Maximum Flow and the Ford-Fulkerson Algorithm: Mastering Network Optimization
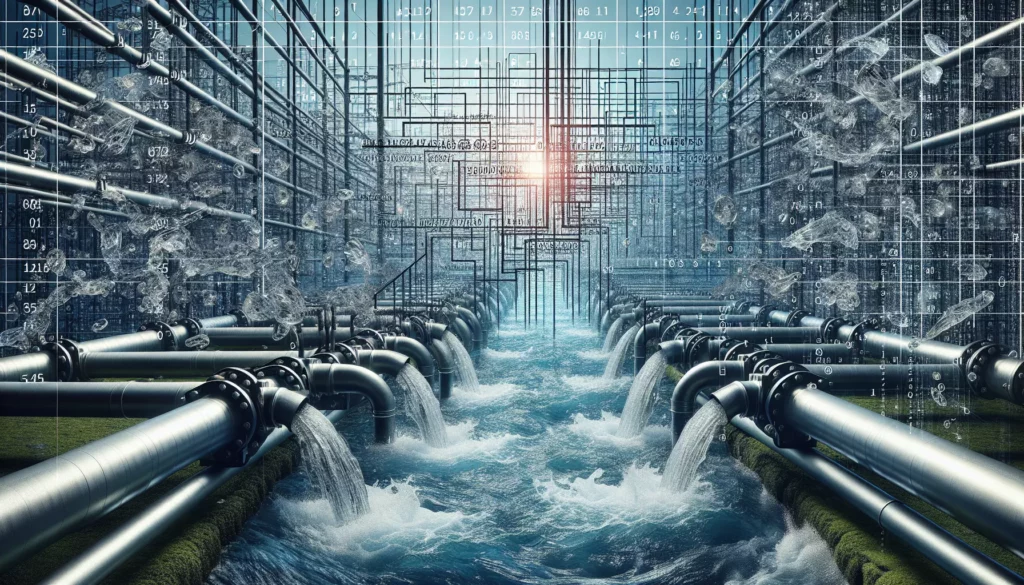
In the world of computer science and algorithm design, network flow problems play a crucial role in solving real-world optimization challenges. One of the most fundamental concepts in this domain is the maximum flow problem, which aims to determine the maximum amount of flow that can be pushed through a network from a source to a sink. At the heart of solving this problem lies the Ford-Fulkerson algorithm, a powerful method that has stood the test of time since its introduction in 1956.
As we delve into the intricacies of maximum flow and the Ford-Fulkerson algorithm, we’ll explore their applications, implementation, and significance in the broader context of algorithmic problem-solving. This knowledge is essential for anyone looking to excel in coding interviews, particularly for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google).
Understanding Maximum Flow
Before we dive into the algorithm itself, it’s crucial to understand what maximum flow is and why it’s important. The maximum flow problem is defined on a flow network, which is a directed graph where each edge has a capacity. The goal is to find the greatest rate at which material can be transported from a source vertex to a sink vertex without exceeding the capacity of any edge.
Here are some key concepts related to maximum flow:
- Flow Network: A directed graph with a source (where flow originates) and a sink (where flow terminates).
- Capacity: Each edge in the network has a maximum capacity it can handle.
- Flow: The amount of “material” moving through each edge, which must be non-negative and not exceed the edge’s capacity.
- Conservation of Flow: The amount of flow entering a vertex must equal the amount leaving it (except for the source and sink).
Maximum flow problems have numerous real-world applications, including:
- Transportation and logistics optimization
- Network routing and bandwidth allocation
- Bipartite matching in job assignments
- Image segmentation in computer vision
- Supply chain management
The Ford-Fulkerson Algorithm
The Ford-Fulkerson algorithm, named after L. R. Ford Jr. and D. R. Fulkerson, is an iterative method for computing the maximum flow in a flow network. The algorithm works by repeatedly finding augmenting paths from the source to the sink and increasing the flow along these paths until no more augmenting paths can be found.
