Kosaraju’s Algorithm: Unraveling Strongly Connected Components in Graphs
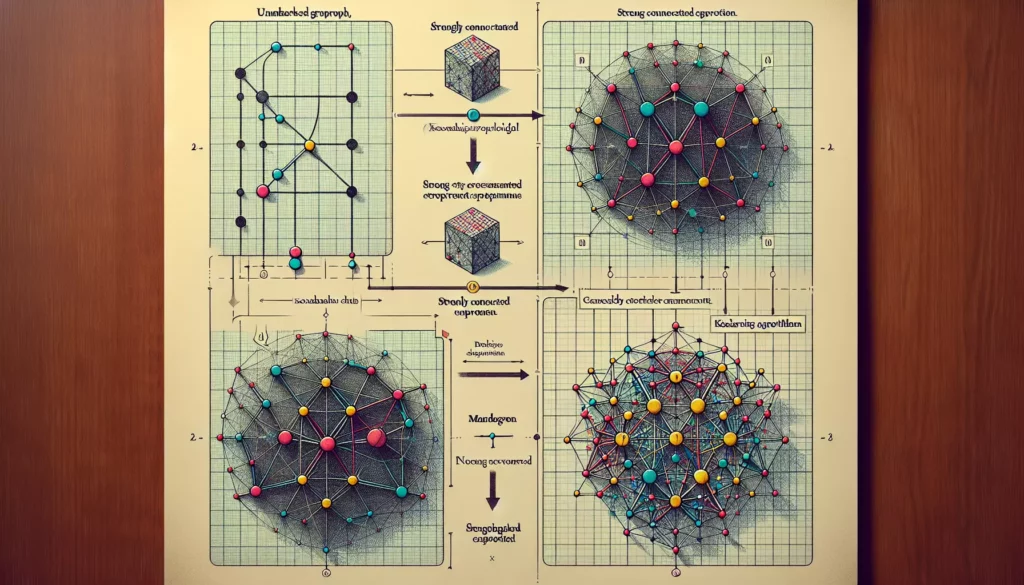
In the vast realm of graph theory and algorithms, Kosaraju’s algorithm stands out as a powerful tool for analyzing the structure of directed graphs. This algorithm, named after its creator S. Rao Kosaraju, is designed to find strongly connected components (SCCs) in a directed graph. Understanding Kosaraju’s algorithm is crucial for anyone delving into advanced graph algorithms, especially those preparing for technical interviews at major tech companies.
What are Strongly Connected Components?
Before we dive into the intricacies of Kosaraju’s algorithm, let’s first understand what strongly connected components are. In a directed graph, a strongly connected component is a subset of vertices where every vertex is reachable from every other vertex in that subset. In other words, for any two vertices u and v in an SCC, there exists a path from u to v and a path from v to u.
Strongly connected components have several important applications in computer science and real-world scenarios, including:
- Social network analysis
- Web page ranking algorithms
- Bioinformatics
- Compiler optimization
The Essence of Kosaraju’s Algorithm
Kosaraju’s algorithm is an elegant and efficient method to find all strongly connected components in a directed graph. The algorithm works in three main steps:
- Perform a depth-first search (DFS) on the original graph and keep track of the finish times of vertices.
- Create the transpose of the graph (reverse all edge directions).
- Perform another DFS on the transposed graph, starting with the vertex that finished last in the first DFS.
The beauty of this algorithm lies in its simplicity and linear time complexity of O(V + E), where V is the number of vertices and E is the number of edges in the graph.
Step-by-Step Explanation of Kosaraju’s Algorithm
Step 1: First Depth-First Search
The first step involves performing a depth-first search on the original graph. During this DFS, we keep track of the finish times of each vertex. The finish time of a vertex is the time when the DFS completes exploring all its neighbors and backtracks.
