Building Cross-Platform Applications: A Comprehensive Guide
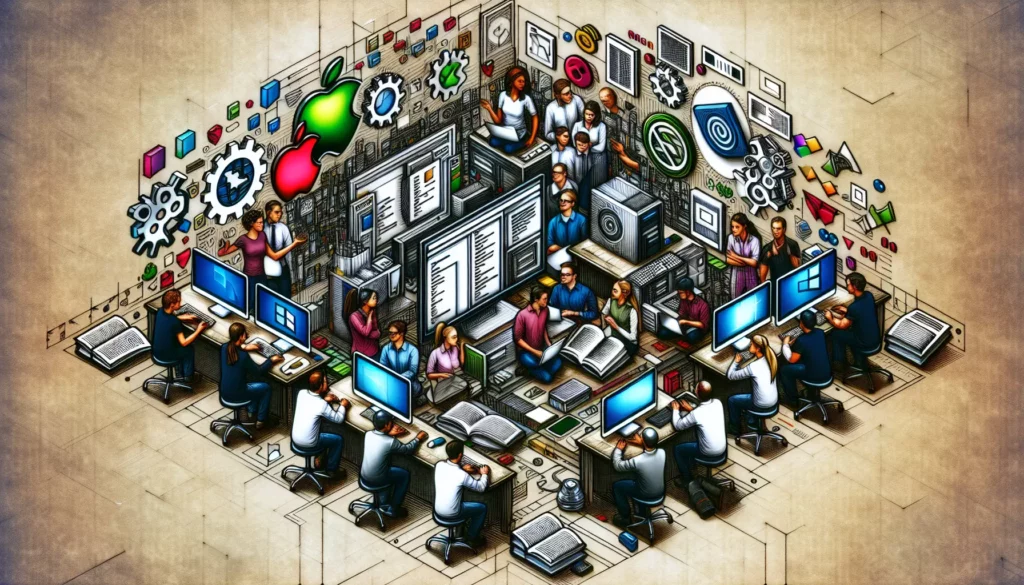
In today’s diverse technological landscape, the ability to create applications that work seamlessly across multiple platforms is more crucial than ever. Cross-platform development allows developers to write code once and deploy it on various operating systems and devices, saving time, effort, and resources. This comprehensive guide will explore the world of cross-platform application development, covering everything from its benefits and challenges to popular frameworks and best practices.
Table of Contents
- Introduction to Cross-Platform Development
- Benefits of Cross-Platform Applications
- Challenges in Cross-Platform Development
- Popular Cross-Platform Frameworks
- Deep Dive: React Native
- Deep Dive: Flutter
- Deep Dive: Xamarin
- Deep Dive: Electron
- Best Practices for Cross-Platform Development
- Testing Cross-Platform Applications
- Optimizing Performance in Cross-Platform Apps
- The Future of Cross-Platform Development
- Conclusion
1. Introduction to Cross-Platform Development
Cross-platform development refers to the practice of creating software applications that can run on multiple computing platforms. Instead of building separate versions of an app for different operating systems (like iOS, Android, Windows, or macOS), developers can write code once and deploy it across various platforms with minimal modifications.
This approach has gained significant traction in recent years due to the proliferation of devices and operating systems. As users increasingly expect applications to be available on all their devices, cross-platform development has become a strategic choice for many businesses and developers.
2. Benefits of Cross-Platform Applications
Cross-platform development offers numerous advantages:
- Cost-Effectiveness: Developing a single codebase for multiple platforms significantly reduces development costs compared to creating separate native applications.
- Faster Time-to-Market: With a single development cycle, apps can be released simultaneously on multiple platforms, accelerating the time-to-market.
- Wider Audience Reach: Cross-platform apps can target users across different devices and operating systems, potentially increasing the user base.
- Easier Maintenance: Updates and bug fixes can be implemented once and applied across all platforms, simplifying maintenance.
- Consistent User Experience: Cross-platform frameworks allow for a more uniform look and feel across different devices.
- Code Reusability: Developers can reuse a significant portion of the codebase across platforms, improving efficiency.
3. Challenges in Cross-Platform Development
While cross-platform development offers many benefits, it also comes with its own set of challenges:
- Performance Issues: Cross-platform apps may not perform as well as native apps, especially for graphics-intensive applications.
- Platform-Specific Features: Accessing unique features of specific platforms can be challenging and may require native modules or plugins.
- User Interface Consistency: Achieving a truly native look and feel across all platforms can be difficult.
- Learning Curve: Developers need to learn new frameworks and tools specific to cross-platform development.
- Debugging Complexity: Identifying and fixing issues across multiple platforms can be more complex than in native development.
- Limited Access to Latest Features: Cross-platform frameworks may lag behind in supporting the latest platform-specific features.
4. Popular Cross-Platform Frameworks
Several frameworks have emerged to facilitate cross-platform development. Here are some of the most popular ones:
- React Native: Developed by Facebook, React Native allows building mobile apps using JavaScript and React.
