Why Focusing on Backend Development Is Better for Beginners
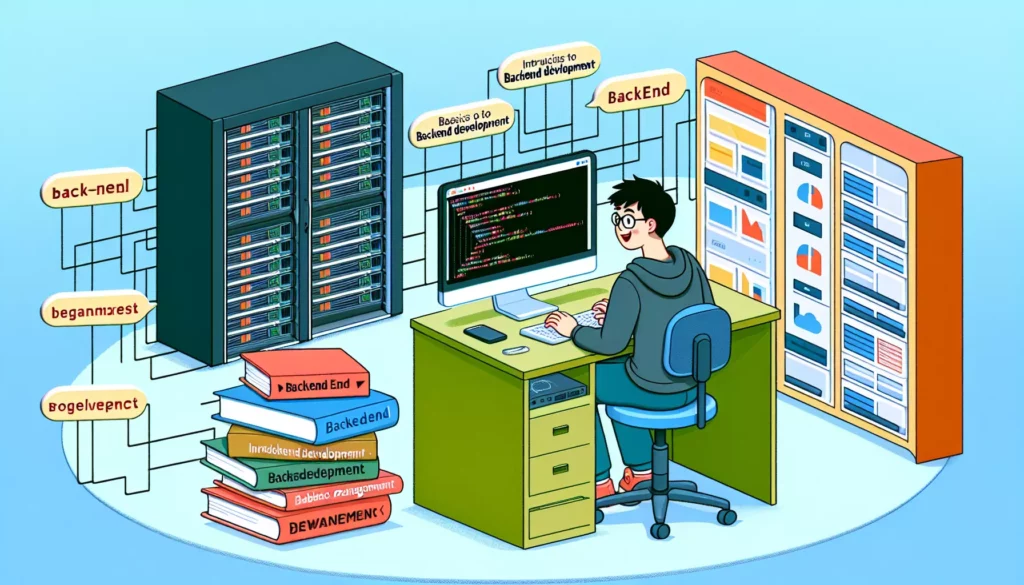
In the vast world of programming, aspiring developers often find themselves at a crossroads when deciding where to begin their journey. While frontend development with its visually appealing results might seem like an attractive starting point, there’s a compelling case to be made for why backend development is actually a better focus for beginners. In this comprehensive guide, we’ll explore the reasons why starting with backend development can provide a stronger foundation and potentially lead to more opportunities in the long run.
Understanding Backend Development
Before diving into the benefits, let’s clarify what backend development entails. Backend development refers to the server-side of web applications. It’s the behind-the-scenes functionality that powers the frontend, handling data storage, server logic, and application programming interfaces (APIs). Backend developers work with databases, server-side languages like Python, Java, or Node.js, and are responsible for the core computational logic of web applications.
Key Components of Backend Development:
- Server-side programming languages (Python, Java, Ruby, PHP, etc.)
- Databases and database management systems (MySQL, PostgreSQL, MongoDB)
- API development and management
- Server architecture and infrastructure
- Security implementations
- Scalability and performance optimization
Why Backend Development is Ideal for Beginners
1. Stronger Grasp of Programming Fundamentals
Backend development often requires a more in-depth understanding of core programming concepts. When you start with backend, you’re more likely to encounter and work with:
- Data structures and algorithms
- Object-oriented programming principles
- Memory management
- Concurrency and multithreading
These foundational concepts are crucial for any programmer, regardless of specialization. By focusing on backend development, beginners can build a robust understanding of these principles, which will serve them well throughout their careers.
2. Logical Thinking and Problem-Solving Skills
Backend development challenges you to think logically and solve complex problems. You’ll often need to:
- Design efficient algorithms
- Optimize database queries
- Handle large-scale data processing
- Implement security measures
These tasks require strong analytical skills and the ability to break down complex problems into manageable parts. Developing these skills early on will make you a more effective programmer, regardless of which direction your career takes.
