Is Following Coding Trends Distracting You from Real Skills?
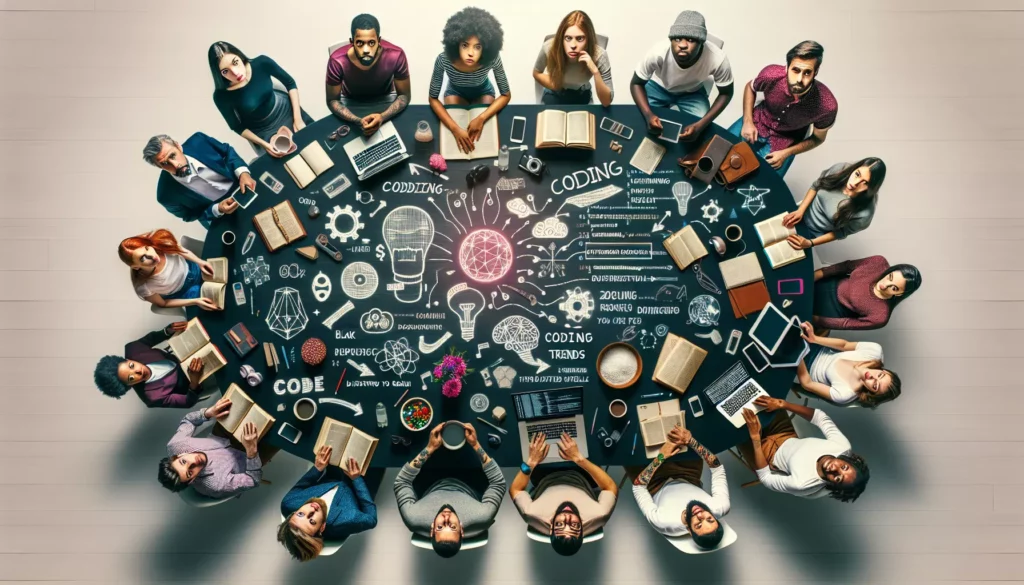
In the ever-evolving world of technology, it’s easy to get caught up in the latest coding trends and buzzwords. From new programming languages to cutting-edge frameworks, the tech industry is constantly introducing shiny new tools and methodologies. But amidst this whirlwind of innovation, an important question arises: Are these trends actually helping you become a better programmer, or are they distracting you from developing the fundamental skills that truly matter?
The Allure of Coding Trends
It’s undeniable that staying current with industry trends is important for any professional, including software developers. New technologies can offer improved efficiency, better user experiences, and solutions to complex problems. However, the rapid pace at which these trends emerge and evolve can create a sense of constant pressure to learn and adapt.
Some of the reasons why developers might feel compelled to chase every new trend include:
- Fear of becoming obsolete in a fast-paced industry
- Desire to stay competitive in the job market
- Excitement about new possibilities and features
- Peer pressure or influence from the developer community
- Marketing hype surrounding new technologies
While these motivations are understandable, it’s crucial to consider whether constantly pivoting to learn new technologies is the most effective way to grow as a developer.
The Core Skills That Really Matter
Regardless of the specific technologies or frameworks you work with, there are fundamental skills that form the backbone of effective software development. These skills transcend individual languages or tools and are essential for long-term success in the field:
1. Problem-Solving and Algorithmic Thinking
At its core, programming is about solving problems. The ability to break down complex issues into manageable components and devise efficient solutions is crucial. This skill involves:
- Analyzing problems systematically
- Developing step-by-step solutions
- Optimizing algorithms for performance
- Considering edge cases and potential issues
Platforms like AlgoCademy focus on nurturing these skills through interactive coding tutorials and challenges that emphasize algorithmic thinking.
2. Data Structures and Algorithms
A solid understanding of data structures and algorithms is fundamental to writing efficient and scalable code. This knowledge helps you:
- Choose the right data structure for specific problems
- Implement and optimize common algorithms
- Analyze the time and space complexity of your solutions
- Design more efficient systems and applications
Many technical interviews, especially at major tech companies, focus heavily on these concepts. Mastering them is essential for career advancement.
3. Code Organization and Design Patterns
Writing clean, maintainable, and scalable code is a skill that transcends specific languages or frameworks. Key aspects include:
- Following SOLID principles and other best practices
- Implementing appropriate design patterns
- Creating modular and reusable code
- Writing clear and concise documentation
These skills ensure that your code remains manageable as projects grow and evolve.
4. Debugging and Troubleshooting
The ability to efficiently identify and fix issues in code is invaluable. Strong debugging skills involve:
- Systematic approach to isolating problems
- Effective use of debugging tools and techniques
- Root cause analysis
- Implementing robust error handling and logging
These skills are crucial for maintaining and improving existing codebases, a common task in many development roles.
