Largest Rectangle in Histogram: A Comprehensive Guide
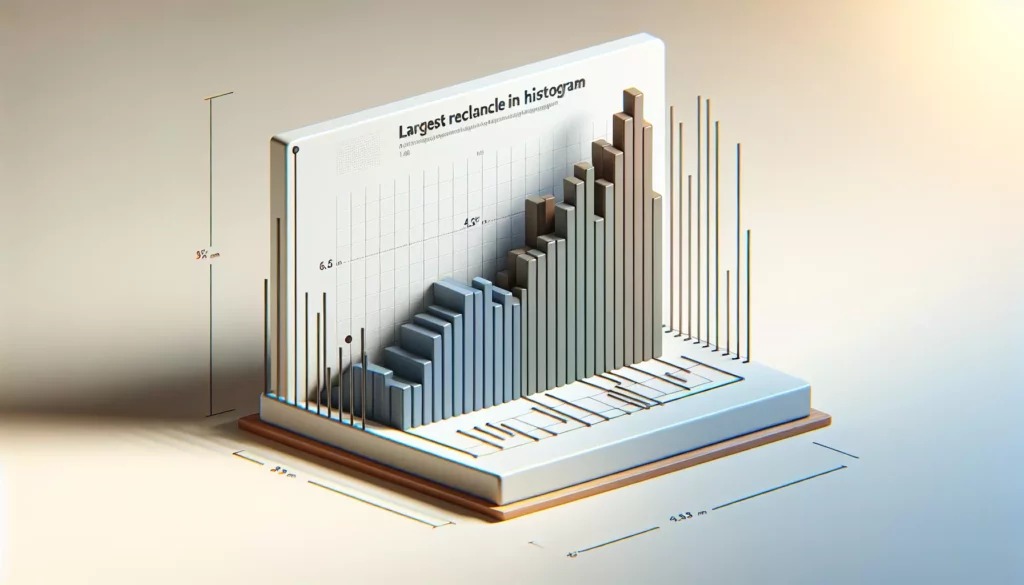
Welcome to AlgoCademy’s deep dive into one of the most intriguing and challenging algorithmic problems: finding the largest rectangle in a histogram. This problem is not just a favorite among interviewers at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), but it’s also a fantastic exercise to sharpen your problem-solving skills and algorithmic thinking.
Understanding the Problem
Before we jump into the solution, let’s clearly define the problem:
Given an array of integers heights
where heights[i]
represents the height of the i-th bar in a histogram, we need to find the area of the largest rectangle that can be formed using these bars.
For example, consider the following histogram:
heights = [2,1,5,6,2,3]
The largest rectangle in this histogram has an area of 10 units (formed by the 5 and 6 height bars).
Naive Approach
Let’s start with a simple, brute-force approach to understand the problem better:
def largestRectangleArea(heights):
max_area = 0
n = len(heights)
for i in range(n):
min_height = float('inf')
for j in range(i, n):
min_height = min(min_height, heights[j])
area = min_height * (j - i + 1)
max_area = max(max_area, area)
return max_area
This approach works by considering every possible pair of bars and calculating the area of the rectangle formed between them. The height of this rectangle is determined by the shortest bar in this range.
While this solution is correct, it has a time complexity of O(n^2), which is not efficient for large inputs. In coding interviews, especially at top tech companies, you’ll often be asked to optimize such solutions.
Optimized Approach: Using a Stack
Now, let’s look at an optimized solution that uses a stack to solve this problem in O(n) time:
def largestRectangleArea(heights):
stack = []
max_area = 0
heights.append(0) # Add a 0 at the end to process all remaining bars
for i, h in enumerate(heights):
start = i
while stack and stack[-1][1] > h:
index, height = stack.pop()
max_area = max(max_area, height * (i - index))
start = index
stack.append((start, h))
return max_area
This solution might look complex at first glance, but let’s break it down step by step:
- We use a stack to keep track of the indices of the bars and their heights.
- We iterate through each bar in the histogram.
- For each bar, we pop bars from the stack that are taller than the current bar.
- When we pop a bar, we calculate the area of the rectangle that ends at the current position and starts at the position of the popped bar.
- We keep track of the maximum area seen so far.
- We push the current bar onto the stack.
The key insight here is that each bar is pushed and popped at most once, giving us a time complexity of O(n).
