KMP Algorithm: Mastering Efficient Pattern Matching in Strings
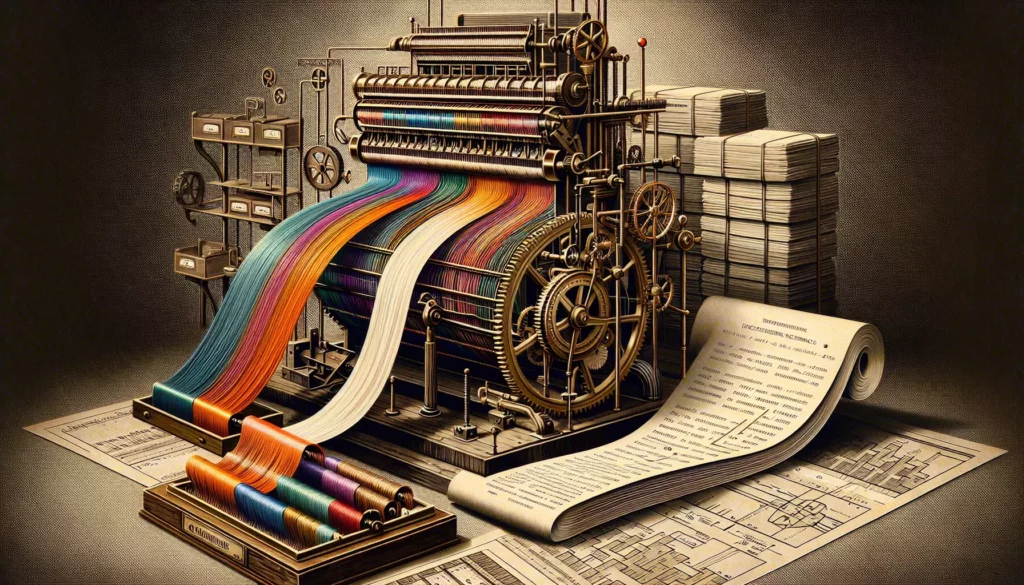
In the realm of computer science and algorithmic problem-solving, pattern matching in strings is a fundamental task with wide-ranging applications. From text editors to bioinformatics, the ability to efficiently locate a specific pattern within a larger text is crucial. Among the various algorithms designed for this purpose, the Knuth-Morris-Pratt (KMP) algorithm stands out as a powerful and elegant solution. In this comprehensive guide, we’ll dive deep into the KMP algorithm, exploring its inner workings, implementation, and practical applications.
Understanding the Need for Efficient Pattern Matching
Before we delve into the intricacies of the KMP algorithm, let’s consider why efficient pattern matching is so important in computer science and software development:
- Text Processing: In applications like word processors or code editors, fast pattern matching is essential for features such as find-and-replace or syntax highlighting.
- Bioinformatics: Analyzing DNA sequences often involves finding specific patterns within long genetic strings.
- Network Security: Intrusion detection systems may need to scan network traffic for specific patterns indicating potential threats.
- Data Analysis: Many data mining and analysis tasks involve searching for patterns within large datasets.
- Compiler Design: Lexical analysis in compilers relies on efficient pattern matching to identify tokens in source code.
Given these diverse applications, it’s clear that having a fast and reliable algorithm for pattern matching can significantly impact the performance and capabilities of many software systems.
The Naive Approach: Brute Force Pattern Matching
Before we explore the KMP algorithm, it’s helpful to understand the simplest approach to pattern matching: the brute force method. This naive algorithm works as follows:
- Align the pattern with the beginning of the text.
- Compare characters one by one from left to right.
- If a mismatch is found, shift the pattern one position to the right and start over.
- Repeat until the pattern is found or the end of the text is reached.
Here’s a simple implementation of the brute force approach in Python:
def brute_force_search(text, pattern):
n = len(text)
m = len(pattern)
for i in range(n - m + 1):
j = 0
while j < m and text[i + j] == pattern[j]:
j += 1
if j == m:
return i # Pattern found at index i
return -1 # Pattern not found
While this method is straightforward and works correctly, it can be inefficient for large texts or patterns. In the worst case, where the pattern almost matches at every position but fails at the last character, the time complexity can be O(nm), where n is the length of the text and m is the length of the pattern.
