Are Traditional Coding Interviews Failing Us? A Deep Dive into Modern Tech Hiring Practices
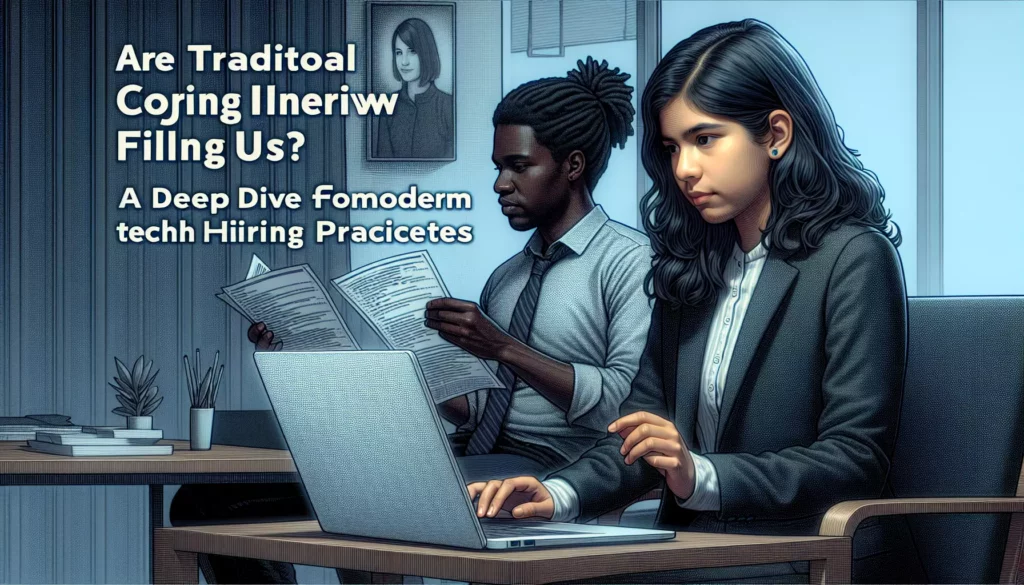
In the fast-paced world of technology, the way we hire software engineers has remained surprisingly static. For decades, the coding interview has been the gatekeeper to coveted positions at tech giants and startups alike. But as the industry evolves and the demands on developers shift, a growing chorus of voices is questioning whether these traditional methods are still serving their purpose. Are we truly identifying the best talent, or are we perpetuating a system that may be leaving exceptional candidates behind?
The Current State of Coding Interviews
Before we dive into the criticisms and potential alternatives, let’s take a moment to understand what we mean by “traditional coding interviews.” Typically, these interviews consist of:
- Algorithmic problem-solving on a whiteboard or in a code editor
- Data structure manipulation
- Time and space complexity analysis
- Verbal explanation of thought processes
- Sometimes, system design questions for more senior roles
Companies like Google, Facebook, Amazon, Apple, and Netflix (often collectively referred to as FAANG) have popularized this style of interview, which has since been adopted by countless other organizations in the tech industry.
The Case for Traditional Coding Interviews
Proponents of the traditional coding interview argue that it offers several benefits:
1. Standardization
These interviews provide a consistent way to evaluate candidates across different backgrounds and experiences.
2. Problem-Solving Skills
They test a candidate’s ability to think on their feet and solve complex problems under pressure.
3. Fundamental Knowledge
Algorithmic questions ensure that candidates have a solid grasp of computer science fundamentals.
4. Communication
The process often requires candidates to explain their thought process, testing their ability to communicate technical concepts clearly.
The Growing Criticisms
Despite these perceived benefits, the traditional coding interview has come under fire from various quarters of the tech community. Here are some of the main criticisms:
1. Lack of Real-World Relevance
Many argue that inverting binary trees or implementing complex sorting algorithms from scratch has little to do with day-to-day software development tasks.
2. Bias Towards Recent Graduates
The focus on algorithmic puzzles can favor recent computer science graduates who have these concepts fresh in their minds, potentially disadvantaging experienced developers who may be more skilled in practical application.
3. High-Stress Environment
The artificial pressure of solving complex problems on the spot can cause even skilled developers to underperform.
4. Preparation Burden
Candidates often spend months preparing for these interviews, time that could be spent on more practical skill development.
5. False Negatives
There’s a concern that this style of interview may be filtering out talented developers who simply don’t excel in this particular format.
The Impact on the Industry
The implications of these criticisms are far-reaching. If the traditional coding interview is indeed failing us, it could mean that:
- Companies are missing out on diverse talent that could bring fresh perspectives and skills to their teams.
- The industry is perpetuating a narrow definition of what makes a “good” programmer.
- Resources are being misallocated as both companies and candidates invest heavily in a process that may not yield the best results.
- There’s a growing disconnect between the skills tested in interviews and those required on the job.
Alternative Approaches
Recognizing these issues, some companies and thought leaders in the tech industry have begun exploring alternative hiring practices. Let’s look at some of these approaches:
1. Take-Home Projects
Some companies are opting for take-home coding assignments that more closely mimic real-world tasks. This approach allows candidates to work in a comfortable environment and showcase their practical skills.
2. Pair Programming Interviews
This method involves the candidate working alongside an existing team member on a real problem. It tests not just coding ability but also collaboration and communication skills.
3. Open Source Contributions
Evaluating a candidate’s contributions to open source projects can provide insight into their coding style, ability to work with others, and passion for technology.
4. Technical Discussions
Instead of coding on the spot, some interviews focus on in-depth discussions about past projects, architectural decisions, and problem-solving approaches.
