Ace Your Next Tech Interview/ 50 Common Coding Questions and Answers
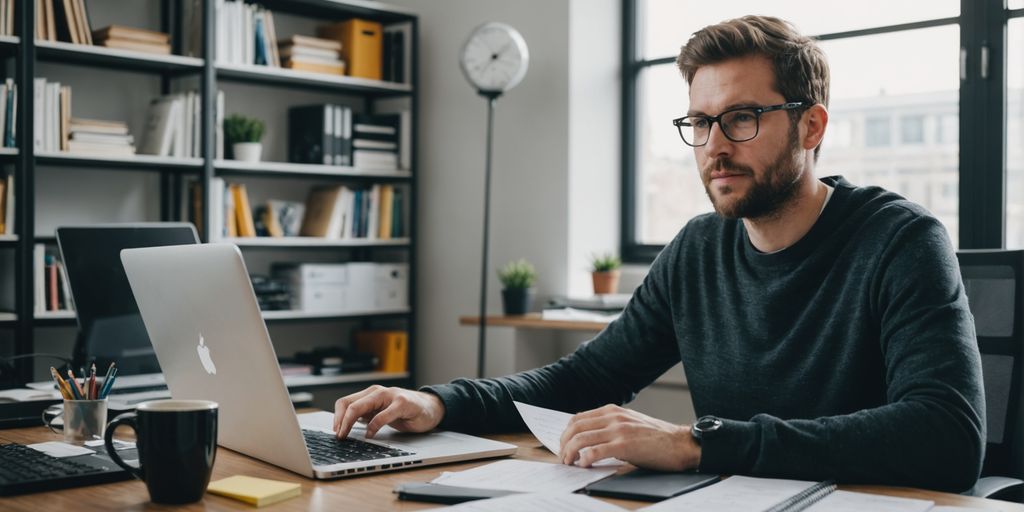
Preparing for a tech interview can be tough, especially with the many coding questions you might face. We talked to 50 developers who recently got jobs to find out the hardest questions they were asked. This article will help you get ready by covering 50 common coding questions and their answers. Whether you’re dealing with arrays, linked lists, or dynamic programming, we’ve got you covered.
Key Takeaways
- Tech interviews are essential for assessing your coding and problem-solving skills.
- Common topics include arrays, linked lists, and sorting algorithms.
- Practice coding problems to improve your chances of success.
- Understanding data structures and algorithms is crucial.
- Soft skills like communication are also important.
Arrays
Arrays are a fundamental data structure in computer science, often used to store collections of elements. They are essential for many coding problems and are frequently tested in technical interviews. Mastering arrays can significantly boost your chances of acing your next tech interview. Here are some common coding questions on arrays:
- Given an unordered array of integers, write a program that finds a contiguous subarray whose sum is equal to a given value.
- For two unsorted arrays, write a code to merge them into a new array in ascending order.
- Given an array with values from 1 to N and another array with elements at every position in the first array, find the position of the missing element.
- Write a code to compute the sum of elements in a given array of positive integers.
- Rotate an unsorted array of size N by D elements anticlockwise.
- Determine a code to print the original sequence for a given sequence of size N.
- Write a function to locate and delete duplicate elements from an ordered array.
- For a given 2D array of integers, find the minimum and maximum lengths.
Arrays are versatile and can be used to solve a wide range of problems, making them a crucial topic for any coding interview preparation.
Linked Lists
Linked lists are a common topic in tech interviews, especially at top companies. Here are some sample questions to get you started:
- How to insert a node at the end of a Linked List?
- How do you remove a particular node from a Linked List?
- Write a function to reverse a singly linked list.
- Add two numbers represented by linked lists.
- Write a recursive function to remove the nth node from a Linked List.
- Write a function to move nodes in a linked list by swapping adjacent nodes.
- Write a code that will invert the values of an array at a specific point.
Mastering these questions can significantly boost your confidence and performance in interviews.
Stacks
Stacks are a fundamental data structure in computer science, often used in various algorithms and systems. They follow the Last In, First Out (LIFO) principle, meaning the last element added is the first one to be removed.
Common Operations
- Push: Add an element to the top of the stack.
- Pop: Remove the top element from the stack.
- Peek: View the top element without removing it.
- IsEmpty: Check if the stack is empty.
Coding Questions
Here are some common coding questions related to stacks that you might encounter in a tech interview:
- Implement a stack using arrays or linked lists.
- Design a stack that supports push, pop, and retrieving the minimum element in constant time.
