10 Code Optimization Techniques to Improve Your Algorithm Efficiency
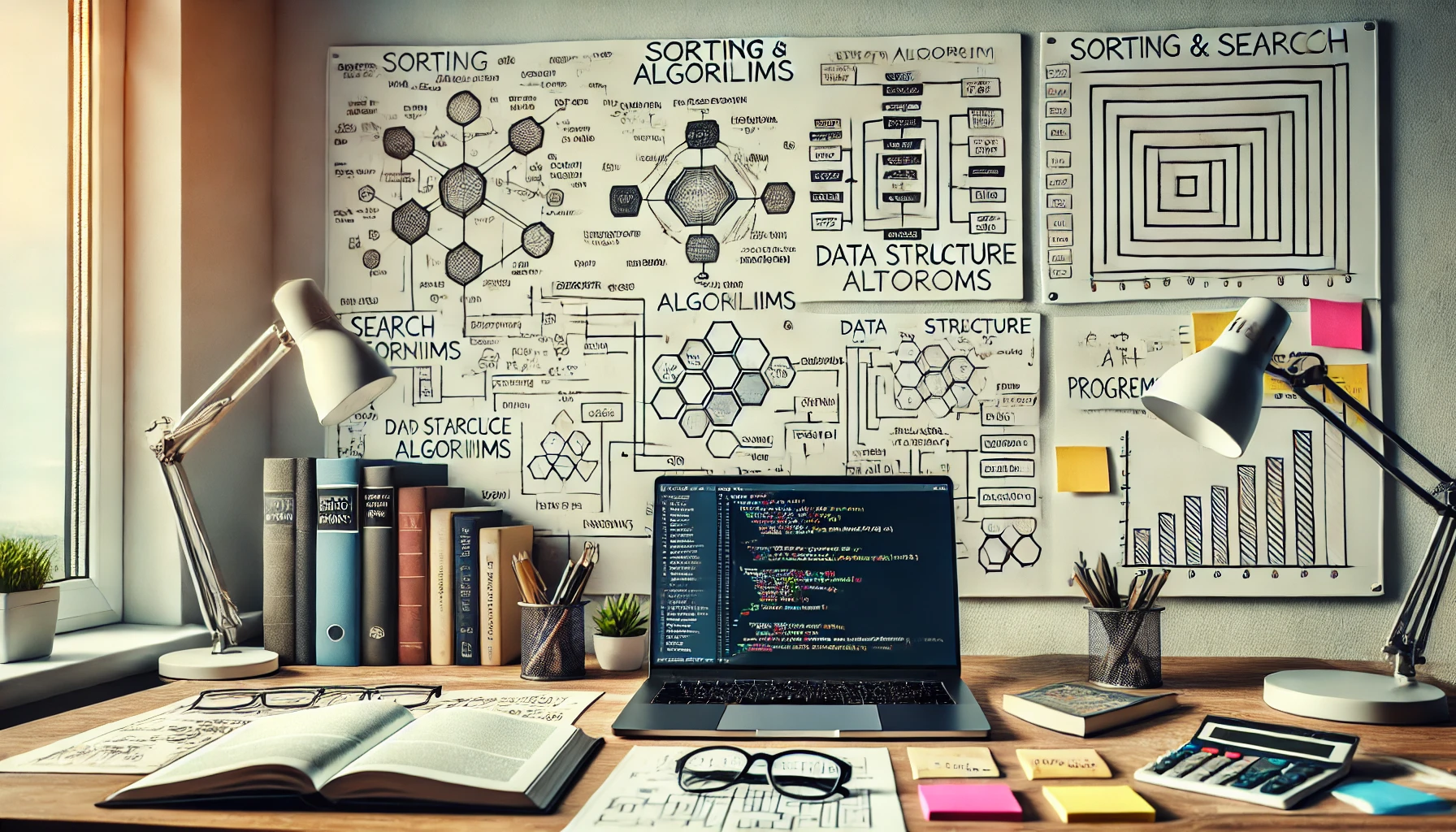
Optimizing your code is like giving your computer a turbo boost. By making your code run faster and use less memory, you can make your programs work better and more efficiently. In this article, we will look at 10 simple techniques to make your code run smoother and faster.
