Does Memorizing Syntax Make You a Better Coder?
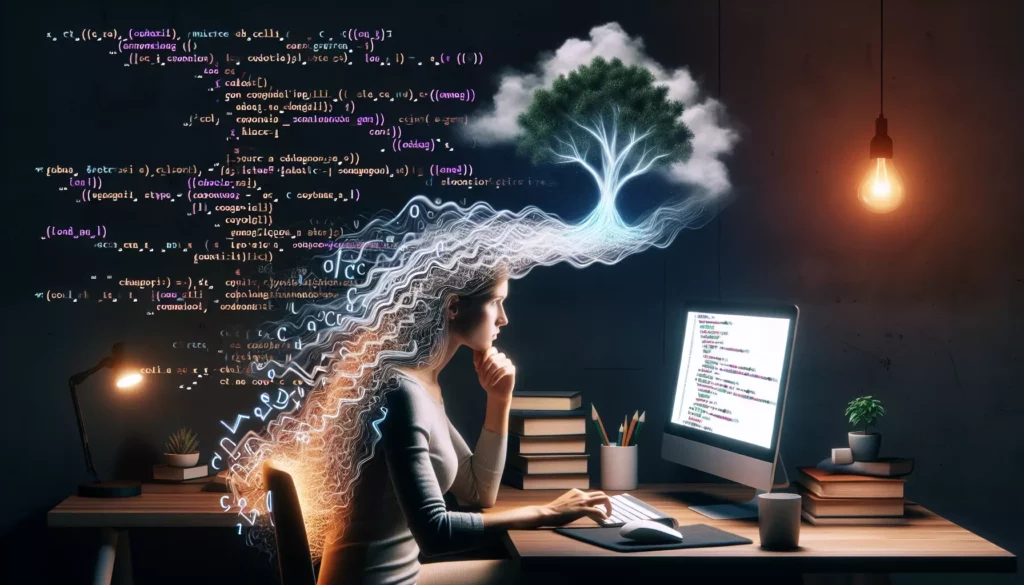
In the world of programming, there’s an ongoing debate about the importance of memorizing syntax versus understanding core concepts. As aspiring coders embark on their journey to mastery, many wonder: “Does memorizing syntax actually make you a better coder?” This question is particularly relevant for those using platforms like AlgoCademy, which focuses on coding education and programming skills development. In this comprehensive exploration, we’ll dive deep into the role of syntax memorization in coding proficiency, examine its benefits and limitations, and provide insights on how to balance syntax knowledge with other crucial programming skills.
The Role of Syntax in Programming
Before we delve into the question at hand, let’s first understand what syntax is and why it matters in programming:
What is Syntax?
In programming, syntax refers to the set of rules that define how to write code in a particular language. It’s the grammar of programming languages, dictating how statements, expressions, and other elements should be structured to create valid code. Just as natural languages have rules for sentence structure, programming languages have specific syntax rules that must be followed for the code to be understood by the compiler or interpreter.
Why is Syntax Important?
Syntax is crucial for several reasons:
- Code Correctness: Proper syntax ensures that your code can be successfully compiled or interpreted.
- Readability: Consistent syntax makes code easier to read and understand, both for yourself and other developers.
- Efficiency: Knowing syntax well can speed up the coding process, as you spend less time looking up basic language structures.
- Error Prevention: Familiarity with syntax helps in identifying and avoiding common coding errors.
The Case for Memorizing Syntax
Proponents of syntax memorization argue that it offers several advantages:
1. Increased Coding Speed
When you have syntax committed to memory, you can write code more quickly without constantly referring to documentation. This speed can be particularly beneficial in time-sensitive situations like coding interviews or hackathons.
2. Improved Focus on Problem-Solving
By not having to think about the basic structure of your code, you can dedicate more mental energy to solving the problem at hand. This is especially relevant when working on complex algorithms or data structures.
3. Enhanced Readability of Others’ Code
A strong grasp of syntax allows you to more easily read and understand code written by others. This skill is invaluable when working on team projects or maintaining existing codebases.