5. Skill-Based Assessments
Platforms like HackerRank and Codility offer standardized coding tests that can be completed in a less stressful environment while still evaluating technical skills.
The Role of Coding Education Platforms
As the debate around coding interviews continues, platforms like AlgoCademy play a crucial role in bridging the gap between traditional interview preparation and practical skill development. These platforms offer several advantages:
1. Comprehensive Skill Development
While preparing candidates for algorithmic interviews, they also focus on broader programming concepts and real-world application.
2. Adaptive Learning
AI-powered systems can tailor the learning experience to each individual’s strengths and weaknesses.
3. Practical Application
Interactive tutorials and projects help learners apply concepts in contexts similar to real-world scenarios.
4. Continuous Learning
These platforms encourage ongoing skill development, not just cramming for interviews.
The Future of Tech Hiring
As we look to the future, it’s clear that the tech industry needs to evolve its hiring practices to keep pace with the changing landscape of software development. Here are some trends and predictions:
1. Holistic Evaluation
We’re likely to see a move towards more comprehensive assessment methods that consider a candidate’s full range of skills and experiences.
2. Emphasis on Soft Skills
As teamwork and communication become increasingly important in tech roles, interviews may place greater emphasis on these soft skills.
3. Continuous Assessment
Some companies may opt for probationary periods or extended evaluation processes rather than relying on a single high-stakes interview.
4. AI-Assisted Hiring
Artificial intelligence could play a role in initial candidate screening and skill assessment, potentially reducing bias and improving efficiency.
5. Focus on Diversity and Inclusion
There will likely be a continued push to develop hiring practices that promote diversity and inclusion in the tech industry.
Balancing Tradition and Innovation
While it’s clear that traditional coding interviews have their flaws, it’s important to recognize that they’ve played a significant role in shaping the tech industry as we know it. The challenge moving forward is to strike a balance between preserving what works and innovating to address the shortcomings.
Here are some ways companies might approach this balance:
1. Hybrid Approaches
Combining traditional algorithmic questions with more practical, job-specific tasks could provide a more rounded assessment of a candidate’s abilities.
2. Customized Interviews
Tailoring the interview process to the specific role and level of experience required could help ensure that the assessment is relevant and fair.
3. Ongoing Skill Development
Companies might consider investing in ongoing training and development for their employees, rather than relying solely on pre-hire assessments.
4. Feedback Loops
Regularly evaluating the effectiveness of hiring practices and correlating them with on-the-job performance could help refine the process over time.
The Role of Candidates
While much of the responsibility for improving hiring practices lies with companies, candidates also have a role to play in this evolving landscape:
1. Diverse Skill Development
Focusing on a broad range of skills, including both algorithmic problem-solving and practical development experience, can help candidates prepare for various interview styles.
2. Portfolio Building
Creating a strong portfolio of projects and contributions can provide tangible evidence of skills and passion for technology.
3. Continuous Learning
Embracing a mindset of lifelong learning and staying current with industry trends can make candidates more adaptable to changing interview practices.
4. Advocating for Fair Practices
Candidates can play a role in pushing for more relevant and inclusive hiring practices by providing feedback and engaging in discussions about the interview process.
Code Example: A Traditional Coding Interview Question
To illustrate the type of problem often encountered in traditional coding interviews, let’s look at a classic example: reversing a linked list. This is a common question that tests understanding of data structures and ability to manipulate pointers.
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverseLinkedList(head):
prev = None
current = head
while current is not None:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
# Example usage:
# Create a linked list: 1 -> 2 -> 3 -> 4 -> 5
head = ListNode(1)
head.next = ListNode(2)
head.next.next = ListNode(3)
head.next.next.next = ListNode(4)
head.next.next.next.next = ListNode(5)
# Reverse the linked list
new_head = reverseLinkedList(head)
# Print the reversed list
while new_head:
print(new_head.val, end=" -> ")
new_head = new_head.next
print("None")
This code demonstrates a solution to reverse a singly linked list, a common data structure manipulation problem. While this showcases important computer science concepts, critics argue that such questions may not reflect the day-to-day work of many software development roles.
Conclusion: Evolving for the Future
The question “Are traditional coding interviews failing us?” doesn’t have a simple yes or no answer. While these interviews have served the industry for years, it’s clear that there’s room for improvement and evolution.
As the tech industry continues to grow and diversify, our hiring practices must adapt to ensure we’re building teams that can tackle the complex challenges of tomorrow. This may mean moving beyond algorithmic puzzles to assess a broader range of skills, including practical coding ability, problem-solving in real-world contexts, collaboration, and adaptability.
The future of tech hiring is likely to be more holistic, considering a candidate’s full spectrum of abilities and experiences. It may involve a combination of traditional methods and innovative approaches, tailored to the specific needs of each role and organization.
Platforms like AlgoCademy will continue to play a crucial role in this landscape, helping bridge the gap between academic knowledge and practical skills, and preparing candidates for whatever form the tech interview of the future may take.
Ultimately, the goal remains the same: to identify and nurture the best talent to drive innovation and progress in the tech industry. By critically examining our hiring practices and being open to change, we can ensure that we’re not just maintaining the status quo, but actively shaping a more inclusive, efficient, and effective future for tech recruitment.
As we move forward, it’s essential for companies, candidates, and educational platforms to work together in refining the hiring process. By doing so, we can create a tech industry that not only excels in innovation but also in identifying and developing diverse talent that will shape the technological landscape for years to come.