Mastering the “Subarray Sum Equals K” Problem: A Comprehensive Guide
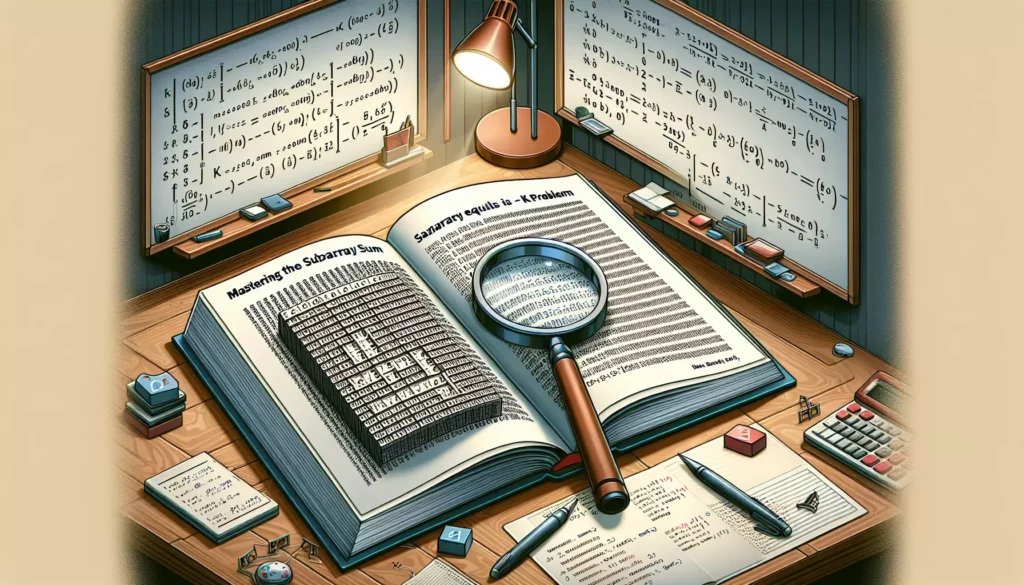
Welcome to another exciting deep dive into algorithmic problem-solving! Today, we’re tackling a classic coding challenge that frequently appears in technical interviews, especially those conducted by top tech companies. The problem we’ll be dissecting is known as “Subarray Sum Equals K.” This problem is not only a favorite among interviewers but also serves as an excellent exercise to sharpen your algorithmic thinking and coding skills.
Understanding the Problem
Before we dive into the solution, let’s clearly define the problem:
Given an array of integers
nums
and an integerk
, return the total number of continuous subarrays whose sum equals tok
.
At first glance, this problem might seem straightforward. However, it presents several challenges that make it an ideal candidate for testing a programmer’s problem-solving abilities and understanding of data structures.
Breaking Down the Problem
To solve this problem efficiently, we need to consider a few key points:
- We’re looking for continuous subarrays, meaning the elements must be adjacent in the original array.
- The array can contain both positive and negative integers.
- The sum of the subarray should exactly equal
k
. - We need to count all such subarrays, not just find one.
These considerations will guide our approach to solving the problem efficiently.
Naive Approach: Brute Force
Let’s start with the most straightforward approach – the brute force method. This approach involves checking every possible subarray and counting those whose sum equals k
.
class Solution {
public:
int subarraySum(vector<int>& nums, int k) {
int count = 0;
int n = nums.size();
for (int start = 0; start < n; start++) {
int sum = 0;
for (int end = start; end < n; end++) {
sum += nums[end];
if (sum == k) {
count++;
}
}
}
return count;
}
};
While this approach works, it has a time complexity of O(n^2), where n is the length of the input array. For large arrays, this solution becomes impractical due to its inefficiency.
Optimized Approach: Using Cumulative Sum and Hash Map
To improve upon the brute force method, we can use a more efficient approach that utilizes the concept of cumulative sum and a hash map. This approach allows us to solve the problem in O(n) time complexity.
The key idea behind this approach is:
- Calculate the cumulative sum as we iterate through the array.
- Use a hash map to store the frequency of cumulative sums we’ve seen so far.
- For each cumulative sum, check if (sum – k) exists in our hash map. If it does, it means we’ve found a subarray with sum k.
