Mastering the Unbounded Knapsack Problem: A Comprehensive Guide
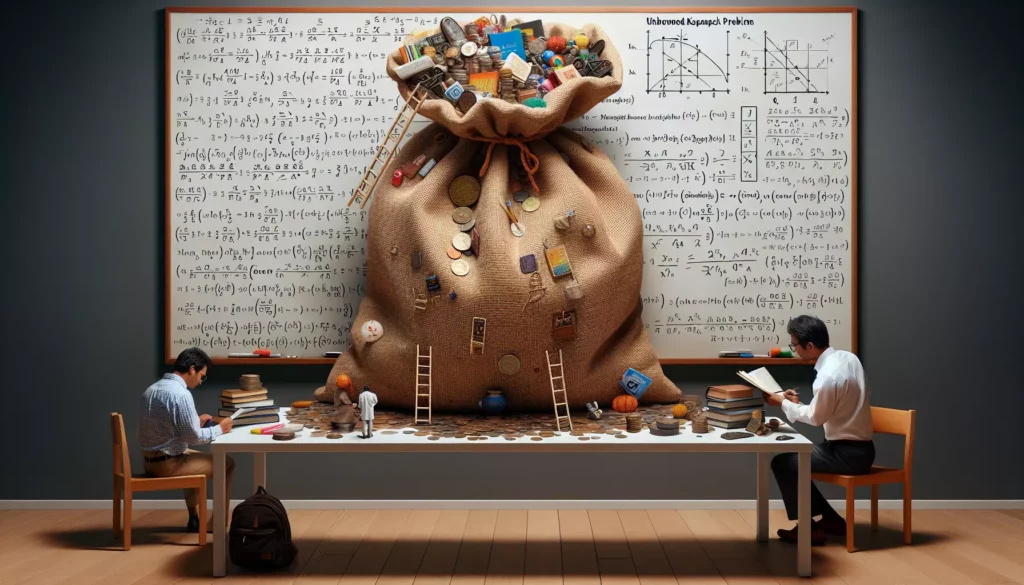
Welcome to our in-depth exploration of the Unbounded Knapsack problem, a classic algorithmic challenge that tests your problem-solving skills and dynamic programming prowess. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your coding abilities, understanding this problem is crucial. In this comprehensive guide, we’ll break down the Unbounded Knapsack problem, explore its intricacies, and provide you with the tools to solve it efficiently.
What is the Unbounded Knapsack Problem?
The Unbounded Knapsack problem is a variation of the classic Knapsack problem in computer science and mathematics. In this scenario, you’re given a knapsack with a maximum weight capacity and a set of items, each with its own weight and value. The goal is to fill the knapsack with items to maximize the total value while staying within the weight limit. The key difference in the Unbounded Knapsack problem is that you can use each item an unlimited number of times, unlike the 0/1 Knapsack problem where each item can be used only once.
Problem Statement
Given:
- A set of n items, each with a weight w[i] and a value v[i]
- A knapsack with a maximum weight capacity W
Objective: Determine the maximum value that can be achieved by selecting items (with repetition allowed) such that the total weight does not exceed W.
Understanding the Challenge
The Unbounded Knapsack problem presents several key challenges:
- Optimization: We need to find the optimal combination of items that maximizes value while respecting the weight constraint.
- Repetition: Unlike the 0/1 Knapsack, we can use items multiple times, adding complexity to our decision-making process.
- Efficiency: As with many algorithmic problems, we need to solve this efficiently, avoiding unnecessary computations and optimizing our approach.
Approaching the Solution
To solve the Unbounded Knapsack problem, we’ll use dynamic programming. This approach allows us to break down the problem into smaller subproblems and build up to the final solution, avoiding redundant calculations along the way.
The Dynamic Programming Approach
Here’s how we can approach the problem using dynamic programming:
- Create a DP (Dynamic Programming) table to store intermediate results.
- Initialize the base cases.
- Iterate through all possible weights up to the knapsack’s capacity.
- For each weight, consider all items and update the maximum value achievable.
- The final cell in the DP table will contain the maximum value for the given knapsack capacity.
Implementing the Solution
Let’s implement the solution in Python. We’ll create a function that takes the knapsack capacity, weights, and values as inputs and returns the maximum achievable value.
def unbounded_knapsack(W, n, weights, values):
dp = [0 for i in range(W + 1)]
for w in range(W + 1):
for i in range(n):
if weights[i] <= w:
dp[w] = max(dp[w], dp[w - weights[i]] + values[i])
return dp[W]
# Example usage
W = 100
weights = [10, 20, 30]
values = [60, 100, 120]
n = len(weights)
max_value = unbounded_knapsack(W, n, weights, values)
print(f"Maximum value in Knapsack = {max_value}")
In this implementation:
- We create a DP table
dp
wheredp[i]
represents the maximum value achievable with a knapsack of capacity i. - We iterate through all possible weights from 0 to W.
