Mastering the Longest Repeating Subsequence Problem: A Comprehensive Guide
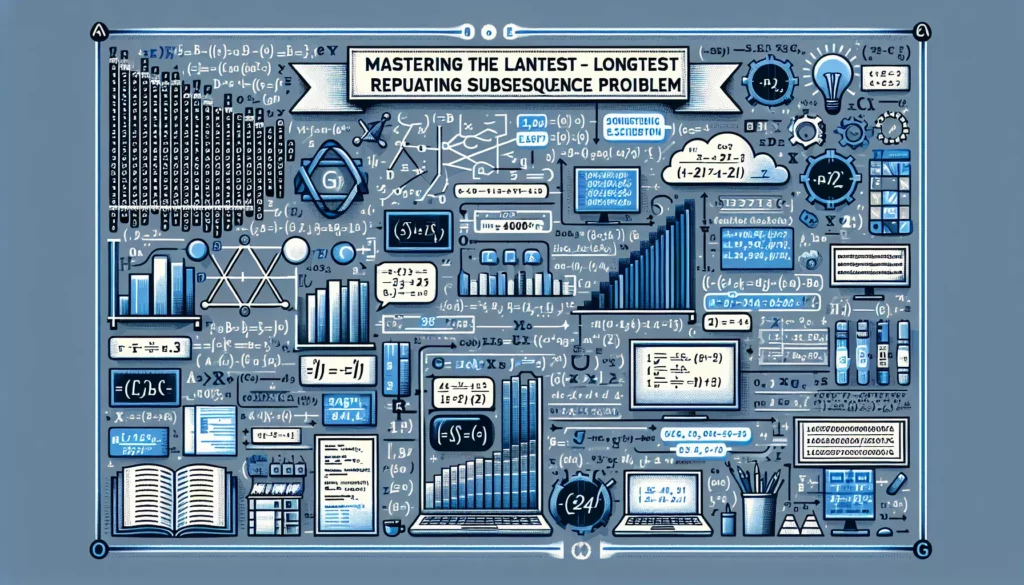
In the vast world of algorithmic problem-solving, the Longest Repeating Subsequence (LRS) problem stands out as an intriguing challenge that tests a programmer’s ability to manipulate strings and apply dynamic programming concepts. This problem is not just an academic exercise; it has practical applications in fields like bioinformatics, data compression, and pattern recognition. In this comprehensive guide, we’ll dive deep into the LRS problem, understand its nuances, and explore efficient solutions to tackle it.
What is the Longest Repeating Subsequence?
Before we delve into the solution, let’s clearly define what we mean by the Longest Repeating Subsequence:
The Longest Repeating Subsequence in a string is the longest subsequence that appears at least twice in the string, but the characters in the subsequence should not use the same index in the original string.
For example, in the string “AABEBCDD”:
- The subsequence “ABD” appears twice, but it uses the same index for ‘A’ in both occurrences, so it’s not a valid repeating subsequence.
- The subsequence “ABD” where we take ‘A’ from index 0, ‘B’ from index 3, and ‘D’ from index 6 for the first occurrence, and ‘A’ from index 1, ‘B’ from index 3, and ‘D’ from index 7 for the second occurrence is a valid repeating subsequence.
- The longest such subsequence in this string is “ABD”, with a length of 3.
Why is the Longest Repeating Subsequence Problem Important?
The LRS problem is significant for several reasons:
- Pattern Recognition: It helps in identifying repeated patterns in data, which is crucial in various fields like genetics and data analysis.
- String Processing: It’s a fundamental problem in string algorithms, helping developers improve their skills in string manipulation.
- Dynamic Programming Practice: The problem serves as an excellent exercise for understanding and applying dynamic programming techniques.
- Interview Preparation: It’s a common question in technical interviews, especially for roles that involve algorithmic problem-solving.
Approaching the Problem
To solve the Longest Repeating Subsequence problem, we’ll use a dynamic programming approach. The key insight is that this problem is similar to finding the Longest Common Subsequence (LCS) of the string with itself, but with an additional constraint that the same index should not be used twice.
The Algorithm
- Create a 2D table (dp) of size (n+1) x (n+1), where n is the length of the input string.
