Mastering Eulerian Paths and Circuits: A Comprehensive Guide for Coding Interviews
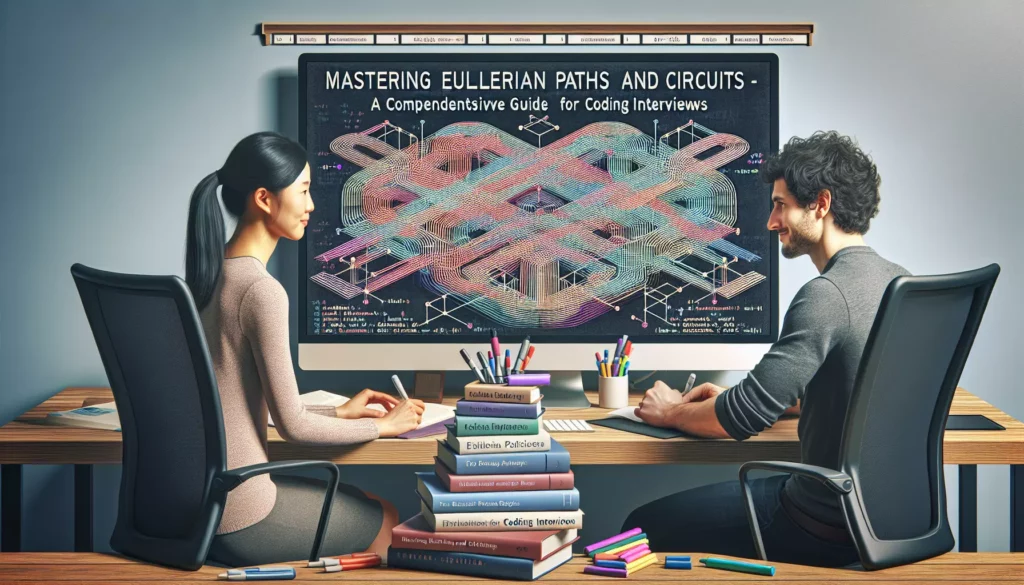
In the world of computer science and graph theory, Eulerian paths and circuits play a crucial role in solving various real-world problems. As aspiring software engineers prepare for coding interviews, particularly those targeting major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding these concepts is essential. This comprehensive guide will dive deep into Eulerian paths and circuits, exploring their definitions, properties, applications, and implementation in code.
What are Eulerian Paths and Circuits?
Before we delve into the intricacies of Eulerian paths and circuits, let’s start with their basic definitions:
- Eulerian Path: A path in a graph that visits every edge exactly once.
- Eulerian Circuit: A circuit (closed path) in a graph that visits every edge exactly once and returns to the starting vertex.
These concepts are named after the famous mathematician Leonhard Euler, who solved the Seven Bridges of Königsberg problem in 1736. This problem laid the foundation for graph theory and the study of Eulerian paths and circuits.
Properties of Eulerian Paths and Circuits
To determine whether a graph has an Eulerian path or circuit, we need to consider the following properties:
For Undirected Graphs:
- Eulerian Circuit: All vertices in the graph must have an even degree (number of edges connected to the vertex).
- Eulerian Path: Either all vertices have an even degree, or exactly two vertices have an odd degree (these will be the start and end vertices of the path).
For Directed Graphs:
- Eulerian Circuit: All vertices must have equal in-degree and out-degree, and the graph must be strongly connected.
- Eulerian Path: At most one vertex can have (out-degree) – (in-degree) = 1, and at most one vertex can have (in-degree) – (out-degree) = 1. All other vertices must have equal in-degree and out-degree. The graph must also be weakly connected.
Applications of Eulerian Paths and Circuits
Eulerian paths and circuits have numerous practical applications in computer science and real-world scenarios. Some notable examples include:
- DNA Fragment Assembly: In bioinformatics, Eulerian paths are used to reconstruct DNA sequences from smaller fragments.
- Circuit Design: Eulerian circuits are useful in designing efficient circuit board layouts and minimizing wire crossings.
- Network Flow Optimization: In transportation and logistics, Eulerian paths help optimize routes for delivery vehicles or data packets in computer networks.
- Chinese Postman Problem: This problem involves finding the shortest route that covers all streets in a city, which is essentially finding an Eulerian circuit or path in a graph.
- Puzzle Solving: Many puzzle games, such as drawing figures without lifting the pen or tracing paths through mazes, are based on Eulerian paths and circuits.
Implementing Eulerian Path and Circuit Algorithms
Now that we understand the concepts and their applications, let’s dive into implementing algorithms to find Eulerian paths and circuits. We’ll use Python for our examples, but the concepts can be applied to any programming language.
1. Checking if a Graph has an Eulerian Circuit or Path
First, let’s implement a function to check if an undirected graph has an Eulerian circuit or path:
from collections import defaultdict
def check_eulerian(graph):
odd_degree_count = 0
for vertex in graph:
if len(graph[vertex]) % 2 != 0:
odd_degree_count += 1
if odd_degree_count == 0:
return "Eulerian Circuit"
elif odd_degree_count == 2:
return "Eulerian Path"
else:
return "Not Eulerian"
# Example usage
graph = defaultdict(list)
graph[0] = [1, 2, 3]
graph[1] = [0, 2]
graph[2] = [0, 1, 3]
graph[3] = [0, 2]
print(check_eulerian(graph)) # Output: Eulerian Circuit
This function counts the number of vertices with odd degrees. If there are no odd-degree vertices, the graph has an Eulerian circuit. If there are exactly two odd-degree vertices, it has an Eulerian path. Otherwise, it’s not Eulerian.
