Range Minimum Query: Mastering Segment Trees and Sparse Tables
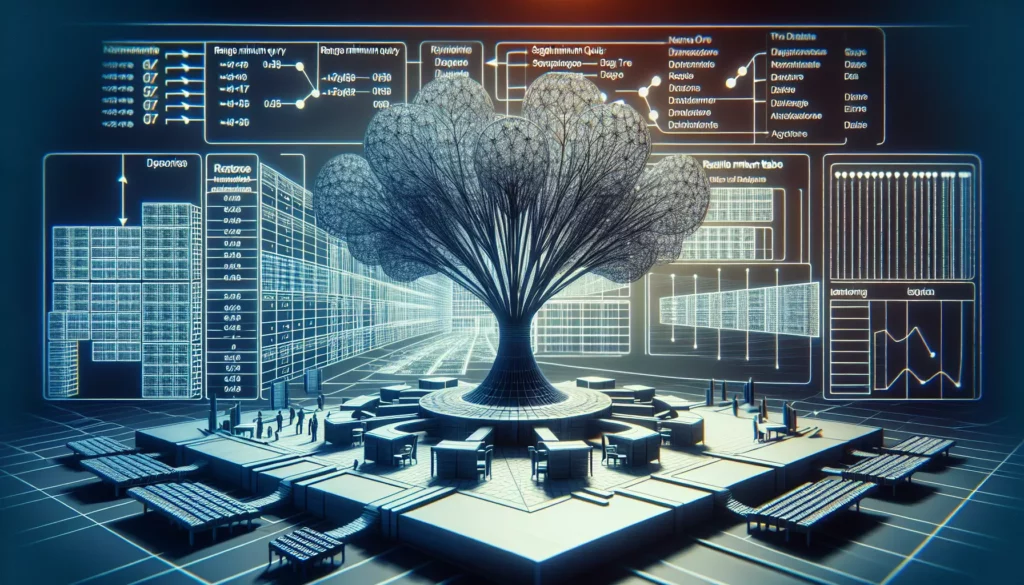
In the world of competitive programming and technical interviews, efficient data structures and algorithms are the key to solving complex problems. One such problem that frequently appears in coding challenges and real-world applications is the Range Minimum Query (RMQ). In this comprehensive guide, we’ll dive deep into two powerful techniques for solving RMQ problems: Segment Trees and Sparse Tables. By the end of this article, you’ll have a solid understanding of these data structures and be well-equipped to tackle RMQ problems in your coding interviews or competitions.
What is Range Minimum Query?
Before we delve into the solutions, let’s first understand what a Range Minimum Query problem is. Given an array of numbers, an RMQ asks for the minimum value in a specified range of the array. For example, if we have an array [3, 1, 4, 1, 5, 9, 2, 6], and we want to find the minimum value between indices 2 and 5 (inclusive), the answer would be 1.
While this problem might seem simple at first glance, it becomes challenging when we need to perform multiple such queries efficiently. A naive approach of iterating through the range for each query would result in O(n) time complexity per query, which is not optimal for large datasets or numerous queries.
Segment Trees: A Powerful Data Structure for RMQ
Segment Trees are versatile data structures that allow us to perform various range queries, including RMQ, efficiently. They work by dividing the array into segments and storing precomputed results for these segments in a tree-like structure.
Building a Segment Tree
To construct a Segment Tree for RMQ, we follow these steps:
- Create a binary tree with the original array elements as leaf nodes.
- Each internal node represents the minimum value of its children.
- The root node contains the minimum value of the entire array.
Here’s a C++ implementation of the Segment Tree construction:
#include <vector>
#include <limits>
class SegmentTree {
private:
std::vector<int> tree;
int n;
void build(const std::vector<int>& arr, int node, int start, int end) {
if (start == end) {
tree[node] = arr[start];
} else {
int mid = (start + end) / 2;
build(arr, 2 * node, start, mid);
build(arr, 2 * node + 1, mid + 1, end);
tree[node] = std::min(tree[2 * node], tree[2 * node + 1]);
}
}
public:
SegmentTree(const std::vector<int>& arr) {
n = arr.size();
tree.resize(4 * n);
build(arr, 1, 0, n - 1);
}
// ... (query and update methods will be added here)
};
In this implementation, we use a vector to represent the tree, where each node’s children are at indices 2*node and 2*node+1. The build function recursively constructs the tree by dividing the array into halves and storing the minimum values at each node.
Querying the Segment Tree
To perform a range minimum query on the Segment Tree, we use a recursive approach:
- If the current node’s range is completely inside the query range, return the node’s value.
- If the current node’s range is completely outside the query range, return infinity (or a large value).
- Otherwise, split the range and recursively query both children, then return the minimum of the two results.
Here’s the C++ implementation of the query function:
int query(int node, int start, int end, int l, int r) {
if (r < start || end < l) {
return std::numeric_limits<int>::max();
}
if (l <= start && end <= r) {
return tree[node];
}
int mid = (start + end) / 2;
int left = query(2 * node, start, mid, l, r);
int right = query(2 * node + 1, mid + 1, end, l, r);
return std::min(left, right);
}
int rangeMinimumQuery(int l, int r) {
return query(1, 0, n - 1, l, r);
}
Updating the Segment Tree
One of the advantages of Segment Trees is that they allow for efficient updates to the original array. When an element is updated, we need to recalculate the minimum values along the path from the leaf node to the root.