- Evaluate a postfix expression using a stack.
- Check for balanced parentheses in an expression.
- Implement a stack using two queues.
Tip: Always ask clarifying questions before jumping into coding. This helps in understanding the problem better and avoids incorrect assumptions.
Real-World Applications
- Function Call Management: Stacks are used to manage function calls in programming languages.
- Expression Evaluation: They are used in evaluating expressions and syntax parsing.
- Backtracking Algorithms: Stacks help in implementing backtracking algorithms like solving mazes or puzzles.
Understanding stacks and their applications can significantly improve your problem-solving skills in tech interviews. In-depth full-stack developer questions often include scenarios where you need to use stacks effectively.
Queues
Queues are a fundamental data structure in computer science, often used in various applications like task scheduling and breadth-first search algorithms. A queue follows the First-In-First-Out (FIFO) principle, meaning the first element added is the first one to be removed.
Common Operations
- Enqueue: Adding an element to the end of the queue.
- Dequeue: Removing the front element from the queue.
- Peek/Front: Retrieving the front element without removing it.
- IsEmpty: Checking if the queue is empty.
Types of Queues
- Simple Queue: Basic FIFO queue.
- Circular Queue: The last position is connected back to the first position to make a circle.
- Priority Queue: Elements are dequeued based on their priority, not just their order in the queue. This is a commonly asked data structure interview question.
- Double-Ended Queue (Deque): Elements can be added or removed from both ends.
Queues are essential for managing tasks in a sequential manner, ensuring that each task is processed in the order it was received.
Trees
Trees are a fundamental data structure in computer science, often used to represent hierarchical data. Mastering common binary tree interview questions is crucial for acing tech interviews.
Common Binary Tree Questions
- Maximum Path Sum: Given a binary tree where each node contains a positive integer, find the maximum sum that can be found over a path from one node to another.
- Height Calculation: For a given binary tree, calculate its height.
- Lowest Common Ancestor: For any given binary tree with unique values, write code to find the lowest common ancestor of two nodes.
- Vertical Distance Connection: How to connect nodes that have the same vertical distance.
- Level-Order Traversal: For a given binary tree, write a function to find its level-order traversal.
- Vertical Traversal: Write a program to trace the vertical traversal of a given binary tree.
- Bottom View: Write a program to print the bottom view of a binary search tree as you scroll from left to right.
- Binary Search Tree Check: Given the root of a binary tree, write a program to determine if it is a binary search tree.
Trees and Graphs are crucial topics for tech interviews. Here are some sample coding interview questions to help you prepare.
Graphs
Graphs are a fundamental topic in tech interviews, especially for companies like Amazon. Here are some common questions you might encounter:
- Find the shortest path between two nodes in an unweighted graph.
- Implement a function to detect a cycle in a directed graph.
- Write a program to find the connected components in an undirected graph.
- Given a graph, determine if it is bipartite.
- For a given graph, find the minimum spanning tree using Kruskal’s or Prim’s algorithm.
- Implement Dijkstra’s algorithm to find the shortest path in a weighted graph.
- Write a function to perform a topological sort on a directed acyclic graph.
- Given a graph, find all articulation points (or cut vertices).
- Implement the Floyd-Warshall algorithm to find shortest paths between all pairs of nodes.
- Write a program to find the strongly connected components in a directed graph.
Graph problems can be tricky, but with practice, you can master them. Remember, the key is to understand the underlying concepts and practice regularly.
Hash Tables
Hash tables are a fundamental data structure used to store key-value pairs. They offer O(1) average time complexity for search, insert, and delete operations, making them highly efficient for many applications.
Common Hash Table Operations
- Insertion: Adding a key-value pair to the hash table.
- Deletion: Removing a key-value pair from the hash table.
- Search: Finding the value associated with a given key.
Collision Handling Techniques
- Chaining: Uses a list to store multiple values that hash to the same index.
- Open Addressing: Finds another open slot within the hash table.
Applications of Hash Tables
- Caching: Storing frequently accessed data for quick retrieval.
- Database Indexing: Speeding up data retrieval in databases.
- Sets: Implementing set operations like union and intersection efficiently.
Hash tables are versatile and can be optimized for both speed and memory usage, making them a go-to choice for many software engineers.
Optimizing Hash Tables
When optimizing hash tables, consider the trade-offs between speed, memory usage, and future maintainability. For example, if memory is a constraint, you might use a smaller set or array instead of an unbounded set. On the other hand, if speed is crucial, a hash table with O(1) lookup time is ideal.
