Maximum Area of Island: Mastering Graph Traversal in Coding Interviews
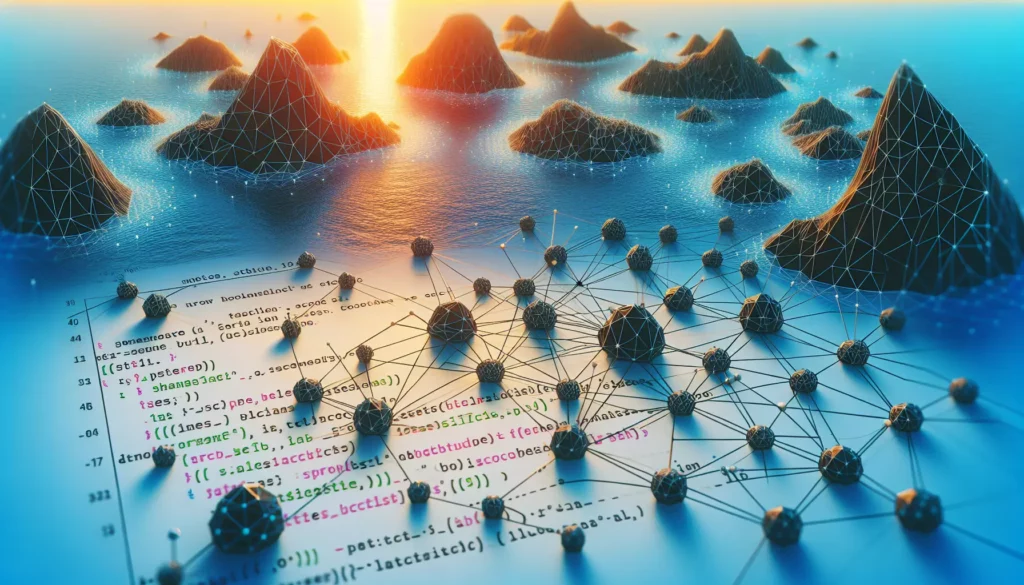
Welcome to another exciting deep dive into algorithmic problem-solving! Today, we’re tackling a classic graph traversal problem that often appears in coding interviews, especially those for FAANG (Facebook, Amazon, Apple, Netflix, Google) companies. Our focus will be on the “Maximum Area of Island” problem, a challenge that tests your understanding of depth-first search (DFS) and your ability to navigate 2D grids efficiently.
Understanding the Problem
Before we dive into the solution, let’s break down the problem statement:
Given a 2D binary matrix filled with 0’s and 1’s, find the largest island in the matrix. An island is a group of 1’s (representing land) connected horizontally or vertically (not diagonally). The matrix is surrounded by water (0’s).
This problem is an excellent test of your graph traversal skills, as it requires you to:
- Identify islands in the matrix
- Calculate the area of each island
- Keep track of the maximum area encountered
Approaching the Solution
To solve this problem, we’ll use a depth-first search (DFS) approach. Here’s a high-level overview of our strategy:
- Iterate through each cell in the matrix
- When we find a land cell (1), start a DFS from that cell
- During the DFS, mark visited cells and count the area of the island
- Update the maximum area if the current island is larger
- Return the maximum area found
Implementing the Solution
Let’s implement this solution step by step in Python:
class Solution:
def maxAreaOfIsland(self, grid: List[List[int]]) -> int:
if not grid:
return 0
max_area = 0
rows, cols = len(grid), len(grid[0])
def dfs(i, j):
if i < 0 or i >= rows or j < 0 or j >= cols or grid[i][j] == 0:
return 0
grid[i][j] = 0 # Mark as visited
return 1 + dfs(i+1, j) + dfs(i-1, j) + dfs(i, j+1) + dfs(i, j-1)
for i in range(rows):
for j in range(cols):
if grid[i][j] == 1:
max_area = max(max_area, dfs(i, j))
return max_area
Now, let’s break down this solution and explain each part in detail:
1. Class and Method Definition
class Solution:
def maxAreaOfIsland(self, grid: List[List[int]]) -> int:
We define a class Solution
with a method maxAreaOfIsland
that takes a 2D list (our grid) as input and returns an integer (the maximum area).
2. Edge Case Handling
if not grid:
return 0
We first check if the grid is empty. If it is, we return 0 as there can’t be any islands in an empty grid.
3. Initializing Variables
max_area = 0
rows, cols = len(grid), len(grid[0])
We initialize max_area
to keep track of the largest island we’ve found. We also store the number of rows and columns in the grid for easy access later.
4. Defining the DFS Function
def dfs(i, j):
if i < 0 or i >= rows or j < 0 or j >= cols or grid[i][j] == 0:
return 0
grid[i][j] = 0 # Mark as visited
return 1 + dfs(i+1, j) + dfs(i-1, j) + dfs(i, j+1) + dfs(i, j-1)
This is the heart of our solution. The dfs
function does the following:
- Checks if the current cell is out of bounds or is water (0). If so, it returns 0.
