Wildcard Matching: Mastering Pattern Matching in Programming
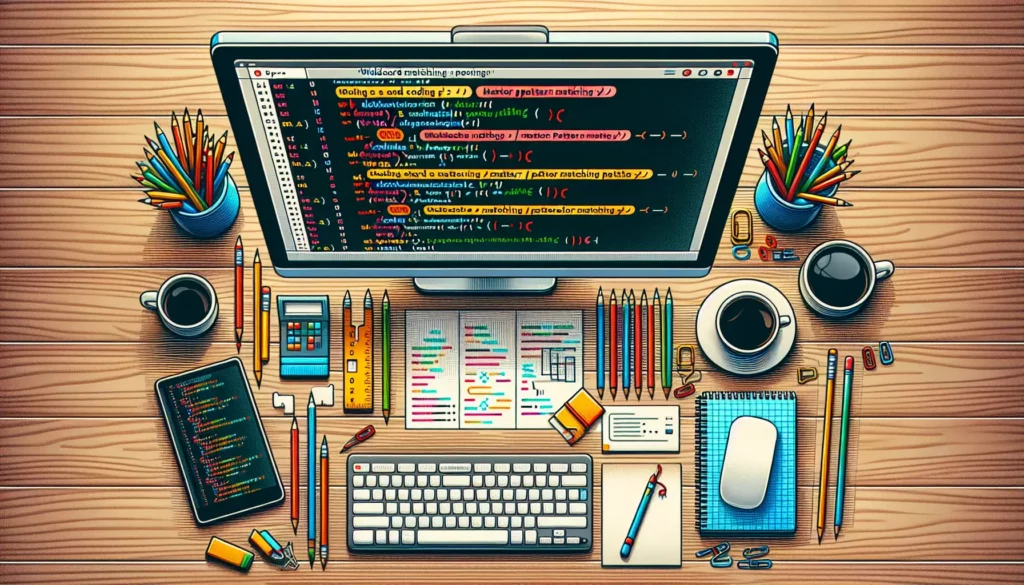
In the vast landscape of programming challenges, wildcard matching stands out as a fundamental problem that tests a developer’s ability to handle pattern recognition and string manipulation. This concept is not only crucial for coding interviews but also finds practical applications in various real-world scenarios. In this comprehensive guide, we’ll dive deep into wildcard matching, exploring its intricacies, implementation strategies, and how it can elevate your problem-solving skills.
What is Wildcard Matching?
Wildcard matching, at its core, is a pattern matching technique where special characters (wildcards) can represent one or more characters in a string. The two most common wildcard characters are:
- ‘?’ – Matches any single character
- ‘*’ – Matches any sequence of characters (including an empty sequence)
The goal is to determine whether a given string matches a pattern that includes these wildcard characters. This problem is often presented in coding interviews and is a staple in many algorithm courses due to its ability to test multiple programming concepts simultaneously.
The Importance of Wildcard Matching in Programming
Understanding and implementing wildcard matching is crucial for several reasons:
- File System Operations: Many file systems use wildcard patterns to search for files or directories.
- Text Processing: It’s extensively used in text editors and search functionalities.
- Regular Expressions: Wildcard matching is a simplified version of regex, serving as a stepping stone to more complex pattern matching.
- Database Queries: SQL’s LIKE operator uses similar pattern matching concepts.
- Problem-Solving Skills: It challenges programmers to think about string manipulation, recursion, and dynamic programming.
Approaching the Wildcard Matching Problem
When tackling a wildcard matching problem, there are several approaches you can consider. Let’s explore them in order of increasing complexity and efficiency.
1. Recursive Approach
The recursive approach is often the most intuitive way to solve the wildcard matching problem. It breaks down the problem into smaller subproblems and solves them recursively.
def is_match(s: str, p: str) -> bool:
if not p:
return not s
first_match = bool(s) and p[0] in {s[0], '?'}
if p[0] == '*':
return is_match(s, p[1:]) or (bool(s) and is_match(s[1:], p))
else:
return first_match and is_match(s[1:], p[1:])
This recursive solution is elegant but can be inefficient for large inputs due to redundant computations.
2. Dynamic Programming Approach
To optimize the recursive solution, we can use dynamic programming to avoid redundant calculations. This approach uses a 2D array to store intermediate results.
def is_match(s: str, p: str) -> bool:
m, n = len(s), len(p)
dp = [[False] * (n + 1) for _ in range(m + 1)]
dp[0][0] = True
for j in range(1, n + 1):
if p[j-1] == '*':
dp[0][j] = dp[0][j-1]
for i in range(1, m + 1):
for j in range(1, n + 1):
if p[j-1] == '*':
dp[i][j] = dp[i][j-1] or dp[i-1][j]
elif p[j-1] in {s[i-1], '?'}:
dp[i][j] = dp[i-1][j-1]
return dp[m][n]
This dynamic programming solution has a time complexity of O(mn) and space complexity of O(mn), where m and n are the lengths of the string and pattern respectively.
