Why “Fake It Till You Make It” Doesn’t Work in Coding
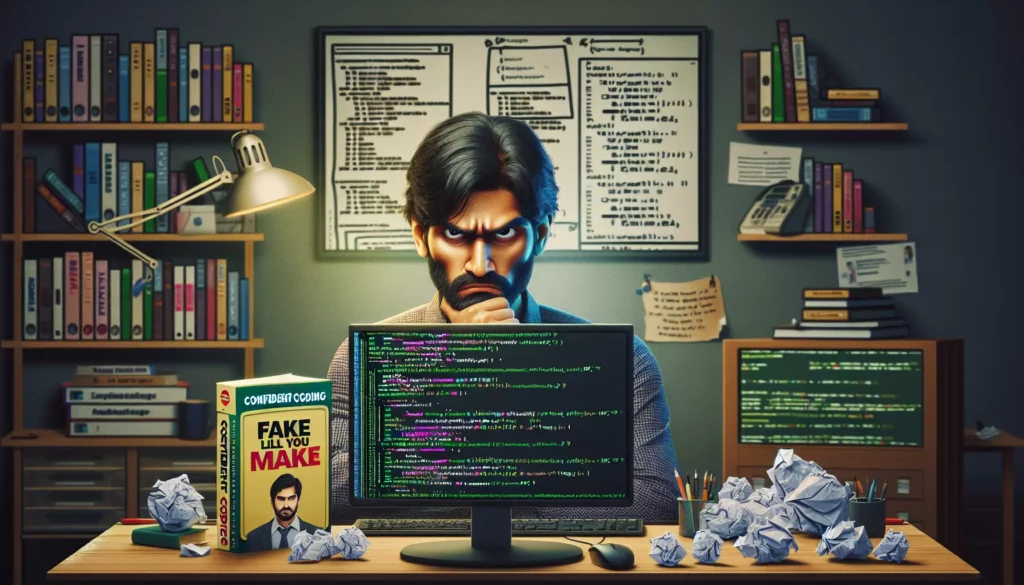
In the world of professional development, we often hear the phrase “fake it till you make it.” This mantra suggests that by pretending to have certain skills or knowledge, we can eventually acquire them through experience. While this approach might work in some fields, when it comes to coding and software development, it’s a strategy that can lead to disastrous consequences. In this article, we’ll explore why the “fake it till you make it” mindset is not only ineffective but potentially harmful in the coding world, and what aspiring programmers should do instead.
The Allure of “Fake It Till You Make It”
Before we dive into why this approach doesn’t work in coding, let’s understand why it’s so tempting in the first place:
- Rapid Industry Growth: The tech industry is booming, with a constant demand for skilled programmers. This can create pressure to appear more competent than one actually is.
- Imposter Syndrome: Many developers, even experienced ones, suffer from imposter syndrome, feeling like they don’t belong or aren’t as skilled as their peers.
- Complex Technologies: The vast array of programming languages, frameworks, and tools can be overwhelming, leading some to exaggerate their proficiency.
- Competitive Job Market: With high salaries and attractive perks, there’s intense competition for coding jobs, tempting some to oversell their abilities.
Why “Fake It Till You Make It” Fails in Coding
While the idea of faking it might seem like a shortcut to success, it’s particularly ill-suited to the field of programming. Here’s why:
1. Code Doesn’t Lie
Unlike some professions where results can be subjective or open to interpretation, code either works or it doesn’t. There’s no gray area when it comes to a program compiling and functioning as intended. You can’t fake your way through a coding task – the outcome is binary: success or failure.
// This code either works or it doesn't
function addNumbers(a, b) {
return a + b;
}
console.log(addNumbers(5, 3)); // Output: 8
console.log(addNumbers("5", "3")); // Output: "53" (string concatenation)
In the example above, the function works as expected with numbers but produces unexpected results with strings. There’s no way to fake the correct implementation – it either does what it’s supposed to do, or it doesn’t.
2. Technical Debt Accumulates Quickly
When developers pretend to understand concepts they don’t, they often create solutions that are suboptimal, inefficient, or downright incorrect. This leads to technical debt – the implied cost of additional rework caused by choosing an easy solution now instead of using a better approach that would take longer.
Technical debt compounds over time, making codebases increasingly difficult to maintain and extend. What starts as a small “faked” solution can snowball into a major problem that affects the entire project.
