Tarjan’s Algorithm: Unraveling Strongly Connected Components in Graphs
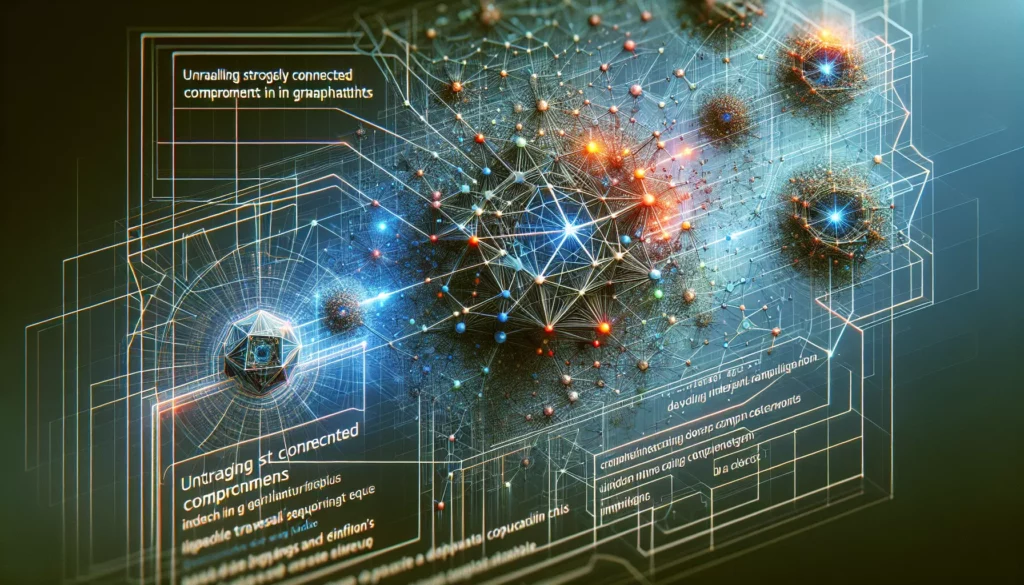
In the vast realm of graph theory and algorithms, Tarjan’s algorithm stands out as a powerful tool for analyzing the structure of directed graphs. Named after its creator, Robert Tarjan, this algorithm efficiently identifies strongly connected components (SCCs) within a graph. Understanding Tarjan’s algorithm is crucial for aspiring programmers and computer scientists, as it has numerous applications in various fields, including social network analysis, compiler optimization, and scientific computing.
In this comprehensive guide, we’ll dive deep into Tarjan’s algorithm, exploring its inner workings, implementation, and real-world applications. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your algorithmic problem-solving skills, mastering Tarjan’s algorithm will undoubtedly be a valuable addition to your coding repertoire.
What are Strongly Connected Components?
Before we delve into the intricacies of Tarjan’s algorithm, it’s essential to understand the concept of strongly connected components. In a directed graph, a strongly connected component (SCC) is a subgraph where every vertex is reachable from every other vertex within that subgraph. In other words, for any two vertices u and v in an SCC, there exists a path from u to v and a path from v to u.
Identifying SCCs is crucial in many graph-related problems, as it allows us to simplify complex graphs by condensing strongly connected regions into single nodes. This simplification can lead to more efficient algorithms and better insights into the overall structure of the graph.
The Basics of Tarjan’s Algorithm
Tarjan’s algorithm is a depth-first search (DFS) based approach that identifies all SCCs in a directed graph in linear time complexity, O(V + E), where V is the number of vertices and E is the number of edges. The algorithm’s efficiency stems from its ability to identify SCCs in a single pass through the graph, unlike other algorithms that may require multiple passes.
The key ideas behind Tarjan’s algorithm are:
- Perform a depth-first search traversal of the graph.
- Assign each vertex two values: a unique index and a lowlink value.
- Use a stack to keep track of vertices in the current SCC being explored.
- Identify SCCs when a vertex’s index equals its lowlink value.
