Morris Traversal: A Space-Efficient Approach to Tree Traversal Without Recursion
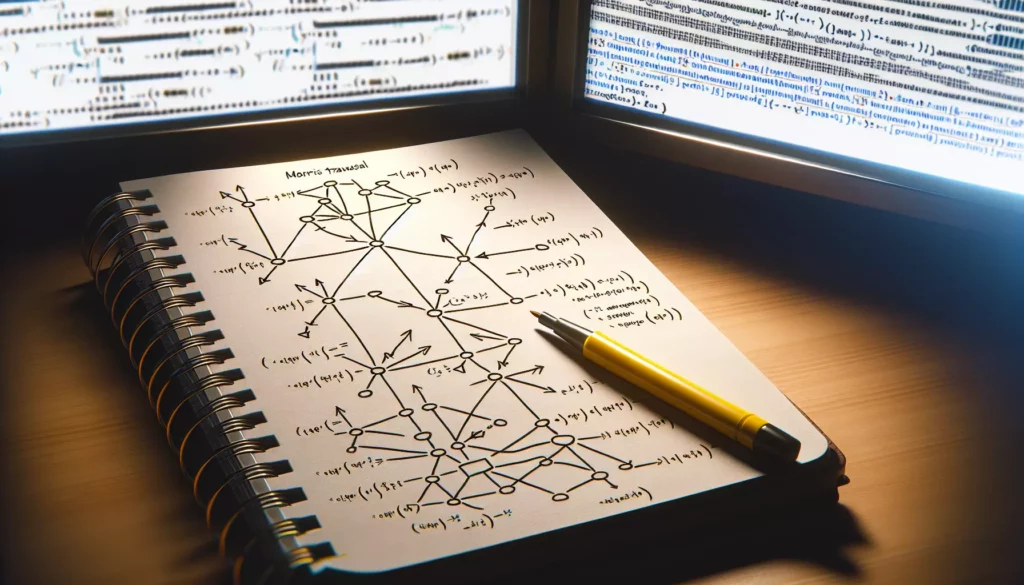
When it comes to traversing binary trees, most developers are familiar with recursive methods or stack-based iterative approaches. However, there’s a lesser-known but highly efficient technique called Morris Traversal that deserves attention. This algorithm, developed by Joseph M. Morris in 1979, offers a unique way to traverse binary trees without using recursion or a stack, achieving O(1) space complexity. In this comprehensive guide, we’ll dive deep into Morris Traversal, exploring its implementation, benefits, and applications in the context of coding interviews and efficient programming.
Understanding Tree Traversal
Before we delve into Morris Traversal, let’s briefly recap the concept of tree traversal. Tree traversal is the process of visiting each node in a tree data structure exactly once. The three most common types of tree traversal are:
- In-order traversal: Left subtree, root, right subtree
- Pre-order traversal: Root, left subtree, right subtree
- Post-order traversal: Left subtree, right subtree, root
Traditionally, these traversals are implemented using recursive functions or iterative approaches with a stack. While these methods are intuitive and widely used, they come with a space complexity of O(h), where h is the height of the tree. This can be problematic for very deep trees or in memory-constrained environments.
Enter Morris Traversal
Morris Traversal offers a clever solution to the space complexity issue. It achieves O(1) space complexity by using the tree’s structure itself to navigate, eliminating the need for additional data structures like stacks or recursion. The key idea behind Morris Traversal is to create temporary links between nodes, allowing us to move back up the tree without explicitly storing the path.
How Morris Traversal Works
The algorithm works by establishing temporary links from the rightmost node of the left subtree to the current node. This link serves as a “thread” that allows us to return to the parent after processing the left subtree. Here’s a step-by-step breakdown of the process:
- Start with the current node as the root of the tree.