Let’s implement this approach:
class Solution {
public:
int subarraySum(vector<int>& nums, int k) {
unordered_map<int, int> sumFrequency;
sumFrequency[0] = 1; // Initialize with 0 sum seen once
int count = 0;
int sum = 0;
for (int num : nums) {
sum += num;
if (sumFrequency.find(sum - k) != sumFrequency.end()) {
count += sumFrequency[sum - k];
}
sumFrequency[sum]++;
}
return count;
}
};
Breaking Down the Optimized Solution
Let’s break down this solution to understand each part:
- We use an
unordered_map
calledsumFrequency
to store the frequency of cumulative sums we’ve seen. - We initialize
sumFrequency[0] = 1
to account for the case where the entire subarray from the beginning sums tok
. - We iterate through the array, keeping track of the cumulative sum in the
sum
variable. - For each element, we check if
(sum - k)
exists in our map. If it does, we’ve found a subarray with sumk
, and we add its frequency to our count. - We then update the frequency of the current sum in our map.
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our optimized solution:
- Time Complexity: O(n), where n is the length of the input array. We iterate through the array once, and each operation within the loop (hash map lookup and update) is O(1) on average.
- Space Complexity: O(n) in the worst case, where we might need to store all cumulative sums in the hash map.
This is a significant improvement over the brute force approach, especially for large input arrays.
Common Pitfalls and Edge Cases
When solving this problem, be aware of these common pitfalls and edge cases:
- Zero Sum Subarrays: Don’t forget to initialize
sumFrequency[0] = 1
to handle cases where a subarray starting from the beginning sums tok
. - Negative Numbers: The array can contain negative numbers, which is why we can’t use a sliding window approach that works for positive numbers only.
- Large Numbers: Be cautious of integer overflow when dealing with large numbers or long arrays. Consider using long long for sum calculations if necessary.
- Empty Array: Handle the case of an empty input array gracefully.
Variations of the Problem
The “Subarray Sum Equals K” problem has several variations that you might encounter:
- Maximum Length Subarray with Sum K: Instead of counting all subarrays, find the length of the longest subarray with sum K.
- Minimum Length Subarray with Sum K: Find the length of the shortest subarray with sum K.
- Subarray Sum Divisible by K: Count the number of subarrays where the sum is divisible by K.
- Subarray Product Less Than K: Count the number of subarrays where the product of elements is less than K.
Understanding the core concept of this problem will help you tackle these variations more effectively.
Real-World Applications
The “Subarray Sum Equals K” problem and its variations have several real-world applications:
- Financial Analysis: Identifying periods in financial data where the cumulative sum reaches a specific target, useful in stock market analysis or budget tracking.
- Network Packet Analysis: Finding sequences of network packets that sum to a particular size, which could be useful in network traffic analysis or intrusion detection.
- Image Processing: In image processing, this algorithm can be used to find regions in an image that sum to a particular intensity value.
- Genomics: Analyzing DNA sequences for specific patterns or cumulative properties.
Interview Strategies
If you encounter this problem or a similar one in an interview, here are some strategies to keep in mind:
- Clarify the Problem: Make sure you understand all aspects of the problem. Ask about the range of input values, array size, and any other constraints.
- Discuss the Brute Force Approach: Even if you immediately recognize the optimal solution, briefly mentioning the brute force approach shows your problem-solving process.
- Explain Your Thought Process: As you work towards the optimal solution, explain your thinking. This gives the interviewer insight into your problem-solving skills.
- Optimize Step-by-Step: If you start with a less efficient solution, explain how you would optimize it. This demonstrates your ability to improve and refine your code.
- Analyze Complexity: Be prepared to discuss the time and space complexity of your solution.
- Consider Edge Cases: Mention and handle edge cases in your code, such as empty arrays or arrays with a single element.
- Test Your Code: If time permits, walk through your code with a small example to demonstrate its correctness.
Practice and Further Learning
To master this problem and similar ones, consider the following steps:
- Practice Variations: Solve the variations mentioned earlier to deepen your understanding of the concept.
- Implement in Different Languages: Try implementing the solution in languages you’re less familiar with to broaden your skills.
- Optimize for Space: Challenge yourself to solve the problem with O(1) space complexity, even if it means sacrificing some time efficiency.
- Explore Related Problems: Look into other problems involving subarrays or cumulative sums, such as “Maximum Subarray” or “Continuous Subarray Sum”.
- Study Hash Maps: Deepen your understanding of hash maps and their applications in solving array-related problems.
Conclusion
The “Subarray Sum Equals K” problem is a fantastic example of how a seemingly simple question can test a wide range of programming skills and concepts. By mastering this problem, you’re not just learning how to count subarrays; you’re honing your ability to think critically about data structures, recognize patterns, and optimize algorithms.
Remember, the key to excelling in coding interviews and becoming a proficient programmer is not just about memorizing solutions, but understanding the underlying principles and being able to apply them creatively to new problems. Keep practicing, stay curious, and always look for ways to improve your solutions.
Happy coding, and may your subarrays always sum up to success!