A* Search Algorithm: The Pathfinding Powerhouse in Computer Science
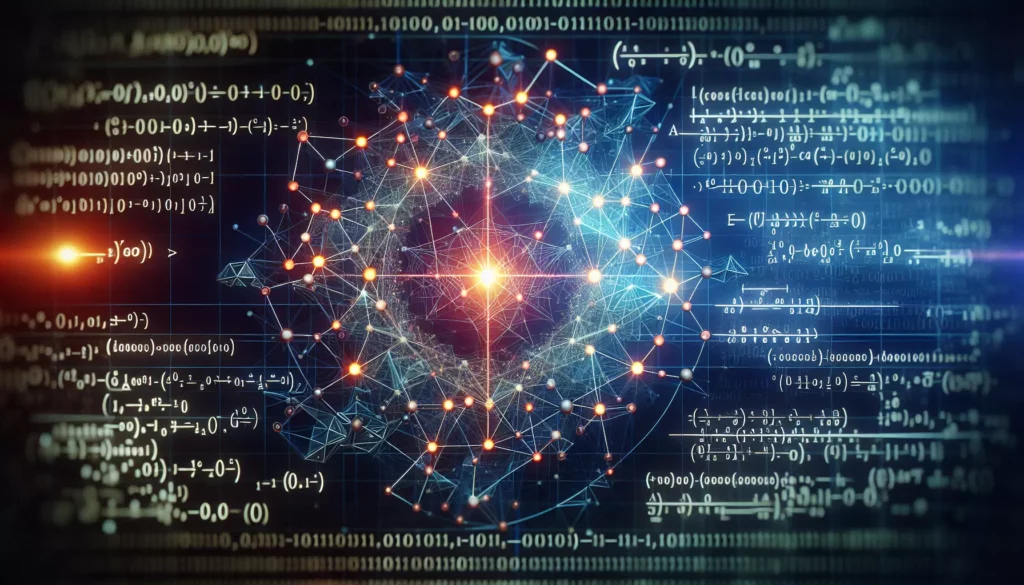
In the vast landscape of computer science and artificial intelligence, few algorithms have gained as much prominence and practical application as the A* (pronounced “A-star”) search algorithm. Whether you’re a budding programmer, an aspiring game developer, or simply curious about the inner workings of navigation systems, understanding A* is crucial. This powerful pathfinding algorithm combines the best of breadth-first search and Dijkstra’s algorithm, making it an essential tool in various domains, from robotics to video games.
In this comprehensive guide, we’ll dive deep into the A* search algorithm, exploring its origins, mechanics, implementation, and real-world applications. By the end of this article, you’ll have a solid grasp of how A* works and why it’s such a valuable asset in a programmer’s toolkit.
Table of Contents
- Introduction to A* Search
- How A* Works: The Core Mechanics
- Key Components of A*
- Implementing A* in Code
- Optimizations and Variations
- Real-World Applications
- Comparing A* to Other Pathfinding Algorithms
- Challenges and Limitations
- The Future of A* and Pathfinding
- Conclusion
1. Introduction to A* Search
The A* search algorithm, first described by Peter Hart, Nils Nilsson, and Bertram Raphael in 1968, is an extension of Edsger Dijkstra’s 1959 algorithm. A* is designed to find the shortest path between two points in a graph or grid, making it ideal for navigation and pathfinding tasks.
What sets A* apart is its use of heuristics to guide the search process. This makes it more efficient than algorithms like breadth-first search or Dijkstra’s algorithm, especially in large search spaces. A* is considered “complete” and “optimal,” meaning it will always find a solution if one exists, and that solution will be the best possible path.
2. How A* Works: The Core Mechanics
At its core, A* is a best-first search algorithm. It works by maintaining a priority queue of nodes to explore, always choosing the most promising node to expand next. The algorithm’s efficiency comes from its smart evaluation of nodes using a combination of two factors:
- g(n): The cost of the path from the start node to the current node n.
- h(n): The estimated cost (heuristic) from the current node n to the goal.
A* combines these factors into a single evaluation function:
f(n) = g(n) + h(n)
Where f(n) represents the estimated total cost of the path through node n. The algorithm always expands the node with the lowest f(n) value, balancing the known cost of reaching a node with the estimated cost to reach the goal.
3. Key Components of A*
To implement A* effectively, you need to understand its key components:
3.1 The Open List
This is a priority queue that contains nodes that have been discovered but not yet evaluated. Nodes in the open list are sorted by their f(n) value, with the lowest value at the top.
3.2 The Closed List
This list contains nodes that have already been evaluated. It helps prevent the algorithm from re-evaluating nodes and getting stuck in cycles.
3.3 The Heuristic Function
The heuristic function h(n) estimates the cost from any node to the goal. Common heuristics include:
- Manhattan distance: |x1 – x2| + |y1 – y2|
- Euclidean distance: sqrt((x1 – x2)^2 + (y1 – y2)^2)
- Diagonal distance: max(|x1 – x2|, |y1 – y2|)
The choice of heuristic can significantly impact the algorithm’s performance and the optimality of the solution.