Enter the KMP Algorithm: A Smarter Approach
The Knuth-Morris-Pratt (KMP) algorithm, named after its inventors Donald Knuth, James H. Morris, and Vaughan Pratt, addresses the inefficiencies of the brute force approach. The key insight of KMP is to utilize the information gained from previous comparisons to avoid unnecessary re-comparisons.
The main advantages of the KMP algorithm are:
- It achieves a linear time complexity of O(n+m) in the worst case.
- It never needs to backtrack in the main text, always moving forward.
- It precomputes a “failure function” or “prefix function” that allows it to skip comparisons intelligently.
The Core Idea: Prefix Function
The heart of the KMP algorithm lies in its prefix function, also known as the failure function. This function encapsulates information about the pattern itself, specifically how the pattern matches against prefixes of itself. By precomputing this information, the algorithm can make intelligent decisions about where to continue matching after a mismatch occurs.
The prefix function π for a pattern P of length m is defined as an array where:
- Ï€[i] is the length of the longest proper prefix of P[0…i] which is also a suffix of P[0…i].
- A proper prefix is a prefix that is not equal to the entire string.
For example, consider the pattern “ABABC”:
- π[0] = 0 (no proper prefix for a single character)
- Ï€[1] = 0 (“A” has no proper prefix that’s also a suffix)
- Ï€[2] = 1 (“AB” has “A” as a proper prefix and suffix)
- Ï€[3] = 2 (“ABA” has “AB” as a proper prefix and suffix)
- Ï€[4] = 0 (“ABAB” has no proper prefix that’s also a suffix)
Computing the Prefix Function
The computation of the prefix function is a crucial step in the KMP algorithm. Here’s an efficient way to compute it:
def compute_prefix_function(pattern):
m = len(pattern)
pi = [0] * m
k = 0
for i in range(1, m):
while k > 0 and pattern[k] != pattern[i]:
k = pi[k - 1]
if pattern[k] == pattern[i]:
k += 1
pi[i] = k
return pi
This function builds the prefix function incrementally, using previously computed values to optimize the process. The time complexity of this computation is O(m), where m is the length of the pattern.
The KMP Algorithm in Action
Now that we have the prefix function, let’s see how the KMP algorithm uses it to perform efficient pattern matching:
def kmp_search(text, pattern):
n = len(text)
m = len(pattern)
pi = compute_prefix_function(pattern)
j = 0 # Index for pattern
for i in range(n):
while j > 0 and pattern[j] != text[i]:
j = pi[j - 1]
if pattern[j] == text[i]:
j += 1
if j == m:
return i - m + 1 # Pattern found at index i - m + 1
return -1 # Pattern not found
The algorithm works as follows:
- Precompute the prefix function for the pattern.
- Iterate through the text, comparing characters with the pattern.
- If a mismatch occurs, use the prefix function to determine where to continue matching in the pattern.
- If a full match is found, return the starting index of the match.
The key advantage here is that the algorithm never backtracks in the text. It always moves forward, using the information from the prefix function to skip unnecessary comparisons.
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of the KMP algorithm:
Time Complexity
- Prefix Function Computation: O(m), where m is the length of the pattern.
- Main Search Algorithm: O(n), where n is the length of the text.
- Overall Time Complexity: O(n + m)
The linear time complexity is a significant improvement over the quadratic worst-case of the brute force approach, especially for large texts and patterns.
Space Complexity
- Prefix Function Array: O(m)
- Additional Variables: O(1)
- Overall Space Complexity: O(m)
The space complexity is linear in the length of the pattern, which is typically much smaller than the text.
