K-way Merge: A Comprehensive Guide to Merging Sorted Arrays
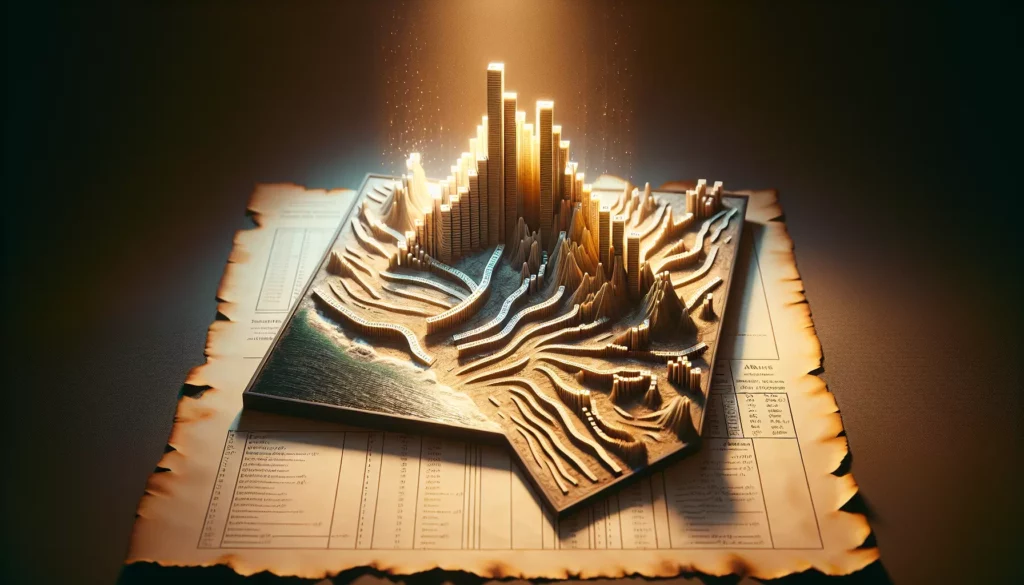
In the world of computer science and algorithmic problem-solving, efficient data manipulation is crucial. One common challenge that developers often face is merging multiple sorted arrays or lists into a single sorted output. This is where the K-way merge algorithm comes into play. In this comprehensive guide, we’ll dive deep into the concept of K-way merge, explore its applications, and learn how to implement it effectively.
Table of Contents
- Introduction to K-way Merge
- Applications of K-way Merge
- The K-way Merge Algorithm
- Implementing K-way Merge
- Optimization Techniques
- Time and Space Complexity Analysis
- Variations and Extensions
- Interview Tips and Common Questions
- Conclusion
1. Introduction to K-way Merge
K-way merge, also known as K-way merging or multi-way merge, is an algorithm used to combine multiple sorted arrays or lists into a single sorted output. The “K” in K-way merge represents the number of input arrays or lists that need to be merged. This algorithm is an extension of the classic two-way merge used in merge sort, but it can handle more than two input sources simultaneously.
The primary goal of K-way merge is to efficiently combine these sorted inputs while maintaining the overall sorted order in the final output. This process is particularly useful when dealing with large datasets that are already partially sorted or when merging results from multiple sources.
2. Applications of K-way Merge
K-way merge finds applications in various domains of computer science and software engineering. Some common use cases include:
- External Sorting: When dealing with datasets too large to fit in memory, K-way merge is used to combine partially sorted chunks of data.
- Database Operations: Merging sorted results from multiple database queries or tables.
- File Systems: Combining sorted file segments in distributed file systems.
- Information Retrieval: Merging sorted document lists in search engine indexing.
- Parallel Computing: Combining sorted results from multiple parallel processes.
- Stream Processing: Merging multiple sorted data streams in real-time applications.
Understanding and implementing K-way merge efficiently can significantly improve the performance of these applications, especially when dealing with large-scale data processing tasks.
3. The K-way Merge Algorithm
The K-way merge algorithm works on the principle of comparing the smallest elements from each input array and selecting the overall smallest element to add to the output. Here’s a step-by-step breakdown of the algorithm:
- Initialize an empty output array to store the merged result.
- Create a min-heap (or priority queue) to keep track of the smallest elements from each input array.