Here’s a Python implementation of the first DFS:
def dfs_first(graph, v, visited, stack):
visited[v] = True
for neighbor in graph[v]:
if not visited[neighbor]:
dfs_first(graph, neighbor, visited, stack)
stack.append(v)
def kosaraju_step1(graph):
visited = [False] * len(graph)
stack = []
for v in range(len(graph)):
if not visited[v]:
dfs_first(graph, v, visited, stack)
return stack
In this step, we use a stack to keep track of the vertices in the order of their finish times. The vertex that finishes last will be at the top of the stack.
Step 2: Transpose the Graph
The second step involves creating the transpose of the original graph. In the transposed graph, all edge directions are reversed. This step is crucial because it helps us identify the strongly connected components.
Here’s how we can implement the graph transposition:
def transpose_graph(graph):
transposed = [[] for _ in range(len(graph))]
for v in range(len(graph)):
for neighbor in graph[v]:
transposed[neighbor].append(v)
return transposed
Step 3: Second Depth-First Search
The final step involves performing another depth-first search on the transposed graph. We start with the vertex that finished last in the first DFS (top of the stack) and continue with the next vertices in the stack order. Each DFS tree formed in this step represents a strongly connected component.
Here’s the implementation of the second DFS:
def dfs_second(graph, v, visited, component):
visited[v] = True
component.append(v)
for neighbor in graph[v]:
if not visited[neighbor]:
dfs_second(graph, neighbor, visited, component)
def kosaraju_step3(graph, stack):
visited = [False] * len(graph)
sccs = []
while stack:
v = stack.pop()
if not visited[v]:
component = []
dfs_second(graph, v, visited, component)
sccs.append(component)
return sccs
Putting It All Together: The Complete Kosaraju’s Algorithm
Now that we’ve explored each step in detail, let’s combine them to create the complete Kosaraju’s algorithm:
def kosaraju(graph):
# Step 1: First DFS
stack = kosaraju_step1(graph)
# Step 2: Transpose the graph
transposed_graph = transpose_graph(graph)
# Step 3: Second DFS
sccs = kosaraju_step3(transposed_graph, stack)
return sccs
# Example usage
graph = [
[1, 2],
[2, 3],
[3, 1],
[3, 4],
[4, 5],
[5, 4]
]
strongly_connected_components = kosaraju(graph)
print("Strongly Connected Components:", strongly_connected_components)
This implementation will find all strongly connected components in the given graph and return them as a list of lists, where each inner list represents a strongly connected component.
Time and Space Complexity Analysis
One of the remarkable aspects of Kosaraju’s algorithm is its efficiency. Let’s analyze its time and space complexity:
Time Complexity
The time complexity of Kosaraju’s algorithm is O(V + E), where V is the number of vertices and E is the number of edges in the graph. This linear time complexity is achieved because:
- The first DFS takes O(V + E) time to visit all vertices and edges.
- Transposing the graph takes O(V + E) time to reverse all edges.
- The second DFS also takes O(V + E) time to visit all vertices and edges in the transposed graph.
Since these steps are performed sequentially, the overall time complexity remains O(V + E).
Space Complexity
The space complexity of Kosaraju’s algorithm is O(V), where V is the number of vertices. This space is used for:
- The visited array in both DFS passes: O(V)
- The stack to store vertices: O(V)
- The transposed graph: O(V + E), but since we’re dealing with the adjacency list representation, it’s effectively O(V)
The space complexity remains linear with respect to the number of vertices, making Kosaraju’s algorithm space-efficient as well.
Applications and Importance in Technical Interviews
Understanding and implementing Kosaraju’s algorithm is crucial for several reasons, especially when preparing for technical interviews at major tech companies:
- Graph Analysis: It demonstrates a deep understanding of graph theory and algorithms, which is fundamental in many areas of computer science.
- Problem-Solving Skills: The algorithm showcases the ability to break down complex problems into manageable steps and implement efficient solutions.
- Optimization Techniques: Kosaraju’s algorithm is an excellent example of how clever algorithmic techniques can achieve linear time complexity for seemingly complex problems.