- While the current node is not null:
- If the current node has no left child:
- Process the current node (e.g., print its value)
- Move to the right child
- If the current node has a left child:
- Find the rightmost node in the left subtree
- If this rightmost node’s right pointer is null:
- Set its right pointer to the current node (creating the thread)
- Move to the left child
- If the rightmost node’s right pointer is already pointing to the current node:
- Set its right pointer back to null (removing the thread)
- Process the current node
- Move to the right child
- If the current node has no left child:
Implementing Morris Traversal
Let’s implement Morris Traversal for in-order traversal in Java. First, we’ll define our binary tree node structure:
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int val) {
this.val = val;
}
}
Now, let’s implement the Morris Traversal algorithm:
import java.util.ArrayList;
import java.util.List;
public class MorrisTraversal {
public List<Integer> inorderTraversal(TreeNode root) {
List<Integer> result = new ArrayList<>();
TreeNode current = root;
while (current != null) {
if (current.left == null) {
result.add(current.val);
current = current.right;
} else {
TreeNode predecessor = findPredecessor(current);
if (predecessor.right == null) {
predecessor.right = current;
current = current.left;
} else {
predecessor.right = null;
result.add(current.val);
current = current.right;
}
}
}
return result;
}
private TreeNode findPredecessor(TreeNode node) {
TreeNode predecessor = node.left;
while (predecessor.right != null && predecessor.right != node) {
predecessor = predecessor.right;
}
return predecessor;
}
}
Explanation of the Implementation
- We start with the root node as our current node.
- We enter a loop that continues until we’ve processed all nodes.
- If the current node has no left child, we process it and move to the right.
- If the current node has a left child, we find its in-order predecessor (the rightmost node in its left subtree).
- If the predecessor’s right is null, we create a thread by pointing it to the current node and move left.
- If the predecessor’s right already points to the current node, we remove the thread, process the current node, and move right.
- We repeat this process until all nodes are visited.
Time and Space Complexity Analysis
One of the most significant advantages of Morris Traversal is its space efficiency:
- Time Complexity: O(n), where n is the number of nodes in the tree. Although we may visit some nodes multiple times to find predecessors, each edge in the tree is traversed at most three times.
- Space Complexity: O(1), as we only use a constant amount of extra space, regardless of the tree’s size.
This makes Morris Traversal particularly useful in scenarios where memory is constrained or when dealing with very large trees.
Advantages and Disadvantages of Morris Traversal
Advantages:
- Space Efficiency: Uses O(1) extra space, making it ideal for memory-constrained environments.
- No Stack Overflow: Eliminates the risk of stack overflow that can occur with deep recursion.
- In-place Algorithm: Modifies the tree temporarily but restores it to its original state.
Disadvantages:
- Complexity: The algorithm is more complex to understand and implement compared to recursive or stack-based approaches.
- Tree Modification: Temporarily modifies the tree structure, which might not be desirable in some scenarios.
- Not Suitable for All Trees: Works best with binary trees; adaptation for other tree types can be challenging.
Applications and Use Cases
Morris Traversal finds its applications in various scenarios:
- Memory-Constrained Environments: Ideal for systems with limited memory resources.
- Large-Scale Data Processing: Efficient for traversing extremely large binary trees.
- Embedded Systems: Useful in embedded systems where memory usage needs to be minimized.
- Interview Preparation: A great algorithm to showcase advanced tree traversal knowledge in technical interviews.
Variations of Morris Traversal
While we’ve focused on in-order traversal, Morris Traversal can be adapted for other traversal types:
Pre-order Morris Traversal
For pre-order traversal, we simply move the node processing step earlier in the algorithm:
public List<Integer> preorderTraversal(TreeNode root) {
List<Integer> result = new ArrayList<>();
TreeNode current = root;
while (current != null) {
if (current.left == null) {
result.add(current.val);
current = current.right;
} else {
TreeNode predecessor = findPredecessor(current);
if (predecessor.right == null) {
result.add(current.val);
predecessor.right = current;
current = current.left;
} else {
predecessor.right = null;
current = current.right;
}
}
}
return result;
}
Post-order Morris Traversal
Post-order traversal using Morris Traversal is more complex and requires additional steps. Here’s a basic implementation:
public List<Integer> postorderTraversal(TreeNode root) {
List<Integer> result = new ArrayList<>();
TreeNode dummy = new TreeNode(0);
dummy.left = root;
TreeNode current = dummy;
while (current != null) {
if (current.left == null) {
current = current.right;
} else {
TreeNode predecessor = findPredecessor(current);
if (predecessor.right == null) {
predecessor.right = current;
current = current.left;
} else {
predecessor.right = null;
addReversed(result, current.left);
current = current.right;
}
}
}
return result;
}
private void addReversed(List<Integer> result, TreeNode from) {
TreeNode end = reverseRight(from);
TreeNode node = end;
while (true) {
result.add(node.val);
if (node == from) break;
node = node.right;
}
reverseRight(end);
}
private TreeNode reverseRight(TreeNode node) {
TreeNode prev = null;
TreeNode current = node;
while (current != null) {
TreeNode next = current.right;
current.right = prev;
prev = current;
current = next;
}
return prev;
}
Comparison with Other Traversal Methods
Let’s compare Morris Traversal with other common tree traversal methods:
Method | Time Complexity | Space Complexity | Pros | Cons |
---|---|---|---|---|
Recursive | O(n) | O(h) – height of tree | Simple to implement | Risk of stack overflow for deep trees |
Iterative (with stack) | O(n) | O(h) – height of tree | No recursion, controlled space usage | Requires additional data structure |
Morris Traversal | O(n) | O(1) | Constant space, no stack overflow risk | More complex implementation |
Best Practices and Tips
When working with Morris Traversal, keep these best practices in mind:
- Understand the Algorithm Thoroughly: Morris Traversal can be tricky; make sure you grasp the concept fully before implementation.