- Insert the first element from each input array into the min-heap, along with its array index and element index.
- While the min-heap is not empty:
- Extract the minimum element from the min-heap.
- Add this element to the output array.
- If there are more elements in the input array from which this element came, add the next element from that array to the min-heap.
- Return the output array containing the merged and sorted elements.
This algorithm ensures that we always select the smallest available element across all input arrays, maintaining the sorted order in the final output.
4. Implementing K-way Merge
Let’s implement the K-way merge algorithm in Python. We’ll use the heapq
module to create a min-heap, which will help us efficiently track the smallest elements from each input array.
import heapq
def k_way_merge(arrays):
result = []
heap = []
# Initialize the heap with the first element from each array
for i, arr in enumerate(arrays):
if arr:
heapq.heappush(heap, (arr[0], i, 0))
# Merge arrays
while heap:
val, array_index, element_index = heapq.heappop(heap)
result.append(val)
if element_index + 1 < len(arrays[array_index]):
next_element = arrays[array_index][element_index + 1]
heapq.heappush(heap, (next_element, array_index, element_index + 1))
return result
# Example usage
sorted_arrays = [
[1, 4, 7],
[2, 5, 8],
[3, 6, 9]
]
merged_array = k_way_merge(sorted_arrays)
print(merged_array)
# Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
In this implementation:
- We use a min-heap to keep track of the smallest elements from each input array.
- Each element in the heap is a tuple containing the value, the index of its source array, and its index within that array.
- We initialize the heap with the first element from each input array.
- We repeatedly extract the minimum element from the heap, add it to the result, and push the next element from the same input array (if available) onto the heap.
- This process continues until the heap is empty, at which point all elements have been merged.
5. Optimization Techniques
While the basic implementation of K-way merge is efficient, there are several optimization techniques we can apply to further improve its performance:
5.1. Early Termination
If we know that some input arrays may be shorter than others, we can implement an early termination check. Once an array is exhausted, we remove it from consideration, potentially reducing the number of comparisons in subsequent iterations.
5.2. Chunk-based Processing
For very large input arrays, we can process elements in chunks rather than one at a time. This can improve cache efficiency and reduce the number of heap operations.
5.3. Custom Comparison Functions
If we’re merging complex objects rather than simple numbers, we can provide a custom comparison function to the heap to determine the order of elements.
5.4. Parallel Processing
For large-scale merge operations, we can parallelize the process by dividing the input arrays among multiple threads or processes, merging them independently, and then combining the results.
5.5. Memory-efficient Merging
When dealing with very large datasets that don’t fit in memory, we can implement an external merge algorithm that reads and writes data to disk in chunks.
Here’s an example of how we might implement chunk-based processing:
import heapq
def k_way_merge_chunked(arrays, chunk_size=1000):
result = []
heap = []
# Initialize the heap with the first chunk from each array
for i, arr in enumerate(arrays):
chunk = arr[:chunk_size]
if chunk:
heapq.heappush(heap, (chunk[0], i, 0, chunk))
# Merge arrays
while heap:
val, array_index, element_index, current_chunk = heapq.heappop(heap)
result.append(val)
if element_index + 1 < len(current_chunk):
next_element = current_chunk[element_index + 1]
heapq.heappush(heap, (next_element, array_index, element_index + 1, current_chunk))
elif len(arrays[array_index]) > (element_index + 1 + chunk_size):
start = element_index + 1 + chunk_size
new_chunk = arrays[array_index][start:start+chunk_size]
heapq.heappush(heap, (new_chunk[0], array_index, 0, new_chunk))
return result
This chunked implementation processes the input arrays in chunks of a specified size, potentially improving performance for very large inputs.
6. Time and Space Complexity Analysis
Understanding the time and space complexity of the K-way merge algorithm is crucial for assessing its efficiency and scalability.
6.1. Time Complexity
The time complexity of K-way merge can be analyzed as follows:
- Let N be the total number of elements across all K input arrays.
- For each element, we perform a heap operation (either push or pop) which takes O(log K) time.
- We do this for all N elements.
Therefore, the overall time complexity is O(N log K).
6.2. Space Complexity
The space complexity of K-way merge is as follows:
- We use a heap that stores at most K elements at any time.