- Initialize the first row and column of the table with 0.
- Iterate through the string:
- If the characters at positions i and j are the same and i != j, dp[i][j] = dp[i-1][j-1] + 1
- Otherwise, dp[i][j] = max(dp[i-1][j], dp[i][j-1])
- The value in dp[n][n] will give the length of the longest repeating subsequence.
Implementing the Solution
Let’s implement this algorithm in Python:
def longest_repeating_subsequence(s):
n = len(s)
dp = [[0] * (n + 1) for _ in range(n + 1)]
for i in range(1, n + 1):
for j in range(1, n + 1):
if s[i-1] == s[j-1] and i != j:
dp[i][j] = dp[i-1][j-1] + 1
else:
dp[i][j] = max(dp[i-1][j], dp[i][j-1])
return dp[n][n]
# Example usage
s = "AABEBCDD"
print(f"Length of Longest Repeating Subsequence: {longest_repeating_subsequence(s)}")
This implementation will return the length of the longest repeating subsequence. In our example, it will output:
Length of Longest Repeating Subsequence: 3
Understanding the Time and Space Complexity
Let’s analyze the complexity of our solution:
- Time Complexity: O(n^2), where n is the length of the input string. This is because we’re filling a 2D table of size n x n.
- Space Complexity: O(n^2) as well, due to the 2D dp table we’re using.
While this solution is efficient for most practical purposes, for very large strings, we might need to consider more optimized approaches or approximation algorithms.
Extending the Solution: Finding the Actual Subsequence
So far, we’ve only found the length of the longest repeating subsequence. But what if we want to find the actual subsequence? We can modify our algorithm to backtrack through the dp table and construct the subsequence. Here’s how we can do it:
def longest_repeating_subsequence_with_sequence(s):
n = len(s)
dp = [[0] * (n + 1) for _ in range(n + 1)]
for i in range(1, n + 1):
for j in range(1, n + 1):
if s[i-1] == s[j-1] and i != j:
dp[i][j] = dp[i-1][j-1] + 1
else:
dp[i][j] = max(dp[i-1][j], dp[i][j-1])
# Backtracking to find the subsequence
subsequence = []
i, j = n, n
while i > 0 and j > 0:
if s[i-1] == s[j-1] and i != j:
subsequence.append(s[i-1])
i -= 1
j -= 1
elif dp[i-1][j] > dp[i][j-1]:
i -= 1
else:
j -= 1
return dp[n][n], ''.join(reversed(subsequence))
# Example usage
s = "AABEBCDD"
length, subsequence = longest_repeating_subsequence_with_sequence(s)
print(f"Length of Longest Repeating Subsequence: {length}")
print(f"Longest Repeating Subsequence: {subsequence}")
This extended version will output:
Length of Longest Repeating Subsequence: 3
Longest Repeating Subsequence: ABD
Variations and Related Problems
The Longest Repeating Subsequence problem is part of a family of string and subsequence problems. Understanding this problem can help you tackle similar challenges. Here are some related problems:
- Longest Common Subsequence (LCS): Find the longest subsequence common to two sequences.
- Longest Palindromic Subsequence: Find the longest subsequence of a string that reads the same backwards as forwards.
- Shortest Common Supersequence: Find the shortest supersequence that contains two given sequences as subsequences.
- Edit Distance: Find the minimum number of operations required to transform one string into another.
Each of these problems can be solved using dynamic programming techniques similar to what we’ve used for the LRS problem.
Practical Applications
Understanding and implementing the Longest Repeating Subsequence algorithm has several real-world applications:
- Bioinformatics: In DNA sequence analysis, finding repeating subsequences can help identify important genetic markers or repeated segments in genomes.
- Data Compression: Identifying repeating patterns in data can be used to develop more efficient compression algorithms.
- Plagiarism Detection: By finding common subsequences between documents, this algorithm can assist in identifying potential cases of plagiarism.
- Text Analysis: In natural language processing, identifying repeating patterns can help in understanding text structure and style.
- Cryptography: Analyzing repeating subsequences in encrypted text can be a step in cryptanalysis techniques.
