Longest Substring with At Most K Distinct Characters: A Comprehensive Guide
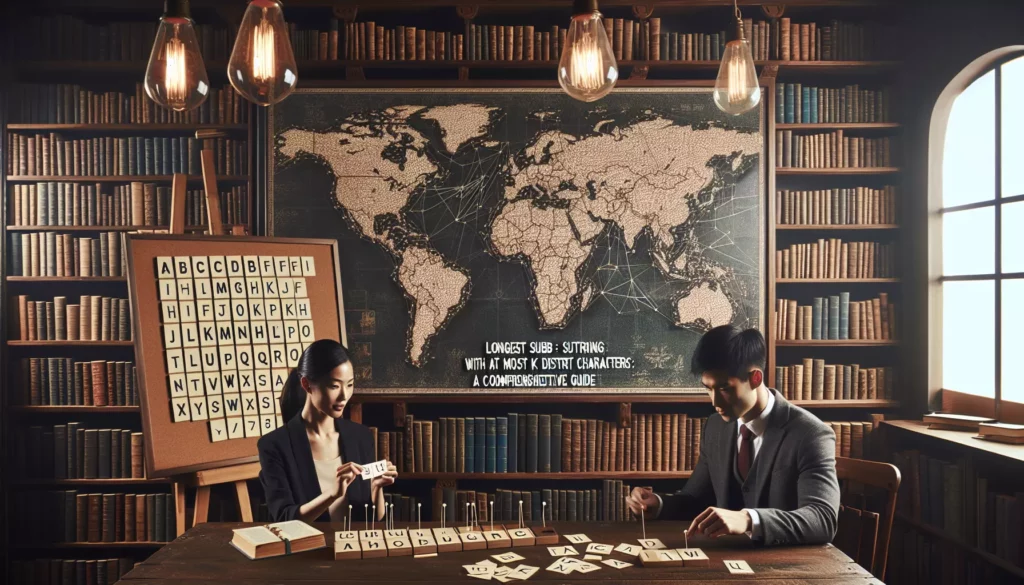
In the world of coding interviews and algorithmic problem-solving, string manipulation problems are a common occurrence. One such problem that often appears in technical interviews, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), is finding the “Longest Substring with At Most K Distinct Characters.” This problem not only tests a candidate’s ability to work with strings but also their understanding of data structures and algorithmic thinking.
In this comprehensive guide, we’ll dive deep into this problem, understand its nuances, explore multiple approaches to solve it, and discuss its relevance in the broader context of coding education and interview preparation. Whether you’re a beginner looking to improve your coding skills or an experienced developer preparing for a technical interview, this article will provide valuable insights and practical knowledge.
Understanding the Problem
Before we delve into the solutions, let’s clearly define the problem:
Given a string s and an integer k, find the length of the longest substring that contains at most k distinct characters.
For example:
- If s = “aabacbebebe” and k = 3, the answer would be 7. The longest substring with at most 3 distinct characters is “cbebebe”.
- If s = “aaaa” and k = 2, the answer would be 4. The entire string is the longest substring with at most 2 distinct characters.
This problem tests several key concepts:
- String manipulation
- Sliding window technique
- Hash map usage
- Time and space complexity optimization
Approach 1: Brute Force
Let’s start with the most straightforward approach – the brute force method. While this isn’t the most efficient solution, it’s often a good starting point to understand the problem better.
Algorithm:
- Generate all possible substrings of the given string.
- For each substring, count the number of distinct characters.
- If the count is less than or equal to k, update the maximum length if necessary.
- Return the maximum length found.
Implementation:
def longest_substring_with_k_distinct(s: str, k: int) -> int:
max_length = 0
for i in range(len(s)):
for j in range(i, len(s)):
substring = s[i:j+1]
if len(set(substring)) <= k:
max_length = max(max_length, len(substring))
return max_length
# Example usage
s = "aabacbebebe"
k = 3
result = longest_substring_with_k_distinct(s, k)
print(f"The length of the longest substring with at most {k} distinct characters is: {result}")
While this approach works, it has a time complexity of O(n^3), where n is the length of the string. This is because we have two nested loops to generate all substrings (O(n^2)), and for each substring, we’re creating a set to count distinct characters (O(n)). Clearly, this solution won’t be efficient for large inputs and wouldn’t be acceptable in a coding interview for a top tech company.
Approach 2: Sliding Window with Hash Map
A more efficient approach to this problem involves using the sliding window technique along with a hash map. This method allows us to solve the problem in a single pass through the string.
Algorithm:
- Initialize two pointers, left and right, both pointing to the start of the string.
- Use a hash map to keep track of characters and their frequencies in the current window.
- Move the right pointer to expand the window, adding characters to the hash map.
- If the number of distinct characters (keys in the hash map) exceeds k, move the left pointer to shrink the window, removing characters from the hash map.
- Update the maximum length at each step.
- Return the maximum length found.
Implementation:
from collections import defaultdict
def longest_substring_with_k_distinct(s: str, k: int) -> int:
char_frequency = defaultdict(int)
max_length = 0
left = 0
for right in range(len(s)):
char_frequency[s[right]] += 1
while len(char_frequency) > k:
char_frequency[s[left]] -= 1
if char_frequency[s[left]] == 0:
del char_frequency[s[left]]
left += 1
max_length = max(max_length, right - left + 1)
return max_length
# Example usage
s = "aabacbebebe"
k = 3
result = longest_substring_with_k_distinct(s, k)
print(f"The length of the longest substring with at most {k} distinct characters is: {result}")
This solution has a time complexity of O(n), where n is the length of the string. We only iterate through the string once, and the operations inside the loop (adding/removing from the hash map) are constant time on average. The space complexity is O(k) since the hash map will contain at most k distinct characters.
