Longest Path in a DAG: Mastering Graph Algorithms for Technical Interviews
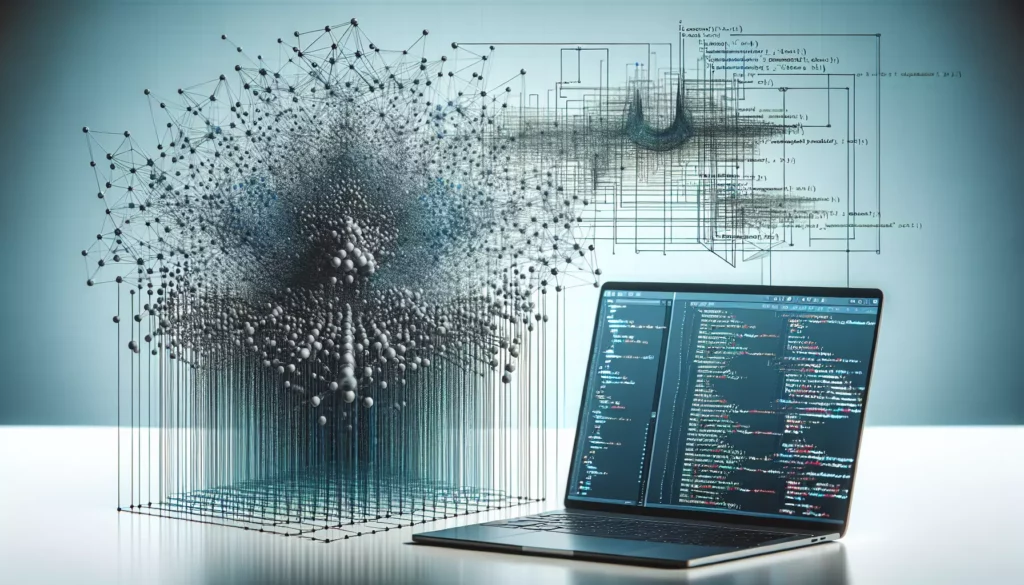
Welcome to another deep dive into the world of algorithms and data structures! Today, we’re going to explore an intriguing problem that often appears in technical interviews, especially for positions at top tech companies like Google, Amazon, or Facebook. Our topic of focus is finding the longest path in a Directed Acyclic Graph (DAG).
This problem is not only a favorite among interviewers but also serves as a fundamental concept in various real-world applications, from project scheduling to dependency resolution in build systems. By the end of this article, you’ll have a solid understanding of the problem, its solution, and how to implement it efficiently.
Table of Contents
- Understanding the Problem
- What is a Directed Acyclic Graph (DAG)?
- The Longest Path Problem
- Approach to Solving the Problem
- Topological Sorting
- Dynamic Programming Solution
- Implementation in Python
- Time and Space Complexity Analysis
- Real-world Applications
- Common Interview Questions and Variations
- Conclusion
1. Understanding the Problem
Before we dive into the solution, let’s make sure we have a clear understanding of what the problem is asking. The longest path in a DAG is defined as the path with the maximum number of edges (or maximum total weight if the edges are weighted) from any starting vertex to any ending vertex in the graph.
This problem might seem similar to finding the shortest path, which is more commonly discussed. However, the longest path problem has some unique characteristics that make it particularly interesting in the context of DAGs.
2. What is a Directed Acyclic Graph (DAG)?
A Directed Acyclic Graph (DAG) is a graph that has the following properties:
- Directed: Each edge has a direction, pointing from one vertex to another.
- Acyclic: There are no cycles in the graph. In other words, if you start from any vertex and follow the directed edges, you can never return to the same vertex.
DAGs have many interesting properties and are used to model various real-world scenarios, such as task dependencies, version control systems, and more.
3. The Longest Path Problem
Now that we understand what a DAG is, let’s formally define the longest path problem:
Given a Directed Acyclic Graph, find the longest path from any starting vertex to any ending vertex in the graph. The length of a path is defined as the number of edges in the path (or the sum of edge weights if the edges are weighted).
It’s worth noting that while finding the shortest path in a graph with cycles can be done efficiently (e.g., using Dijkstra’s algorithm), finding the longest path in a graph with cycles is NP-hard. However, the acyclic nature of DAGs allows us to solve the longest path problem efficiently.
4. Approach to Solving the Problem
The key to solving the longest path problem in a DAG lies in leveraging two important concepts:
- Topological Sorting: This allows us to process the vertices in an order that respects the direction of the edges.
- Dynamic Programming: This helps us build the solution incrementally, reusing previously computed results.
Let’s explore each of these concepts in detail.
5. Topological Sorting
Topological sorting is an ordering of the vertices in a DAG such that for every directed edge (u, v), vertex u comes before vertex v in the ordering. This sorting is possible only for DAGs and is not unique – there can be multiple valid topological orderings for a given DAG.
Here’s a simple algorithm to perform topological sorting:
- Choose a vertex with no incoming edges.
- Add it to the result list and remove it from the graph.
- Repeat steps 1 and 2 until all vertices are processed.
Here’s a Python implementation of topological sorting using Depth-First Search (DFS):
from collections import defaultdict
def topological_sort(graph):
def dfs(v):
visited.add(v)
for neighbor in graph[v]:
if neighbor not in visited:
dfs(neighbor)
stack.append(v)
visited = set()
stack = []
for vertex in graph:
if vertex not in visited:
dfs(vertex)
return stack[::-1] # Reverse the stack to get topological order
# Example usage
graph = defaultdict(list)
graph[0] = [1, 2]
graph[1] = [3]
graph[2] = [3]
graph[3] = [4, 5]
graph[4] = []
graph[5] = []
print(topological_sort(graph)) # Output: [0, 2, 1, 3, 5, 4]
This topological sorting will be crucial in our solution to the longest path problem.