- We also need space for the output array, which will contain N elements.
Thus, the space complexity is O(N + K).
It’s worth noting that the space complexity can be reduced to O(K) if we use an in-place merging strategy, although this may complicate the implementation.
7. Variations and Extensions
The basic K-way merge algorithm can be extended and modified to handle various scenarios and requirements. Some interesting variations include:
7.1. K-way Merge for Linked Lists
Instead of arrays, we might need to merge K sorted linked lists. The core algorithm remains similar, but the implementation details change to handle linked list operations.
7.2. External K-way Merge
When dealing with datasets too large to fit in memory, we can implement an external K-way merge that reads and writes data to disk in chunks.
7.3. K-way Merge with Duplicates
In some cases, we might need to handle duplicate elements across the input arrays. We can modify the algorithm to either keep or remove duplicates based on the requirements.
7.4. Parallel K-way Merge
For large-scale merge operations, we can parallelize the process by dividing the input arrays among multiple threads or processes and then combining the results.
7.5. K-way Merge with Limited Memory
In memory-constrained environments, we might need to implement a K-way merge that uses a fixed amount of memory regardless of the input size.
Here’s a simple example of how we might implement K-way merge for linked lists:
import heapq
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def merge_k_lists(lists):
dummy = ListNode(0)
current = dummy
heap = []
# Initialize the heap
for i, node in enumerate(lists):
if node:
heapq.heappush(heap, (node.val, i, node))
# Merge lists
while heap:
val, i, node = heapq.heappop(heap)
current.next = ListNode(val)
current = current.next
if node.next:
heapq.heappush(heap, (node.next.val, i, node.next))
return dummy.next
# Example usage
# Create some sample linked lists
list1 = ListNode(1, ListNode(4, ListNode(7)))
list2 = ListNode(2, ListNode(5, ListNode(8)))
list3 = ListNode(3, ListNode(6, ListNode(9)))
merged = merge_k_lists([list1, list2, list3])
# Print the merged list
while merged:
print(merged.val, end=' ')
merged = merged.next
# Output: 1 2 3 4 5 6 7 8 9
This implementation demonstrates how the K-way merge algorithm can be adapted to work with linked lists instead of arrays.
8. Interview Tips and Common Questions
K-way merge is a popular topic in technical interviews, especially for positions at major tech companies. Here are some tips and common questions you might encounter:
8.1. Tips for Solving K-way Merge Problems
- Start by clearly stating the problem and clarifying any assumptions.
- Consider edge cases, such as empty input arrays or arrays of different lengths.
- Explain your approach before diving into the implementation.
- Discuss the time and space complexity of your solution.
- If asked to optimize, consider the techniques mentioned earlier in this article.
8.2. Common Interview Questions
- How would you merge K sorted arrays efficiently?
- What data structure would you use to implement K-way merge, and why?
- Can you implement K-way merge without using extra space?
- How would you modify the algorithm to handle very large input arrays that don’t fit in memory?
- What’s the time complexity of your K-way merge implementation? Can you optimize it further?
- How would you parallelize the K-way merge algorithm?
- Can you implement K-way merge for linked lists instead of arrays?
Remember, interviewers are often more interested in your problem-solving approach and ability to communicate your thoughts clearly than in perfect code. Be prepared to discuss trade-offs between different approaches and to analyze the efficiency of your solution.
9. Conclusion
K-way merge is a powerful and versatile algorithm that plays a crucial role in various areas of computer science and software engineering. From external sorting to database operations and parallel computing, understanding and implementing K-way merge efficiently can significantly enhance your ability to handle large-scale data processing tasks.
In this comprehensive guide, we’ve explored the concept of K-way merge, its applications, implementation details, optimization techniques, and variations. We’ve also discussed its time and space complexity and provided tips for tackling K-way merge problems in technical interviews.
As you continue your journey in algorithmic problem-solving and coding education, remember that mastering techniques like K-way merge not only prepares you for technical interviews but also equips you with valuable skills for real-world software development challenges. Practice implementing and optimizing K-way merge in different scenarios, and don’t hesitate to explore its applications in your own projects.
Keep coding, keep learning, and remember that every algorithm you master brings you one step closer to becoming a more proficient and versatile developer. Happy coding!