Mastering the Shortest Palindrome Algorithm: A Comprehensive Guide
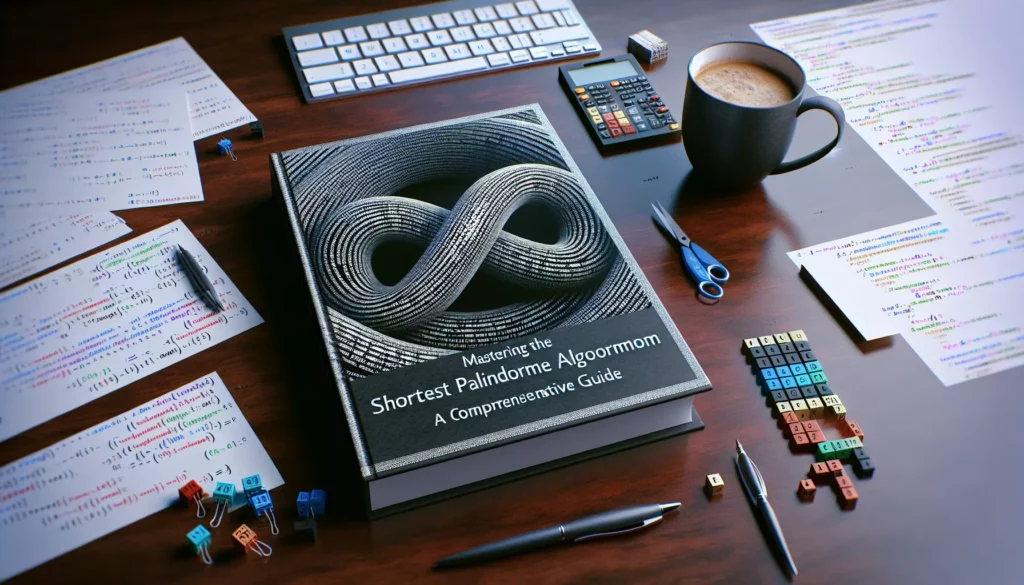
Welcome to another deep dive into the fascinating world of algorithms! Today, we’re going to explore a particularly intriguing problem: the Shortest Palindrome. This algorithm challenge is not only a favorite in coding interviews but also a great exercise to sharpen your string manipulation and pattern recognition skills. Whether you’re preparing for a technical interview at a FAANG company or simply looking to level up your coding abilities, understanding this algorithm will be incredibly valuable.
What is a Palindrome?
Before we dive into the algorithm, let’s refresh our understanding of palindromes. A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward. For example:
- “radar” is a palindrome
- “A man, a plan, a canal: Panama” is a palindrome (ignoring spaces and punctuation)
- “12321” is a numeric palindrome
Palindromes have fascinated mathematicians, linguists, and now programmers for centuries due to their symmetrical nature.
The Shortest Palindrome Problem
The Shortest Palindrome problem asks us to do the following: Given a string s, we need to find the shortest palindrome that can be formed by adding characters in front of s. In other words, we need to add the minimum number of characters at the beginning of the string to make it a palindrome.
For example:
- If s = “aacecaaa”, the shortest palindrome would be “aaacecaaa”
- If s = “abcd”, the shortest palindrome would be “dcbabcd”
This problem might seem simple at first glance, but it requires a clever approach to solve efficiently, especially for very long strings.
Naive Approach
Before we dive into the optimal solution, let’s consider a naive approach to understand the problem better. One straightforward way to solve this would be:
- Start with the entire string.
- Check if it’s a palindrome. If yes, return the string as is.
- If not, remove the last character and prepend it to a new string.
- Repeat steps 2-3 until we find a palindrome.
Here’s how this might look in Python:
def shortest_palindrome_naive(s):
if not s:
return s
rev = s[::-1]
for i in range(len(s)):
if s.startswith(rev[i:]):
return rev[:i] + s
return rev + s
While this approach works, it has a time complexity of O(n^2) in the worst case, where n is the length of the string. This is because for each character we remove (up to n times), we’re doing a string comparison that can take up to n time.
Optimal Solution: KMP Algorithm
The efficient solution to the Shortest Palindrome problem involves using the Knuth-Morris-Pratt (KMP) algorithm. The KMP algorithm is typically used for pattern matching in strings, but we can adapt it for our palindrome problem.
The key insight is this: the shortest palindrome will be formed by finding the longest palindrome prefix of the string and then adding the reverse of the remaining suffix to the beginning of the string.