Optimizing the Solution
While our current solution is efficient for most practical purposes, there are ways to optimize it further:
1. Space Optimization
We can reduce the space complexity from O(n^2) to O(n) by using only two rows of the dp table at a time. Here’s how we can implement this:
def optimized_longest_repeating_subsequence(s):
n = len(s)
dp = [[0] * (n + 1) for _ in range(2)]
for i in range(1, n + 1):
for j in range(1, n + 1):
if s[i-1] == s[j-1] and i != j:
dp[i % 2][j] = dp[(i-1) % 2][j-1] + 1
else:
dp[i % 2][j] = max(dp[(i-1) % 2][j], dp[i % 2][j-1])
return dp[n % 2][n]
# Example usage
s = "AABEBCDD"
print(f"Length of Longest Repeating Subsequence: {optimized_longest_repeating_subsequence(s)}")
This optimization reduces the space complexity to O(n) while maintaining the same time complexity of O(n^2).
2. Early Termination
In some cases, we can terminate the algorithm early if we know that the maximum possible length of the repeating subsequence has been reached. For example, if we find a repeating subsequence of length n/2 (where n is the length of the string), we know that this is the maximum possible length and can stop the algorithm.
Handling Edge Cases
When implementing the Longest Repeating Subsequence algorithm, it’s important to consider various edge cases:
- Empty String: The LRS of an empty string should be 0.
- String with All Unique Characters: If all characters in the string are unique, the LRS length will be 0.
- String with All Same Characters: If all characters are the same (e.g., “AAAA”), the LRS length will be the total length minus 1.
- Very Long Strings: For extremely long strings, consider using more efficient data structures or approximation algorithms to handle potential memory constraints.
Here’s an updated version of our function that handles these edge cases:
def robust_longest_repeating_subsequence(s):
n = len(s)
# Handle empty string
if n == 0:
return 0
# Handle string with all unique characters
if len(set(s)) == n:
return 0
# Handle string with all same characters
if len(set(s)) == 1:
return n - 1
# Proceed with the standard algorithm for other cases
dp = [[0] * (n + 1) for _ in range(n + 1)]
for i in range(1, n + 1):
for j in range(1, n + 1):
if s[i-1] == s[j-1] and i != j:
dp[i][j] = dp[i-1][j-1] + 1
else:
dp[i][j] = max(dp[i-1][j], dp[i][j-1])
return dp[n][n]
# Test cases
print(robust_longest_repeating_subsequence("")) # 0
print(robust_longest_repeating_subsequence("ABCDE")) # 0
print(robust_longest_repeating_subsequence("AAAAA")) # 4
print(robust_longest_repeating_subsequence("AABEBCDD")) # 3
Advanced Techniques and Further Study
For those looking to dive deeper into the world of string algorithms and dynamic programming, here are some advanced topics and techniques to explore:
- Suffix Arrays and Longest Common Prefix (LCP) Arrays: These data structures can be used to solve string problems, including variants of the LRS problem, more efficiently.
- Ukkonen’s Algorithm: An efficient algorithm for constructing suffix trees, which can be used to solve various string problems.
- Knuth-Morris-Pratt (KMP) Algorithm: While not directly related to LRS, understanding this string matching algorithm can enhance your overall string manipulation skills.
- Approximate String Matching: Explore algorithms that find subsequences that are “close enough” rather than exact matches.
- Parallel Computing for String Algorithms: Learn how to leverage multi-core processors or distributed systems to solve string problems on very large datasets.
Conclusion
The Longest Repeating Subsequence problem is a fascinating challenge that combines string manipulation with dynamic programming. By mastering this problem, you’re not just solving an isolated algorithmic puzzle; you’re developing skills that are applicable to a wide range of computational problems in bioinformatics, data analysis, and beyond.
Remember, the key to becoming proficient in algorithmic problem-solving is practice. Try implementing the LRS algorithm in different programming languages, experiment with various input strings, and challenge yourself to optimize the solution further. As you continue to explore and practice, you’ll find that the principles you’ve learned here will serve you well in tackling even more complex algorithmic challenges.
Happy coding, and may your journey through the world of algorithms be filled with exciting discoveries and elegant solutions!