Articulation Points in Graphs: Understanding Critical Nodes in Network Structures
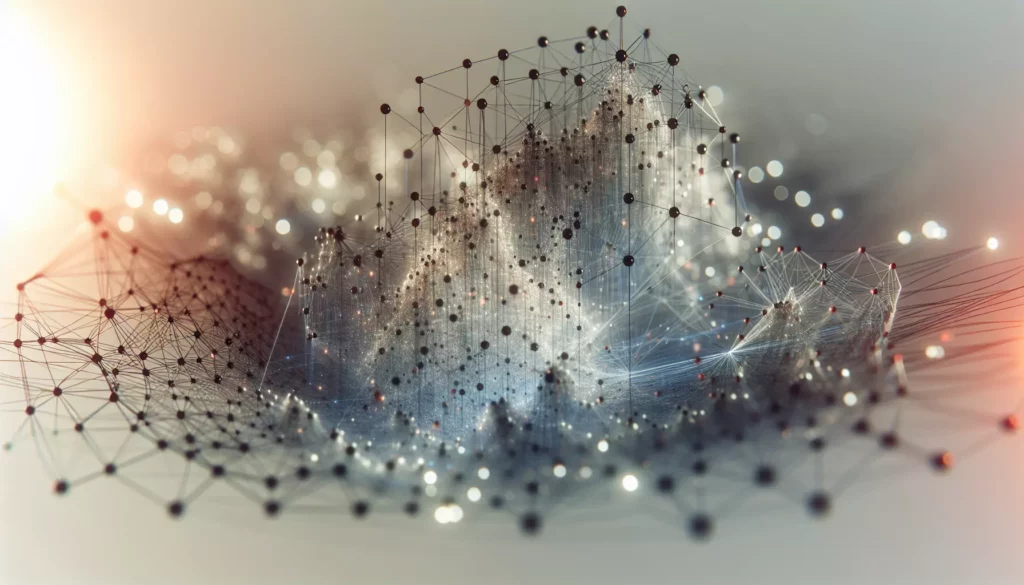
In the world of computer science and graph theory, understanding the structure and properties of graphs is crucial for solving complex problems efficiently. One important concept in graph theory is that of articulation points, also known as cut vertices. These are nodes in a graph whose removal increases the number of connected components in the graph. In this comprehensive guide, we’ll explore articulation points in depth, discussing their properties, applications, and how to find them algorithmically.
What are Articulation Points?
An articulation point, or cut vertex, is a vertex in a graph whose removal (along with its incident edges) increases the number of connected components in the graph. In other words, it’s a node that, if removed, would disconnect the graph or increase its number of disconnected subgraphs.
To understand this concept better, let’s consider a simple example:
A
/ \
B C
/ \ \
D E F
\
G
In this graph, vertices A and E are articulation points. If we remove A, the graph splits into two disconnected components: {B, D, E, G} and {C, F}. Similarly, if we remove E, the graph splits into {A, B, C, D, F} and {G}.
Properties of Articulation Points
Articulation points have several important properties:
- Connectivity Impact: Removing an articulation point increases the number of connected components in the graph.
- Bridge Relationship: Every endpoint of a bridge (an edge whose removal disconnects the graph) is an articulation point, unless it’s a leaf node.
- Tree Structure: In a tree, every non-leaf node is an articulation point.
- Biconnected Components: Articulation points are the vertices that belong to more than one biconnected component in a graph.
- Cycle Consideration: A vertex in a cycle is not necessarily an articulation point, unless it connects the cycle to other parts of the graph.
Applications of Articulation Points
Understanding and identifying articulation points has numerous practical applications in various fields:
1. Network Reliability
In computer networks, articulation points represent critical nodes whose failure could disconnect parts of the network. Identifying these points helps in designing more robust network architectures and implementing redundancy measures.
2. Social Network Analysis
In social networks, articulation points can represent key individuals who bridge different communities. Removing these individuals would fragment the social structure.
3. Transportation Systems
In transportation networks, articulation points might represent crucial junctions or stations. Their identification can help in planning alternative routes and improving system resilience.
4. Biological Networks
In biological networks, such as protein interaction networks, articulation points might represent proteins that play critical roles in maintaining the overall structure of the network.
5. Supply Chain Management
In supply chain networks, articulation points could represent critical suppliers or distribution centers whose disruption would significantly impact the entire supply chain.
Finding Articulation Points: The Algorithm
Now that we understand the importance of articulation points, let’s dive into how we can find them algorithmically. We’ll use a depth-first search (DFS) based approach, often referred to as Tarjan’s algorithm for finding articulation points.
The Intuition
The key idea behind the algorithm is to use DFS to traverse the graph and keep track of two important pieces of information for each node:
- Discovery Time: The time at which a node is first discovered during the DFS traversal.
- Low Value: The lowest discovery time of any node that can be reached from the subtree rooted at the current node, including the current node itself.
A node u is an articulation point if either:
- u is the root of the DFS tree and has at least two children.
- u is not the root, and it has a child v such that no vertex in the subtree rooted at v has a back edge to one of u’s ancestors.
The Algorithm in Detail
Here’s a step-by-step breakdown of the algorithm:
- Perform a DFS traversal of the graph.
- For each node, keep track of its discovery time and low value.
- For the root node, count its children in the DFS tree. If it has more than one child, it’s an articulation point.
- For non-root nodes, if any child has a low value greater than or equal to the discovery time of the current node, then the current node is an articulation point.
- Update the low value of the current node based on its children and back edges.