Example Interview Questions
- Find whether an array is a subset of another array.
- Union and intersection of two linked lists.
- Find a pair of numbers that add up to a given sum using a hash table.
These questions often appear in top 20 hashing technique based interview questions lists and are essential for mastering hash tables.
Sorting Algorithms
Sorting algorithms are used to rearrange a given array or list of elements according to a comparison operator on the elements. These algorithms are fundamental in computer science and are often asked in technical interviews. Understanding different sorting algorithms and their complexities is crucial for optimizing your solutions.
Recursion
Recursion is a method where the solution to a problem depends on solutions to smaller instances of the same problem. It’s a powerful tool in coding interviews.
Common Recursion Problems
- Fibonacci Series: Write a function to print a Fibonacci series using recursion.
- Factorial Calculation: Create a recursive function to find the factorial of a number.
- String Reversal: Develop a recursive function to reverse a string.
- Sum of Digits: Write a recursive function to find the sum of digits of a number.
- Tower of Hanoi: Solve the Tower of Hanoi problem using recursion.
Tips for Solving Recursion Problems
- Base Case: Always define a base case to stop the recursion.
- Recursive Case: Ensure each recursive call reduces the problem size.
- Stack Overflow: Be cautious of stack overflow errors in deep recursion.
Recursion can simplify complex problems by breaking them down into smaller, more manageable parts. However, it’s crucial to understand the base and recursive cases to avoid infinite loops.
Example: Fibonacci Series
Here’s a simple example to print a Fibonacci series using recursion:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
This function calls itself to calculate the Fibonacci number, demonstrating the power of recursion.
Dynamic Programming
Dynamic Programming (DP) is a method for solving complex problems by breaking them down into simpler subproblems. It is particularly useful for optimization problems where the solution can be constructed from solutions to subproblems.
Key Concepts
- Overlapping Subproblems: Many subproblems are solved multiple times. DP solves each subproblem once and stores the result.
- Optimal Substructure: The optimal solution to the problem can be constructed from optimal solutions of its subproblems.
Common DP Problems
Here are some of the most important dynamic programming problems asked in various technical interviews:
- Longest Common Subsequence: Find the longest subsequence present in both sequences in the same order.
- Longest Increasing Subsequence: Find the longest subsequence such that all elements are sorted in increasing order.
- 0/1 Knapsack Problem: Given weights and values of items, determine the maximum value that can be put in a knapsack of a given capacity.
- Coin Change Problem: Find the minimum number of coins needed to make a certain amount of change.
- Edit Distance: Find the minimum number of operations required to convert one string into another.
Optimizing Your Solution
When optimizing your DP solution, consider the trade-offs between speed, memory usage, and maintainability. For example, if memory is a constraint, you might not need to store every single result, just the most recent ones.
A word about optimizing: you could argue that these optimizations make it more difficult to change the algorithm if the requirements change in the future. That’s a reasonable stand. As long as you can hold a good conversation about pros and cons, I am happy with a high-quality discussion on how you would balance these decisions.
Tips for Interviews
- Start with a Naive Solution: Begin with a simple solution to understand the problem better. This often involves a brute-force approach.
- Identify Overlapping Subproblems: Look for subproblems that are solved multiple times and think about how to store their results.
- Optimize for Time and Space: Once you have a working solution, think about how to make it more efficient in terms of time and space complexity.
By mastering these concepts and practicing common DP problems, you’ll be well-prepared for your next tech interview.
Graph Algorithms
Graph algorithms are essential for solving many complex problems in computer science. Understanding these algorithms can give you a significant edge in tech interviews. Here are some common graph algorithms you should know:
Depth-First Search (DFS)
DFS explores as far as possible along each branch before backtracking. It’s useful for tasks like finding connected components and detecting cycles in a graph.
Breadth-First Search (BFS)
BFS explores all neighbors at the present depth before moving on to nodes at the next depth level. It’s often used for finding the shortest path in unweighted graphs.
Dijkstra’s Algorithm
This algorithm finds the shortest path between nodes in a graph with non-negative weights. It’s widely used in network routing protocols.
A* Search Algorithm
A* is an informed search algorithm that uses heuristics to find the shortest path more efficiently than Dijkstra’s in many cases.
Floyd-Warshall Algorithm
This algorithm computes shortest paths between all pairs of nodes in a graph. It’s useful for dense graphs where you need to know the shortest path between every pair of nodes.
