Mastering the Activity Selection Problem: A Comprehensive Guide
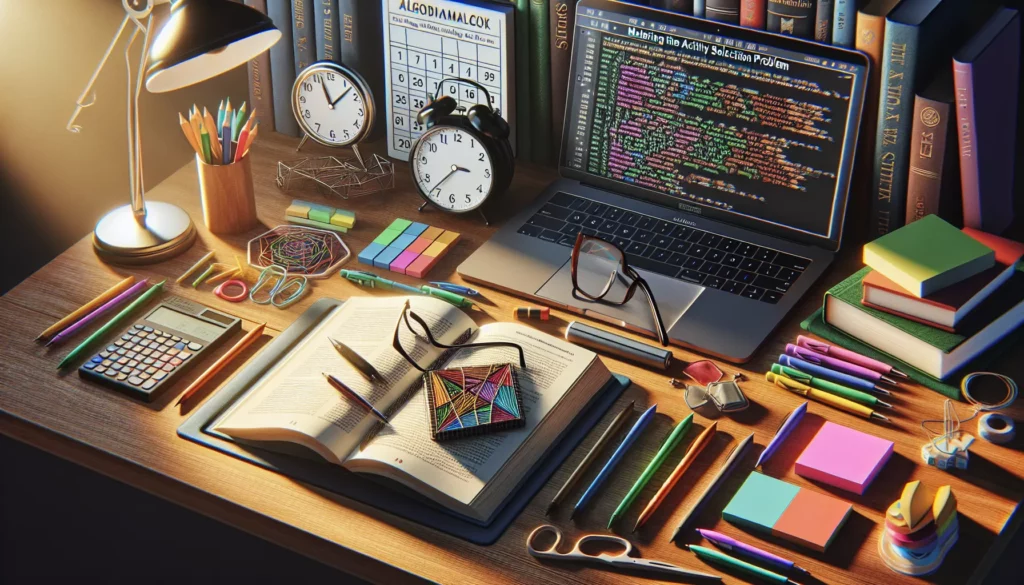
Welcome to another exciting deep dive into algorithmic problem-solving! Today, we’re tackling the Activity Selection Problem, a classic challenge that’s not only fascinating but also highly relevant in real-world scenarios. Whether you’re preparing for technical interviews at top tech companies or simply looking to sharpen your coding skills, understanding this problem and its solutions will significantly boost your algorithmic thinking.
What is the Activity Selection Problem?
The Activity Selection Problem is a optimization problem in computer science and operations research. It involves selecting the maximum number of non-overlapping activities that can be performed by a single person or machine, given their start and finish times.
Here’s a more concrete example:
Imagine you’re managing a conference room, and you have a list of meeting requests, each with a start time and an end time. Your goal is to schedule as many meetings as possible without any overlaps. This scenario perfectly illustrates the Activity Selection Problem.
Why is the Activity Selection Problem Important?
Understanding and solving the Activity Selection Problem is crucial for several reasons:
- Real-world Applications: This problem has numerous practical applications in scheduling, resource allocation, and time management.
- Algorithmic Thinking: It helps develop your ability to think algorithmically and approach complex problems systematically.
- Interview Preparation: The Activity Selection Problem is a favorite among interviewers at major tech companies, including FAANG (Facebook, Amazon, Apple, Netflix, Google).
- Greedy Algorithms: It’s an excellent introduction to greedy algorithms, a powerful problem-solving technique in computer science.
Problem Formulation
Let’s formally define the Activity Selection Problem:
Given a set of n activities, each with a start time (si) and finish time (fi), select the maximum number of non-overlapping activities.
Two activities i and j are considered non-overlapping if si >= fj or sj >= fi.
Approach to Solving the Activity Selection Problem
The most efficient approach to solve this problem is using a Greedy Algorithm. Here’s the step-by-step process:
- Sort all activities according to their finish time.
- Select the first activity (the one that finishes first).
- For the remaining activities, select an activity if its start time is greater than or equal to the finish time of the previously selected activity.
This approach works because by always choosing the activity that finishes first, we’re leaving as much time as possible for the remaining activities, thus maximizing our options.
Implementing the Solution
Let’s implement this solution in Python:
def activity_selection(activities):
# Sort activities based on finish time
sorted_activities = sorted(activities, key=lambda x: x[1])
selected = [sorted_activities[0]] # Select the first activity
last_finish = sorted_activities[0][1]
for activity in sorted_activities[1:]:
if activity[0] >= last_finish:
selected.append(activity)
last_finish = activity[1]
return selected
# Example usage
activities = [(1, 4), (3, 5), (0, 6), (5, 7), (3, 8), (5, 9), (6, 10), (8, 11), (8, 12), (2, 13), (12, 14)]
result = activity_selection(activities)
print("Selected activities:")
for activity in result:
print(f"Start time: {activity[0]}, Finish time: {activity[1]}")
This implementation does the following:
- We define a function
activity_selection
that takes a list of activities as input. - We sort the activities based on their finish time using the
sorted()
function with a lambda function as the key. - We select the first activity (the one that finishes earliest) and keep track of its finish time.
- We iterate through the remaining activities, selecting those that start after the finish time of the previously selected activity.
- Finally, we return the list of selected activities.
Time Complexity Analysis
Let’s break down the time complexity of our solution:
- Sorting the activities: O(n log n), where n is the number of activities.