Key Takeaways
- Always measure your code’s performance before optimizing to identify real bottlenecks.
- Choose the right algorithms and data structures to improve efficiency.
- Reduce the number of input/output operations to save time.
- Use caching to store and reuse results of expensive operations.
- Avoid using global variables to keep your code fast and clean.
1. Measure Before You Optimize
Before you start optimizing your code, it’s crucial to have a baseline to compare against. Profiling tools like Python’s cProfile or Chrome DevTools can help you identify bottlenecks in your code. This step is essential because guessing where the problem lies often leads to wasted effort.
Steps to Follow
- Measure: Use profiling tools to gather data on your code’s performance.
- Analyze: Look at the data to find the bottlenecks that are slowing down your code.
- Decide: Determine if the potential improvements are worth the effort. Sometimes, the gains might be too small to justify the changes.
- Implement: Make the necessary changes to optimize your code.
- Repeat: Go back to the first step and measure again to see if your changes had the desired effect.
Always remember, premature optimization is the root of all evil. Focus on the big picture first, like choosing the right algorithm, before diving into micro-optimizations.
Don’t assume you know where the issues are. Profiling gives you the data you need to make informed decisions. This approach ensures that your efforts are directed towards the most impactful areas, making your optimization process efficient and effective.
2. Use Efficient Algorithms and Data Structures
Choosing the right algorithm and data structure for your problem can significantly impact performance. A linear search through an unsorted list is much less efficient than using a hash map or a binary search on a sorted array. Take time to understand the complexity of your algorithms (e.g., O(1), O(log n), O(n)) and select the most appropriate one.
Know Your Big-O
Understanding the time complexity of your algorithms is crucial. For example, a loop inside another loop, where the inner loop repeats a calculation, will be slower. When sorting a million 32-bit integers, bubble sort would be the wrong way to go. Instead, look for an algorithm that’s at least O(n * log n).
Choose the Right Data Structure
Different data structures have different performance characteristics. For instance, searching a linked list is O(n), meaning the time taken to search it increases with the size of the data. In contrast, searching a hash table is O(1), so you can add more data without increasing the search time.
Integer Arithmetic Hierarchy
When programming, remember that some arithmetic operations are more expensive than others. For integers, the hierarchy goes something like this (least expensive first):
-
-
- ~ & | ^
-
- << >>
-
- /
Efficient code not only runs faster but also consumes fewer resources, making it a fundamental aspect of software development.
3. Minimize I/O Operations
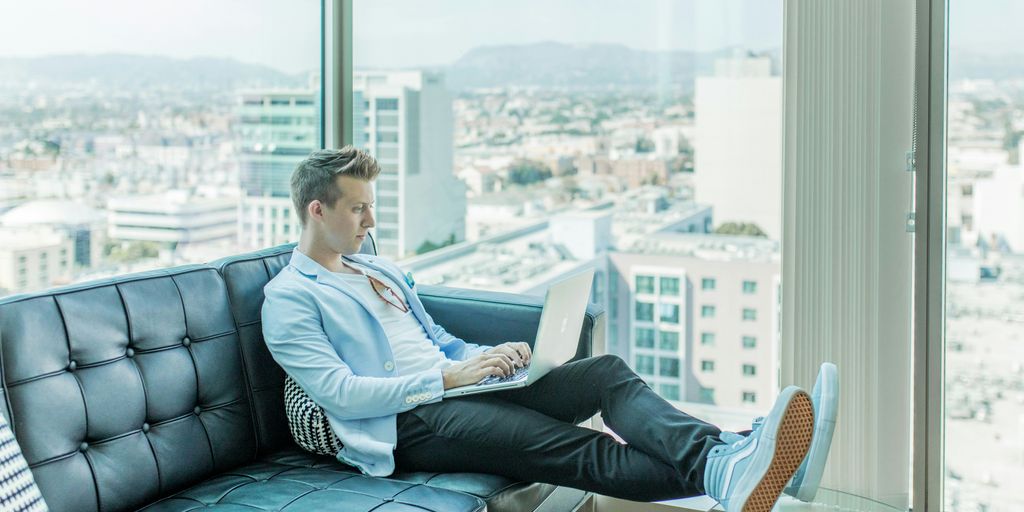
I/O operations, such as reading from or writing to a file or a database, are usually slower compared to in-memory operations. Reducing the number of I/O operations can significantly improve your program’s performance. Here are some effective techniques to minimize I/O operations:
- Batch Processing: Instead of processing I/O operations one at a time, group them together. This reduces the overhead associated with each individual operation.