Kruskal’s Algorithm
Kruskal’s algorithm finds the minimum spanning tree for a connected, undirected graph. It adds edges in increasing order of weight, ensuring no cycles are formed.
Prim’s Algorithm
Prim’s algorithm also finds the minimum spanning tree but starts from a single vertex and grows the tree one edge at a time.
Topological Sort
Topological sorting is used for ordering vertices in a directed acyclic graph (DAG). It’s useful for scheduling tasks that depend on each other.
Bellman-Ford Algorithm
This algorithm computes shortest paths from a single source node to all other nodes, even if the graph has negative weights. However, it is slower than Dijkstra’s algorithm.
Mastering these graph algorithms can make you a strong candidate in tech interviews. Each algorithm is like a powerful tool in your problem-solving toolkit.
Binary Search
Binary search is a fundamental algorithm used to find an element in a sorted array. It works by repeatedly dividing the search interval in half. If the value of the search key is less than the item in the middle of the interval, narrow the interval to the lower half. Otherwise, narrow it to the upper half. Repeatedly check until the value is found or the interval is empty.
Key Concepts
- Time Complexity: O(log n)
- Space Complexity: O(1)
Steps to Implement Binary Search
- Start with the middle element of the array.
- If the middle element is the target value, return the index.
- If the target value is less than the middle element, repeat the search on the left half of the array.
- If the target value is greater, repeat the search on the right half of the array.
- Continue until the target value is found or the subarray size becomes zero.
Common Variants
- Lower Bound Binary Search: Finds the first position where the target can be inserted without violating the order.
- Upper Bound Binary Search: Finds the first position where the target is greater than the element.
Understanding the top interview questions on binary search can give you a significant edge in your next tech interview. Make sure to practice different variants of binary search to be well-prepared.
Bit Manipulation
Bit manipulation is a technique in competitive programming that involves the manipulation of individual bits in binary representations of numbers. Mastering bit manipulation can give you a significant edge in coding interviews. Here are some common bit manipulation problems you might encounter:
- Counting Set Bits: Write a function to count the number of 1s in the binary representation of a number.
- Bitwise AND of Numbers Range: Given a range [m, n], return the bitwise AND of all numbers in this range.
- Power of Two: Determine if a given number is a power of two.
- Single Number: In an array where every element appears twice except for one, find that single one using bitwise operations.
- Reverse Bits: Reverse the bits of a given 32-bit unsigned integer.
Bit manipulation problems often require a deep understanding of binary operations and can be optimized for both speed and memory usage. Balancing these optimizations is a key trait of a great engineer.
Tips for Bit Manipulation
- Use Masks: Masks can help isolate or modify specific bits in a number.
- Shift Operators: Left and right shift operators are useful for multiplying or dividing by powers of two.
- XOR Trick: XOR can be used to swap two numbers without a temporary variable.
Common Bit Manipulation Functions
Function | Description |
---|---|
& |
Bitwise AND |
` | ` |
^ |
Bitwise XOR |
~ |
Bitwise NOT |
<< |
Left Shift |
>> |
Right Shift |
Understanding these basic operations and their applications can help you solve complex problems efficiently.
String Manipulation
String manipulation is a crucial skill for any tech interview. Here are some common coding questions you might encounter:
- Reverse a String: Write code to reverse a string without changing the order of the words.
- String Permutations: Create a program that returns all possible permutations of a given string.
- Longest Palindrome Substring: Write a program to find the longest palindrome substring in a given string.
- Roman Numerals Conversion: Convert a string with elements represented in Roman numerals to their numeric values.
- Remove Duplicate Letters: Write a program to delete duplicate letters from a string.
- Convert to Palindrome: Develop code to convert a given string into a palindrome.
- Longest Subsequence with Distinct Characters: Find the longest subsequence of a string that has all distinct characters.
- Remove Successive Identical Characters: Write code to remove successive identical characters from a string recursively.
String manipulation: crack the DSA coding interview by mastering these common questions. Practice these problems to improve your skills and boost your confidence for your next tech interview.
Matrix Operations
Matrix operations are a common topic in coding interviews, especially for roles that require strong problem-solving skills. Understanding how to manipulate matrices efficiently can set you apart from other candidates. Here are some key operations and questions you might encounter:
Common Matrix Operations
- Matrix Addition: Adding two matrices by adding corresponding elements.
- Matrix Subtraction: Subtracting one matrix from another by subtracting corresponding elements.