Here’s how we can use KMP to solve this problem:
- Create a new string temp by concatenating the original string s, a special character (like ‘#’ that doesn’t appear in s), and the reverse of s.
- Compute the KMP failure function for this new string.
- The last value in the failure function array will give us the length of the longest proper prefix of s that is also a suffix of the reversed s.
- Use this information to construct the shortest palindrome.
Let’s implement this step by step:
def shortest_palindrome(s):
if not s:
return s
temp = s + '#' + s[::-1]
failure = [0] * len(temp)
# Compute the KMP failure function
for i in range(1, len(temp)):
j = failure[i - 1]
while j > 0 and temp[i] != temp[j]:
j = failure[j - 1]
failure[i] = j + (temp[i] == temp[j])
# The last entry of failure array gives us the length of the longest palindrome prefix
return s[failure[-1]:][::-1] + s
This solution has a time complexity of O(n), where n is the length of the string, making it much more efficient than the naive approach.
Understanding the KMP Algorithm
The Knuth-Morris-Pratt (KMP) algorithm is a string matching algorithm that uses the failure function (also known as the prefix function) to achieve linear time complexity. In our case, we’re using it to find the longest proper prefix of s that is also a suffix of the reversed s.
The failure function f(i) for a string is defined as the length of the longest proper prefix of the substring s[0:i+1] which is also a suffix of this substring. For example, for the string “ABABC”:
- f(0) = 0 (for “A”)
- f(1) = 0 (for “AB”)
- f(2) = 1 (for “ABA”)
- f(3) = 2 (for “ABAB”)
- f(4) = 0 (for “ABABC”)
In our shortest palindrome solution, we’re applying this concept to the string s + ‘#’ + reverse(s). The last value in the failure function for this string tells us how much of the original string is already a palindrome prefix.
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our optimal solution:
Time Complexity
The time complexity of this algorithm is O(n), where n is the length of the input string. Here’s why:
- Creating the temp string takes O(n) time.
- Computing the KMP failure function involves a single pass through the temp string, which is O(2n + 1) = O(n).
- The final string concatenation is also O(n).
Therefore, the overall time complexity is O(n).
Space Complexity
The space complexity is also O(n):
- We create a new string temp of length 2n + 1.
- We create a failure array of length 2n + 1.
Both of these are O(n) in terms of space complexity.
Common Pitfalls and Edge Cases
When implementing the Shortest Palindrome algorithm, be aware of these common pitfalls and edge cases:
- Empty String: Always handle the case of an empty input string. Our implementation returns an empty string in this case.
- Single Character: A string with a single character is already a palindrome, so it should be returned as is.
- All Identical Characters: A string like “aaaa” is already a palindrome and should be returned as is.
- No Palindrome Prefix: In cases like “abcd”, where there’s no palindrome prefix except the first character, the algorithm should correctly add the entire reversed string minus the first character.
Practical Applications
While the Shortest Palindrome problem might seem like a purely academic exercise, understanding and implementing this algorithm can have practical benefits:
- String Processing: This algorithm showcases efficient string manipulation techniques that can be applied to various text processing tasks.
- Pattern Matching: The use of the KMP algorithm in this solution demonstrates its versatility beyond simple string matching, which is valuable in many data processing scenarios.
- Algorithmic Thinking: Solving this problem requires creative thinking about string properties and efficient algorithm design, skills that are crucial in many areas of software development.
- Interview Preparation: This problem is a favorite in technical interviews, especially for positions that require strong algorithmic skills.
Related Problems and Variations
If you’ve mastered the Shortest Palindrome problem, you might want to try your hand at these related problems:
- Longest Palindromic Substring: Find the longest substring of a given string that is a palindrome.
- Palindrome Partitioning: Partition a string into palindromic substrings.
- Manacher’s Algorithm: An O(n) algorithm to find all palindromic substrings in a string.
- Palindrome Pairs: Given a list of words, find all pairs of distinct indices (i, j) such that the concatenation of the two words words[i] + words[j] is a palindrome.
Conclusion
The Shortest Palindrome problem is a fascinating algorithm challenge that combines string manipulation, pattern matching, and creative problem-solving. By understanding and implementing this algorithm, you’ve not only prepared yourself for potential interview questions but also honed your skills in efficient algorithm design and string processing.
Remember, the key to mastering algorithms like this is practice. Try implementing this solution in different programming languages, test it with various inputs, and explore how you can optimize it further. Happy coding!