Why “Move Fast and Break Things” Hurts Code Quality
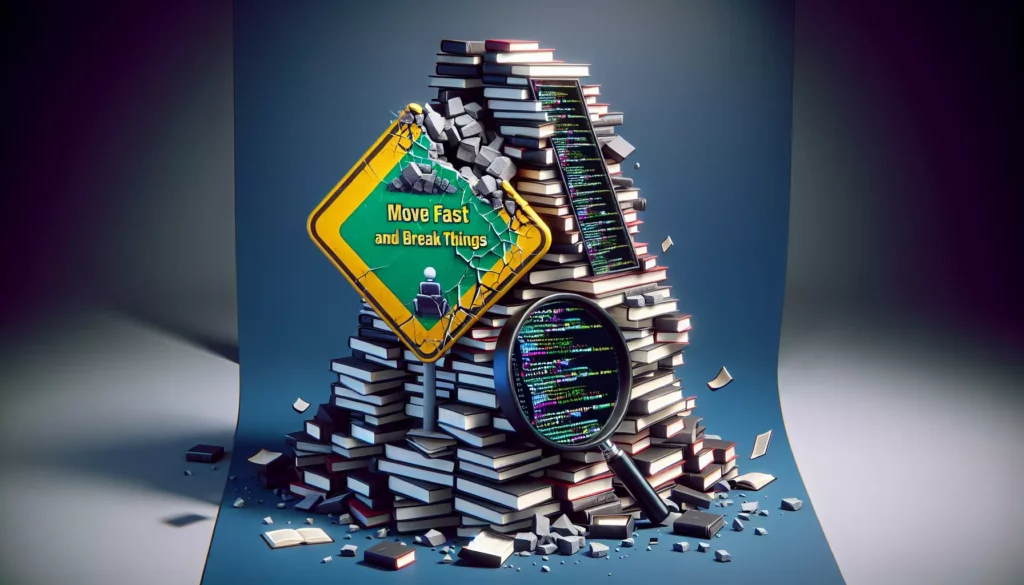
In the fast-paced world of software development, the mantra “Move Fast and Break Things” has gained significant traction, especially among startups and tech giants aiming for rapid growth and innovation. This philosophy, popularized by Facebook’s early days, encourages developers to prioritize speed and experimentation over caution and perfection. While this approach can lead to quick product iterations and market advantages, it often comes at the cost of code quality, technical debt, and long-term sustainability. In this article, we’ll explore why this mindset can be detrimental to code quality and discuss alternative approaches that balance speed with maintainability.
The Origins of “Move Fast and Break Things”
Before diving into the drawbacks of this philosophy, it’s essential to understand its origins and initial purpose. Mark Zuckerberg, Facebook’s co-founder and CEO, introduced this motto in the company’s early days to encourage rapid innovation and risk-taking. The idea was to prioritize quick development cycles and frequent releases, even if it meant occasional bugs or errors.
At its core, the philosophy aimed to:
- Encourage innovation and experimentation
- Reduce fear of failure among developers
- Accelerate product development and feature releases
- Gain market share quickly in a competitive landscape
While these goals are admirable, the long-term consequences of this approach on code quality and maintainability have become increasingly apparent over time.
The Impact on Code Quality
When developers are encouraged to prioritize speed over quality, several issues can arise that negatively impact code quality:
1. Accumulation of Technical Debt
Technical debt refers to the implied cost of additional rework caused by choosing an easy (limited) solution now instead of using a better approach that would take longer. When teams move fast without considering long-term implications, they often accumulate technical debt rapidly. This debt can manifest in various ways:
- Poorly structured code that’s difficult to maintain
- Lack of proper documentation
- Inconsistent coding standards
- Suboptimal architectural decisions
Over time, this technical debt can slow down development, increase the likelihood of bugs, and make it challenging to add new features or scale the application.
2. Inadequate Testing
When speed is the primary focus, comprehensive testing often takes a backseat. This can lead to:
- Insufficient unit tests
- Lack of integration and end-to-end tests
- Overlooked edge cases
- Reduced test coverage
Without proper testing, bugs are more likely to slip into production, potentially causing issues for users and damaging the product’s reputation.