- Matrix Multiplication: Multiplying two matrices, which involves the dot product of rows and columns.
- Transpose of a Matrix: Flipping a matrix over its diagonal, switching the row and column indices of each element.
- Determinant of a Matrix: A scalar value that can be computed from the elements of a square matrix and encodes certain properties of the matrix.
- Inverse of a Matrix: A matrix that, when multiplied with the original matrix, yields the identity matrix.
Sample Coding Problems
- Matrix Rotation: Rotate a matrix by 90 degrees in place.
- Spiral Order Traversal: Print all elements of a matrix in spiral order.
- Search in a Sorted Matrix: Search for a value in an m x n matrix. This matrix has the following properties: Integers in each row are sorted from left to right. The first integer of each row is greater than the last integer of the previous row.
- Set Matrix Zeroes: Given an m x n matrix, if an element is 0, set its entire row and column to 0.
Mastering these operations and problems will give you a solid foundation for tackling more complex coding problems on matrix data structure.
Greedy Algorithms
Greedy algorithms are a popular approach in coding interviews, especially for optimization problems. These algorithms make a series of choices, each of which looks best at the moment, with the hope of finding a global optimum.
Key Concepts
- Local Optimum: Greedy algorithms make decisions based on the best choice at each step, aiming for a local optimum.
- Global Optimum: The goal is to achieve the best overall solution, or global optimum, by combining these local choices.
Common Problems
Here are some of the top 20 greedy algorithms interview questions:
- Activity Selection Problem: Choose the maximum number of activities that don’t overlap.
- Kruskal’s Minimum Spanning Tree Algorithm: Find the minimum spanning tree in a graph.
- Huffman Coding: Create an efficient prefix code for data compression.
Advantages and Disadvantages
- Advantages: Simple to implement and often faster than other algorithms.
- Disadvantages: May not always produce the optimal solution for all problems.
Greedy algorithms are a powerful tool in your software engineering arsenal, particularly for problems where a local optimum leads to a global optimum.
Example: Activity Selection Problem
Consider a set of activities with start and end times. The goal is to select the maximum number of activities that don’t overlap. Here’s a simple approach:
- Sort activities by their end times.
- Select the first activity and add it to the result.
- For each subsequent activity, if its start time is greater than or equal to the end time of the last selected activity, add it to the result.
This approach ensures that you always pick the activity that leaves the most room for future activities, thus maximizing the number of non-overlapping activities.
Backtracking
Backtracking is a powerful algorithmic technique used to solve problems incrementally, one piece at a time, and remove solutions that fail to satisfy the problem’s constraints. It’s often used in problems involving permutations, combinations, and subsets.
Divide and Conquer
The divide and conquer algorithm is a problem-solving strategy that involves breaking down a complex problem into smaller, more manageable parts. This method is widely used in computer science to optimize solutions and improve efficiency.
Key Steps in Divide and Conquer
- Divide: Split the problem into smaller sub-problems that are similar to the original but simpler to solve.
- Conquer: Solve the sub-problems recursively. If the sub-problems are small enough, solve them directly.
- Combine: Merge the solutions of the sub-problems to form the solution to the original problem.
Examples of Divide and Conquer Algorithms
- Merge Sort: This algorithm divides the array into halves, recursively sorts them, and then merges the sorted halves.
- Quick Sort: It picks a pivot element, partitions the array around the pivot, and recursively sorts the partitions.
- Binary Search: This algorithm repeatedly divides a sorted array in half to locate a target value.
Divide and conquer is a powerful technique that can significantly reduce the time complexity of many algorithms. However, it requires careful consideration of the base cases and the merging process to ensure correctness and efficiency.