Here’s how we can implement the update function:
void update(int node, int start, int end, int idx, int val) {
if (start == end) {
tree[node] = val;
} else {
int mid = (start + end) / 2;
if (idx <= mid) {
update(2 * node, start, mid, idx, val);
} else {
update(2 * node + 1, mid + 1, end, idx, val);
}
tree[node] = std::min(tree[2 * node], tree[2 * node + 1]);
}
}
void updateValue(int idx, int val) {
update(1, 0, n - 1, idx, val);
}
Time and Space Complexity
The Segment Tree offers the following complexities:
- Construction: O(n)
- Query: O(log n)
- Update: O(log n)
- Space: O(n)
These efficient complexities make Segment Trees an excellent choice for problems involving frequent range queries and updates.
Sparse Tables: A Static RMQ Solution
While Segment Trees are versatile and allow for updates, there are scenarios where we only need to perform queries on a static array. In such cases, Sparse Tables provide an even more efficient solution for RMQ problems.
Building a Sparse Table
The Sparse Table algorithm precomputes all possible range minimum queries for power-of-two sized ranges. It’s based on the concept of dynamic programming and the fact that any range can be expressed as the minimum of two overlapping power-of-two sized ranges.
Here’s how we can implement the Sparse Table construction in C++:
#include <vector>
#include <cmath>
class SparseTable {
private:
std::vector<std::vector<int>> table;
std::vector<int> log;
int n;
public:
SparseTable(const std::vector<int>& arr) {
n = arr.size();
int max_log = std::log2(n) + 1;
table.resize(n, std::vector<int>(max_log));
log.resize(n + 1);
// Precompute logs
log[1] = 0;
for (int i = 2; i <= n; i++) {
log[i] = log[i / 2] + 1;
}
// Initialize the table with the original array
for (int i = 0; i < n; i++) {
table[i][0] = arr[i];
}
// Build the sparse table
for (int j = 1; j < max_log; j++) {
for (int i = 0; i + (1 << j) <= n; i++) {
table[i][j] = std::min(table[i][j-1], table[i + (1 << (j-1))][j-1]);
}
}
}
// ... (query method will be added here)
};
In this implementation, we create a 2D vector table
where table[i][j]
stores the minimum value in the range [i, i + 2^j – 1]. We also precompute logarithms to optimize the query process.
Querying the Sparse Table
To perform a range minimum query using the Sparse Table, we find the largest power of two that fits within the query range and take the minimum of two overlapping intervals:
int rangeMinimumQuery(int l, int r) {
int j = log[r - l + 1];
return std::min(table[l][j], table[r - (1 << j) + 1][j]);
}
Time and Space Complexity
The Sparse Table offers the following complexities:
- Construction: O(n log n)
- Query: O(1)
- Space: O(n log n)
The constant-time query makes Sparse Tables extremely efficient for scenarios with numerous queries on a static array.
Comparing Segment Trees and Sparse Tables
Both Segment Trees and Sparse Tables are powerful tools for solving RMQ problems, but they have different strengths and use cases:
Segment Trees:
- Allow for updates to the original array
- More versatile, can be used for various range queries (sum, max, etc.)
- Better space complexity: O(n) vs O(n log n) for Sparse Tables
- Logarithmic time for both queries and updates
Sparse Tables:
- Provide constant-time queries
- Simpler to implement for RMQ specifically
- More efficient for scenarios with only queries (no updates)
- Higher preprocessing time and space complexity
Real-world Applications of RMQ
Range Minimum Query and its related data structures have numerous practical applications beyond coding interviews and competitions. Some real-world use cases include:
- Financial analysis: Finding the minimum stock price over a given time range.
- Geographic Information Systems (GIS): Querying the lowest elevation point in a specific area.
- Network routing: Finding the minimum latency path between two nodes in a network.
- Image processing: Identifying the darkest pixel in a region of an image.
- Time series analysis: Detecting the lowest value in a time series dataset within a specific time window.
Advanced Topics and Variations
As you become more comfortable with RMQ and its solutions, you may want to explore some advanced topics and variations:
1. 2D Range Minimum Query
Extending RMQ to two dimensions, where you need to find the minimum value in a rectangular region of a 2D array. This can be solved using 2D Segment Trees or a combination of 1D solutions.
2. Persistent Segment Trees
A variation of Segment Trees that allows for querying previous versions of the data structure, useful in scenarios where you need to maintain historical data.
3. Lazy Propagation
An optimization technique for Segment Trees that allows for efficient range updates by delaying the propagation of updates to child nodes.
4. Fischer-Heun RMQ
An advanced algorithm that achieves O(n) preprocessing time, O(n) space, and O(1) query time for RMQ problems, combining ideas from Sparse Tables and Cartesian Trees.
5. Range Mode Query
A related problem where instead of finding the minimum, you need to find the most frequent element in a given range. This problem often requires more sophisticated data structures and algorithms.
Practice Problems
To solidify your understanding of Range Minimum Query and its solutions, try solving these practice problems:
- SPOJ – RMQSQ (Range Minimum Query)
- Codeforces – Xenia and Bit Operations
- CodeChef – Flipping Coins
- LeetCode – Range Sum Query – Mutable
- HackerEarth – Range Minimum Query
Conclusion
Range Minimum Query is a fundamental problem in computer science with wide-ranging applications. By mastering Segment Trees and Sparse Tables, you’ve added powerful tools to your algorithmic toolkit. These data structures not only solve RMQ efficiently but also form the basis for solving more complex range query problems.
As you continue your journey in competitive programming and prepare for technical interviews, remember that understanding the trade-offs between different solutions is crucial. Segment Trees offer flexibility and support for updates, while Sparse Tables provide lightning-fast queries for static data. Choosing the right approach depends on the specific requirements of the problem at hand.
Keep practicing with various RMQ problems and explore the advanced topics we’ve mentioned. With time and experience, you’ll develop the intuition to quickly recognize when and how to apply these techniques in diverse problem-solving scenarios. Happy coding, and may your range queries always be swift and efficient!