6. Dynamic Programming Solution
Now that we have a way to order the vertices, let’s discuss how we can use dynamic programming to find the longest path. The idea is to process the vertices in topological order and maintain the longest path ending at each vertex.
Here’s the step-by-step approach:
- Perform a topological sort of the graph.
- Initialize an array
dist
to store the longest path lengths. Setdist[v] = 0
for all vertices initially. - For each vertex
u
in the topological order:- For each neighbor
v
ofu
:- Update
dist[v] = max(dist[v], dist[u] + weight(u, v))
- Update
- For each neighbor
- The maximum value in
dist
array is the length of the longest path.
This approach works because when we process a vertex, we’ve already processed all its predecessors (thanks to topological sorting), so we have the correct longest path lengths for them.
7. Implementation in Python
Let’s implement this solution in Python:
from collections import defaultdict
def longest_path_in_dag(graph, weights):
def dfs(v):
visited.add(v)
for neighbor in graph[v]:
if neighbor not in visited:
dfs(neighbor)
stack.append(v)
# Topological Sort
visited = set()
stack = []
for vertex in graph:
if vertex not in visited:
dfs(vertex)
# Initialize distances
dist = {v: float('-inf') for v in graph}
for v in graph:
dist[v] = 0
# Process vertices in topological order
while stack:
u = stack.pop()
for v in graph[u]:
if dist[v] < dist[u] + weights[(u, v)]:
dist[v] = dist[u] + weights[(u, v)]
# Find the maximum distance and the corresponding vertex
max_dist = max(dist.values())
max_vertex = max(dist, key=dist.get)
return max_dist, max_vertex
# Example usage
graph = defaultdict(list)
graph[0] = [1, 2]
graph[1] = [3]
graph[2] = [3]
graph[3] = [4, 5]
graph[4] = []
graph[5] = []
weights = {(0, 1): 5, (0, 2): 3, (1, 3): 6, (2, 3): 4, (3, 4): 2, (3, 5): 7}
longest_path, end_vertex = longest_path_in_dag(graph, weights)
print(f"The longest path has length {longest_path} and ends at vertex {end_vertex}")
This implementation not only finds the length of the longest path but also identifies the ending vertex of this path.
8. Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our solution:
- Time Complexity: O(V + E), where V is the number of vertices and E is the number of edges in the graph. We perform a topological sort (O(V + E)) and then process each edge once (O(E)).
- Space Complexity: O(V), where V is the number of vertices. We use additional space for the visited set, stack, and dist dictionary, each of which can contain at most V elements.
This efficient solution makes the longest path in DAG problem tractable for large graphs, unlike the general longest path problem which is NP-hard for graphs with cycles.
9. Real-world Applications
The longest path in DAG problem has several practical applications:
- Project Scheduling: In project management, tasks can be represented as vertices in a DAG, with edges representing dependencies. The longest path in this DAG is called the critical path, which determines the minimum time needed to complete the project.
- Build Systems: In software build systems, the DAG represents dependencies between different modules or files. The longest path can help in optimizing the build process.
- Course Scheduling: In academic planning, courses and their prerequisites form a DAG. The longest path can help determine the minimum number of semesters needed to complete a set of courses.
- Pipeline Processing: In computer architecture, instruction pipelines can be modeled as DAGs. The longest path represents the critical path that determines the clock cycle time.
10. Common Interview Questions and Variations
When preparing for technical interviews, it’s helpful to consider variations of the longest path in DAG problem. Here are some common questions and variations you might encounter:
- Shortest Path in DAG: This is similar to the longest path problem, but you’re minimizing instead of maximizing. The approach is nearly identical, just change max to min in the dynamic programming step.
- All Paths in DAG: Instead of finding just the longest path, you might be asked to find all paths from a source to a destination in a DAG. This can be solved using DFS or dynamic programming.
- Longest Path with Constraints: You might be given additional constraints, such as finding the longest path that passes through a specific vertex or set of vertices.
- Longest Increasing Path in a Matrix: This is a popular variation where you’re given a matrix of integers and need to find the length of the longest increasing path. This problem can be solved by treating the matrix as a DAG.
- Parallel Task Scheduling: Given a DAG representing task dependencies and multiple processors, schedule the tasks to minimize the total execution time. This is related to the longest path problem as the minimum time is determined by the critical path.
For each of these variations, the key is to recognize how they relate to the basic longest path in DAG problem and adapt the solution accordingly.
11. Conclusion
The longest path in a Directed Acyclic Graph is a fascinating problem that combines several important concepts in computer science: graph theory, dynamic programming, and topological sorting. By mastering this problem, you’re not only preparing yourself for technical interviews but also gaining insights into solving a wide range of real-world problems.
Remember, the key steps to solving this problem are:
- Understand the properties of DAGs
- Perform a topological sort
- Use dynamic programming to build the solution
As you continue your journey in algorithm mastery, try to solve variations of this problem and think about how you can apply these concepts to other graph problems. Happy coding, and best of luck in your technical interviews!
If you found this article helpful, don’t forget to check out our other tutorials and practice problems on AlgoCademy. Our platform offers a wealth of resources to help you prepare for technical interviews and improve your coding skills. Keep learning, keep practicing, and remember – every expert was once a beginner!