Heap
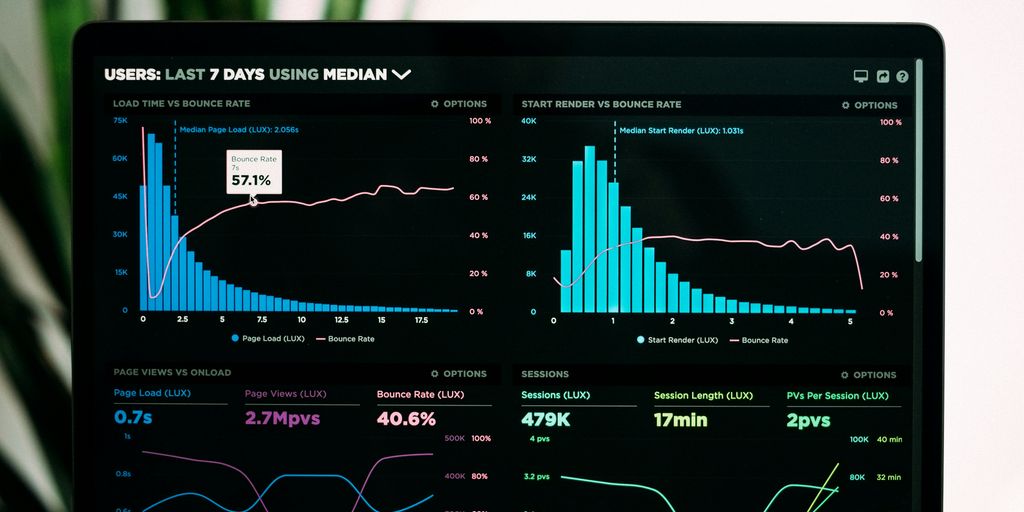
A heap is a specialized tree-based data structure that satisfies the heap property. There are two types of heaps: max-heaps and min-heaps.
Max-Heap
In a max-heap, the key at the root must be the largest among all keys present in the heap. The same property must be recursively true for all sub-trees in that heap.
Min-Heap
In a min-heap, the key at the root must be the smallest among all keys present in the heap. The same property must be recursively true for all sub-trees in that heap.
Common Operations
- Insertion: Adding a new key to the heap.
- Deletion: Removing the root key from the heap.
- Peek: Viewing the root key without removing it.
Applications
- Priority Queues: Heaps are often used to implement priority queues.
- Heap Sort: A comparison-based sorting technique based on a binary heap data structure.
- Graph Algorithms: Used in algorithms like Dijkstra’s and Prim’s for finding the shortest path and minimum spanning tree, respectively.
Understanding heaps is crucial for solving problems related to priority queues and efficient sorting. Mastering this data structure can give you an edge in technical interviews.
Trie
A trie data structure consists of nodes connected by edges. Each node represents a character or a part of a string. The root node, the starting point of the trie, is usually empty and does not contain any character.
Key Operations
- Insert: Adding a word to the trie involves creating nodes for each character of the word. If a character node already exists, you move to the next node.
- Search: To find a word, you traverse the trie following the characters of the word. If you reach the end of the word and all characters are found, the word exists in the trie.
- Delete: Removing a word requires you to delete nodes from the end of the word back to the root, but only if the node is not part of another word.
Advantages
- Efficient Prefix Search: Tries are excellent for prefix-based searches, making them ideal for autocomplete features.
- Space Optimization: Although they can consume a lot of space, tries can be optimized using techniques like compressing nodes.
Use Cases
- Autocomplete Systems: Used in search engines and text editors to suggest words based on prefixes.
- Spell Checkers: Helps in quickly finding and suggesting corrections for misspelled words.
- IP Routing: Used in networking to store routing information efficiently.
Tries are powerful data structures that offer efficient solutions for many common problems involving strings and prefixes.
Breadth-First Search
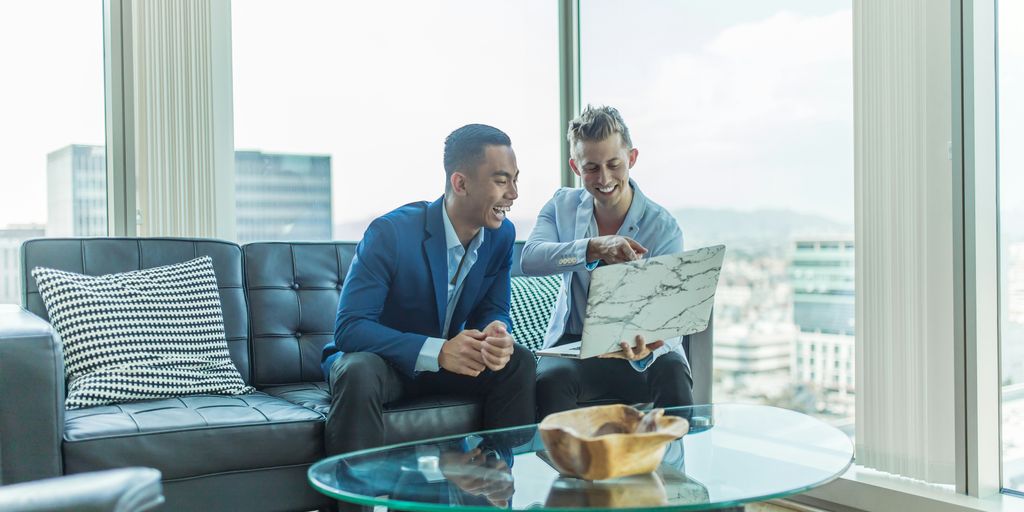
Breadth-First Search (BFS) is a fundamental algorithm used for traversing or searching tree or graph data structures. It explores all nodes at the present depth level before moving on to nodes at the next depth level.