- Iterating through the sorted activities: O(n)
Therefore, the overall time complexity is O(n log n), which is dominated by the sorting step.
Space Complexity Analysis
The space complexity of our solution is O(n), where n is the number of activities. This is because we need to store the sorted list of activities and the list of selected activities.
Variations of the Activity Selection Problem
While we’ve covered the basic Activity Selection Problem, there are several interesting variations you might encounter:
1. Weighted Activity Selection
In this variation, each activity has a weight or value associated with it. The goal is to select activities that maximize the total value rather than just the number of activities.
This problem can be solved using Dynamic Programming. Here’s a basic implementation:
def weighted_activity_selection(activities):
# Sort activities based on finish time
sorted_activities = sorted(activities, key=lambda x: x[1])
n = len(sorted_activities)
# Initialize DP table
dp = [0] * n
dp[0] = sorted_activities[0][2] # Value of first activity
for i in range(1, n):
# Find the last non-overlapping activity
j = i - 1
while j >= 0 and sorted_activities[j][1] > sorted_activities[i][0]:
j -= 1
# Calculate maximum value
if j >= 0:
dp[i] = max(dp[i-1], dp[j] + sorted_activities[i][2])
else:
dp[i] = max(dp[i-1], sorted_activities[i][2])
return dp[n-1]
# Example usage
activities = [(1, 4, 5), (3, 5, 6), (0, 6, 8), (5, 7, 4), (3, 8, 3), (5, 9, 7), (6, 10, 2), (8, 11, 1), (8, 12, 3), (2, 13, 10), (12, 14, 6)]
result = weighted_activity_selection(activities)
print(f"Maximum value: {result}")
2. Activity Selection with Multiple Resources
In this variation, you have multiple resources (e.g., multiple conference rooms) and you want to schedule as many activities as possible across all resources.
This problem can be solved using a greedy approach similar to the original problem, but with the addition of a min-heap to keep track of the earliest available resource:
import heapq
def activity_selection_multiple_resources(activities, k):
# Sort activities based on start time
sorted_activities = sorted(activities, key=lambda x: x[0])
resources = [0] * k # Initialize k resources
heapq.heapify(resources)
count = 0
for activity in sorted_activities:
earliest_available = heapq.heappop(resources)
if earliest_available <= activity[0]:
heapq.heappush(resources, activity[1])
count += 1
else:
heapq.heappush(resources, earliest_available)
return count
# Example usage
activities = [(1, 4), (3, 5), (0, 6), (5, 7), (3, 8), (5, 9), (6, 10), (8, 11), (8, 12), (2, 13), (12, 14)]
k = 3 # Number of resources
result = activity_selection_multiple_resources(activities, k)
print(f"Maximum number of activities that can be scheduled: {result}")
Real-world Applications
The Activity Selection Problem and its variations have numerous real-world applications:
- Scheduling: Conference room booking systems, class scheduling in schools and universities.
- Resource Allocation: Allocating machines to jobs in a factory, assigning tasks to processors in a computer system.
- Transportation: Scheduling flights at an airport, managing railway platforms.
- Project Management: Scheduling tasks in a project with limited resources.
- Sports and Entertainment: Organizing tournaments, scheduling TV programs.
Common Mistakes and Pitfalls
When solving the Activity Selection Problem, be aware of these common mistakes:
- Sorting by Start Time: A common mistake is to sort activities by start time instead of finish time. This doesn’t guarantee an optimal solution.
- Not Handling Edge Cases: Make sure your solution handles edge cases like empty input or activities with zero duration.
- Overlooking the Greedy Choice Property: The key to the greedy approach is understanding why selecting the activity that finishes first is always optimal.
- Inefficient Implementation: Using nested loops to check for conflicts can lead to O(n^2) time complexity, which is inefficient for large inputs.
Practice Problems
To reinforce your understanding of the Activity Selection Problem, try solving these related problems:
- Non-overlapping Intervals (LeetCode)
- Meeting Rooms II (LeetCode – Premium)
- Weighted Job Scheduling (GeeksforGeeks)
- Activity Selection Problem (GeeksforGeeks)
Advanced Concepts
Once you’re comfortable with the basic Activity Selection Problem, you can explore these advanced concepts:
- Interval Scheduling: A generalization of the Activity Selection Problem where activities can have different weights or values.
- Online Scheduling: Solving the Activity Selection Problem when activities arrive in real-time and decisions must be made immediately.
- Approximation Algorithms: When dealing with NP-hard variations of the problem, approximation algorithms can provide near-optimal solutions in polynomial time.
- Interval Graphs: Understanding the connection between the Activity Selection Problem and interval graphs can provide deeper insights into the problem structure.
Conclusion
The Activity Selection Problem is a fundamental challenge in computer science that showcases the power of greedy algorithms. By mastering this problem, you’re not only preparing yourself for technical interviews but also developing crucial problem-solving skills applicable to a wide range of real-world scenarios.
Remember, the key to solving the Activity Selection Problem efficiently lies in the greedy choice of always selecting the activity that finishes first. This simple yet powerful insight allows us to develop an O(n log n) algorithm that optimally solves the problem.
As you continue your journey in algorithmic problem-solving, keep practicing variations of this problem and exploring its connections to other areas of computer science. The skills you develop here will serve you well in tackling more complex challenges in the future.
Happy coding, and may your activities always be optimally selected!