3. Neglected Code Reviews
Code reviews are crucial for maintaining code quality, sharing knowledge, and catching potential issues early. However, in a “move fast” environment, thorough code reviews may be rushed or skipped entirely. This can result in:
- Inconsistent coding styles
- Missed opportunities for optimization
- Overlooked security vulnerabilities
- Reduced knowledge sharing among team members
4. Shortcuts and Quick Fixes
When developers are pressured to deliver quickly, they may resort to taking shortcuts or implementing quick fixes instead of robust, scalable solutions. This can lead to:
- Hardcoded values instead of configurable parameters
- Duplicate code instead of reusable components
- Ignoring best practices and design patterns
- Prioritizing immediate functionality over long-term maintainability
5. Inadequate Documentation
In the rush to ship code, documentation often becomes an afterthought. This can cause problems such as:
- Difficulty onboarding new team members
- Challenges in maintaining and updating the codebase
- Increased time spent understanding existing code
- Reduced collaboration and knowledge sharing
Real-World Consequences
The negative impact of prioritizing speed over quality isn’t just theoretical. Many companies have faced significant consequences due to this approach. Let’s look at a few notable examples:
1. Therac-25 Radiation Therapy Machine
While not a direct result of the “Move Fast and Break Things” philosophy, the Therac-25 incident illustrates the dangers of rushing software development in critical systems. This radiation therapy machine, developed in the 1980s, had a software bug that resulted in patients receiving massive overdoses of radiation. The root cause was traced back to poor software design and inadequate testing.
2. Knight Capital’s $460 Million Loss
In 2012, Knight Capital, a financial services firm, lost $460 million in just 45 minutes due to a software glitch. The issue stemmed from a combination of old code that wasn’t properly removed and new code that wasn’t adequately tested. This incident highlights the importance of thorough testing and careful code management, especially in high-stakes environments.
3. Zoom’s Security Issues
As Zoom’s popularity skyrocketed during the COVID-19 pandemic, several security vulnerabilities came to light. These issues, including “Zoombombing” and weak encryption, were partly attributed to the company’s focus on ease of use and rapid growth at the expense of security. Zoom had to pause feature development for 90 days to address these concerns, demonstrating the long-term costs of prioritizing speed over security and quality.
Balancing Speed and Quality
While the “Move Fast and Break Things” approach has its drawbacks, the need for speed in software development is undeniable. The key is to find a balance between rapid development and maintaining code quality. Here are some strategies to achieve this balance:
1. Adopt Agile Methodologies
Agile methodologies, when implemented correctly, can help teams move quickly while maintaining quality. Key practices include:
- Short, focused sprints
- Regular retrospectives to identify and address issues
- Continuous integration and delivery (CI/CD)
- Emphasis on sustainable development practices
2. Implement Automated Testing
Automated testing allows teams to move quickly while ensuring code quality. This includes:
- Unit tests for individual components
- Integration tests for interactions between components
- End-to-end tests for full user scenarios
- Performance and security tests
By integrating these tests into the CI/CD pipeline, teams can catch issues early and deploy with confidence.
3. Prioritize Code Reviews
Code reviews are crucial for maintaining code quality and sharing knowledge. To make them effective without slowing down development:
- Use pull requests for all code changes
- Implement automated code quality checks
- Encourage constructive feedback and knowledge sharing
- Set clear expectations for review turnaround times
4. Invest in Architecture and Design
Taking the time to design a solid architecture upfront can save significant time and effort in the long run. This includes:
- Defining clear interfaces and abstractions
- Using design patterns appropriate for the problem domain
- Planning for scalability and maintainability
- Regularly reviewing and refining the architecture
5. Embrace Technical Debt Management
While some technical debt is inevitable, it’s crucial to manage it actively:
- Regularly allocate time for refactoring and paying down technical debt
- Use tools to track and visualize technical debt
- Prioritize addressing high-impact technical debt
- Educate stakeholders on the importance of managing technical debt
6. Foster a Culture of Quality
Building a culture that values quality is essential for long-term success:
- Encourage developers to take pride in their work
- Recognize and reward efforts to improve code quality
- Provide training and resources for best practices
- Lead by example, with senior developers and managers emphasizing quality
Alternative Philosophies
As the software industry has matured, several alternative philosophies have emerged that aim to balance speed with quality:
1. “Move Fast with Stable Infra”
Facebook itself has evolved its motto to “Move Fast with Stable Infra.” This approach emphasizes building robust infrastructure and tools that enable rapid development without compromising stability. Key aspects include:
- Investing in developer tools and automation
- Building scalable and reliable infrastructure
- Implementing robust testing and deployment pipelines
- Focusing on long-term sustainability alongside rapid innovation
2. “Make It Work, Make It Right, Make It Fast”
This philosophy, often attributed to Kent Beck, suggests a staged approach to development:
- Make It Work: Focus on creating a functional solution
- Make It Right: Refactor and improve the code quality
- Make It Fast: Optimize for performance
This approach allows for rapid initial development while ensuring that the final product is of high quality and performs well.