4. Easier Debugging
When you’re familiar with correct syntax, it becomes easier to spot syntax errors in your code. This can save time during the debugging process.
5. Confidence Boost
Knowing the syntax of a language inside and out can boost your confidence as a programmer. This confidence can translate into better performance in coding tasks and interviews.
The Limitations of Syntax Memorization
While there are clear benefits to memorizing syntax, it’s important to recognize its limitations:
1. Syntax Changes Over Time
Programming languages evolve, and syntax can change with new versions. Relying solely on memorized syntax may leave you behind as languages update.
2. Multiple Languages Use Different Syntax
In today’s polyglot programming environment, developers often work with multiple languages. Memorizing syntax for each can be overwhelming and may lead to confusion.
3. Syntax ≠Understanding
Knowing how to write syntactically correct code doesn’t necessarily mean you understand the underlying concepts or best practices in programming.
4. Overemphasis on Memorization
Focusing too much on memorizing syntax can detract from learning more important aspects of programming, such as algorithm design, data structures, and problem-solving techniques.
5. Tools Reduce the Need for Perfect Recall
Modern integrated development environments (IDEs) and code editors often provide features like auto-completion and syntax highlighting, reducing the need for perfect syntax recall.
Beyond Syntax: What Makes a Great Coder?
While syntax knowledge is important, it’s just one piece of the puzzle. Great coders possess a range of skills and qualities:
1. Problem-Solving Ability
The core of programming is solving problems. Strong analytical and logical thinking skills are crucial for breaking down complex problems into manageable parts.
2. Algorithmic Thinking
Understanding how to design efficient algorithms is often more important than knowing the exact syntax to implement them.
3. Data Structure Knowledge
Familiarity with various data structures and when to use them is essential for writing efficient and scalable code.
4. Design Patterns and Architecture
Knowledge of software design patterns and architectural principles helps in creating maintainable and extensible code.
5. Debugging Skills
The ability to effectively troubleshoot and fix issues in code is a hallmark of a skilled programmer.
6. Continuous Learning
The tech field is constantly evolving. Great coders are those who embrace lifelong learning and adapt to new technologies and practices.
7. Collaboration and Communication
In most professional settings, coding is a team effort. The ability to work well with others and clearly communicate ideas is invaluable.
Balancing Syntax Knowledge with Other Skills
So, how can aspiring coders strike the right balance between syntax memorization and other crucial skills? Here are some strategies:
1. Focus on Concepts First
Prioritize understanding programming concepts and principles. Once you grasp these, applying them in different syntaxes becomes easier.
2. Practice Active Coding
Instead of rote memorization, engage in active coding practice. Platforms like AlgoCademy offer interactive tutorials and coding challenges that help reinforce syntax naturally through use.
3. Use Spaced Repetition
If you do want to memorize syntax, use spaced repetition techniques. Review and practice syntax at increasing intervals to improve long-term retention.
4. Leverage Tools Wisely
Use IDE features and documentation as learning aids rather than crutches. Gradually reduce your reliance on these tools as you become more familiar with the syntax.
5. Cross-Language Learning
Study multiple programming languages to understand common programming paradigms. This approach helps you focus on underlying concepts rather than specific syntax.
6. Real-World Projects
Work on practical projects that interest you. This approach naturally reinforces syntax while also developing problem-solving and design skills.
7. Code Review and Collaboration
Engage in code reviews and collaborative projects. This exposes you to different coding styles and reinforces best practices beyond just syntax.
The AlgoCademy Approach
Platforms like AlgoCademy recognize the importance of balancing syntax knowledge with broader coding skills. Here’s how AlgoCademy’s approach aligns with effective coding education:
1. Interactive Learning
AlgoCademy provides interactive coding tutorials that allow learners to practice syntax in context, reinforcing both language structure and problem-solving skills.
2. Focus on Algorithmic Thinking
The platform emphasizes algorithmic thinking and problem-solving, encouraging learners to focus on the logic behind solutions rather than just memorizing code snippets.
3. Progressive Skill Development
AlgoCademy’s curriculum is designed to progressively build skills, from basic syntax to complex algorithms and data structures, ensuring a well-rounded coding education.
