Mastering Interleaving Strings: A Comprehensive Guide for Coding Interviews
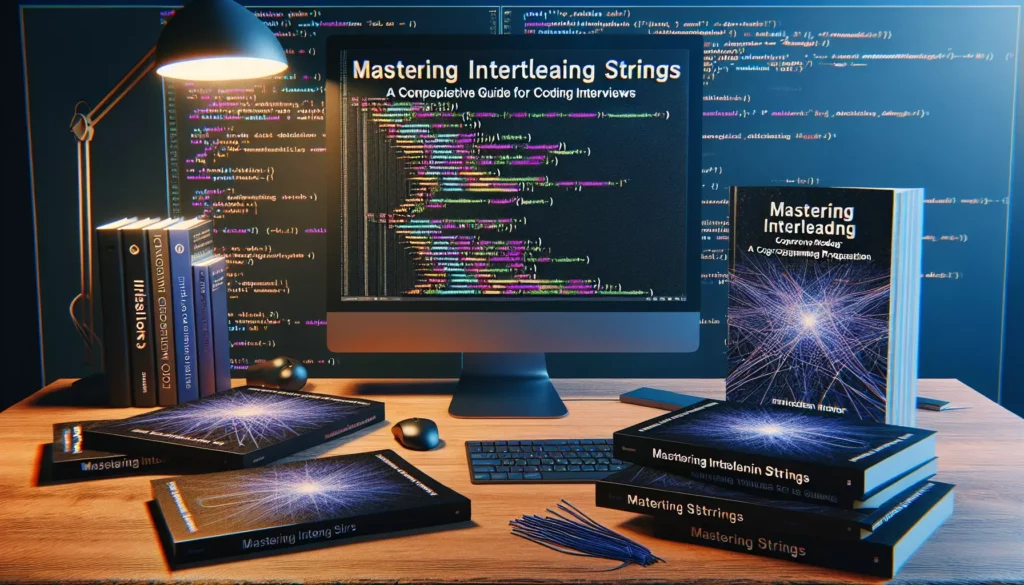
Welcome to another in-depth AlgoCademy tutorial! Today, we’re diving into a fascinating algorithmic problem that often appears in coding interviews, especially for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google). Our topic of focus is “Interleaving Strings” – a challenge that tests your understanding of string manipulation, dynamic programming, and problem-solving skills.
What are Interleaving Strings?
Before we delve into the problem and its solutions, let’s first understand what interleaving strings are. Interleaving strings is a process where we combine two strings in a way that maintains the original order of characters from both strings.
For example, given two strings “abc” and “def”, some valid interleavings would be:
- “abcdef”
- “adbecf”
- “adefbc”
- “dabecf”
However, “acbdef” would not be a valid interleaving because it doesn’t maintain the original order of characters in the first string “abc”.
The Interleaving Strings Problem
Now that we understand what interleaving strings are, let’s look at the problem statement often encountered in coding interviews:
Given three strings s1, s2, and s3, determine whether s3 is formed by an interleaving of s1 and s2.
For instance:
- If s1 = “aabcc”, s2 = “dbbca”, and s3 = “aadbbcbcac”, the function should return true.
- If s1 = “aabcc”, s2 = “dbbca”, and s3 = “aadbbbaccc”, the function should return false.
Approaching the Problem
As with many algorithmic problems, there are multiple ways to approach the Interleaving Strings problem. We’ll explore three different methods, each with its own pros and cons:
- Recursive Solution
- Dynamic Programming Solution
- 2D Dynamic Programming Solution
Let’s dive into each of these approaches in detail.
1. Recursive Solution
The recursive solution is often the most intuitive approach when first encountering this problem. The idea is to compare characters from s1 and s2 with s3, moving forward in the strings as we find matches.
Here’s a Python implementation of the recursive solution:
def is_interleave(s1, s2, s3):
if len(s1) + len(s2) != len(s3):
return False
def recursive_check(i, j, k):
if k == len(s3):
return i == len(s1) and j == len(s2)
if i < len(s1) and s1[i] == s3[k]:
if recursive_check(i+1, j, k+1):
return True
if j < len(s2) and s2[j] == s3[k]:
if recursive_check(i, j+1, k+1):
return True
return False
return recursive_check(0, 0, 0)
This solution works as follows:
- First, we check if the total length of s1 and s2 equals the length of s3. If not, s3 can’t be an interleaving of s1 and s2.
- We define a helper function
recursive_check
that takes three pointers: i for s1, j for s2, and k for s3. - If we’ve reached the end of s3 (k == len(s3)), we check if we’ve also reached the end of both s1 and s2.
- If the current character in s1 matches the current character in s3, we recursively check the next characters.
- Similarly, if the current character in s2 matches the current character in s3, we recursively check the next characters.
- If neither match, we return False.
While this solution is intuitive, it has a time complexity of O(2^(m+n)), where m and n are the lengths of s1 and s2 respectively. This is because for each character, we have two choices: either match with s1 or s2. This exponential time complexity makes the recursive solution impractical for large inputs.
2. Dynamic Programming Solution
To improve upon the recursive solution, we can use dynamic programming. The idea is to store the results of subproblems to avoid redundant computations.
Here’s a Python implementation of the dynamic programming solution:
def is_interleave(s1, s2, s3):
if len(s1) + len(s2) != len(s3):
return False
dp = {}
def dfs(i, j):
if i == len(s1) and j == len(s2):
return True
if (i, j) in dp:
return dp[(i, j)]
if i < len(s1) and s1[i] == s3[i+j] and dfs(i+1, j):
return True
if j < len(s2) and s2[j] == s3[i+j] and dfs(i, j+1):
return True
dp[(i, j)] = False
return False
return dfs(0, 0)
This solution uses a technique called memoization. Here’s how it works:
- We use a dictionary
dp
to store the results of subproblems. - The
dfs
function now takes only two parameters: i for the current index in s1, and j for the current index in s2. - Before computing a subproblem, we check if we’ve already solved it (if (i, j) is in dp).