How Tarjan’s Algorithm Works
Let’s break down the algorithm step by step:
-
Initialization:
- Create an empty stack to store vertices.
- Initialize arrays to store the index, lowlink, and on-stack status for each vertex.
- Set a global index counter to 0.
-
Depth-First Search:
- For each unvisited vertex, call the DFS function.
- In the DFS function:
- Assign the current index to the vertex and increment the index counter.
- Set the lowlink value equal to the index.
- Push the vertex onto the stack and mark it as on-stack.
- Explore all adjacent vertices.
-
Lowlink Update:
- For each adjacent vertex v of the current vertex u:
- If v is unvisited, recursively call DFS on v and update u’s lowlink.
- If v is on the stack, update u’s lowlink to the minimum of its current lowlink and v’s index.
- For each adjacent vertex v of the current vertex u:
-
SCC Identification:
- If a vertex’s index equals its lowlink value, it’s the root of an SCC.
- Pop vertices from the stack until the root vertex is reached, forming an SCC.
Implementing Tarjan’s Algorithm
Now that we understand the algorithm conceptually, let’s implement it in Python. This implementation will help solidify our understanding and provide a practical tool for solving graph-related problems.
from typing import List, Dict, Set
class TarjanSCC:
def __init__(self, graph: Dict[int, List[int]]):
self.graph = graph
self.index = 0
self.stack = []
self.on_stack = set()
self.indexes = {}
self.lowlinks = {}
self.sccs = []
def tarjan(self) -> List[List[int]]:
for node in self.graph:
if node not in self.indexes:
self.strong_connect(node)
return self.sccs
def strong_connect(self, node: int):
# Set the depth index for node to the smallest unused index
self.indexes[node] = self.index
self.lowlinks[node] = self.index
self.index += 1
self.stack.append(node)
self.on_stack.add(node)
# Consider successors of node
for successor in self.graph[node]:
if successor not in self.indexes:
# Successor has not yet been visited; recurse on it
self.strong_connect(successor)
self.lowlinks[node] = min(self.lowlinks[node], self.lowlinks[successor])
elif successor in self.on_stack:
# Successor is in stack and hence in the current SCC
self.lowlinks[node] = min(self.lowlinks[node], self.indexes[successor])
# If node is a root node, pop the stack and generate an SCC
if self.lowlinks[node] == self.indexes[node]:
scc = []
while True:
successor = self.stack.pop()
self.on_stack.remove(successor)
scc.append(successor)
if successor == node:
break
self.sccs.append(scc)
# Example usage
graph = {
0: [1, 3],
1: [2],
2: [1],
3: [4],
4: [5],
5: [3, 6],
6: []
}
tarjan = TarjanSCC(graph)
strongly_connected_components = tarjan.tarjan()
print("Strongly Connected Components:", strongly_connected_components)
This implementation creates a TarjanSCC
class that encapsulates the algorithm’s logic. The tarjan
method is the entry point, which iterates through all nodes in the graph and calls strong_connect
for each unvisited node. The strong_connect
method performs the depth-first search and identifies the SCCs.
Time and Space Complexity Analysis
Tarjan’s algorithm is remarkably efficient, with the following complexity characteristics:
- Time Complexity: O(V + E), where V is the number of vertices and E is the number of edges in the graph. This linear time complexity is achieved because each vertex and edge is visited exactly once during the depth-first search.
- Space Complexity: O(V), where V is the number of vertices. This space is used for the stack, the index and lowlink arrays, and the recursion stack (which in the worst case can be as deep as the number of vertices).
The efficiency of Tarjan’s algorithm makes it an excellent choice for large graphs, outperforming many other SCC-finding algorithms in practice.
Applications of Tarjan’s Algorithm
Tarjan’s algorithm for finding strongly connected components has numerous practical applications across various domains of computer science and beyond. Some notable applications include:
-
Social Network Analysis:
In social networks, SCCs can represent closely-knit communities or groups of users with strong mutual interactions. Identifying these components can help in community detection, influence analysis, and targeted advertising.