4. AI-Powered Assistance
The AI-powered assistance feature helps learners understand and correct syntax errors, providing a balance between guidance and independent problem-solving.
5. Technical Interview Preparation
By focusing on preparing learners for technical interviews, especially for major tech companies, AlgoCademy emphasizes the importance of both syntax proficiency and higher-level coding skills.
Practical Tips for Effective Syntax Learning
While memorizing every aspect of syntax isn’t necessary, having a solid grasp of language basics is beneficial. Here are some tips for effective syntax learning:
1. Start with the Fundamentals
Focus on mastering the basic syntax elements first, such as variable declarations, control structures, and function definitions. Here’s a simple example in Python:
# Basic Python syntax example
def greet(name):
if name:
print(f"Hello, {name}!")
else:
print("Hello, World!")
greet("Alice") # Output: Hello, Alice!
greet("") # Output: Hello, World!
2. Use Mnemonics and Analogies
Create memory aids to remember tricky syntax. For example, in JavaScript, remember “VAR let’s CONST(antly) be” to recall the three variable declaration keywords.
3. Write Code by Hand
Occasionally practice writing code snippets by hand. This reinforces muscle memory and helps internalize syntax patterns.
4. Implement Common Algorithms
Practice implementing basic algorithms in your chosen language. This reinforces syntax while also improving your problem-solving skills. Here’s an example of a simple sorting algorithm in Java:
// Bubble sort implementation in Java
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
// swap arr[j+1] and arr[j]
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
5. Read and Analyze Code
Regularly read code written by experienced developers. This exposes you to different syntax usage and coding styles.
6. Teach Others
Explaining syntax to others can solidify your own understanding. Consider participating in coding forums or mentoring beginners.
The Evolving Landscape of Coding and Syntax
As we consider the role of syntax memorization in coding proficiency, it’s important to acknowledge the evolving landscape of programming:
1. Rise of Low-Code and No-Code Platforms
The increasing popularity of low-code and no-code platforms is changing the way we think about syntax. These platforms allow users to create applications with minimal traditional coding, emphasizing logical thinking over syntax knowledge.
2. AI-Assisted Coding
AI-powered coding assistants, like GitHub Copilot, are becoming more prevalent. These tools can suggest code snippets and even complete functions, potentially reducing the need for extensive syntax memorization.
3. Cross-Platform Development
With the rise of cross-platform development frameworks, developers increasingly need to work with multiple syntaxes simultaneously. This trend underscores the importance of understanding core programming concepts that transcend specific language syntax.
4. Emphasis on Soft Skills
The tech industry is placing greater emphasis on soft skills like communication, collaboration, and adaptability. While technical proficiency remains crucial, these skills are increasingly valued alongside coding abilities.
Conclusion: The Balanced Approach to Coding Mastery
So, does memorizing syntax make you a better coder? The answer isn’t a simple yes or no. While a solid grasp of syntax is undoubtedly beneficial, it’s just one component of becoming a proficient programmer. True coding mastery comes from a balanced approach that combines syntax familiarity with strong problem-solving skills, algorithmic thinking, and a deep understanding of programming concepts.
Platforms like AlgoCademy recognize this need for balance, offering comprehensive learning experiences that go beyond mere syntax memorization. They provide interactive environments where learners can practice syntax in context, develop problem-solving skills, and prepare for real-world coding challenges.
As you continue your coding journey, remember that the goal isn’t to become a human syntax reference, but rather to develop the ability to think like a programmer. Focus on understanding the underlying logic of programming, practice regularly, and don’t be afraid to reference documentation when needed. With time and experience, you’ll find that syntax becomes second nature, allowing you to focus on what truly matters: creating efficient, elegant solutions to complex problems.
Embrace the learning process, stay curious, and remember that every great coder was once a beginner. Whether you’re using AlgoCademy or other resources, keep pushing yourself to learn, experiment, and grow. The world of coding is vast and ever-changing, offering endless opportunities for those who approach it with passion, perseverance, and a balanced skill set.