- We compute the result for each subproblem only once and store it in dp.
This solution has a time complexity of O(mn) and a space complexity of O(mn), where m and n are the lengths of s1 and s2 respectively. This is a significant improvement over the recursive solution.
3. 2D Dynamic Programming Solution
We can further optimize our solution by using a 2D dynamic programming approach. This method uses a 2D array to store intermediate results, which can lead to better space efficiency in some cases.
Here’s a Python implementation of the 2D dynamic programming solution:
def is_interleave(s1, s2, s3):
if len(s1) + len(s2) != len(s3):
return False
dp = [[False] * (len(s2) + 1) for _ in range(len(s1) + 1)]
dp[0][0] = True
for i in range(1, len(s1) + 1):
dp[i][0] = dp[i-1][0] and s1[i-1] == s3[i-1]
for j in range(1, len(s2) + 1):
dp[0][j] = dp[0][j-1] and s2[j-1] == s3[j-1]
for i in range(1, len(s1) + 1):
for j in range(1, len(s2) + 1):
dp[i][j] = (dp[i-1][j] and s1[i-1] == s3[i+j-1]) or \
(dp[i][j-1] and s2[j-1] == s3[i+j-1])
return dp[len(s1)][len(s2)]
This solution works as follows:
- We create a 2D array
dp
wheredp[i][j]
represents whether the first i characters of s1 and the first j characters of s2 can interleave to form the first i+j characters of s3. - We initialize the base cases: an empty string is always an interleaving of two empty strings.
- We fill the first row and first column of dp based on matches with s1 and s2 respectively.
- We then fill the rest of the dp array. For each cell, we check if we can form a valid interleaving by either:
- Using the current character from s1 if it matches s3, or
- Using the current character from s2 if it matches s3
- The final answer is stored in
dp[len(s1)][len(s2)]
.
This solution also has a time complexity of O(mn) and a space complexity of O(mn), but it can be more efficient in practice due to better cache locality.
Time and Space Complexity Analysis
Let’s compare the time and space complexities of our three solutions:
Solution | Time Complexity | Space Complexity |
---|---|---|
Recursive | O(2^(m+n)) | O(m+n) |
Dynamic Programming (Memoization) | O(mn) | O(mn) |
2D Dynamic Programming | O(mn) | O(mn) |
Where m and n are the lengths of s1 and s2 respectively.
Further Optimization: 1D Dynamic Programming
It’s worth noting that we can further optimize the space complexity to O(min(m,n)) by using a 1D array instead of a 2D array in our dynamic programming solution. This optimization is based on the observation that we only need the previous row to compute the current row in our 2D dp array.
Here’s how we can implement this 1D DP solution:
def is_interleave(s1, s2, s3):
if len(s1) + len(s2) != len(s3):
return False
if len(s2) < len(s1):
return is_interleave(s2, s1, s3)
dp = [False] * (len(s2) + 1)
for i in range(len(s1) + 1):
for j in range(len(s2) + 1):
if i == 0 and j == 0:
dp[j] = True
elif i == 0:
dp[j] = dp[j-1] and s2[j-1] == s3[j-1]
elif j == 0:
dp[j] = dp[j] and s1[i-1] == s3[i-1]
else:
dp[j] = (dp[j] and s1[i-1] == s3[i+j-1]) or \
(dp[j-1] and s2[j-1] == s3[i+j-1])
return dp[len(s2)]
This solution has a time complexity of O(mn) and a space complexity of O(min(m,n)), which is optimal for this problem.
Interview Tips and Tricks
When tackling the Interleaving Strings problem in a coding interview, keep these tips in mind:
- Start with the brute force solution: Begin by explaining the recursive approach. This shows you can think through the problem logically.
- Identify the overlapping subproblems: Point out why the recursive solution is inefficient and how dynamic programming can help.
- Optimize step by step: Move from the recursive solution to the memoized solution, then to the 2D DP solution, and finally to the 1D DP solution if time permits. This demonstrates your ability to continuously improve your solution.
- Analyze time and space complexity: For each solution you propose, be ready to discuss its time and space complexity.
- Consider edge cases: Don’t forget to handle cases where the input strings have different lengths or when one or more strings are empty.
- Code clarity: Write clean, well-commented code. Even if you don’t get to the most optimal solution, clear code is always appreciated.
Related Problems
If you’ve mastered the Interleaving Strings problem, you might want to try your hand at these related problems:
- Edit Distance
- Longest Common Subsequence
- Regular Expression Matching
- Wildcard Matching
These problems also involve string manipulation and can often be solved using dynamic programming techniques.
Conclusion
The Interleaving Strings problem is a classic example of how dynamic programming can dramatically improve the efficiency of a solution. By breaking down the problem into smaller subproblems and storing the results, we can transform an exponential time algorithm into a linear one.
Remember, the key to mastering these types of problems is practice. Try implementing each solution yourself, analyze their performance with different inputs, and don’t hesitate to revisit this problem as you continue your coding interview preparation journey.
At AlgoCademy, we believe in learning by doing. We encourage you to code up these solutions, test them with various inputs, and really understand how they work. This deep understanding is what will set you apart in coding interviews.
Happy coding, and best of luck with your interview preparation!