How BFS Works
- Start at the root node (or any arbitrary node in the case of a graph).
- Visit all the neighboring nodes.
- Move to the next level of nodes and repeat the process until all nodes are visited.
BFS Algorithm
Here’s a simple version of the BFS algorithm:
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
while queue:
node = queue.popleft()
if node not in visited:
visited.add(node)
queue.extend(neighbor for neighbor in graph[node] if neighbor not in visited)
return visited
Applications of BFS
- Shortest Path: BFS can be used to find the shortest path in an unweighted graph.
- Level Order Traversal: In trees, BFS is used for level order traversal.
- Connected Components: It helps in finding all connected components in a graph.
BFS and Depth-First Search (DFS) are two fundamental algorithms used for traversing or searching graphs and trees.
BFS vs. DFS
Feature | BFS | DFS |
---|---|---|
Data Structure | Queue | Stack |
Time Complexity | O(V + E) | O(V + E) |
Space Complexity | O(V) | O(V) |
Use Case | Shortest Path | Topological Sorting |
Understanding BFS is crucial for solving many graph-related problems efficiently.
Depth-First Search
Depth-First Search (DFS) is a fundamental algorithm used to traverse or search through a graph. Mastering DFS is crucial for technical interviews as it helps in solving various problems efficiently.
How DFS Works
DFS starts at a chosen node and explores as far as possible along each branch before backtracking. This method is useful for tasks like finding connected components or detecting cycles in a graph.
Steps to Implement DFS
- Start at the root node (or any arbitrary node in the case of a graph).
- Mark the node as visited.
- Explore each adjacent node that hasn’t been visited yet.
- Recursively apply the same process to each of these adjacent nodes.
- Backtrack when no unvisited adjacent nodes are left.
Example Code
Here’s a simple implementation of DFS in Python:
# Depth-First Search in Python
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start)
for next in graph[start] - visited:
dfs(graph, next, visited)
return visited
# Example usage
graph = {'A': {'B', 'C'}, 'B': {'A', 'D', 'E'}, 'C': {'A', 'F'}, 'D': {'B'}, 'E': {'B', 'F'}, 'F': {'C', 'E'}}
dfs(graph, 'A')
Applications of DFS
- Pathfinding: DFS can be used to find a path between two nodes in a graph.
- Topological Sorting: Useful in scheduling tasks.
- Cycle Detection: Helps in identifying cycles in a graph.
- Connected Components: Finds all connected components in a graph.
Understanding how to implement the depth-first search (DFS) algorithm is essential for solving many graph-related problems. It provides a clear method to traverse or search through a graph, making it a valuable tool in your coding arsenal.
Topological Sort
Topological sorting is a method used for ordering vertices in a directed acyclic graph (DAG). In this order, for every directed edge u-v
, vertex u
comes before v
.
Steps to Perform Topological Sort
- Identify vertices with no incoming edges and add them to a list.
- Remove these vertices and their outgoing edges from the graph.
- Repeat the process until all vertices are removed.
Applications of Topological Sort
- Task Scheduling: Helps in scheduling tasks based on dependencies.
- Course Prerequisites: Determines the order in which courses should be taken.
Topological sorting for directed acyclic graph (DAG) is a linear ordering of vertices such that for every directed edge u-v, vertex u comes before v in the order.
Example
Consider the following graph:
Vertex | Edges |
---|---|
A | B, C |
B | D |
C | D |
D |
A possible topological sort for this graph is: A -> B -> C -> D
or A -> C -> B -> D
.
Key Points
- Topological sort is only possible for DAGs.
- There can be multiple valid topological sorts for a given graph.
Union-Find
The Union-Find algorithm is a powerful tool used to solve problems related to disjoint sets. It is particularly useful in scenarios where you need to determine the connected components in a graph.
Key Operations
- Find: This operation determines the root of the set containing a particular element. It helps in identifying which subset a particular element is in.
- Union: This operation merges two subsets into a single subset. It is used to combine two sets into one.