4. Implementing A* in Code
Let’s implement a basic version of A* in Python to demonstrate how it works in practice. We’ll use a simple 2D grid as our search space.
import heapq
class Node:
def __init__(self, position, g_cost, h_cost, parent):
self.position = position
self.g_cost = g_cost
self.h_cost = h_cost
self.f_cost = g_cost + h_cost
self.parent = parent
def __lt__(self, other):
return self.f_cost < other.f_cost
def manhattan_distance(a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1])
def a_star(grid, start, goal):
rows, cols = len(grid), len(grid[0])
open_list = []
closed_set = set()
start_node = Node(start, 0, manhattan_distance(start, goal), None)
heapq.heappush(open_list, start_node)
while open_list:
current = heapq.heappop(open_list)
if current.position == goal:
path = []
while current:
path.append(current.position)
current = current.parent
return path[::-1]
closed_set.add(current.position)
for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
next_pos = (current.position[0] + dx, current.position[1] + dy)
if (next_pos[0] < 0 or next_pos[0] >= rows or
next_pos[1] < 0 or next_pos[1] >= cols or
grid[next_pos[0]][next_pos[1]] == 1 or
next_pos in closed_set):
continue
g_cost = current.g_cost + 1
h_cost = manhattan_distance(next_pos, goal)
next_node = Node(next_pos, g_cost, h_cost, current)
if next_node not in open_list:
heapq.heappush(open_list, next_node)
else:
# Update the node if this path is better
idx = open_list.index(next_node)
if open_list[idx].g_cost > g_cost:
open_list[idx].g_cost = g_cost
open_list[idx].f_cost = g_cost + h_cost
open_list[idx].parent = current
heapq.heapify(open_list)
return None # No path found
# Example usage
grid = [
[0, 0, 0, 0, 1],
[1, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0]
]
start = (0, 0)
goal = (4, 4)
path = a_star(grid, start, goal)
print(f"Path found: {path}")
This implementation uses a min-heap (priority queue) for the open list, ensuring efficient retrieval of the node with the lowest f-cost. The Manhattan distance is used as the heuristic function, which works well for grid-based pathfinding where movement is restricted to cardinal directions.
5. Optimizations and Variations
While the basic A* algorithm is powerful, there are several optimizations and variations that can enhance its performance in specific scenarios:
5.1 Jump Point Search (JPS)
JPS is an optimization of A* for uniform-cost grids. It reduces the number of nodes evaluated by identifying “jump points” and skipping straight sections.
5.2 Hierarchical Pathfinding
For very large maps, hierarchical pathfinding divides the map into clusters and performs pathfinding at multiple levels of abstraction.
5.3 Bidirectional A*
This variation runs two simultaneous searches: one from the start to the goal, and another from the goal to the start. It can be faster in some scenarios.
5.4 Weighted A*
By multiplying the heuristic by a weight > 1, the algorithm can find solutions faster at the cost of optimality. This is useful when speed is more important than finding the absolute shortest path.
6. Real-World Applications
A* and its variants find application in numerous real-world scenarios:
6.1 Video Games
A* is extensively used in video game AI for character navigation, especially in strategy games and RPGs.
6.2 Robotics
Autonomous robots use A* and similar algorithms for navigation and obstacle avoidance.
6.3 Geographic Information Systems (GIS)
A* is used in mapping software and GPS navigation systems to find optimal routes.
6.4 Network Routing
In computer networks, A*-like algorithms can be used to determine efficient paths for data packets.
7. Comparing A* to Other Pathfinding Algorithms
To understand A*’s strengths, it’s helpful to compare it with other popular pathfinding algorithms:
7.1 Dijkstra’s Algorithm
Dijkstra’s algorithm is guaranteed to find the shortest path but explores nodes in all directions equally. A* improves upon this by using heuristics to guide the search towards the goal.
7.2 Breadth-First Search (BFS)
BFS finds the shortest path in unweighted graphs but can be inefficient in large search spaces. A* is more efficient due to its heuristic-guided approach.
7.3 Greedy Best-First Search
This algorithm only considers the heuristic cost h(n), making it faster but not guaranteed to find the optimal path. A* balances between path cost and heuristic, ensuring optimality.
8. Challenges and Limitations
While A* is powerful, it’s not without its challenges:
8.1 Memory Usage
A* can consume significant memory in large search spaces, as it needs to keep track of all discovered nodes.
8.2 Heuristic Design
The efficiency of A* heavily depends on the quality of the heuristic function. A poor heuristic can lead to suboptimal performance.
8.3 Real-Time Constraints
In dynamic environments or real-time applications, the time taken by A* to find a path might be too long, necessitating the use of approximations or incremental search techniques.
9. The Future of A* and Pathfinding
As technology advances, so do the challenges and opportunities in pathfinding:
9.1 Machine Learning Integration
Researchers are exploring ways to use machine learning to improve heuristics or even learn entire pathfinding strategies.
9.2 Quantum Computing
Quantum algorithms might offer new ways to approach pathfinding problems, potentially solving them much faster than classical algorithms.
9.3 Multi-Agent Pathfinding
As systems become more complex, there’s increasing focus on algorithms that can efficiently plan paths for multiple agents simultaneously.
10. Conclusion
The A* search algorithm stands as a testament to the power of combining simple ideas to solve complex problems. Its blend of Dijkstra’s algorithm’s thoroughness with the guided approach of best-first search has made it a cornerstone in pathfinding and search problems.
As we’ve explored in this article, A* is not just a theoretical concept but a practical tool with wide-ranging applications. From guiding your favorite video game characters to helping plan the route of autonomous vehicles, A* continues to play a crucial role in our increasingly automated world.
For aspiring programmers and computer scientists, understanding and implementing A* is more than just an academic exercise. It’s a gateway to grasping fundamental concepts in algorithm design, heuristic search, and optimization. As you continue your journey in coding and problem-solving, remember that algorithms like A* are the building blocks upon which much of our digital world is constructed.
Whether you’re preparing for technical interviews, developing games, or working on robotics projects, the principles behind A* will serve you well. Keep exploring, keep coding, and never stop searching for the optimal path in your programming journey!