- Flutter: Google’s UI toolkit for crafting natively compiled applications for mobile, web, and desktop from a single codebase.
- Xamarin: Microsoft’s framework for building Android, iOS, and Windows applications with .NET and C#.
- Electron: A framework for creating native applications with web technologies like JavaScript, HTML, and CSS.
- Ionic: An open-source SDK for hybrid mobile app development.
- PhoneGap (Apache Cordova): An open-source mobile development framework.
- Unity: Primarily used for game development but can be used for other types of applications as well.
5. Deep Dive: React Native
React Native, introduced by Facebook in 2015, has become one of the most popular frameworks for cross-platform mobile development. It allows developers to use React along with native platform capabilities to build mobile applications.
Key Features of React Native:
- Uses JavaScript and React for development
- Renders using native components
- Hot reloading for faster development
- Large community and extensive third-party libraries
- Can integrate with native code when needed
Example of a Simple React Native Component:
import React from 'react';
import { Text, View } from 'react-native';
const HelloWorldApp = () => {
return (
<View style={{ flex: 1, justifyContent: "center", alignItems: "center" }}>
<Text>Hello, world!</Text>
</View>
);
}
export default HelloWorldApp;
This simple component creates a view with centered text, demonstrating the basics of React Native’s component structure and styling.
6. Deep Dive: Flutter
Flutter, developed by Google, is a relatively newer framework that has gained significant popularity. It uses Dart as its programming language and provides a rich set of pre-designed widgets.
Key Features of Flutter:
- Uses Dart programming language
- Hot reload for instant view of changes
- Rich set of customizable widgets
- High performance with the Skia graphics engine
- Single codebase for mobile, web, and desktop
Example of a Simple Flutter Widget:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Welcome to Flutter'),
),
body: Center(
child: Text('Hello World'),
),
),
);
}
}
This example creates a simple Flutter app with an app bar and centered text, showcasing Flutter’s widget-based UI construction.
7. Deep Dive: Xamarin
Xamarin, now part of Microsoft, allows developers to use C# and .NET to build cross-platform applications. It’s particularly popular among developers with a background in Microsoft technologies.
Key Features of Xamarin:
- Uses C# and .NET
- Native UI components
- Shared business logic across platforms
- Integration with Visual Studio
- Access to platform-specific APIs
Example of a Simple Xamarin.Forms Page:
using Xamarin.Forms;
public class MainPage : ContentPage
{
public MainPage()
{
Content = new StackLayout
{
Children = {
new Label {
Text = "Welcome to Xamarin.Forms!",
HorizontalOptions = LayoutOptions.Center,
VerticalOptions = LayoutOptions.CenterAndExpand
}
}
};
}
}
This example creates a simple Xamarin.Forms page with centered text, demonstrating the basics of UI creation in Xamarin.
8. Deep Dive: Electron
Electron is a framework for creating native applications with web technologies. It’s widely used for building desktop applications that can run on Windows, macOS, and Linux.
Key Features of Electron:
- Uses web technologies (HTML, CSS, JavaScript)
- Access to native APIs
- Automatic updates
- Debug tools and crash reporting
- Large community and ecosystem
Example of a Simple Electron App:
const { app, BrowserWindow } = require('electron')
function createWindow () {
const win = new BrowserWindow({
width: 800,
height: 600,
webPreferences: {
nodeIntegration: true
}
})
win.loadFile('index.html')
}
app.whenReady().then(createWindow)
app.on('window-all-closed', () => {
if (process.platform !== 'darwin') {
app.quit()
}
})
This example sets up a basic Electron application, creating a window and loading an HTML file into it.
9. Best Practices for Cross-Platform Development
To ensure the success of your cross-platform project, consider these best practices:
- Plan for Platform Differences: Understand the unique characteristics and constraints of each target platform.
- Design with Flexibility: Create a UI that can adapt to different screen sizes and orientations.
- Optimize Performance: Pay attention to app performance, especially on lower-end devices.
- Use Platform-Specific Features Wisely: Leverage platform-specific features when necessary, but maintain a balance with cross-platform consistency.
- Follow Platform Guidelines: Adhere to the design guidelines of each platform to ensure a native feel.
- Code Organization: Structure your code to maximize reusability across platforms.
- Regular Testing: Test your app thoroughly on all target platforms throughout the development process.
- Keep Dependencies Updated: Regularly update your framework and dependencies to benefit from the latest improvements and security patches.
- Implement Responsive Design: Ensure your app looks good and functions well on various screen sizes and resolutions.
- Consider Offline Functionality: Design your app to work offline or with limited connectivity when possible.
10. Testing Cross-Platform Applications
Testing is crucial in cross-platform development to ensure consistent functionality and user experience across all target platforms. Here are some key aspects of testing cross-platform applications:
Types of Testing:
- Unit Testing: Test individual components or functions of your application.
- Integration Testing: Verify that different parts of your application work together correctly.