3. Less Frequent Technology Changes
Frontend technologies and frameworks tend to evolve rapidly, with new tools and libraries emerging frequently. This can be overwhelming for beginners who are still trying to grasp the basics. Backend technologies, while still evolving, tend to be more stable. Languages like Java, Python, and C# have been around for decades and continue to be widely used.
This stability allows beginners to focus on learning core concepts without the pressure of constantly adapting to new tools or frameworks. It provides a more solid foundation upon which to build your skills.
4. Scalability and Performance Focus
Backend development inherently involves considerations of scalability and performance. As a backend developer, you’ll learn to think about:
- How to handle increasing numbers of users
- Optimizing database queries for speed
- Implementing caching mechanisms
- Designing efficient APIs
These skills are invaluable in building robust, high-performance applications. Understanding scalability from the beginning will make you a more thoughtful and effective developer, capable of creating solutions that can grow with user demand.
5. Diverse Career Opportunities
While both frontend and backend developers are in high demand, backend skills often open doors to a wider range of opportunities. Backend developers can work on:
- Web applications
- Mobile app backends
- Cloud computing services
- Data engineering
- Machine learning and AI systems
- IoT (Internet of Things) applications
This diversity means that as a backend developer, you have the flexibility to explore various domains and industries, potentially leading to a more varied and interesting career path.
6. Higher Earning Potential
While salaries can vary based on location, experience, and specific skills, backend developers often command higher salaries compared to frontend developers. This is partly due to the complexity of backend systems and the critical role they play in an application’s functionality and performance.
According to various salary surveys and job market reports, backend developers typically earn 10-20% more than their frontend counterparts, especially at senior levels. Starting your journey with backend development could potentially lead to higher earning prospects in the long term.
Getting Started with Backend Development
If you’re convinced that backend development is the right path for you, here’s a roadmap to get started:
1. Choose a Programming Language
Start with a versatile language that’s widely used in backend development. Good options for beginners include:
- Python: Known for its readability and versatility
- Java: Widely used in enterprise applications
- JavaScript (Node.js): Allows you to use JavaScript on the server-side
- Ruby: Known for its elegant syntax and Rails framework
Here’s a simple “Hello, World!” example in Python to get you started:
def say_hello():
print("Hello, World!")
say_hello()
2. Learn About Databases
Understanding how to work with databases is crucial for backend development. Start with relational databases like MySQL or PostgreSQL, then explore NoSQL options like MongoDB. Learn basic SQL queries and database design principles.
Here’s a basic SQL query to create a table:
CREATE TABLE users (
id INT PRIMARY KEY,
username VARCHAR(50),
email VARCHAR(100),
created_at TIMESTAMP
);
3. Understand API Development
APIs are the backbone of modern web applications. Learn how to design and implement RESTful APIs. Familiarize yourself with concepts like HTTP methods, status codes, and JSON data format.
Here’s a simple example of a Flask API endpoint in Python:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/hello', methods=['GET'])
def hello_api():
return jsonify({"message": "Hello, World!"})
4. Learn About Server Architecture
Understand the basics of how servers work, including concepts like:
- HTTP/HTTPS protocols
- Load balancing
- Caching mechanisms
- Microservices architecture
5. Practice Security Best Practices
Security is paramount in backend development. Learn about:
- Authentication and authorization
- Data encryption
- SQL injection prevention
- Cross-Site Scripting (XSS) protection
6. Version Control and Collaboration
Learn to use Git for version control and collaborate on projects using platforms like GitHub or GitLab. This is essential for working in teams and managing your code effectively.
Overcoming Challenges in Backend Development
While backend development offers numerous advantages, it’s not without its challenges. Here are some common hurdles beginners might face and how to overcome them:
1. Abstraction and Lack of Visual Feedback
Challenge: Unlike frontend development where you can immediately see the results of your code, backend work is more abstract. This can be frustrating for beginners who crave visual feedback.
Solution: Use logging extensively to track the flow of your program. Implement unit tests to verify your code’s behavior. Use tools like Postman to interact with your APIs and see the results of your backend operations.
2. Complexity of Systems
Challenge: Backend systems can be complex, involving multiple components like databases, servers, and third-party services.