- Caching: Store frequently accessed data in memory. This reduces the need to repeatedly read from or write to slower storage mediums.
- Buffering: Use buffers to temporarily hold data before writing it to disk. This can reduce the number of write operations and improve performance.
- Asynchronous I/O: Perform I/O operations asynchronously to avoid blocking the main execution thread. This can make your program more responsive.
Increasing RAM allows more data to be cached in memory, reducing the frequency of disk I/O operations.
By implementing these techniques, you can make your code more efficient and responsive.
4. Reduce Memory Usage
Efficient memory usage is crucial for optimizing your code. Avoid memory leaks by managing resources correctly and using data structures that minimize memory usage. Here are some tips to help you reduce memory usage:
- Use Generators: By leveraging generators, you can handle large datasets, simulate real-time data streams, and optimize memory usage in your applications.
- Choose the Right Data Structures: Opt for data structures that are memory efficient. For example, use sets instead of lists when you need to store unique items.
- Profile Memory Usage: Use memory profiling tools to identify memory-intensive areas of your code. This can help you pinpoint where optimizations are needed.
- Avoid Unnecessary Copies: Be mindful of creating unnecessary copies of data. Use references or pointers where possible to save memory.
- Release Memory Promptly: Ensure that you release memory as soon as it is no longer needed. This can be done by setting variables to
null
or using language-specific memory management techniques.
Efficient memory usage not only improves performance but also ensures that your application can handle larger datasets and more users without crashing.
5. Code Vectorization
In languages like Python and NumPy, vectorization allows you to perform operations on entire arrays or sequences at once, rather than iterating through them element by element. This can lead to significant performance improvements in numerical and scientific computing tasks.
Benefits of Code Vectorization
- Speed: Vectorized operations are often faster than their loop-based counterparts.
- Readability: Code that uses vectorization is usually more concise and easier to read.
- Efficiency: Reduces the overhead of loop control and function calls.
How to Implement Vectorization
- Identify the parts of your code that can be vectorized.
- Replace loops with vectorized operations.
- Test to ensure that the vectorized code produces the same results as the original.
Vectorization techniques in NLP can significantly speed up text processing tasks, making your code more efficient and easier to maintain.
6. Caching
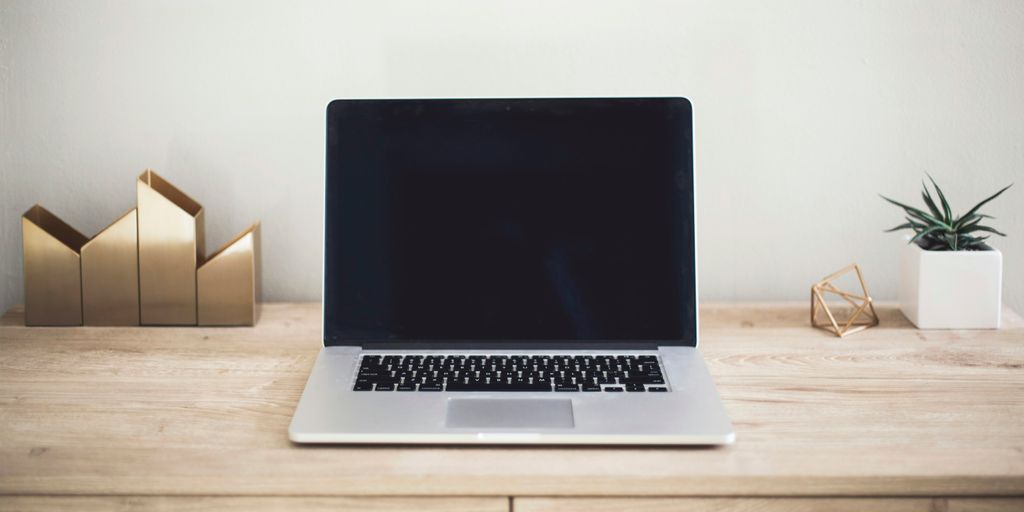
Caching is a technique where you store the results of expensive function calls and retrieve them when needed, rather than recalculating them every time. This is particularly useful for functions with deterministic outputs. Libraries like Redis or Memcached are excellent choices for implementing caching in your applications.