- UI Testing: Ensure the user interface behaves correctly and consistently across platforms.
- Performance Testing: Check the app’s speed, responsiveness, and resource usage on different devices.
- Compatibility Testing: Test on various devices, OS versions, and screen sizes.
Testing Tools:
- Appium: An open-source tool for automating native, mobile web, and hybrid applications on iOS and Android platforms.
- Xamarin Test Cloud: Allows testing Xamarin apps on hundreds of real devices in the cloud.
- Flutter Driver: Provides tools to test Flutter apps on real devices and emulators.
- Jest: A popular JavaScript testing framework often used with React Native.
- Detox: An end-to-end testing and automation framework for mobile apps.
Best Practices for Testing:
- Test on real devices whenever possible, not just emulators or simulators.
- Automate as much of your testing process as possible to save time and ensure consistency.
- Include edge cases and error scenarios in your test cases.
- Test network conditions, including offline scenarios and slow connections.
- Involve beta testers to get real-world feedback before launch.
11. Optimizing Performance in Cross-Platform Apps
Performance optimization is crucial for cross-platform apps to ensure they run smoothly across all target platforms. Here are some strategies to improve performance:
1. Minimize Network Requests:
- Batch API calls where possible.
- Implement efficient caching strategies.
- Use compression for data transfers.
2. Optimize Images and Assets:
- Use appropriate image formats and compress images.
- Implement lazy loading for images and other resources.
- Use vector graphics where possible for resolution independence.
3. Efficient Rendering:
- Minimize the use of animations and complex layouts.
- Use platform-specific optimizations when available.
- Implement virtualized lists for long scrollable content.
4. Memory Management:
- Avoid memory leaks by properly disposing of resources.
- Use appropriate data structures and algorithms.
- Implement pagination for large datasets.
5. Code Optimization:
- Use efficient algorithms and data structures.
- Minimize the use of third-party libraries and evaluate their performance impact.
- Implement code splitting and lazy loading of modules.
6. Platform-Specific Optimizations:
- Use native modules for performance-critical tasks.
- Leverage platform-specific hardware acceleration when available.
Example: Optimizing a React Native FlatList
import React from 'react';
import { FlatList, Text, View } from 'react-native';
const OptimizedList = ({ data }) => {
const renderItem = ({ item }) => (
<View>
<Text>{item.title}</Text>
</View>
);
return (
<FlatList
data={data}
renderItem={renderItem}
keyExtractor={(item) => item.id.toString()}
initialNumToRender={10}
maxToRenderPerBatch={10}
windowSize={5}
removeClippedSubviews={true}
/>
);
};
export default OptimizedList;
This example demonstrates an optimized FlatList in React Native, using properties like initialNumToRender
, maxToRenderPerBatch
, and removeClippedSubviews
to improve performance for long lists.
12. The Future of Cross-Platform Development
The field of cross-platform development is rapidly evolving. Here are some trends and predictions for the future:
1. Progressive Web Apps (PWAs):
PWAs are becoming increasingly popular as they offer a native app-like experience through web technologies. They’re expected to play a significant role in cross-platform development.
2. Improved Performance:
Future advancements in cross-platform frameworks are likely to focus on closing the performance gap with native applications.
3. AI and Machine Learning Integration:
Expect to see more robust AI and ML capabilities integrated into cross-platform development tools, making it easier to build intelligent apps.
4. Internet of Things (IoT):
Cross-platform development will likely expand to better support IoT devices, allowing developers to create applications that seamlessly interact with a wide range of smart devices.
5. Enhanced AR and VR Support:
As AR and VR technologies become more mainstream, cross-platform frameworks will likely improve their support for these immersive experiences.
6. Serverless and Edge Computing:
The integration of serverless architectures and edge computing with cross-platform apps is expected to increase, offering improved performance and scalability.
7. Continued Framework Evolution:
Existing frameworks like React Native, Flutter, and Xamarin will continue to evolve, and new frameworks may emerge to address current limitations.
13. Conclusion
Cross-platform application development has become an essential skill in today’s diverse technological landscape. It offers numerous benefits, including cost-effectiveness, faster development cycles, and wider audience reach. While it comes with its own set of challenges, the continuous evolution of frameworks and best practices is making cross-platform development increasingly viable and attractive.
As we’ve explored in this guide, there are several powerful frameworks available, each with its own strengths and use cases. Whether you choose React Native, Flutter, Xamarin, Electron, or any other framework, the key to success lies in understanding the unique requirements of your project and the characteristics of your target platforms.
Remember to follow best practices, prioritize performance optimization, and conduct thorough testing across all target platforms. Stay updated with the latest trends and advancements in the field, as cross-platform development continues to evolve rapidly.
By mastering cross-platform development, you’ll be well-equipped to create versatile, efficient applications that can reach users across a wide range of devices and operating systems. This skill set will undoubtedly be valuable in the ever-changing landscape of software development.