Job Scheduling Problem: Mastering the Weighted Interval Scheduling Algorithm
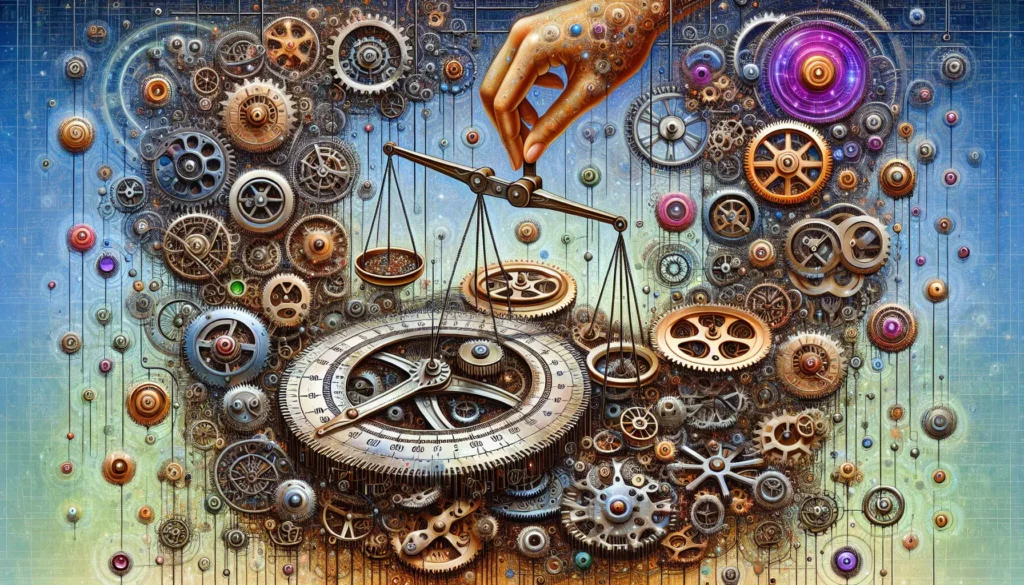
In the world of computer science and algorithm design, efficient resource allocation and optimization are crucial skills. One classic problem that exemplifies these concepts is the Job Scheduling Problem, particularly its variant known as Weighted Interval Scheduling. This problem not only serves as an excellent exercise in algorithmic thinking but also has practical applications in various fields, from operating systems to project management.
In this comprehensive guide, we’ll dive deep into the Weighted Interval Scheduling problem, exploring its intricacies, solution approaches, and real-world applications. By the end of this article, you’ll have a solid understanding of this important algorithmic concept, which will undoubtedly boost your problem-solving skills and prepare you for technical interviews at top tech companies.
Understanding the Job Scheduling Problem
Before we delve into the specifics of Weighted Interval Scheduling, let’s first understand the broader context of the Job Scheduling Problem.
What is the Job Scheduling Problem?
The Job Scheduling Problem is a class of optimization problems in computer science and operations research. It involves assigning a set of jobs or tasks to limited resources (such as machines or time slots) in a way that optimizes certain objectives. These objectives could include minimizing the total completion time, maximizing the number of completed jobs, or maximizing the total value of completed jobs.
Types of Job Scheduling Problems
There are several variants of the Job Scheduling Problem, each with its own set of constraints and objectives. Some common types include:
- Single Machine Scheduling: All jobs are processed on a single machine, one at a time.
- Parallel Machine Scheduling: Multiple identical machines are available to process jobs simultaneously.
- Flow Shop Scheduling: Jobs must be processed in a specific order through a series of machines.
- Job Shop Scheduling: Each job has its own specific processing order through a set of machines.
- Interval Scheduling: Jobs have fixed start and end times, and the goal is to schedule as many non-overlapping jobs as possible.
In this article, we’ll focus on a specific variant of Interval Scheduling known as Weighted Interval Scheduling.
Weighted Interval Scheduling: Problem Definition
Weighted Interval Scheduling is a more complex version of the standard Interval Scheduling problem. Here’s a formal definition of the problem:
Given:
- A set of n jobs
- Each job i has:
- A start time si
- An end time fi
- A weight (or value) wi
Objective: Find a subset of non-overlapping jobs that maximizes the total weight.