- Real-World Applications: Strongly connected components have practical applications in various domains, making this algorithm relevant for solving real-world problems.
- Interview Favorites: Graph algorithms, including those for finding SCCs, are popular topics in technical interviews at companies like Google, Facebook, and Amazon.
Common Interview Questions and Variations
When preparing for technical interviews, it’s essential to be familiar with common questions and variations related to Kosaraju’s algorithm and strongly connected components. Here are some examples:
- Implement Kosaraju’s algorithm to find all strongly connected components in a given graph.
- How would you modify Kosaraju’s algorithm to count the number of SCCs without explicitly storing them?
- Given a directed graph, determine if it is strongly connected (i.e., has only one SCC).
- Find the largest strongly connected component in a directed graph.
- Implement an algorithm to check if removing a given edge would increase the number of SCCs in the graph.
To tackle these questions effectively, it’s crucial to have a solid understanding of Kosaraju’s algorithm and be able to adapt it to different scenarios.
Best Practices for Implementing Kosaraju’s Algorithm
When implementing Kosaraju’s algorithm, keep these best practices in mind:
- Use Adjacency List: Represent the graph using an adjacency list for better space efficiency and faster traversal.
- Optimize DFS: Implement an iterative DFS if you’re concerned about stack overflow for very large graphs.
- Handle Edge Cases: Consider empty graphs, single-node graphs, and graphs with self-loops.
- Use Meaningful Variable Names: Clear naming conventions make your code more readable and maintainable.
- Add Comments: Explain the purpose of each step in the algorithm to demonstrate your understanding.
Common Pitfalls and How to Avoid Them
When working with Kosaraju’s algorithm, be aware of these common pitfalls:
- Forgetting to Reset Visited Array: Remember to reset the visited array before the second DFS.
- Incorrect Graph Transposition: Ensure that all edges are correctly reversed in the transposed graph.
- Misunderstanding Finish Times: The order of vertices in the stack after the first DFS is crucial for the algorithm’s correctness.
- Ignoring Disconnected Graphs: Make sure your implementation handles graphs that are not fully connected.
- Overlooking Memory Management: In languages without automatic garbage collection, be mindful of memory allocation and deallocation.
Advanced Topics and Further Exploration
For those looking to deepen their understanding of strongly connected components and related concepts, consider exploring these advanced topics:
- Tarjan’s Algorithm: An alternative algorithm for finding SCCs in a single DFS pass.
- 2-SAT Problem: Using SCCs to solve the 2-Satisfiability problem efficiently.
- Condensation Graph: Creating a DAG by contracting each SCC into a single vertex.
- Applications in Compiler Design: Using SCCs for data flow analysis and optimization.
- Parallel Implementations: Exploring ways to parallelize Kosaraju’s algorithm for large-scale graphs.
Conclusion: Mastering Kosaraju’s Algorithm for Interview Success
Kosaraju’s algorithm is a powerful tool in the graph algorithm toolkit, essential for anyone pursuing a career in software engineering or computer science. Its elegance lies in its simplicity and efficiency, making it a favorite topic in technical interviews at top tech companies.
By thoroughly understanding Kosaraju’s algorithm, you demonstrate not only your grasp of graph theory but also your ability to implement efficient solutions to complex problems. This knowledge sets you apart as a candidate who can tackle challenging algorithmic problems and apply theoretical concepts to practical scenarios.
As you prepare for technical interviews, remember that mastering Kosaraju’s algorithm is more than just memorizing steps. It’s about understanding the underlying principles, recognizing its applications, and being able to adapt the algorithm to solve various graph-related problems. With practice and a deep understanding of this algorithm, you’ll be well-equipped to tackle even the most challenging graph problems in your technical interviews and future software engineering career.
Keep exploring, keep practicing, and let Kosaraju’s algorithm be your gateway to mastering advanced graph algorithms and acing your technical interviews at top tech companies!