Understanding the Stack-based Approach
To truly grasp this solution, let’s walk through an example. Consider the histogram:
heights = [2,1,5,6,2,3]
Here’s how the algorithm progresses:
- Push (0, 2) onto the stack.
- For 1: Pop (0, 2), calculate area = 2 * 1 = 2, push (0, 1).
- For 5: Push (2, 5).
- For 6: Push (3, 6).
- For 2: Pop (3, 6), area = 6 * 1 = 6, pop (2, 5), area = 5 * 2 = 10, push (2, 2).
- For 3: Push (5, 3).
- For 0 (added at the end): Pop all remaining elements, calculating areas.
The largest area found is 10, which is indeed the correct answer.
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our optimized solution:
- Time Complexity: O(n), where n is the number of bars in the histogram. Each bar is pushed and popped at most once.
- Space Complexity: O(n) in the worst case, where the stack stores all the bars (e.g., for a strictly increasing histogram).
This analysis is crucial for coding interviews, especially at FAANG companies, where understanding the efficiency of your algorithms is as important as getting the correct solution.
Common Variations and Follow-up Questions
In a real interview scenario, especially at top tech companies, you might encounter variations of this problem or follow-up questions. Here are a few to consider:
1. Maximum Rectangle in Binary Matrix
This is a 2D version of our problem. Given a binary matrix filled with 0s and 1s, find the largest rectangle containing only 1s and return its area.
The approach here is to treat each row as a histogram and apply our solution. Here’s a Python implementation:
def maximalRectangle(matrix):
if not matrix or not matrix[0]:
return 0
n = len(matrix[0])
heights = [0] * (n + 1)
max_area = 0
for row in matrix:
for i in range(n):
heights[i] = heights[i] + 1 if row[i] == '1' else 0
stack = [-1]
for i in range(n + 1):
while heights[i] < heights[stack[-1]]:
h = heights[stack.pop()]
w = i - stack[-1] - 1
max_area = max(max_area, h * w)
stack.append(i)
return max_area
2. Skyline Problem
This problem asks you to draw the skyline of a city given the locations and heights of the buildings. It’s a more complex variation that also uses the concept of finding maximum heights efficiently.
3. Optimizing for Space
Can you solve the original problem using O(1) extra space? This is a challenging variation that requires a different approach, often using the concept of “two pointers”.
Tips for Solving Similar Problems in Interviews
When facing problems like the “Largest Rectangle in Histogram” in coding interviews, especially at FAANG companies, keep these tips in mind:
- Understand the problem thoroughly: Make sure you grasp all aspects of the problem before diving into the solution.
- Start with a brute-force approach: Even if it’s not efficient, it helps to have a working solution and shows your problem-solving process.
- Look for patterns and optimizations: Try to identify what makes the brute-force approach inefficient and how you can improve it.
- Consider using data structures: Stacks, queues, heaps, and other data structures often lead to more efficient solutions.
- Analyze time and space complexity: Be prepared to discuss the efficiency of your solution in detail.
- Test your solution: Use various test cases, including edge cases, to verify your solution.
- Communicate your thought process: Explain your approach, decisions, and any trade-offs you’re making.
Conclusion
The “Largest Rectangle in Histogram” problem is a classic example of how a seemingly straightforward question can have a non-obvious, elegant solution. By using a stack, we transformed a quadratic-time algorithm into a linear-time one, demonstrating the power of choosing the right data structure and algorithm.
This problem showcases several important concepts in computer science and coding interviews:
- The importance of optimizing brute-force solutions
- Effective use of stack data structure
- Handling edge cases (like adding a 0 at the end of the histogram)
- The technique of maintaining a monotonic stack
Mastering problems like this one is crucial for success in technical interviews at top tech companies. It’s not just about finding the correct answer, but about demonstrating your problem-solving skills, your ability to optimize solutions, and your understanding of fundamental computer science concepts.
At AlgoCademy, we believe that tackling such problems is key to developing strong algorithmic thinking and coding skills. Our platform provides numerous similar challenges, complete with step-by-step guidance and AI-powered assistance, to help you prepare for technical interviews and improve your coding abilities.
Remember, the journey to becoming a proficient programmer and acing those FAANG interviews is a marathon, not a sprint. Keep practicing, stay curious, and always look for ways to optimize and improve your solutions. Happy coding!