Here’s a high-level overview of the algorithm:
- Initialize the flow in all edges to 0.
- While there exists an augmenting path from source to sink:
- Find an augmenting path
- Compute the bottleneck capacity along this path
- Increase the flow along the path by the bottleneck capacity
- When no augmenting path can be found, the maximum flow has been achieved.
Let’s break down these steps and examine them in more detail.
Finding Augmenting Paths
An augmenting path is a path from the source to the sink where each edge on the path has remaining capacity. The remaining capacity of an edge is the difference between its total capacity and the current flow through it. The algorithm can use any method to find these paths, but common approaches include:
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
Using BFS often leads to better performance, especially in dense graphs, as it tends to find shorter augmenting paths.
Computing Bottleneck Capacity
Once an augmenting path is found, we need to determine how much additional flow we can push through this path. The bottleneck capacity is the minimum remaining capacity among all edges in the path. This ensures that we don’t exceed the capacity of any edge when increasing the flow.
Increasing the Flow
After finding the bottleneck capacity, we increase the flow along the augmenting path by this amount. This involves updating the flow values for each edge in the path, increasing the flow in the forward direction and decreasing it in the backward direction (for residual edges).
Implementation of Ford-Fulkerson Algorithm
Let’s implement the Ford-Fulkerson algorithm in Python. We’ll use an adjacency list to represent the graph and implement a simple version using DFS to find augmenting paths.
from collections import defaultdict
class Graph:
def __init__(self, graph):
self.graph = graph # residual graph
self.ROW = len(graph)
def bfs(self, s, t, parent):
visited = [False] * self.ROW
queue = []
queue.append(s)
visited[s] = True
while queue:
u = queue.pop(0)
for ind, val in enumerate(self.graph[u]):
if visited[ind] == False and val > 0:
queue.append(ind)
visited[ind] = True
parent[ind] = u
if ind == t:
return True
return False
def ford_fulkerson(self, source, sink):
parent = [-1] * self.ROW
max_flow = 0
while self.bfs(source, sink, parent):
path_flow = float("Inf")
s = sink
while(s != source):
path_flow = min(path_flow, self.graph[parent[s]][s])
s = parent[s]
max_flow += path_flow
v = sink
while(v != source):
u = parent[v]
self.graph[u][v] -= path_flow
self.graph[v][u] += path_flow
v = parent[v]
return max_flow
# Example usage
graph = [[0, 16, 13, 0, 0, 0],
[0, 0, 10, 12, 0, 0],
[0, 4, 0, 0, 14, 0],
[0, 0, 9, 0, 0, 20],
[0, 0, 0, 7, 0, 4],
[0, 0, 0, 0, 0, 0]]
g = Graph(graph)
source = 0
sink = 5
print("The maximum possible flow is %d" % g.ford_fulkerson(source, sink))
This implementation uses an adjacency matrix to represent the graph. The bfs
method is used to find augmenting paths, and the ford_fulkerson
method implements the main algorithm.
Time Complexity Analysis
The time complexity of the Ford-Fulkerson algorithm depends on how the augmenting paths are chosen. In the worst case, where capacities are integers and poorly chosen augmenting paths are used, the algorithm can take O(E * max_flow) time, where E is the number of edges in the graph and max_flow is the maximum flow value.
However, when using Breadth-First Search to find augmenting paths (known as the Edmonds-Karp algorithm), the time complexity improves to O(V * E^2), where V is the number of vertices. This is because BFS tends to find shorter augmenting paths, leading to faster convergence.
Optimizations and Variations
While the Ford-Fulkerson algorithm is fundamental, several optimizations and variations have been developed to improve its performance:
1. Edmonds-Karp Algorithm
As mentioned earlier, this variation uses BFS to find augmenting paths, guaranteeing a polynomial time complexity of O(V * E^2).
2. Capacity Scaling
This technique involves initially considering only the edges with large capacities and gradually including edges with smaller capacities. It can improve the algorithm’s performance, especially on networks with a wide range of capacities.