The key difference between this and the standard Interval Scheduling problem is the introduction of weights. In the standard version, we simply aim to schedule as many non-overlapping jobs as possible. In the weighted version, we need to consider the value of each job and find the combination that yields the highest total value.
Example Scenario
Let’s consider a simple example to illustrate the problem:
Job 1: Start time = 1, End time = 4, Weight = 3
Job 2: Start time = 2, End time = 6, Weight = 5
Job 3: Start time = 4, End time = 7, Weight = 2
Job 4: Start time = 6, End time = 8, Weight = 4
In this scenario, we can’t schedule all jobs because some of them overlap. We need to find the combination of non-overlapping jobs that gives us the maximum total weight.
Approaches to Solving Weighted Interval Scheduling
Now that we understand the problem, let’s explore different approaches to solving it, starting from the simplest and moving towards the most efficient.
1. Brute Force Approach
The most straightforward approach is to consider all possible combinations of jobs, check which ones are valid (non-overlapping), and select the one with the highest total weight.
Algorithm:
- Generate all 2n subsets of the n jobs.
- For each subset:
- Check if the jobs in the subset are non-overlapping.
- If they are, calculate the total weight of the subset.
- Return the subset with the highest total weight among all valid subsets.
Time Complexity: O(2n), where n is the number of jobs.
While this approach guarantees finding the optimal solution, it becomes impractical for even moderately sized inputs due to its exponential time complexity.
2. Greedy Approach
A greedy approach involves making the locally optimal choice at each step, hoping to find a global optimum. For the Weighted Interval Scheduling problem, we might try the following greedy strategies:
- Select jobs in order of earliest end time.
- Select jobs in order of shortest duration.
- Select jobs in order of highest weight.
- Select jobs in order of highest weight/duration ratio.
However, none of these greedy strategies guarantee an optimal solution for all instances of the problem. They may work well for some cases but fail for others.
3. Dynamic Programming Approach
Dynamic Programming (DP) is the most efficient and reliable method for solving the Weighted Interval Scheduling problem. It breaks down the problem into smaller subproblems and builds up the solution incrementally.
The key insight for the DP approach is that for each job, we have two choices:
- Include the job in our solution
- Exclude the job from our solution
If we include a job, we need to ensure that we exclude all jobs that overlap with it.
Algorithm:
- Sort the jobs by end time.
- For each job i, find p(i), the largest index j < i such that job j is compatible with job i.
- Define OPT(i) as the maximum weight of a schedule using only the first i jobs.
- Use the recurrence relation:
OPT(i) = max(w[i] + OPT(p(i)), OPT(i-1))
- Build the DP table bottom-up.
- Reconstruct the solution by backtracking through the DP table.
Time Complexity: O(n log n), where n is the number of jobs (dominated by the initial sorting step).
Implementing the Dynamic Programming Solution
Let’s implement the Dynamic Programming solution in Python. We’ll break it down into steps for clarity.
