms
- Exploring memory management concepts
4. Experiment with Lower-Level Languages
Once you have a solid foundation, consider learning a lower-level language like C to gain insights into system-level programming. This doesn’t mean you need to become an expert, but understanding the basics can be beneficial.
The Role of Learning Platforms like AlgoCademy
Platforms like AlgoCademy play a crucial role in this balanced approach to learning programming. Here’s how:
1. Structured Learning Path
AlgoCademy provides a structured curriculum that guides learners from basic concepts to more advanced topics. This ensures that beginners don’t miss crucial foundational knowledge while working with high-level languages.
2. Focus on Algorithmic Thinking
While using high-level languages, AlgoCademy emphasizes algorithmic thinking and problem-solving skills. This focus helps bridge the gap between high-level abstractions and fundamental computer science concepts.
3. Interactive Coding Environments
Platforms like AlgoCademy often provide interactive coding environments where learners can experiment with code without worrying about setup and configuration. This is particularly beneficial for beginners using high-level languages.
4. Gradual Introduction to Lower-Level Concepts
As learners progress, AlgoCademy can introduce lower-level concepts within the context of high-level languages. For example, discussing memory management while teaching Python’s garbage collection.
5. Preparation for Technical Interviews
AlgoCademy’s focus on preparing learners for technical interviews at major tech companies ensures that even those starting with high-level languages are exposed to the fundamental computer science concepts often tested in these interviews.
Real-World Perspectives
To provide a more rounded view, let’s consider some perspectives from industry professionals and educators:
“I always recommend beginners start with Python. It allows them to focus on programming concepts without getting bogged down in syntax. Once they’re comfortable, they can explore other languages and lower-level concepts.” – Sarah Chen, Software Engineer at Google
“In my university courses, we start with Java to teach object-oriented programming, then move to C to cover systems programming. This gives students a good balance of high-level and low-level understanding.” – Dr. Michael Roberts, Computer Science Professor
“As a self-taught developer, I started with JavaScript and built web applications. Later, I learned C and Assembly to understand computer architecture better. Both approaches have their merits, but starting with a high-level language allowed me to build practical skills quickly.” – Alex Thompson, Full-Stack Developer
Addressing Common Concerns
Let’s address some common concerns about starting with high-level languages:
1. “You won’t understand what’s really happening”
While it’s true that high-level languages abstract away many details, this doesn’t mean you can’t learn these concepts later. Many developers start with high-level languages and gradually delve into lower-level concepts as they progress.
2. “You’ll develop bad habits”
This concern is often overstated. Good programming practices like writing clean, efficient code can be learned and applied in any language. It’s more about the quality of instruction and practice than the level of the language.
3. “You won’t be able to optimize your code”
While low-level languages offer more direct control for optimization, high-level languages have their own optimization techniques. Moreover, for beginners, the ability to write functional code is more important than micro-optimizations.
4. “You’ll be limited in what you can do”
Modern high-level languages are incredibly powerful and can be used for a wide range of applications, from web development to machine learning. Limitations are more often due to a developer’s knowledge than the language itself.
Making the Decision: Factors to Consider
When deciding whether to start with a high-level or low-level language, consider the following factors:
1. Your Goals
If you want to quickly build web applications or analyze data, a high-level language might be the best start. If you’re interested in embedded systems or operating system development, a lower-level language could be more appropriate.
2. Available Time
High-level languages generally have a shorter learning curve, allowing you to start building projects faster. If you have limited time to dedicate to learning, this could be a significant advantage.
3. Learning Style
Some people prefer to understand systems from the ground up, while others learn best by building and experimenting. Consider your learning style when choosing your first language.
4. Job Market
Research the job market in your area or your desired field. While foundational knowledge is important, aligning your skills with market demands can be beneficial for your career.
5. Support and Resources
Consider the availability of learning resources, community support, and tools for the language you’re considering. This can significantly impact your learning experience.
Conclusion: Embracing the Learning Journey
In the end, the question shouldn’t be whether to avoid high-level languages as a beginner, but rather how to create a comprehensive learning journey that prepares you for a successful programming career. Here’s a suggested approach:
- Start with a high-level language to grasp fundamental programming concepts and build practical skills quickly.
- Use platforms like AlgoCademy to ensure you’re learning not just syntax, but also important computer science and problem-solving skills.
- As you progress, explore lower-level concepts to deepen your understanding of computer systems.
- Continuously challenge yourself with new projects and problems to apply your knowledge in practical ways.
- Stay curious and open to learning new languages and paradigms throughout your career.
Remember, programming is a vast field, and your learning journey will likely span your entire career. Whether you start with Python or Assembly, the key is to start, stay consistent, and never stop learning. Platforms like AlgoCademy can provide the structure and resources you need to progress from a beginner to an interview-ready developer, regardless of which language you choose to start with.
The most important step is to begin your journey. Choose a language, start coding, and embrace the learning process. With dedication and the right resources, you can become a proficient programmer, whether you start with a high-level language or not.