Mastering Bitmask Dynamic Programming: A Comprehensive Guide
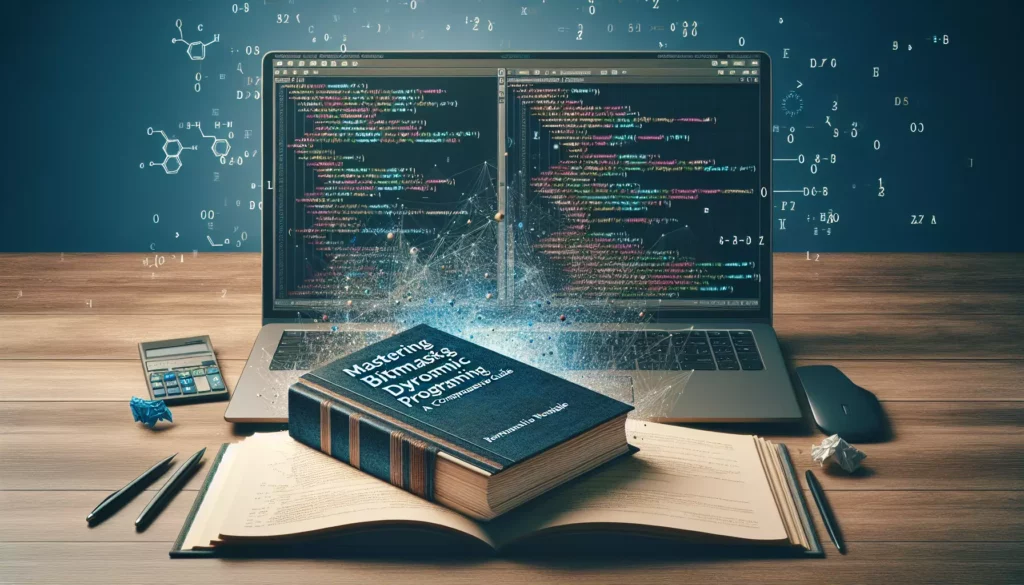
In the world of competitive programming and technical interviews, particularly for top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering advanced algorithmic techniques is crucial. One such powerful technique that often appears in complex problem-solving scenarios is Bitmask Dynamic Programming. This blog post will dive deep into this concept, exploring its fundamentals, applications, and providing practical examples to enhance your coding skills.
Table of Contents
- Introduction to Bitmask Dynamic Programming
- Understanding the Basics
- When to Use Bitmask DP
- Implementing Bitmask DP
- Real-world Examples and Problem Solving
- Optimization Techniques
- Practice Problems
- Conclusion
1. Introduction to Bitmask Dynamic Programming
Bitmask Dynamic Programming is an advanced algorithmic technique that combines the power of dynamic programming with bitwise operations. It’s particularly useful when dealing with problems involving subsets, permutations, or state representations where the number of elements is relatively small (typically less than 32).
The core idea behind Bitmask DP is to use bits to represent the state of a problem, where each bit corresponds to a particular element or condition. This representation allows for efficient storage and manipulation of states, leading to optimized solutions for otherwise complex problems.
2. Understanding the Basics
2.1 Bitwise Operations Refresher
Before diving into Bitmask DP, it’s essential to have a solid understanding of basic bitwise operations. Here’s a quick refresher:
- AND (&): Returns 1 if both bits are 1, otherwise 0
- OR (|): Returns 1 if at least one bit is 1, otherwise 0
- XOR (^): Returns 1 if bits are different, otherwise 0
- NOT (~): Inverts all bits
- Left Shift (<<): Shifts bits to the left, filling with 0
- Right Shift (>>): Shifts bits to the right, filling with 0 (unsigned) or the sign bit (signed)
2.2 Bitmask Representation
In Bitmask DP, we use an integer to represent a set of elements. Each bit in the integer corresponds to the presence (1) or absence (0) of an element in the set. For example, if we have a set of 5 elements, the bitmask 10110 (22 in decimal) represents the subset containing the 2nd, 3rd, and 5th elements.
2.3 Common Bitmask Operations
Here are some common operations used in Bitmask DP:
- Check if the i-th bit is set:
mask & (1 << i)
- Set the i-th bit:
mask | (1 << i)
- Unset the i-th bit:
mask & ~(1 << i)
- Toggle the i-th bit:
mask ^ (1 << i)
- Get the number of set bits:
__builtin_popcount(mask)
(GCC) or implement your own
3. When to Use Bitmask DP
Bitmask DP is particularly useful in scenarios where:
- The problem involves subsets or permutations of a small set of elements (typically < 32).
- You need to keep track of multiple states or conditions simultaneously.
- The problem requires exploring all possible combinations efficiently.
- The state space is too large for a straightforward DP approach but can be compressed using bits.
Common problem types that benefit from Bitmask DP include:
- Traveling Salesman Problem (TSP) and its variants
- Set covering problems
- Assignment problems
- Subset sum and partitioning problems
- Graph problems involving small sets of vertices
4. Implementing Bitmask DP
The general approach to implementing Bitmask DP involves the following steps:
- Define the state representation using bitmasks
- Initialize the DP table
- Define the base cases
- Implement the state transitions
- Iterate through all possible states
- Retrieve the final answer
Let’s look at a simple example to illustrate these steps:
Problem: Subset Sum
Given a set of integers and a target sum, determine if there exists a subset of the given set with a sum equal to the target sum.
