Counting Inversions Using Merge Sort: A Comprehensive Guide
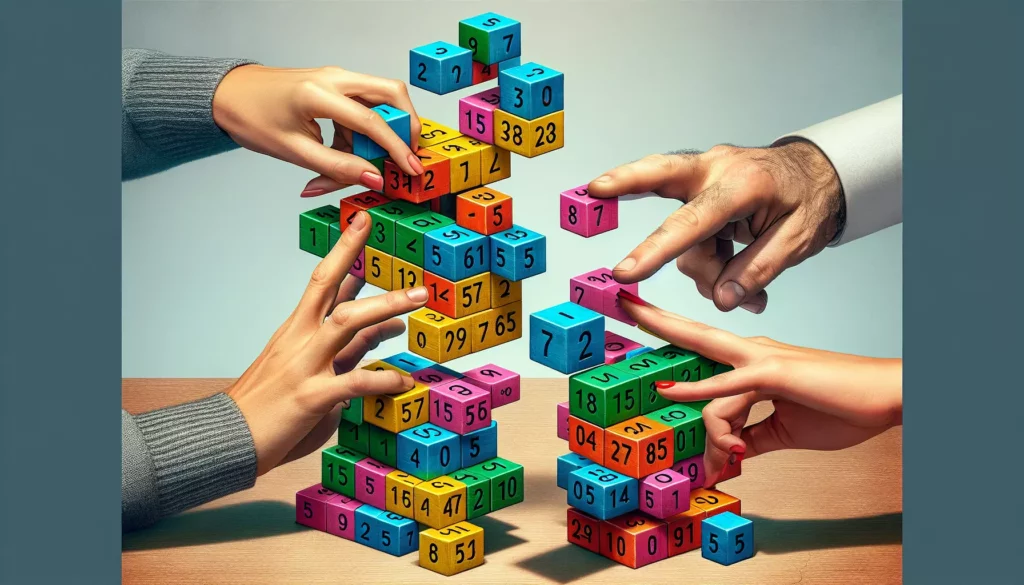
In the world of computer science and algorithmic problem-solving, counting inversions is a classic problem that often comes up in technical interviews, especially for positions at major tech companies. This problem not only tests a candidate’s understanding of sorting algorithms but also their ability to modify existing algorithms to solve related problems. In this comprehensive guide, we’ll dive deep into the concept of counting inversions using the merge sort algorithm, a technique that elegantly combines sorting and counting in a single pass.
What are Inversions?
Before we delve into the solution, let’s first understand what inversions are. In an array, an inversion occurs when a pair of elements are out of their natural order. More formally, for an array A, a pair (i, j) is an inversion if i < j and A[i] > A[j].
For example, consider the array [2, 4, 1, 3, 5]. The inversions in this array are:
- (2, 1) – because 2 > 1 and 2 appears before 1
- (4, 1) – because 4 > 1 and 4 appears before 1
- (4, 3) – because 4 > 3 and 4 appears before 3
The total number of inversions in this array is 3.
Why Count Inversions?
Counting inversions has several practical applications:
- Measuring “Sortedness”: The number of inversions in an array is a measure of how far the array is from being sorted. A completely sorted array has 0 inversions, while a reverse-sorted array has the maximum number of inversions.
- Collaborative Filtering: In recommendation systems, counting inversions can be used to measure the similarity between two users’ preferences.
- Computational Biology: In genome analysis, inversions can represent evolutionary distance between species.
The Naive Approach
The simplest way to count inversions is to use two nested loops to compare each pair of elements:
def count_inversions_naive(arr):
count = 0
n = len(arr)
for i in range(n):
for j in range(i+1, n):
if arr[i] > arr[j]:
count += 1
return count
While this approach is straightforward, it has a time complexity of O(n^2), which becomes inefficient for large arrays.
Enter Merge Sort
Merge Sort is a divide-and-conquer algorithm that sorts an array by recursively dividing it into smaller subarrays, sorting them, and then merging the sorted subarrays. The key insight is that we can count inversions while merging these sorted subarrays.
How Merge Sort Works
- Divide: The array is recursively divided into two halves until we have subarrays of size 1.
- Conquer: The subarrays are sorted (trivial for size 1).
- Combine: The sorted subarrays are merged to produce a single sorted array.
Counting Inversions During Merge
The crucial observation is that inversions can be counted efficiently during the merge step. When we merge two sorted subarrays, if an element from the right subarray is picked, it doesn’t create any new inversions. However, if an element from the left subarray is picked, it creates inversions with all the remaining elements in the right subarray.