Understanding the Sliding Window Technique
The sliding window technique is a powerful tool in solving many string and array problems efficiently. It’s particularly useful when we need to find or calculate something among all the contiguous subarrays (or substrings) of a given size.
In our problem, the window’s size is not fixed, but its content is constrained (at most k distinct characters). The technique works as follows:
- We start with a window of size 1 (left and right pointers at the start).
- We expand the window by moving the right pointer, adding elements to our window.
- When our window violates the constraint (more than k distinct characters), we shrink it from the left until it’s valid again.
- At each step, we update our result if necessary.
This approach is efficient because we never have to backtrack. Each element is added to the window once and removed at most once, leading to a linear time complexity.
Time and Space Complexity Analysis
Understanding the time and space complexity of our solutions is crucial, especially when preparing for technical interviews at top tech companies.
Brute Force Approach:
- Time Complexity: O(n^3)
- Generating all substrings: O(n^2)
- Counting distinct characters for each substring: O(n)
- Space Complexity: O(n) for storing the set of distinct characters
Sliding Window Approach:
- Time Complexity: O(n)
- We iterate through the string once
- Hash map operations are O(1) on average
- Space Complexity: O(k)
- The hash map stores at most k distinct characters
The sliding window approach is clearly superior, especially for large inputs. This kind of optimization is exactly what interviewers at FAANG companies are looking for.
Variations and Related Problems
Understanding this problem and its solution opens the door to solving many related problems. Here are a few variations you might encounter:
- Longest Substring with At Most Two Distinct Characters: This is a special case of our problem where k = 2. The same sliding window approach can be used.
- Fruit Into Baskets: This is a leetcode problem that is essentially the same as the above, but wrapped in a story about picking fruit.
- Longest Substring Without Repeating Characters: Instead of at most k distinct characters, we want all characters to be unique. The sliding window approach still applies, but we shrink the window as soon as we see a repeat.
- Minimum Window Substring: Given two strings s and t, return the minimum window in s which will contain all the characters in t. This uses a similar sliding window approach but with a more complex condition for a valid window.
Practicing these related problems will help reinforce your understanding of the sliding window technique and prepare you for variations that might come up in interviews.
Interview Tips and Tricks
When facing this problem or similar ones in a coding interview, keep these tips in mind:
- Clarify the Problem: Always start by asking clarifying questions. For example, “Are we considering uppercase and lowercase letters as distinct?”, “What should I return if k is greater than the number of distinct characters in the string?”, etc.
- Think Aloud: Even if you immediately recognize the optimal solution, walk through your thought process. Start with the brute force approach, explain why it’s not optimal, and then work your way to the efficient solution.
- Optimize: After implementing a working solution, always think about how you can optimize it further. Can you reduce the space complexity? Can you solve it in a single pass?
- Test Your Code: Before saying you’re done, walk through your code with a few test cases, including edge cases (empty string, k = 0, k greater than the string length, etc.).
- Analyze Complexity: Be prepared to discuss the time and space complexity of your solution. This shows you understand the efficiency of your algorithm.
The Importance of String Manipulation in Coding Interviews
String manipulation problems like “Longest Substring with At Most K Distinct Characters” are popular in coding interviews for several reasons:
- Ubiquity: Strings are one of the most common data types in programming. Nearly every application deals with strings in some form.
- Complexity: String problems often involve multiple concepts (like our problem combines string manipulation, hash maps, and the sliding window technique).
- Efficiency Challenges: Many string problems have obvious brute force solutions but require careful thinking to optimize.
- Language Proficiency: String manipulation often tests a candidate’s familiarity with built-in language features and standard library functions.
Mastering string manipulation problems is therefore crucial for success in coding interviews, especially for positions at top tech companies.
How AlgoCademy Can Help
Preparing for coding interviews, especially for positions at FAANG companies, can be challenging. This is where platforms like AlgoCademy come in handy. AlgoCademy provides:
- Structured Learning Paths: From basic string manipulation to advanced algorithms, AlgoCademy offers a structured approach to learning.
- Interactive Coding Environments: Practice problems like “Longest Substring with At Most K Distinct Characters” in a browser-based coding environment.
- AI-Powered Assistance: Get hints and explanations tailored to your progress and learning style.
- Comprehensive Problem Sets: Practice with a wide range of problems, including variations of popular interview questions.
- Performance Tracking: Monitor your progress and identify areas for improvement.
By leveraging these resources, you can systematically improve your coding skills and increase your chances of success in technical interviews.
Conclusion
The “Longest Substring with At Most K Distinct Characters” problem is a classic example of how seemingly simple string problems can test a wide range of programming skills. From brute force to optimized solutions, this problem challenges you to think critically about efficiency and algorithm design.
Remember, the key to mastering such problems is consistent practice and a methodical approach to problem-solving. Start with understanding the problem thoroughly, consider multiple approaches, optimize your solution, and always analyze the time and space complexity.
As you prepare for coding interviews, especially for positions at top tech companies, make sure to practice a wide variety of string manipulation problems. Platforms like AlgoCademy can provide the structure, resources, and guidance needed to elevate your coding skills to the next level.
Keep coding, keep learning, and approach each problem as an opportunity to grow as a programmer. With dedication and the right resources, you’ll be well-prepared to tackle any coding challenge that comes your way in your technical interviews and beyond.