Step 1: Define the Job class and sort the jobs
class Job:
def __init__(self, start, finish, weight):
self.start = start
self.finish = finish
self.weight = weight
def weighted_interval_scheduling(jobs):
# Sort jobs by finish time
sorted_jobs = sorted(jobs, key=lambda j: j.finish)
n = len(sorted_jobs)
# Rest of the implementation will go here
# Example usage
jobs = [
Job(1, 4, 3),
Job(2, 6, 5),
Job(4, 7, 2),
Job(6, 8, 4),
]
result = weighted_interval_scheduling(jobs)
print(f"Maximum weight: {result[0]}")
print("Selected jobs:")
for job in result[1]:
print(f"Start: {job.start}, Finish: {job.finish}, Weight: {job.weight}")
Step 2: Implement the p(i) function
def binary_search(jobs, i):
lo = 0
hi = i - 1
while lo <= hi:
mid = (lo + hi) // 2
if jobs[mid].finish <= jobs[i].start:
if jobs[mid + 1].finish <= jobs[i].start:
lo = mid + 1
else:
return mid
else:
hi = mid - 1
return -1
# Add this inside the weighted_interval_scheduling function
p = [binary_search(sorted_jobs, i) for i in range(n)]
Step 3: Implement the Dynamic Programming solution
# Add this inside the weighted_interval_scheduling function
dp = [0] * (n + 1)
for i in range(1, n + 1):
# Case 1: Include job i
include = sorted_jobs[i-1].weight
if p[i-1] != -1:
include += dp[p[i-1] + 1]
# Case 2: Exclude job i
exclude = dp[i-1]
# Take the maximum
dp[i] = max(include, exclude)
# Reconstruct the solution
def find_solution(i):
if i == 0:
return []
if i == 1:
return [sorted_jobs[0]] if dp[1] > 0 else []
include = sorted_jobs[i-1].weight
if p[i-1] != -1:
include += dp[p[i-1] + 1]
if include > dp[i-1]:
return find_solution(p[i-1] + 1) + [sorted_jobs[i-1]]
else:
return find_solution(i-1)
solution = find_solution(n)
return dp[n], solution
This implementation solves the Weighted Interval Scheduling problem efficiently using Dynamic Programming. It returns both the maximum weight achievable and the list of jobs that should be scheduled to achieve this maximum weight.
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our Dynamic Programming solution:
Time Complexity
- Sorting: O(n log n)
- Computing p(i) for all i: O(n log n) (binary search for each job)
- Filling the DP table: O(n)
- Reconstructing the solution: O(n) in the worst case
The overall time complexity is dominated by the sorting and p(i) computation steps, resulting in O(n log n).
Space Complexity
- Sorted jobs array: O(n)
- p array: O(n)
- DP table: O(n)
The overall space complexity is O(n).
Real-world Applications
The Weighted Interval Scheduling problem and its solutions have numerous practical applications across various domains:
1. Resource Allocation in Computing Systems
In operating systems and cloud computing environments, the algorithm can be used to schedule tasks or virtual machines on physical resources, maximizing resource utilization and prioritizing high-value tasks.
2. Bandwidth Allocation in Networks
Network providers can use this algorithm to allocate bandwidth to different clients or services, ensuring that high-priority or high-paying customers receive the desired quality of service.
3. Project Management
In project planning, the algorithm can help managers select the most valuable combination of projects to undertake given limited time and resources.
4. Advertisement Scheduling
Media companies can use this algorithm to schedule advertisements in a way that maximizes revenue, considering the time slots and the value of each ad.
5. Transportation and Logistics
In logistics, the algorithm can be applied to optimize the scheduling of deliveries or the allocation of vehicles to routes, maximizing the value of completed deliveries.
Variations and Extensions
The Weighted Interval Scheduling problem has several interesting variations and extensions:
1. Multiple Resource Scheduling
Instead of a single resource (like a single machine), we might have multiple identical resources available. This variation is known as the Multiple Resource Interval Scheduling problem.
2. Online Scheduling
In the online version of the problem, jobs arrive one at a time, and decisions must be made without knowledge of future jobs. This requires different algorithmic approaches, often involving competitive analysis.
3. Scheduling with Conflicts
In this variation, in addition to time conflicts, there might be other types of conflicts between jobs (e.g., they require the same unique resource). This adds another layer of complexity to the problem.
4. Interval Scheduling with Machine Cost
Here, there’s an additional cost associated with using each machine, and the objective is to schedule all jobs while minimizing the number of machines used.
Conclusion
The Weighted Interval Scheduling problem is a fascinating algorithmic challenge that combines elements of optimization, dynamic programming, and practical resource allocation. By mastering this problem and its solutions, you’ll not only enhance your problem-solving skills but also gain insights into efficient algorithm design that can be applied to a wide range of real-world scenarios.
As you continue your journey in computer science and prepare for technical interviews, remember that understanding problems like Weighted Interval Scheduling is crucial. It demonstrates your ability to think algorithmically, optimize for efficiency, and translate abstract problems into practical solutions.
Keep practicing, exploring variations of this problem, and applying the concepts you’ve learned to new challenges. With dedication and consistent effort, you’ll be well-prepared to tackle complex algorithmic problems in your coding interviews and your future career in tech.