Identifying Ideal Candidates for Caching
Not all functions benefit equally from caching. Identifying ideal candidates is crucial for optimal performance. Functions that are called frequently and have high computational costs are prime candidates.
Cache Expiration and Eviction Policies
To ensure your cache remains efficient, you need to set expiration times and eviction policies. This helps in managing the cache size and ensures that stale data is removed.
Conditional Caching
Sometimes, you may not want to cache every result. Conditional caching allows you to cache results based on specific conditions, making your caching strategy more flexible.
Distributed Cache vs. Local Cache
Choosing between a distributed cache and a local cache depends on your application’s needs. A distributed cache is useful for large-scale applications, while a local cache might be sufficient for smaller ones.
Caching can significantly improve your application’s performance by reducing the need for redundant computations and minimizing I/O operations.
7. Avoid Global Variables
Global variables can slow down your code because they need to be constantly looked up and updated. Instead, use local variables whenever possible to limit the scope of data access and modification. This can significantly improve your code’s performance.
Why Avoid Global Variables?
- Performance Impact: Global variables can slow down your code due to the need for Python to look up these variables in the global scope.
- Maintainability: Code with fewer global variables is easier to read and maintain.
- Debugging: Localizing variables makes it easier to track down bugs.
Best Practices
- Use Local Variables: Whenever possible, declare variables within the smallest scope necessary.
- Encapsulation: Use classes and functions to encapsulate variables and limit their scope.
- Constants: If you need a global variable, consider making it a constant to avoid unintended modifications.
Avoiding global variables not only improves performance but also makes your code more maintainable and easier to debug.
8. Lazy Loading
Lazy loading is a strategy where you delay the loading of resources until they are actually needed. This can be very useful in web development, where you might not want to load all images, styles, or scripts right away. By doing this, you can make your initial page load much faster.
Why Use Lazy Loading?
- Improved Performance: By loading only the necessary resources, you can significantly reduce the initial load time of your web pages.
- Better User Experience: Users can start interacting with the page sooner, as the most important parts load first.
- Reduced Bandwidth Usage: Only the resources that are needed are loaded, which can save bandwidth, especially for users on mobile networks.
How to Implement Lazy Loading
- Identify Non-Critical Resources: Determine which resources are not essential for the initial page load. These could be images, videos, or additional scripts.
- Use Intersection Observer API: This API allows you to detect when an element is in the viewport and load it only then.
- Asynchronous Loading: Load scripts and styles asynchronously to avoid blocking the rendering of the page.
- Lazy Loading Images: Use the
loading="lazy"
attribute in your image tags to defer loading until the image is in the viewport.
Lazy loading is a strategy to identify resources as non-blocking (non-critical) and load these only when needed.
By following these steps, you can make your web pages load faster and provide a better experience for your users.
9. Parallelism and Concurrency
Modern hardware often comes with multiple cores, making parallelism and concurrency essential for improving performance. By running tasks simultaneously, you can significantly speed up your programs.
Benefits of Parallelism and Concurrency
- Increased Efficiency: Tasks are completed faster as they run at the same time.
- Better Resource Utilization: Multiple cores are used effectively, preventing idle time.
- Scalability: Programs can handle more tasks as more cores are added.
Tools and Libraries
To implement parallelism and concurrency, you can use various tools and libraries:
- OpenMP: Ideal for C/C++ programs, it simplifies parallel programming.