Solution: Start small and gradually increase complexity. Begin with simple scripts, then move to basic web applications. As you gain confidence, introduce more complex elements like databases and external APIs.
3. Dealing with Large Datasets
Challenge: Backend developers often need to handle and process large amounts of data, which can be overwhelming for beginners.
Solution: Start by working with small datasets to understand the principles. Gradually increase the size of your datasets. Learn about data structures and algorithms to efficiently handle large amounts of data. Explore concepts like pagination and lazy loading.
4. Understanding Server Infrastructure
Challenge: Grasping concepts related to server management, deployment, and scaling can be daunting.
Solution: Start with local development environments. Gradually explore cloud platforms like AWS, Google Cloud, or Heroku, which offer free tiers for beginners. Take advantage of online courses and documentation to learn about server infrastructure step-by-step.
5. Keeping Up with Best Practices
Challenge: Backend development best practices evolve, and it can be challenging to keep up with the latest trends and security measures.
Solution: Follow reputable tech blogs, join developer communities, and participate in forums. Attend webinars and conferences when possible. Remember, it’s okay to not know everything – focus on mastering the fundamentals first.
Building Your First Backend Project
To solidify your learning and gain practical experience, it’s crucial to work on projects. Here’s an idea for a beginner-friendly backend project:
Todo List API
Create a RESTful API for a todo list application. This project will cover many fundamental aspects of backend development:
- Setting up a server (using Express.js with Node.js, for example)
- Implementing CRUD operations (Create, Read, Update, Delete)
- Working with a database (start with SQLite for simplicity)
- Handling user authentication
- Implementing basic security measures
Here’s a basic structure to get you started:
const express = require('express');
const sqlite3 = require('sqlite3').verbose();
const app = express();
const port = 3000;
// Middleware
app.use(express.json());
// Database connection
const db = new sqlite3.Database('./todos.db');
// Create todos table
db.run(`CREATE TABLE IF NOT EXISTS todos (
id INTEGER PRIMARY KEY AUTOINCREMENT,
title TEXT,
completed BOOLEAN
)`);
// Routes
app.get('/todos', (req, res) => {
db.all("SELECT * FROM todos", [], (err, rows) => {
if (err) {
res.status(500).json({error: err.message});
return;
}
res.json(rows);
});
});
app.post('/todos', (req, res) => {
const {title} = req.body;
db.run(`INSERT INTO todos (title, completed) VALUES (?, ?)`, [title, false], function(err) {
if (err) {
res.status(500).json({error: err.message});
return;
}
res.json({id: this.lastID, title, completed: false});
});
});
// Add more routes for updating and deleting todos
app.listen(port, () => {
console.log(`Todo list API running on port ${port}`);
});
This basic structure sets up a simple API with endpoints to get all todos and create a new todo. You can expand on this by adding update and delete functionality, implementing user authentication, and enhancing security measures.
Conclusion
Embarking on your programming journey with a focus on backend development offers numerous advantages. It provides a solid foundation in core programming concepts, encourages logical thinking and problem-solving skills, and opens doors to diverse and potentially lucrative career opportunities. While it may present some initial challenges, the long-term benefits make it an excellent choice for beginners.
Remember, the key to success in backend development (or any area of programming) is consistent practice and a willingness to continuously learn. Start with the basics, work on projects, and gradually increase the complexity of your work. Engage with the developer community, ask questions, and don’t be afraid to make mistakes – they’re an essential part of the learning process.
As you progress in your backend development journey, you’ll find that the skills you acquire are not only valuable in themselves but also provide a strong foundation for understanding full-stack development and even branching into other areas of software engineering. The problem-solving abilities and system thinking you develop will serve you well throughout your career, regardless of the specific technologies you work with.
So, take that first step into backend development. Write your first “Hello, World!” server, create your first database, and start building. The world of backend development is vast and exciting, filled with challenges and opportunities. Embrace the learning process, and before you know it, you’ll be well on your way to becoming a proficient backend developer, equipped with skills that are in high demand in the tech industry.
Happy coding!