3. “Shift Left”
The “Shift Left” approach emphasizes moving quality assurance and testing earlier in the development process. This includes:
- Incorporating security and performance considerations from the start
- Implementing continuous testing throughout the development cycle
- Encouraging developers to write and run tests as they code
- Using static code analysis tools to catch issues early
Practical Tips for Maintaining Code Quality
To help developers and teams maintain code quality while still moving quickly, here are some practical tips:
1. Use Linters and Formatters
Linters and code formatters can automatically catch and fix many common issues, ensuring consistent code style and preventing simple errors. For example, in a JavaScript project, you might use ESLint and Prettier:
// Install ESLint and Prettier
npm install --save-dev eslint prettier
// Add a script to your package.json
"scripts": {
"lint": "eslint 'src/**/*.js'",
"format": "prettier --write 'src/**/*.js'"
}
// Run linting and formatting
npm run lint
npm run format
2. Implement Pre-commit Hooks
Use pre-commit hooks to run linters, formatters, and tests before allowing commits. This ensures that only code meeting certain quality standards makes it into the repository. You can use tools like Husky for this:
// Install Husky
npm install --save-dev husky
// Add a pre-commit hook in your package.json
"husky": {
"hooks": {
"pre-commit": "npm run lint && npm run test"
}
}
3. Use Type Checking
For languages that support it, use static type checking to catch errors early and improve code clarity. For example, in JavaScript, you can use TypeScript:
// Example TypeScript code
function greet(name: string): string {
return `Hello, ${name}!`;
}
// This will cause a compile-time error
greet(123); // Argument of type 'number' is not assignable to parameter of type 'string'.
4. Write Self-Documenting Code
While formal documentation is important, writing clear, self-documenting code can significantly improve maintainability. This includes using meaningful variable and function names, keeping functions small and focused, and using comments judiciously:
// Bad example
function f(x) {
return x * 86400000;
}
// Good example
function convertDaysToMilliseconds(days) {
const MILLISECONDS_PER_DAY = 86400000;
return days * MILLISECONDS_PER_DAY;
}
5. Practice Test-Driven Development (TDD)
TDD can help ensure code quality by requiring you to think about requirements and edge cases upfront. Here’s a simple example using Jest:
// calculator.test.js
const calculator = require('./calculator');
test('adds 1 + 2 to equal 3', () => {
expect(calculator.add(1, 2)).toBe(3);
});
// calculator.js
const calculator = {
add: (a, b) => a + b,
};
module.exports = calculator;
6. Regularly Refactor Code
Set aside time for regular refactoring to improve code quality and reduce technical debt. This might involve extracting reusable components, simplifying complex functions, or updating outdated patterns:
// Before refactoring
function processUser(user) {
if (user.age >= 18) {
if (user.country === 'US') {
// Process US adult
} else {
// Process non-US adult
}
} else {
// Process minor
}
}
// After refactoring
function processUser(user) {
if (isMinor(user)) {
processMinor(user);
} else if (isUSAdult(user)) {
processUSAdult(user);
} else {
processNonUSAdult(user);
}
}
function isMinor(user) {
return user.age < 18;
}
function isUSAdult(user) {
return user.age >= 18 && user.country === 'US';
}
// ... implement processMinor, processUSAdult, and processNonUSAdult functions
Conclusion
While the “Move Fast and Break Things” philosophy has its place in certain contexts, it’s crucial to recognize its potential negative impact on code quality. As the software industry matures, we’re seeing a shift towards more balanced approaches that prioritize both speed and quality.
By adopting practices like automated testing, continuous integration, regular code reviews, and active technical debt management, teams can move quickly while still maintaining high code quality. Remember, the goal isn’t just to ship features fast, but to build sustainable, maintainable software that can evolve with changing requirements and scale as needed.
Ultimately, the most successful teams are those that find the right balance between speed and quality, creating a culture that values both innovation and craftsmanship. By doing so, they can build software that not only meets immediate needs but stands the test of time.