Pseudocode
Here’s the pseudocode for finding articulation points:
function findArticulationPoints(graph):
n = number of nodes in graph
discovered = [false] * n
low = [0] * n
parent = [-1] * n
articulation_points = [false] * n
time = 0
function dfs(u):
nonlocal time
children = 0
discovered[u] = true
low[u] = time
disc[u] = time
time += 1
for v in graph[u]:
if not discovered[v]:
children += 1
parent[v] = u
dfs(v)
low[u] = min(low[u], low[v])
if parent[u] == -1 and children > 1:
articulation_points[u] = true
if parent[u] != -1 and low[v] >= disc[u]:
articulation_points[u] = true
elif v != parent[u]:
low[u] = min(low[u], disc[v])
for i in range(n):
if not discovered[i]:
dfs(i)
return articulation_points
Time and Space Complexity
The time complexity of this algorithm is O(V + E), where V is the number of vertices and E is the number of edges in the graph. This is because we perform a single DFS traversal of the graph.
The space complexity is O(V), as we need to store the discovery time, low value, parent information, and articulation point status for each vertex.
Implementation in Python
Let’s implement the algorithm to find articulation points in Python:
from collections import defaultdict
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = defaultdict(list)
self.time = 0
def add_edge(self, u, v):
self.graph[u].append(v)
self.graph[v].append(u)
def find_articulation_points(self):
discovered = [False] * self.V
low = [0] * self.V
parent = [-1] * self.V
articulation_points = [False] * self.V
disc = [float("inf")] * self.V
def dfs(u):
children = 0
discovered[u] = True
disc[u] = self.time
low[u] = self.time
self.time += 1
for v in self.graph[u]:
if not discovered[v]:
children += 1
parent[v] = u
dfs(v)
low[u] = min(low[u], low[v])
if parent[u] == -1 and children > 1:
articulation_points[u] = True
if parent[u] != -1 and low[v] >= disc[u]:
articulation_points[u] = True
elif v != parent[u]:
low[u] = min(low[u], disc[v])
for i in range(self.V):
if not discovered[i]:
dfs(i)
return [i for i in range(self.V) if articulation_points[i]]
# Example usage
g = Graph(5)
g.add_edge(1, 0)
g.add_edge(0, 2)
g.add_edge(2, 1)
g.add_edge(0, 3)
g.add_edge(3, 4)
articulation_points = g.find_articulation_points()
print("Articulation points in the graph:", articulation_points)
This implementation creates a Graph class with methods to add edges and find articulation points. The find_articulation_points method implements the algorithm we discussed earlier.
Common Pitfalls and Edge Cases
When working with articulation points, it’s important to be aware of some common pitfalls and edge cases:
1. Single Node Graphs
A graph with a single node has no articulation points, as removing the node would leave an empty graph, not increase the number of connected components.
2. Complete Graphs
In a complete graph (where every node is connected to every other node), there are no articulation points. Removing any single node would not disconnect the graph.
3. Cycle Graphs
In a simple cycle graph, no node is an articulation point. Removing any node would turn the cycle into a path, which is still a single connected component.
4. Trees
In a tree, every non-leaf node is an articulation point. This is because removing any non-leaf node would disconnect its subtree from the rest of the tree.
5. Disconnected Graphs
When finding articulation points in a disconnected graph, make sure your algorithm handles multiple connected components correctly.
Advanced Topics Related to Articulation Points
As you delve deeper into graph theory and network analysis, you’ll encounter several advanced topics related to articulation points:
1. Biconnected Components
A biconnected component is a maximal biconnected subgraph. Articulation points are the vertices that belong to more than one biconnected component. Understanding biconnected components can help in analyzing the structure of complex networks.
2. Bridge Edges
Bridge edges, also known as cut edges, are closely related to articulation points. A bridge is an edge whose removal would disconnect the graph. Every bridge has at least one endpoint that is an articulation point (unless it connects two leaf nodes).
3. K-Vertex-Connected Graphs
A graph is k-vertex-connected if it remains connected after removing any k-1 vertices. Articulation points are particularly relevant in 1-vertex-connected graphs (also known as connected graphs that are not 2-vertex-connected).
4. Graph Decomposition
Articulation points play a crucial role in various graph decomposition techniques, such as decomposing a graph into its biconnected components or finding block-cut trees.
5. Dynamic Graph Algorithms
In dynamic graphs, where the structure of the graph changes over time, maintaining information about articulation points efficiently becomes a challenging problem. Researchers have developed algorithms for incrementally updating articulation point information as edges are added or removed from the graph.
Practical Tips for Working with Articulation Points
When dealing with articulation points in real-world scenarios, consider the following tips:
- Visualization: Use graph visualization tools to help understand the structure of your graph and verify the correctness of your articulation point findings.
- Testing: Create a comprehensive set of test cases, including edge cases like single-node graphs, complete graphs, and disconnected graphs.
- Optimization: For very large graphs, consider parallelizing the articulation point finding algorithm or using more advanced data structures to improve performance.
- Context Matters: Remember that the importance of an articulation point depends on the specific application. In some contexts, certain articulation points may be more critical than others.
- Combine with Other Metrics: When analyzing networks, consider combining articulation point analysis with other network metrics like centrality measures for a more comprehensive understanding.
Conclusion
Articulation points are a fundamental concept in graph theory with wide-ranging applications in network analysis, computer science, and beyond. Understanding how to identify and work with articulation points can provide valuable insights into the structure and vulnerabilities of complex networks.
As you continue your journey in algorithm design and graph theory, remember that articulation points are just one piece of the puzzle. They often work in conjunction with other graph properties and algorithms to solve complex real-world problems.
Whether you’re designing resilient computer networks, analyzing social structures, or optimizing transportation systems, the ability to identify and understand articulation points will be a valuable tool in your problem-solving toolkit.
Keep practicing with different graph structures, implement the algorithms yourself, and always be on the lookout for real-world applications of these fascinating graph properties. Happy coding!