3. Coding Requires Continuous Learning
The field of software development is constantly evolving. New languages, frameworks, and best practices emerge regularly. Pretending to know everything is not only impossible but also counterproductive. Successful developers embrace a growth mindset and are always ready to learn.
Faking knowledge closes you off from learning opportunities and can stunt your growth as a developer. It’s much more valuable to admit what you don’t know and use that as motivation to improve.
4. Collaboration Is Key in Software Development
Modern software development is a team sport. Developers work in teams, participate in code reviews, and often pair program. Pretending to have skills you lack will quickly become apparent in these collaborative environments, eroding trust with your colleagues and potentially damaging your professional reputation.
5. Debugging Requires Deep Understanding
When things go wrong (and they will), developers need to be able to debug their code effectively. This requires a thorough understanding of the underlying principles and technologies. You can’t fake your way through debugging – it demands real knowledge and problem-solving skills.
// Debugging requires understanding
function calculateAverage(numbers) {
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum / numbers.length;
}
console.log(calculateAverage([1, 2, 3, 4, 5])); // Output: 3
console.log(calculateAverage([])); // Output: NaN
In this example, the function works fine for non-empty arrays but returns NaN for an empty array. Debugging and fixing this edge case requires understanding of how division by zero works in JavaScript and how to handle empty arrays properly.
6. Security Implications
In the world of coding, ignorance isn’t just inconvenient – it can be dangerous. Developers who don’t fully understand security best practices can inadvertently create vulnerabilities in their code, potentially exposing sensitive data or allowing malicious attacks.
// Insecure code due to lack of understanding
const userInput = req.body.userInput;
const query = `SELECT * FROM users WHERE id = ${userInput}`;
db.query(query, (err, result) => {
// Process result
});
This code is vulnerable to SQL injection attacks. A developer who doesn’t understand the importance of parameterized queries might write code like this, potentially compromising the entire database.
7. The Industry Values Authenticity
Contrary to what the “fake it till you make it” philosophy suggests, the tech industry generally values authenticity and honesty. Admitting that you don’t know something and showing a willingness to learn is often more appreciated than pretending to have all the answers.
What to Do Instead of Faking It
Now that we’ve established why faking it doesn’t work in coding, let’s explore some positive alternatives:
1. Embrace a Growth Mindset
Instead of pretending to know everything, adopt a growth mindset. Be open about what you don’t know and frame it as an opportunity to learn and improve. This approach not only helps you grow as a developer but also earns respect from colleagues who appreciate honesty and eagerness to learn.
2. Focus on Fundamentals
Rather than trying to learn every new framework or tool superficially, focus on mastering the fundamentals of programming. Strong foundational knowledge in areas like algorithms, data structures, and design patterns will serve you well throughout your career, regardless of the specific technologies you work with.
// Understanding fundamentals helps in problem-solving
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
console.log(binarySearch([1, 3, 5, 7, 9], 5)); // Output: 2
console.log(binarySearch([1, 3, 5, 7, 9], 6)); // Output: -1
Understanding algorithms like binary search is more valuable in the long run than memorizing the syntax of a particular framework.
3. Practice, Practice, Practice
There’s no substitute for hands-on coding experience. Regularly work on personal projects, contribute to open-source initiatives, or solve coding challenges on platforms like LeetCode or HackerRank. The more you code, the more comfortable and proficient you’ll become.
4. Seek Mentorship and Feedback
Find mentors or more experienced developers who can guide you and provide constructive feedback. Many developers are happy to share their knowledge and experiences. Don’t be afraid to ask questions or request code reviews – this is how you learn and improve.
5. Stay Current with Industry Trends
While you can’t know everything, staying informed about current trends and emerging technologies in your field is crucial. Follow tech blogs, attend conferences or webinars, and participate in coding communities. This knowledge will help you make informed decisions about what to learn next.
6. Build a Strong Portfolio
Instead of exaggerating your skills, let your work speak for itself. Build a portfolio of projects that demonstrate your abilities and growth over time. This tangible evidence of your skills is far more valuable than any claims you might make about your expertise.
7. Cultivate Soft Skills
Technical skills are crucial, but don’t neglect soft skills like communication, teamwork, and problem-solving. These skills are highly valued in the tech industry and can set you apart from other candidates who may have similar technical abilities.
The Role of Platforms Like AlgoCademy
In the journey from beginner to proficient coder, platforms like AlgoCademy play a crucial role. Instead of encouraging learners to fake their abilities, AlgoCademy provides a structured path to genuine skill development:
- Interactive Tutorials: Step-by-step guidance helps learners build a solid foundation in coding concepts.