5. Version Control and Collaboration
Modern software development is a collaborative effort. Proficiency in version control systems (like Git) and collaborative workflows is essential. This includes:
- Managing code repositories effectively
- Creating meaningful commits and pull requests
- Resolving merge conflicts
- Conducting code reviews
These skills facilitate smooth teamwork and are vital in professional development environments.
The Role of Trends in Skill Development
While core skills should be the primary focus, it’s important to recognize that trends and new technologies do have their place in a developer’s journey. They can:
- Introduce new paradigms and ways of thinking about problems
- Provide solutions to specific challenges in software development
- Offer opportunities to apply core skills in new contexts
- Keep developers engaged and excited about their work
The key is to approach trends with a discerning eye and evaluate them based on their potential to enhance your core skills and solve real-world problems.
Balancing Trends and Core Skills
To strike a balance between staying current and developing fundamental skills, consider the following strategies:
1. Prioritize Core Skills First
Ensure you have a solid foundation in the fundamental skills mentioned earlier. Platforms like AlgoCademy offer structured learning paths that focus on these essential competencies.
2. Evaluate Trends Critically
Before diving into a new technology, ask yourself:
- How does this align with my career goals?
- Will it solve problems I’m currently facing in my work?
- Is it likely to have long-term relevance in the industry?
- Can I apply my core skills while learning this new technology?
3. Learn Concepts, Not Just Syntax
When exploring new technologies, focus on understanding the underlying concepts and principles rather than just memorizing syntax. This approach makes it easier to adapt to future changes and apply your knowledge across different tools.
4. Practice with Real Projects
Apply new technologies to actual projects or problems you’re working on. This hands-on approach helps you understand the practical implications and limitations of new tools.
5. Stay Informed, But Be Selective
Keep an eye on industry trends, but be selective about which ones you invest time in learning. Focus on those that align with your career path and interests.
6. Continuous Learning of Fundamentals
Regularly revisit and deepen your understanding of core concepts. Even experienced developers can benefit from refreshing their knowledge of fundamental algorithms and data structures.
The Long-Term Perspective
In the grand scheme of a developer’s career, the ability to adapt to new technologies is important, but it’s the mastery of core skills that truly sets exceptional programmers apart. These fundamental competencies provide the foundation upon which all other skills are built.
Consider the following scenario:
// Example: Implementing a feature using a trendy framework vs. fundamental skills
// Trendy Framework Approach
import TrendyFramework from 'trendy-framework';
const MyComponent = () => {
const [data, setData] = useState([]);
useEffect(() => {
TrendyFramework.fetchData().then(setData);
}, []);
return (
<TrendyFramework.List data={data} />
);
};
// Fundamental Skills Approach
class DataStructure {
constructor() {
this.data = [];
}
insert(item) {
// Implement efficient insertion algorithm
}
remove(item) {
// Implement efficient removal algorithm
}
search(item) {
// Implement efficient search algorithm
}
}
function processData(rawData) {
const dataStructure = new DataStructure();
for (const item of rawData) {
// Apply problem-solving skills to determine how to process and store the data
dataStructure.insert(processItem(item));
}
return dataStructure;
}
function displayData(dataStructure) {
// Implement efficient rendering logic
}
// Main application logic
fetch('https://api.example.com/data')
.then(response => response.json())
.then(rawData => {
const processedData = processData(rawData);
displayData(processedData);
})
.catch(error => {
console.error('Error fetching data:', error);
// Implement robust error handling
});
In this example, while the trendy framework approach might seem more concise and easier to implement initially, the fundamental skills approach demonstrates a deeper understanding of data structures, algorithms, and problem-solving. This approach is likely to be more performant, flexible, and easier to maintain in the long run.
The Role of Platforms Like AlgoCademy
In the context of balancing trends and core skills, platforms like AlgoCademy play a crucial role. They provide:
- Structured learning paths focusing on fundamental programming concepts
- Interactive coding challenges that reinforce algorithmic thinking
- Resources for preparing for technical interviews at major tech companies
- AI-powered assistance to guide learners through complex problem-solving processes
By emphasizing these core competencies, AlgoCademy helps developers build a strong foundation that enables them to adapt more easily to new trends and technologies as they emerge.
Conclusion: Finding Your Balance
While staying informed about industry trends is important, it shouldn’t come at the expense of developing and honing your core programming skills. The most successful developers are those who can leverage a strong foundation in fundamental concepts to quickly adapt to and evaluate new technologies.
Remember:
- Focus on building a solid foundation in problem-solving, data structures, algorithms, and software design principles.
- Approach new trends with a critical eye, evaluating their potential value and alignment with your goals.
- Use platforms like AlgoCademy to continuously reinforce and expand your core skills.
- Apply new technologies in the context of real projects to gain practical experience.
- Strive for a balance between staying current and deepening your fundamental knowledge.
By maintaining this balance, you’ll position yourself as a versatile and valuable developer capable of tackling complex challenges regardless of the specific tools or frameworks involved. Remember, in the ever-changing landscape of technology, your ability to learn, adapt, and solve problems will always be your most valuable asset.