Practical Applications and Examples
The KMP algorithm finds applications in various domains. Let’s explore some practical scenarios where it can be effectively used:
1. Text Editors and Search Functionality
In text editors, the KMP algorithm can be used to implement efficient “find” or “search” functionality. Here’s a simple example of how it might be used:
def highlight_occurrences(text, pattern):
occurrences = []
start = 0
while True:
index = kmp_search(text[start:], pattern)
if index == -1:
break
occurrences.append(start + index)
start += index + 1
return occurrences
# Example usage
text = "The quick brown fox jumps over the lazy dog. The quick brown fox jumps over the lazy dog."
pattern = "quick brown"
highlights = highlight_occurrences(text, pattern)
print(f"Pattern '{pattern}' found at indices: {highlights}")
2. DNA Sequence Analysis
In bioinformatics, the KMP algorithm can be used to search for specific DNA sequences within a larger genome. For instance:
def find_dna_sequence(genome, sequence):
index = kmp_search(genome, sequence)
if index != -1:
print(f"Sequence found at position {index}")
else:
print("Sequence not found in the genome")
# Example usage
genome = "ATCGAATCGTAGCTAGCTAGCTAGCTAGCTAGCTAGC"
sequence = "CTAGCTAG"
find_dna_sequence(genome, sequence)
3. Network Intrusion Detection
In cybersecurity, the KMP algorithm can be used to scan network packets for specific patterns that might indicate malicious activity:
def scan_packet(packet, malicious_patterns):
for pattern in malicious_patterns:
if kmp_search(packet, pattern) != -1:
print(f"Warning: Malicious pattern '{pattern}' detected in packet!")
return True
return False
# Example usage
packet = "GET /admin/login.php?username=admin&password=123456 HTTP/1.1"
malicious_patterns = ["admin", "password=", "union select"]
is_malicious = scan_packet(packet, malicious_patterns)
Optimizations and Variations
While the KMP algorithm is already efficient, there are some optimizations and variations worth considering:
1. Boyer-Moore Algorithm
The Boyer-Moore algorithm is another string matching algorithm that can be even faster than KMP in practice, especially for larger alphabets. It works by scanning the characters of the pattern from right to left and can skip large portions of the text.
2. Aho-Corasick Algorithm
For matching multiple patterns simultaneously, the Aho-Corasick algorithm, which builds upon ideas similar to KMP, can be more efficient. It’s particularly useful in applications like virus scanning or multi-pattern search in text.
3. Bit-parallel Algorithms
For short patterns, bit-parallel algorithms like the Shift-Or algorithm can leverage the bitwise operations of modern processors to achieve very fast matching, especially for patterns up to the word size of the machine.
Common Pitfalls and Best Practices
When implementing or using the KMP algorithm, keep these points in mind:
- Correct Prefix Function: Ensure that the prefix function is computed correctly, as it’s the core of the algorithm’s efficiency.
- Handling Empty Patterns: Be sure to handle the case of an empty pattern or text appropriately in your implementation.
- Unicode and Multi-byte Characters: If working with Unicode strings, ensure your implementation correctly handles multi-byte characters.
- Memory Efficiency: For very long patterns, consider if the space used by the prefix function is acceptable for your application.
- Testing: Thoroughly test your implementation with various edge cases, including patterns that are longer than the text, patterns with repeating substrings, and texts with multiple occurrences of the pattern.
Conclusion
The Knuth-Morris-Pratt (KMP) algorithm represents a significant advancement in the field of string matching. Its clever use of pattern information to avoid unnecessary comparisons makes it a powerful tool in various applications, from text processing to bioinformatics.
By understanding and implementing the KMP algorithm, you’re not just learning a specific technique, but also gaining insights into broader concepts of algorithm design and optimization. The ideas behind KMP—such as precomputation and utilizing previously gained information—are applicable in many other areas of computer science and software development.
As you continue your journey in algorithmic problem-solving, remember that KMP is just one tool in your arsenal. The key is to understand its strengths, limitations, and when to apply it. With this knowledge, you’ll be better equipped to tackle complex string matching problems and optimize your code for performance and efficiency.
Keep practicing, experimenting with different scenarios, and don’t hesitate to explore variations and alternatives. The world of algorithms is vast and fascinating, and mastering techniques like KMP will undoubtedly make you a more skilled and versatile programmer.