Applications
- Cycle Detection: The union-find approach provides an efficient way to detect cycles in a graph and identify the redundant edge that needs to be removed to convert the graph into a tree.
- Kruskal’s Algorithm: Used in finding the Minimum Spanning Tree (MST) of a graph.
- Connected Components: Helps in finding all connected components in an undirected graph.
The union-find algorithm is essential for solving many graph-related problems efficiently.
Example
Consider a graph with nodes and edges. Using union-find, you can quickly determine if adding an edge will form a cycle or not. This is done by checking if the two nodes of the edge belong to the same set.
Pseudocode
function find(x)
if parent[x] != x
parent[x] = find(parent[x])
return parent[x]
function union(x, y)
rootX = find(x)
rootY = find(y)
if rootX != rootY
parent[rootY] = rootX
By understanding and implementing the union-find algorithm, you can efficiently manage and query disjoint sets, making it a valuable tool in your coding arsenal.
Segment Tree and more
Segment Trees are a powerful data structure used for answering range queries efficiently. They are particularly useful when dealing with problems that involve frequent updates and queries on an array.
Segment Tree Basics
A Segment Tree is a binary tree used for storing intervals or segments. It allows querying which of the stored segments contain a given point. Each node in the Segment Tree represents an interval or segment of the array.
Building a Segment Tree
To build a Segment Tree, follow these steps:
- Start with the leaf nodes, which represent individual elements of the array.
- Combine leaf nodes to form internal nodes, which represent the union of their child nodes.
- Continue this process until you reach the root node, which represents the entire array.
Querying a Segment Tree
To query a Segment Tree, you need to:
- Start at the root node and check if the query range overlaps with the node’s range.
- If it does, move to the child nodes and repeat the process.
- Combine the results from the child nodes to get the final answer.
Updating a Segment Tree
Updating a Segment Tree involves:
- Finding the leaf node that corresponds to the element to be updated.
- Updating the leaf node and then updating its parent nodes to reflect the change.
- Continue this process until you reach the root node.
Segment Trees are essential for solving range query problems efficiently, especially when frequent updates are involved.
More Advanced Data Structures
In addition to Segment Trees, there are other advanced data structures that are useful for specific types of problems:
- Fenwick Tree (Binary Indexed Tree): Useful for cumulative frequency tables.
- Suffix Tree: Ideal for string processing problems.
- K-D Tree: Used for multi-dimensional search queries.
These data structures, along with Segment Trees, form a crucial part of the toolkit for solving complex coding interview questions.
Discover the power of Segment Trees and more in our latest article. Whether you’re a beginner or an experienced coder, our interactive tutorials and step-by-step guides will help you master these essential data structures. Ready to take your coding skills to the next level?
Conclusion
Preparing for a tech interview can be tough, but with the right practice and mindset, you can ace it. We’ve gathered insights from 50 developers who recently landed jobs to bring you the most challenging coding questions they faced. Remember, technical interviews are not just about solving problems but also about showing your thought process and communication skills. Practice different types of questions, understand the concepts behind them, and don’t forget to stay calm and confident. Good luck on your next tech interview!
Frequently Asked Questions
What is the purpose of technical interviews?
Technical interviews help companies see if you have the right skills for the job. They check how you solve problems, your coding skills, and how you work with others.
How are technical interviews usually structured?
Technical interviews often include coding challenges, system design questions, and behavioral questions. They want to see both your technical and soft skills.
What types of coding questions are asked in technical interviews?
You might get questions about basic data structures like arrays, linked lists, and trees, as well as algorithms. The questions can range from easy to very hard.
How can I prepare for a coding interview?
Practice coding problems, study data structures and algorithms, and review your past projects. Also, try explaining your solutions out loud as if teaching someone.
What should I do if I don’t know the answer to a question during the interview?
Stay calm and be honest. Try to think out loud and explain your thought process. Interviewers appreciate when you show how you approach solving problems, even if you don’t get the answer right away.
Why do companies ask tricky coding questions?
Companies want to hire people who can solve real-world problems. Tricky questions help them see how you think and solve problems using your coding skills.
What are some common behavioral questions in technical interviews?
Behavioral questions might ask about your past projects, how you work with others, or how you handle challenges. They want to see your teamwork and problem-solving skills.
How important is it to explain technical terms to non-technical people during an interview?
It’s very important. Being able to explain complex ideas in simple terms shows that you can communicate well with all team members, not just other developers.