3. Greedy Approach
For an even more efficient solution, we can use a greedy approach. This method is particularly effective when dealing with patterns that have many ‘*’ characters.
def is_match(s: str, p: str) -> bool:
s_len, p_len = len(s), len(p)
s_idx = p_idx = 0
star_idx = s_tmp_idx = -1
while s_idx < s_len:
if p_idx < p_len and p[p_idx] in {s[s_idx], '?'}:
s_idx += 1
p_idx += 1
elif p_idx < p_len and p[p_idx] == '*':
star_idx = p_idx
s_tmp_idx = s_idx
p_idx += 1
elif star_idx == -1:
return False
else:
p_idx = star_idx + 1
s_idx = s_tmp_idx + 1
s_tmp_idx = s_idx
return all(x == '*' for x in p[p_idx:])
This greedy approach has a time complexity of O(m + n) and space complexity of O(1), making it highly efficient for large inputs.
Common Pitfalls and Edge Cases
When implementing wildcard matching, be aware of these common pitfalls and edge cases:
- Empty Strings: Always consider cases where either the string or pattern might be empty.
- All Stars: A pattern consisting of only ‘*’ characters should match any string.
- Consecutive Stars: Multiple consecutive ‘*’ characters are equivalent to a single ‘*’.
- Leading/Trailing Stars: Pay special attention to patterns that start or end with ‘*’.
- Long Strings with Many Stars: Ensure your solution can handle very long strings and patterns with numerous ‘*’ characters efficiently.
Practical Applications of Wildcard Matching
Understanding wildcard matching opens doors to solving various real-world problems efficiently. Here are some practical applications:
1. File System Search
Many command-line interfaces and file explorers use wildcard matching for searching files. For example:
ls *.txt # List all .txt files
find . -name "log*.log" # Find all log files starting with "log"
2. Database Queries
SQL’s LIKE operator uses similar pattern matching concepts. For instance:
SELECT * FROM users WHERE name LIKE 'John%';
3. Text Editors and IDEs
Many text editors and IDEs use wildcard matching for search and replace operations. For example, searching for “test*.py” might find all Python test files.
4. Configuration Management
In configuration files, wildcard patterns are often used to specify groups of files or resources. For instance, in a .gitignore file:
*.log
build/*
!build/important.txt
5. URL Routing
Some web frameworks use wildcard-like patterns for URL routing. For example:
@app.route('/user/<username>')
def user_profile(username):
# ...
Advanced Concepts Related to Wildcard Matching
As you become more comfortable with basic wildcard matching, you can explore these advanced related concepts:
1. Regular Expressions
Regular expressions (regex) are a more powerful and flexible form of pattern matching. They include additional metacharacters and constructs that allow for more complex pattern definitions.
2. Finite Automata
The theory behind pattern matching often involves finite automata. Understanding these can provide deeper insights into how pattern matching algorithms work.
3. Approximate String Matching
This involves finding strings that match a pattern approximately (allowing for a certain number of errors). It’s useful in spell checkers and DNA sequence alignment.
4. Suffix Trees and Arrays
These are advanced data structures that can be used for efficient string matching and are often employed in more complex pattern matching scenarios.
Improving Your Wildcard Matching Skills
To become proficient in wildcard matching and related string manipulation problems, consider the following tips:
- Practice Regularly: Solve wildcard matching problems on platforms like LeetCode, HackerRank, or CodeSignal.
- Understand the Underlying Concepts: Don’t just memorize solutions. Understand the principles of dynamic programming, recursion, and greedy algorithms.
- Analyze Different Approaches: For each problem, try to come up with multiple solutions and analyze their time and space complexities.
- Implement from Scratch: Try implementing wildcard matching without relying on built-in functions or libraries.
- Review Real-World Code: Look at how wildcard matching is implemented in open-source projects or standard libraries.
- Teach Others: Explaining the concept to others can solidify your understanding and reveal any gaps in your knowledge.
Conclusion
Wildcard matching is a fundamental concept in computer science that bridges the gap between simple string comparison and complex pattern recognition. By mastering this skill, you not only prepare yourself for coding interviews but also gain insights into efficient algorithm design and practical problem-solving techniques.
Remember, the key to excelling in wildcard matching lies in understanding the problem deeply, considering various approaches, and practicing consistently. As you progress, you’ll find that the principles learned here apply to a wide range of programming challenges, making you a more versatile and capable developer.
Whether you’re preparing for a technical interview, working on a file system utility, or diving into more advanced string processing tasks, the skills you develop through wildcard matching will serve you well throughout your programming journey. Keep practicing, stay curious, and don’t hesitate to explore the more advanced topics as you grow more comfortable with the basics.