2. Finding an Eulerian Circuit
Now, let’s implement Hierholzer’s algorithm to find an Eulerian circuit in a graph:
def find_eulerian_circuit(graph):
# Find a vertex with non-zero degree
start_vertex = next(iter(graph))
for v in graph:
if len(graph[v]) % 2 != 0:
start_vertex = v
break
stack = [start_vertex]
circuit = []
while stack:
v = stack[-1]
if graph[v]:
u = graph[v].pop()
stack.append(u)
graph[u].remove(v)
else:
circuit.append(stack.pop())
return circuit[::-1]
# Example usage
graph = defaultdict(list)
graph[0] = [1, 2, 3]
graph[1] = [0, 2]
graph[2] = [0, 1, 3]
graph[3] = [0, 2]
circuit = find_eulerian_circuit(graph)
print(" -> ".join(map(str, circuit))) # Output: 0 -> 3 -> 2 -> 1 -> 2 -> 0
This algorithm works by starting at any vertex and following unused edges until we get stuck. When we get stuck, we add the current vertex to the circuit and backtrack. The process continues until all edges are used.
3. Finding an Eulerian Path
To find an Eulerian path, we can modify the Eulerian circuit algorithm slightly:
def find_eulerian_path(graph):
# Find start and end vertices
start_vertex = end_vertex = next(iter(graph))
for v in graph:
if len(graph[v]) % 2 != 0:
if len(graph[v]) > len(graph[start_vertex]):
start_vertex = v
else:
end_vertex = v
# Add an edge between start and end vertices if they're different
if start_vertex != end_vertex:
graph[start_vertex].append(end_vertex)
graph[end_vertex].append(start_vertex)
# Find Eulerian circuit
circuit = find_eulerian_circuit(graph)
# Remove the added edge if necessary
if start_vertex != end_vertex:
circuit.pop()
return circuit
# Example usage
graph = defaultdict(list)
graph[0] = [1, 2]
graph[1] = [0, 2, 3]
graph[2] = [0, 1, 4]
graph[3] = [1, 4]
graph[4] = [2, 3]
path = find_eulerian_path(graph)
print(" -> ".join(map(str, path))) # Output: 0 -> 2 -> 4 -> 3 -> 1 -> 2 -> 0
This algorithm first identifies the start and end vertices (if they exist), adds a temporary edge between them to create an Eulerian circuit, finds the circuit, and then removes the temporary edge to obtain the Eulerian path.
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our Eulerian path and circuit algorithms:
Time Complexity:
- Checking for Eulerian Circuit/Path: O(V + E), where V is the number of vertices and E is the number of edges.
- Finding Eulerian Circuit/Path: O(E), as we visit each edge exactly once.
Space Complexity:
- Checking for Eulerian Circuit/Path: O(V) to store the graph representation.
- Finding Eulerian Circuit/Path: O(E) to store the circuit/path and the stack used in the algorithm.
These efficient complexities make Eulerian path and circuit algorithms suitable for large-scale graph problems.
Common Interview Questions and Variations
When preparing for coding interviews, especially for FAANG companies, it’s essential to be familiar with variations and related problems involving Eulerian paths and circuits. Here are some common questions and variations you might encounter:
- Reconstruct Itinerary: Given a list of airline tickets with [from, to] routes, find the itinerary that uses all tickets exactly once, starting from a specific airport.
- Valid Arrangement of Pairs: Given pairs of integers, find a valid arrangement where each pair appears exactly once, and consecutive integers in the arrangement belong to a pair.
- Cracking the Safe: Find the shortest string that contains all possible n-length combinations of k digits as substrings.
- Minimum Number of Edges to Add: Given a graph, find the minimum number of edges to add to make it Eulerian.
- De Bruijn Sequence: Generate a cyclic sequence of a given alphabet, such that every possible substring of length n appears exactly once.
Tips for Solving Eulerian Path and Circuit Problems
To excel in coding interviews and effectively solve problems related to Eulerian paths and circuits, consider the following tips:
- Visualize the Graph: Draw the graph on paper or a whiteboard to better understand its structure and properties.
- Check Degree Conditions: Always start by verifying if the graph satisfies the necessary conditions for an Eulerian path or circuit.
- Consider Edge Cases: Think about special cases, such as empty graphs, disconnected graphs, or graphs with self-loops.
- Implement Helper Functions: Break down the problem into smaller functions, such as checking connectivity or counting degrees, to make your code more modular and easier to debug.
- Practice Different Representations: Be comfortable working with various graph representations (adjacency list, adjacency matrix, edge list) as the input format may vary.
- Optimize for Space: In some cases, you may need to modify the graph in-place to achieve better space complexity. Be prepared to discuss trade-offs between time and space.
- Understand Related Concepts: Familiarize yourself with related graph concepts like DFS, BFS, and connectivity, as they often come in handy when solving Eulerian path problems.
Conclusion
Mastering Eulerian paths and circuits is crucial for aspiring software engineers, especially those aiming for positions at top tech companies. These concepts not only form the basis for solving complex graph problems but also have practical applications in various fields, from bioinformatics to network optimization.
By understanding the properties of Eulerian paths and circuits, implementing efficient algorithms, and practicing related problems, you’ll be well-prepared to tackle graph theory questions in coding interviews. Remember to approach each problem systematically, consider edge cases, and optimize your solutions for both time and space complexity.
As you continue your journey in coding education and skill development, platforms like AlgoCademy can provide valuable resources, interactive tutorials, and AI-powered assistance to help you master these concepts and prepare for technical interviews. Keep practicing, stay curious, and don’t hesitate to explore the fascinating world of graph theory beyond Eulerian paths and circuits.