- Marks the current cell as visited by setting it to 0.
- Recursively explores the four adjacent cells (up, down, left, right) and sums their areas.
- Returns the total area of the island (including the current cell).
5. Iterating Through the Grid
for i in range(rows):
for j in range(cols):
if grid[i][j] == 1:
max_area = max(max_area, dfs(i, j))
We iterate through each cell in the grid. When we find a land cell (1), we start a DFS from that cell and update max_area
if necessary.
6. Returning the Result
return max_area
Finally, we return the maximum area we’ve found.
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our solution:
Time Complexity: O(m * n)
Where m is the number of rows and n is the number of columns in the grid.
- We iterate through each cell in the grid once: O(m * n)
- For each land cell, we perform a DFS, but each cell is visited at most once (since we mark it as visited): O(m * n)
Therefore, the total time complexity is O(m * n).
Space Complexity: O(m * n)
In the worst case, if the entire grid is one large island, the recursion stack will go as deep as the number of cells in the grid. Therefore, the space complexity is O(m * n).
Common Pitfalls and Optimization
When solving this problem, there are a few common pitfalls to watch out for:
1. Forgetting to Mark Visited Cells
If you forget to mark visited cells (by setting them to 0 in our solution), you’ll end up in an infinite loop. Always ensure you’re marking cells as visited to avoid revisiting them.
2. Incorrect Boundary Checks
Make sure your boundary checks are correct. It’s easy to mix up the conditions or forget to check all four boundaries.
3. Not Considering Empty Grids
Always handle the edge case of an empty grid at the beginning of your function.
Optimization: Avoiding Recursion
While our recursive DFS solution is clean and intuitive, it could potentially lead to a stack overflow for very large grids. An iterative approach using a stack can solve this issue:
class Solution:
def maxAreaOfIsland(self, grid: List[List[int]]) -> int:
if not grid:
return 0
max_area = 0
rows, cols = len(grid), len(grid[0])
for i in range(rows):
for j in range(cols):
if grid[i][j] == 1:
area = 0
stack = [(i, j)]
while stack:
r, c = stack.pop()
if 0 <= r < rows and 0 <= c < cols and grid[r][c] == 1:
area += 1
grid[r][c] = 0
stack.extend([(r+1, c), (r-1, c), (r, c+1), (r, c-1)])
max_area = max(max_area, area)
return max_area
This iterative approach has the same time and space complexity as the recursive one, but it avoids the potential for stack overflow in extreme cases.
Related Problems and Variations
The “Maximum Area of Island” problem is part of a family of graph traversal problems often seen in coding interviews. Here are some related problems you might encounter:
1. Number of Islands
Instead of finding the largest island, count the total number of islands in the grid.
2. Pacific Atlantic Water Flow
Given an m x n matrix of non-negative integers representing the height of each unit cell in a continent, determine which cells can flow to both the Pacific and Atlantic oceans.
3. Surrounded Regions
Given a 2D board containing ‘X’ and ‘O’, capture all regions surrounded by ‘X’. A region is captured by flipping all ‘O’s into ‘X’s in that surrounded region.
4. Word Search
Given a 2D board and a word, find if the word exists in the grid. The word can be constructed from letters of sequentially adjacent cells, where adjacent cells are horizontally or vertically neighboring.
Practical Applications
Understanding and solving problems like “Maximum Area of Island” isn’t just about acing coding interviews. These concepts have real-world applications in various fields:
1. Image Processing
Similar algorithms are used in image processing to identify and measure objects or features in images. For example, measuring the size of tumors in medical imaging or identifying landmasses in satellite imagery.
2. Game Development
In game development, these algorithms can be used for map generation, pathfinding, or identifying connected game elements.
3. Network Analysis
In network analysis, similar techniques can be used to identify clusters or communities within social networks or other graph-based data structures.
4. Geographic Information Systems (GIS)
GIS applications often use these types of algorithms for analyzing geographical data, such as identifying contiguous land areas or water bodies.
Conclusion
The “Maximum Area of Island” problem is an excellent example of how graph traversal algorithms can be applied to solve complex problems efficiently. By mastering this problem, you’re not only preparing yourself for coding interviews but also developing skills that are applicable in various real-world scenarios.
Remember, the key to solving these types of problems is to:
- Understand the problem thoroughly
- Identify the appropriate algorithm (in this case, DFS)
- Implement the solution carefully, paying attention to edge cases
- Analyze and optimize your solution
As you continue your journey in algorithmic problem-solving, keep practicing similar problems to reinforce your understanding of graph traversal techniques. Happy coding!