// C++ implementation of Subset Sum using Bitmask DP
#include <iostream>
#include <vector>
using namespace std;
bool subsetSum(vector<int>& nums, int target) {
int n = nums.size();
vector<bool> dp(1 << n, false);
dp[0] = true; // Empty subset has sum 0
for (int mask = 0; mask < (1 << n); mask++) {
for (int i = 0; i < n; i++) {
if (mask & (1 << i)) {
int prevMask = mask ^ (1 << i);
if (dp[prevMask]) {
dp[mask] |= (dp[prevMask] && nums[i] <= target);
target -= nums[i];
}
}
}
}
return dp[(1 << n) - 1] && target == 0;
}
int main() {
vector<int> nums = {3, 34, 4, 12, 5, 2};
int target = 9;
cout << (subsetSum(nums, target) ? "True" : "False") << endl;
return 0;
}
In this implementation:
- We use a bitmask to represent subsets of the given set.
- The DP table
dp
stores whether a subset sum is possible for each bitmask. - We iterate through all possible subsets (bitmasks) and update the DP table.
- The final answer is stored in
dp[(1 << n) - 1]
, which represents the full set.
5. Real-world Examples and Problem Solving
Let’s explore a more complex problem that showcases the power of Bitmask DP in real-world scenarios.
Problem: Traveling Salesman Problem (TSP)
Given a set of cities and the distances between each pair of cities, find the shortest possible route that visits each city exactly once and returns to the starting city.
#include <iostream>
#include <vector>
#include <climits>
using namespace std;
const int INF = INT_MAX / 2;
int tsp(vector<vector<int>>& dist) {
int n = dist.size();
vector<vector<int>> dp(1 << n, vector<int>(n, INF));
// Base case: start from city 0
dp[1][0] = 0;
// Iterate through all subsets of cities
for (int mask = 1; mask < (1 << n); mask++) {
for (int u = 0; u < n; u++) {
if (mask & (1 << u)) {
for (int v = 0; v < n; v++) {
if (v != u && (mask & (1 << v))) {
dp[mask][u] = min(dp[mask][u], dp[mask ^ (1 << u)][v] + dist[v][u]);
}
}
}
}
}
// Find the minimum cost to return to city 0
int finalMask = (1 << n) - 1;
int minCost = INF;
for (int u = 1; u < n; u++) {
minCost = min(minCost, dp[finalMask][u] + dist[u][0]);
}
return minCost;
}
int main() {
vector<vector<int>> dist = {
{0, 10, 15, 20},
{10, 0, 35, 25},
{15, 35, 0, 30},
{20, 25, 30, 0}
};
cout << "Minimum cost of TSP: " << tsp(dist) << endl;
return 0;
}
In this TSP implementation:
- We use a 2D DP table where
dp[mask][u]
represents the minimum cost to visit all cities in the bitmask and end at city u. - The bitmask represents the set of cities visited.
- We iterate through all subsets of cities and compute the minimum cost for each state.
- Finally, we find the minimum cost to return to the starting city.
This solution demonstrates how Bitmask DP can efficiently solve the NP-hard Traveling Salesman Problem for small to medium-sized inputs.
6. Optimization Techniques
While Bitmask DP is already an optimization technique, there are ways to further improve its performance:
6.1 Memoization
Instead of using a bottom-up approach, you can implement a top-down approach with memoization. This can be more intuitive and may avoid unnecessary computations.
6.2 Bit Manipulation Tricks
Use efficient bit manipulation techniques to speed up operations:
- Use
__builtin_popcount()
(GCC) for counting set bits - Use
mask & -mask
to get the least significant set bit - Use
mask & (mask - 1)
to turn off the least significant set bit
6.3 State Compression
If the number of elements is larger than what can fit in a single integer, consider using multiple integers or a custom bitset implementation.
6.4 Parallel Processing
For very large problem sizes, consider parallelizing the computation of independent subproblems.
7. Practice Problems
To master Bitmask DP, practice is key. Here are some problems you can try:
- Maximum Students Taking Exam (LeetCode)
- Kefa and Dishes (Codeforces)
- Courier (SPOJ)
- Matching (AtCoder)
- Elevator Rides (CSES)
These problems cover a range of difficulties and applications of Bitmask DP, helping you build confidence and intuition for when and how to apply this technique.
8. Conclusion
Bitmask Dynamic Programming is a powerful technique that combines the efficiency of bitwise operations with the problem-solving capabilities of dynamic programming. By mastering this approach, you’ll be better equipped to tackle complex algorithmic challenges in competitive programming and technical interviews, especially for top tech companies.
Remember that like any advanced technique, proficiency in Bitmask DP comes with practice. Start with simpler problems and gradually work your way up to more complex scenarios. As you gain experience, you’ll develop an intuition for when Bitmask DP can be applied to optimize your solutions.
Keep in mind that while Bitmask DP is incredibly useful, it’s not always the best solution. Always consider the problem constraints and requirements before deciding on your approach. Sometimes, a simpler solution or a different algorithm might be more appropriate.
As you continue your journey in algorithmic problem-solving, don’t forget to explore other advanced techniques as well. The combination of Bitmask DP with other algorithms and data structures can lead to even more powerful and efficient solutions.
Happy coding, and may your bits be ever in your favor!