-
Compiler Optimization:
In static analysis of programs, SCCs in the control flow graph can represent loops. Identifying these components helps in loop optimization techniques, such as code motion and strength reduction.
-
Scientific Computing:
In numerical analysis, SCCs can be used to identify cyclic dependencies in systems of equations, which is crucial for choosing appropriate solving methods.
-
Web Crawling and Search Engines:
SCCs in the web graph can represent sets of pages that link to each other. This information is valuable for indexing and ranking algorithms used by search engines.
-
Biological Network Analysis:
In bioinformatics, SCCs in protein interaction networks or gene regulatory networks can represent functional modules or feedback loops in biological systems.
-
Transportation and Logistics:
In transportation networks, SCCs can help identify regions with strong interconnectivity, which is useful for route planning and network optimization.
Common Interview Questions and Variations
When preparing for technical interviews, especially for positions at top tech companies, it’s essential to be familiar with Tarjan’s algorithm and its variations. Here are some common questions and problem variations you might encounter:
-
Implement Tarjan’s algorithm to find all SCCs in a given graph.
This is the most straightforward application of the algorithm. Be prepared to implement it from scratch and explain its working.
-
Find the number of SCCs in a graph.
A slight variation where you only need to count the SCCs without necessarily storing them.
-
Determine if a graph is strongly connected.
Use Tarjan’s algorithm to check if the entire graph forms a single SCC.
-
Find the size of the largest SCC in a graph.
Modify the algorithm to keep track of the size of each SCC and return the maximum.
-
Detect cycles in a directed graph.
Any SCC with more than one vertex contains a cycle. Modify Tarjan’s algorithm to report the presence of such SCCs.
-
Compute the condensation graph (meta-graph of SCCs).
After finding SCCs, create a new graph where each SCC is represented by a single node.
-
Find articulation points or bridges in an undirected graph.
While not directly related to SCCs, these problems use similar DFS-based techniques and are often asked alongside Tarjan’s algorithm.
Tips for Mastering Tarjan’s Algorithm
To truly master Tarjan’s algorithm and excel in related interview questions, consider the following tips:
-
Understand the underlying concepts:
Make sure you have a solid grasp of depth-first search, graph theory basics, and the definition of strongly connected components.
-
Practice implementation:
Implement the algorithm from scratch multiple times until you can do it confidently without referring to notes.
-
Visualize the process:
Draw out small graphs and step through the algorithm manually to understand how the index and lowlink values change during execution.
-
Analyze different graph structures:
Practice applying the algorithm to various graph types, including cyclic, acyclic, and disconnected graphs.
-
Optimize your code:
Look for ways to improve the efficiency of your implementation, such as using appropriate data structures and minimizing unnecessary operations.
-
Solve related problems:
Work on problems that involve finding cycles, topological sorting, or other graph traversal techniques to broaden your understanding of graph algorithms.
-
Explain your approach:
Practice explaining the algorithm and your implementation choices, as this is often required in technical interviews.
Conclusion
Tarjan’s algorithm for finding strongly connected components is a powerful tool in the arsenal of any proficient programmer or computer scientist. Its elegance lies in its ability to solve a complex graph problem with linear time complexity, making it invaluable for large-scale applications.
By mastering Tarjan’s algorithm, you not only enhance your graph manipulation skills but also gain insights into efficient algorithm design and depth-first search techniques. These skills are highly valued in technical interviews, especially at top tech companies where complex problem-solving abilities are crucial.
As you continue your journey in algorithm mastery, remember that understanding the theoretical foundations and practicing implementation go hand in hand. Tarjan’s algorithm serves as an excellent example of how deep comprehension of a problem can lead to elegant and efficient solutions.
Keep exploring, keep practicing, and don’t hesitate to apply Tarjan’s algorithm to real-world problems. The more you engage with these concepts, the better prepared you’ll be for technical interviews and the challenges of software development in the industry.
Happy coding, and may your graphs always be strongly connected!