- Be Careful with Tree Modifications: Always ensure that the tree is restored to its original state after traversal.
- Consider Thread Safety: If working in a multi-threaded environment, be aware that Morris Traversal temporarily modifies the tree structure.
- Use for the Right Scenarios: While powerful, Morris Traversal isn’t always necessary. Use it when space efficiency is crucial.
- Practice Implementation: Due to its complexity, practice implementing Morris Traversal for different traversal orders to solidify your understanding.
Common Pitfalls and How to Avoid Them
When implementing Morris Traversal, be aware of these common pitfalls:
- Infinite Loops: Ensure that you’re correctly setting and unsetting the temporary links to avoid infinite loops.
- Incomplete Traversal: Make sure your implementation visits all nodes, including the rightmost path of the tree.
- Tree Corruption: Always restore the tree to its original state by removing all temporary links.
- Overlooking Edge Cases: Test your implementation with various tree structures, including unbalanced trees and trees with a single node.
Morris Traversal in Interview Scenarios
Morris Traversal can be an impressive technique to showcase in coding interviews, especially when discussing space optimization. Here are some tips for using Morris Traversal in interview scenarios:
- Explain the Trade-offs: Discuss why you might choose Morris Traversal over other methods, focusing on its space efficiency.
- Demonstrate Understanding: Clearly explain how the algorithm works, possibly using diagrams to illustrate the temporary link creation and removal.
- Mention Practical Applications: Discuss real-world scenarios where Morris Traversal would be particularly useful.
- Be Prepared for Follow-up Questions: Interviewers might ask about adapting the algorithm for different traversal orders or tree structures.
Advanced Topics and Further Exploration
For those looking to dive deeper into tree traversal and related concepts, consider exploring these advanced topics:
- Threaded Binary Trees: Understand how Morris Traversal relates to the concept of threaded binary trees.
- Adapting for N-ary Trees: Explore how Morris Traversal might be adapted for trees with more than two children per node.
- Concurrent Tree Traversal: Investigate methods for safe concurrent traversal of binary trees.
- Morris Traversal in Functional Programming: Consider how Morris Traversal might be implemented in functional programming paradigms.
Conclusion
Morris Traversal stands out as a unique and efficient method for tree traversal, offering unparalleled space efficiency. While it may not be the go-to solution for every tree traversal problem, understanding and mastering this algorithm can significantly enhance your problem-solving toolkit, especially in scenarios where memory constraints are a concern.
As you continue your journey in algorithm and data structure mastery, remember that techniques like Morris Traversal showcase the importance of creative thinking in computer science. They remind us that there’s often more than one way to approach a problem, and sometimes, thinking outside the box can lead to elegant and efficient solutions.
Whether you’re preparing for technical interviews, working on memory-constrained systems, or simply expanding your algorithmic knowledge, Morris Traversal is a valuable technique to have in your repertoire. Keep practicing, exploring variations, and considering how this approach might be applied to solve complex problems in your coding projects.