- Threading and Multiprocessing: In Python, these libraries help you run tasks in parallel.
- Java Concurrency Utilities: This guide explores basic and advanced concepts of multithreading, providing insights into various synchronization techniques, thread management, and modern practices.
Best Practices
- Identify Independent Tasks: Ensure tasks can run without depending on each other.
- Avoid Shared State: Minimize the use of global variables to prevent conflicts.
- Use Synchronization: Properly manage access to shared resources to avoid issues like race conditions.
- Profile and Test: Always measure performance to ensure that parallelism is actually improving efficiency.
Parallelism and concurrency can transform your programs, making them faster and more efficient. However, they require careful planning and testing to get right.
10. Compiler Optimizations
Compiler optimizations are techniques used by compilers to improve the performance and efficiency of the generated machine code. These optimizations can significantly reduce execution time, minimize resource usage, and enhance overall system performance without altering the program’s functionality.
Common Compiler Optimization Techniques
- Peephole Optimization: This technique involves examining a small set of instructions (a peephole) and replacing them with a more efficient set. It focuses on local improvements and can eliminate redundancies and unnecessary instructions.
- Loop Optimization: Since loops often consume a significant portion of execution time, optimizing them can lead to substantial performance gains. Techniques include loop unrolling, loop fusion, and loop invariant code motion.
- Dead Code Elimination: This process removes code that does not affect the program’s output, thereby reducing the size of the code and improving execution speed.
- Constant Folding: This technique evaluates constant expressions at compile time and replaces them with their results, reducing runtime computations and improving performance.
Advantages and Disadvantages
Advantages:
- Improved performance: Optimized code executes faster and uses fewer resources.
- Reduced code size: Optimization can make the code smaller, which is easier to distribute and deploy.
- Increased portability: Optimized code is often more portable across different platforms.
- Reduced power consumption: Efficient code consumes less power, making it more energy-efficient.
Disadvantages:
- Increased compilation time: Optimization can significantly increase the time it takes to compile the code.
- Increased complexity: Optimized code can be more complex, making it harder to understand and debug.
- Potential for introducing bugs: If not done carefully, optimization can introduce bugs into the code.
Compiler optimizations are essential for improving performance, but they must be applied judiciously to avoid potential pitfalls.
Compiler optimizations can make your code run faster and more efficiently. By understanding how compilers work, you can write better code that performs well in real-world applications. Want to learn more about how to optimize your code?
Conclusion
In conclusion, optimizing your code is essential for creating efficient and high-performing software. By applying the techniques discussed, such as choosing the right algorithms, minimizing I/O operations, and leveraging compiler optimizations, you can significantly improve your code’s performance. Remember, the key is to measure and analyze your code before making changes. This ensures that your optimizations are effective and do not introduce new issues. Keep practicing these techniques, and over time, you’ll become more adept at writing optimized and efficient code.
Frequently Asked Questions
What is code optimization?
Code optimization is the process of making your code run more efficiently by improving its speed, reducing its memory usage, or both.
Why should I measure performance before optimizing?
Measuring performance helps you identify the actual bottlenecks in your code. Without measuring, you might waste time optimizing parts that don’t significantly affect performance.
How do I choose the right algorithm or data structure?
Choosing the right algorithm or data structure depends on your specific problem. Learn about their time and space complexities and select the one that best fits your needs.
What are I/O operations, and why should I minimize them?
I/O operations involve reading from or writing to external resources like files or databases. They are usually slower than in-memory operations, so minimizing them can improve performance.
What is caching, and how does it help?
Caching stores the results of expensive function calls so you can reuse them instead of recalculating. This can significantly speed up your code, especially for functions with predictable outputs.
Why are global variables bad for performance?
Global variables can slow down your code because they need to be constantly accessed and updated. Using local variables can make your code run faster.
What is lazy loading?
Lazy loading is a technique where you delay loading resources until they are actually needed. This can make your application start faster and use resources more efficiently.
How can parallelism and concurrency improve performance?
Parallelism and concurrency allow your code to run multiple tasks at the same time, taking advantage of multi-core processors. This can make your code run much faster.