- Focus on Algorithmic Thinking: By emphasizing problem-solving skills, AlgoCademy prepares learners for real-world coding challenges.
- AI-Powered Assistance: Personalized feedback and hints help learners overcome obstacles without resorting to “faking it.”
- Preparation for Technical Interviews: Practice with interview-style questions builds genuine confidence and competence.
Platforms like AlgoCademy encourage a growth mindset by providing a safe space to learn, make mistakes, and improve. This approach aligns perfectly with the idea that in coding, it’s better to be honest about your current skill level and work on improving, rather than pretending to know more than you do.
Real-World Success Stories
To illustrate the benefits of genuine learning over faking it, let’s look at some real-world success stories:
Case Study 1: The Self-Taught Developer
Sarah, a history major, decided to switch careers to web development. Instead of falsely claiming coding expertise, she was upfront about her beginner status. She dedicated time to learning fundamentals through online courses and building projects. Her GitHub portfolio, showcasing her learning journey, impressed employers more than any exaggerated claims could have. She landed a junior developer role and quickly progressed due to her strong foundation and eagerness to learn.
Case Study 2: The Honest Intern
During his internship, Alex was assigned a task involving a technology he wasn’t familiar with. Instead of pretending to know it, he admitted his lack of experience but expressed enthusiasm to learn. His supervisor appreciated his honesty and paired him with a senior developer for mentorship. By the end of the internship, Alex had not only completed the project successfully but had also gained a valuable skill and a strong recommendation for future employment.
Case Study 3: The Collaborative Team Player
Emma joined a startup as a mid-level developer. During team discussions, she wasn’t afraid to ask questions or admit when she didn’t understand something. This openness fostered a culture of knowledge sharing within the team. Her colleagues respected her honesty and willingness to learn, often seeking her input on problems. Emma’s approach led to faster problem-solving and a more cohesive team dynamic.
The Long-Term Benefits of Authenticity in Coding
Choosing authenticity over “faking it” in your coding journey offers numerous long-term benefits:
1. Sustainable Career Growth
By focusing on genuine skill development, you build a solid foundation for long-term career growth. This approach allows you to take on increasingly complex projects with confidence, leading to natural career progression.
2. Respect from Peers and Employers
Honesty about your skills and limitations earns respect from colleagues and employers. This respect can lead to better opportunities, as people are more likely to recommend or hire someone they trust.
3. Reduced Stress and Improved Job Satisfaction
Pretending to have skills you lack is stressful and can lead to burnout. Being authentic allows you to enjoy your work more, as you’re not constantly worried about being “found out.”
4. Better Learning Opportunities
When you’re honest about what you don’t know, you open yourself up to learning opportunities. Colleagues and mentors are more likely to share knowledge with someone who shows a genuine desire to learn.
5. Stronger Problem-Solving Skills
Tackling challenges honestly helps develop robust problem-solving skills. Instead of relying on quick fixes or copied solutions, you learn to think critically and develop innovative solutions.
6. Improved Code Quality
Writing code you truly understand leads to higher quality work. This not only benefits your current projects but also builds a reputation for reliability and excellence.
7. Adaptability in a Changing Field
The tech industry is constantly evolving. By cultivating a genuine learning mindset, you become more adaptable to new technologies and methodologies, ensuring long-term relevance in your career.
Conclusion
In the world of coding, the “fake it till you make it” approach is not just ineffective – it’s counterproductive and potentially harmful. The nature of programming demands genuine knowledge, continuous learning, and honest collaboration. Instead of pretending to have skills you lack, focus on building a solid foundation, embracing a growth mindset, and being authentic about your journey as a developer.
Remember, every expert was once a beginner. What sets successful developers apart is not their ability to fake expertise, but their commitment to genuine learning and improvement. By choosing authenticity over pretense, you not only become a better coder but also contribute to a healthier, more collaborative coding community.
Platforms like AlgoCademy play a crucial role in this journey, offering structured learning paths that encourage genuine skill development. By leveraging such resources and maintaining an honest approach to your coding education, you set yourself up for long-term success in the ever-evolving world of software development.
In the end, the most valuable asset you can bring to your coding career is not a façade of knowledge, but a genuine passion for learning and problem-solving. Embrace the challenge, be honest about where you are in your journey, and commit to continuous improvement. That’s the true path to becoming a skilled and respected developer in the competitive world of coding.