- For each weight, we consider all items and update the maximum value if including the current item leads to a better solution.
- The final answer is stored in
dp[W]
.
Time and Space Complexity
Let’s analyze the time and space complexity of our solution:
- Time Complexity: O(W * n), where W is the knapsack capacity and n is the number of items. We have two nested loops: one iterating W times and the inner loop iterating n times.
- Space Complexity: O(W), as we only need a 1D array of size W + 1 to store our DP values.
This solution is quite efficient, especially compared to a naive recursive approach which would have exponential time complexity.
Optimizing the Solution
While our current solution is already efficient, there are a few optimizations we can consider:
1. Early Termination
If we know that no item has a weight smaller than a certain value, we can start our DP calculation from that value instead of 0. This can be particularly useful when the minimum item weight is large compared to the knapsack capacity.
def optimized_unbounded_knapsack(W, n, weights, values):
min_weight = min(weights)
dp = [0 for i in range(W + 1)]
for w in range(min_weight, W + 1):
for i in range(n):
if weights[i] <= w:
dp[w] = max(dp[w], dp[w - weights[i]] + values[i])
return dp[W]
2. Value-to-Weight Ratio Sorting
While this doesn’t change the worst-case time complexity, sorting items by their value-to-weight ratio and considering higher ratio items first can lead to faster convergence to the optimal solution in practice.
def ratio_sorted_unbounded_knapsack(W, n, weights, values):
items = sorted(zip(weights, values), key=lambda x: x[1]/x[0], reverse=True)
dp = [0 for i in range(W + 1)]
for w in range(W + 1):
for weight, value in items:
if weight <= w:
dp[w] = max(dp[w], dp[w - weight] + value)
return dp[W]
Variations and Related Problems
Understanding the Unbounded Knapsack problem opens the door to solving various related problems. Here are a few variations you might encounter:
1. Rod Cutting Problem
Given a rod of length n and prices of pieces of different lengths, determine the maximum value obtainable by cutting up the rod and selling the pieces.
2. Coin Change Problem
Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.
3. Minimum Cost to Fill Given Weight in a Bag
Similar to the Unbounded Knapsack, but the goal is to minimize the cost while reaching a specific weight.
Real-World Applications
The Unbounded Knapsack problem and its variations have numerous real-world applications:
- Finance: Portfolio optimization with fractional shares.
- Manufacturing: Cutting stock problems in industries like paper and metal.
- Logistics: Optimal loading of cargo with repeatable items.
- Resource Allocation: Distributing resources in project management or computing environments.
Tips for Solving Knapsack Problems in Interviews
When tackling Knapsack problems in technical interviews, keep these tips in mind:
- Identify the Problem Type: Determine whether it’s a 0/1 Knapsack or Unbounded Knapsack problem.
- Start with a Simple Approach: Begin with a recursive solution to demonstrate your understanding of the problem.
- Optimize with DP: Explain how you can improve the solution using dynamic programming.
- Consider Space Optimization: Discuss potential ways to optimize space usage, such as using a 1D array instead of a 2D array.
- Analyze Complexity: Be prepared to discuss the time and space complexity of your solution.
- Handle Edge Cases: Consider scenarios like empty input, negative weights or values, etc.
- Propose Further Optimizations: If time allows, suggest additional optimizations or discuss how you might handle very large inputs.
Practice Problems
To reinforce your understanding of the Unbounded Knapsack problem, try solving these related problems:
- Coin Change (LeetCode 322)
- Coin Change 2 (LeetCode 518)
- Rod Cutting Problem (GeeksforGeeks)
- Minimum Cost to Cut a Stick (LeetCode 1547)
Conclusion
Mastering the Unbounded Knapsack problem is a significant milestone in your journey as a programmer and problem solver. This problem not only tests your ability to think algorithmically but also introduces you to the powerful technique of dynamic programming. By understanding and implementing efficient solutions to the Unbounded Knapsack problem, you’re building a strong foundation for tackling a wide range of optimization challenges in computer science and beyond.
Remember, the key to mastering these types of problems is practice. Don’t just read about the solutions – implement them, experiment with different inputs, and try to optimize your code further. As you become more comfortable with the Unbounded Knapsack problem, you’ll find that the problem-solving strategies you’ve learned here will apply to many other algorithmic challenges you’ll face in your coding journey.
Keep coding, keep learning, and don’t hesitate to dive deep into complex problems like this one. Your skills in solving such algorithmic puzzles will set you apart in technical interviews and make you a more versatile and capable programmer. Happy coding!