3. Dinic’s Algorithm
Dinic’s algorithm is a strongly polynomial variant of Ford-Fulkerson that runs in O(V^2 * E) time. It uses the concept of blocking flows and level graphs to find augmenting paths more efficiently.
4. Push-Relabel Algorithm
This algorithm takes a different approach by working with preflows instead of flows and uses local operations to move flow through the network. It has a better theoretical time complexity of O(V^3) for the basic version, with more advanced implementations achieving even better performance.
Applications in Interview Questions
Maximum flow problems and the Ford-Fulkerson algorithm are popular topics in technical interviews, especially for positions at top tech companies. Here are some common types of questions you might encounter:
1. Direct Application
You might be asked to implement the Ford-Fulkerson algorithm or one of its variations. Be prepared to code the algorithm and explain its workings.
2. Problem Transformation
Many problems can be transformed into maximum flow problems. For example:
- Bipartite Matching: Finding the maximum number of job assignments or project allocations.
- Image Segmentation: Separating the foreground from the background in an image.
- Network Reliability: Determining the minimum number of edges to remove to disconnect a network.
3. Optimization Problems
You might be asked to solve optimization problems that can be modeled as flow networks, such as:
- Maximizing the throughput in a computer network
- Optimizing the distribution of resources in a supply chain
- Finding the maximum number of edge-disjoint paths between two vertices in a graph
4. Variations and Extensions
Interviewers might ask about variations of the maximum flow problem, such as:
- Minimum Cost Maximum Flow: Finding the maximum flow with the minimum total cost
- Multi-source Multi-sink Flow: Dealing with multiple sources and sinks in the network
- Maximum Flow over Time: Incorporating time constraints into the flow problem
Tips for Mastering Maximum Flow Problems
To excel in solving maximum flow problems and related interview questions, consider the following tips:
- Understand the Fundamentals: Make sure you have a solid grasp of graph theory, BFS, DFS, and the basic principles of flow networks.
- Practice Implementation: Implement the Ford-Fulkerson algorithm and its variations from scratch. This will help you understand the nuances of the algorithm and improve your coding skills.
- Recognize Problem Patterns: Learn to identify problems that can be transformed into maximum flow problems. This skill is crucial for tackling complex interview questions.
- Study Optimizations: Familiarize yourself with optimizations like the Edmonds-Karp algorithm and capacity scaling. Understanding these can give you an edge in optimizing solutions.
- Solve Diverse Problems: Practice solving a wide range of problems that use maximum flow as a building block. This will help you develop the intuition to apply the concept in various scenarios.
- Analyze Time and Space Complexity: Be prepared to discuss the time and space complexity of your solutions. Understanding the trade-offs between different approaches is important.
- Consider Edge Cases: When solving problems, always consider edge cases like empty graphs, disconnected components, or networks with cycles.
- Explain Your Approach: Practice explaining your thought process and approach to solving maximum flow problems. Clear communication is often as important as the solution itself in interviews.
Conclusion
The maximum flow problem and the Ford-Fulkerson algorithm are fundamental concepts in computer science and optimization. Mastering these topics can significantly boost your problem-solving skills and prepare you for technical interviews at top tech companies.
Remember that while understanding the algorithm is crucial, the real skill lies in recognizing when and how to apply it to solve diverse problems. As you practice, focus not just on implementation but also on problem transformation and optimization techniques.
Keep challenging yourself with increasingly complex problems, and don’t hesitate to explore advanced topics like minimum cost flow or multi-commodity flow. With dedication and practice, you’ll be well-equipped to tackle even the most challenging flow-related problems in your coding interviews and future software development career.
As you continue your journey in mastering algorithms and data structures, remember that platforms like AlgoCademy offer valuable resources, interactive tutorials, and AI-powered assistance to help you progress from basic coding skills to advanced algorithmic thinking. Leverage these tools to enhance your learning experience and prepare effectively for technical interviews at FAANG and other top tech companies.