Implementing the Solution
Let’s implement the solution step by step:
def merge_sort_and_count(arr):
# Base case: if the array has 0 or 1 element, no inversions
if len(arr) <= 1:
return arr, 0
# Divide the array into two halves
mid = len(arr) // 2
left, inv_left = merge_sort_and_count(arr[:mid])
right, inv_right = merge_sort_and_count(arr[mid:])
# Merge the sorted halves and count inversions
merged, inv_merge = merge_and_count(left, right)
# Total inversions = inversions in left + inversions in right + inversions during merge
return merged, inv_left + inv_right + inv_merge
def merge_and_count(left, right):
merged = []
inv_count = 0
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
merged.append(left[i])
i += 1
else:
merged.append(right[j])
j += 1
# Count inversions: all remaining elements in left are greater than right[j]
inv_count += len(left) - i
# Append remaining elements
merged.extend(left[i:])
merged.extend(right[j:])
return merged, inv_count
def count_inversions(arr):
_, inversions = merge_sort_and_count(arr)
return inversions
Understanding the Code
Let’s break down the key components of our implementation:
1. merge_sort_and_count Function
This function is the heart of our algorithm. It recursively divides the array, sorts each half, and then merges them while counting inversions. The function returns two values: the sorted array and the number of inversions.
2. merge_and_count Function
This function merges two sorted arrays while counting inversions. The crucial line is:
inv_count += len(left) - i
When we pick an element from the right array, it forms an inversion with all remaining elements in the left array. This is because the left array is sorted, so if right[j] is smaller than left[i], it’s also smaller than all elements after left[i].
3. count_inversions Function
This is a wrapper function that calls merge_sort_and_count and returns only the inversion count.
Time Complexity Analysis
The time complexity of this algorithm is O(n log n), the same as merge sort. Here’s why:
- The array is recursively divided into halves, which takes log n levels.
- At each level, we perform a linear-time merge operation.
- Therefore, the total time is O(n) * O(log n) = O(n log n).
This is a significant improvement over the naive O(n^2) approach, especially for large arrays.
Space Complexity
The space complexity of this algorithm is O(n). Although we’re using recursion, at any given time, we only have O(log n) recursive calls on the stack. The merge step requires an additional array of size n to store the merged result.
Example Usage
Let’s see how to use our implementation:
arr = [2, 4, 1, 3, 5]
inversions = count_inversions(arr)
print(f"Number of inversions: {inversions}") # Output: Number of inversions: 3
arr = [5, 4, 3, 2, 1]
inversions = count_inversions(arr)
print(f"Number of inversions: {inversions}") # Output: Number of inversions: 10
Common Pitfalls and Tips
- Modifying the Original Array: Our implementation creates new arrays at each recursive step. While this is cleaner, it uses more memory. For large arrays, you might want to modify the array in-place to save space.
- Integer Overflow: For very large arrays, the number of inversions can exceed the maximum value of an integer. In such cases, use a long integer or implement modular arithmetic if required.
- Handling Duplicates: Our implementation considers equal elements as not forming an inversion. Make sure this aligns with your problem’s requirements.
Variations and Related Problems
Understanding the inversion counting problem can help you solve related problems:
- Counting Inversions in a Range: Count inversions where the difference between elements is greater than a given value.
- Minimum Swaps to Sort: The minimum number of swaps required to sort an array is closely related to the number of inversions.
- Longest Increasing Subsequence: While not directly related, both problems involve analyzing the order of elements in an array.
Interview Tips
If you encounter this problem in an interview, keep these points in mind:
- Clarify the Problem: Make sure you understand what constitutes an inversion. Ask about handling duplicates if it’s not clear.
- Discuss the Naive Approach: Start by mentioning the O(n^2) solution. This shows you can think of simple solutions before optimizing.
- Explain Your Thought Process: As you move towards the merge sort solution, explain your reasoning. Interviewers are often more interested in your problem-solving approach than the final code.
- Analyze Complexity: Be prepared to discuss both time and space complexity of your solution.
- Consider Edge Cases: Discuss how your solution handles empty arrays, arrays with one element, or already sorted/reverse sorted arrays.
Conclusion
Counting inversions using merge sort is a classic problem that elegantly combines sorting and counting. It’s a prime example of how modifying a well-known algorithm can solve seemingly unrelated problems efficiently. By understanding this problem, you’re not just learning about inversions; you’re honing your skills in algorithmic thinking, divide-and-conquer strategies, and efficient problem-solving.
As you continue your journey in coding education and programming skills development, remember that problems like these are stepping stones to mastering algorithmic thinking. They prepare you not just for technical interviews at major tech companies, but for tackling complex computational problems in your future career.
Keep practicing, keep exploring variations of this problem, and most importantly, keep thinking about how you can apply these concepts to solve real-world problems. Happy coding!