Mastering the Rabin-Karp Algorithm: A Comprehensive Guide to Efficient String Matching
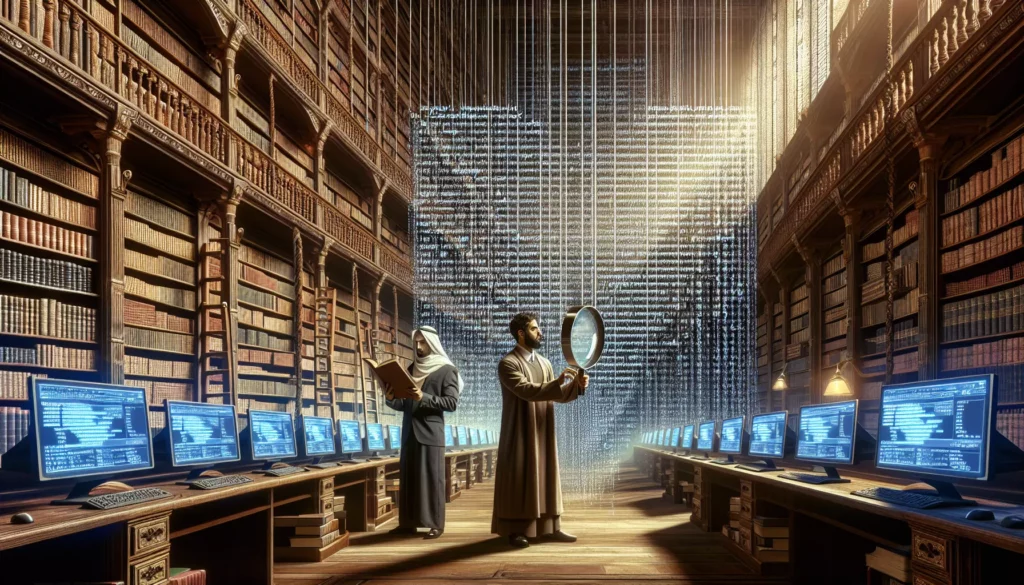
In the vast realm of computer science and programming, efficient string matching algorithms play a crucial role in various applications, from text editors to bioinformatics. Among these algorithms, the Rabin-Karp algorithm stands out as a powerful and elegant solution for pattern searching within larger texts. In this comprehensive guide, we’ll dive deep into the Rabin-Karp algorithm, exploring its mechanics, implementation, and practical applications.
What is the Rabin-Karp Algorithm?
The Rabin-Karp algorithm, developed by Michael O. Rabin and Richard M. Karp in 1987, is a string-searching algorithm that uses hashing to find patterns within a larger text. Unlike naive string matching algorithms that compare characters one by one, Rabin-Karp utilizes a rolling hash function to efficiently identify potential matches, making it particularly effective for multiple pattern searches.
Key Concepts of the Rabin-Karp Algorithm
1. Hashing
At the heart of the Rabin-Karp algorithm lies the concept of hashing. A hash function is used to convert the pattern and substrings of the text into numerical values, allowing for quick comparisons. The algorithm uses a rolling hash, which enables efficient updates of hash values as the algorithm slides through the text.
2. Rolling Hash
The rolling hash is a critical component that allows the algorithm to compute hash values for subsequent substrings in constant time. As the algorithm moves through the text, it updates the hash value by removing the contribution of the first character and adding the contribution of the new character.
3. Modular Arithmetic
To prevent integer overflow and maintain efficiency, the Rabin-Karp algorithm typically uses modular arithmetic. This involves performing calculations with a large prime number as the modulus, ensuring that hash values remain within a manageable range.
How the Rabin-Karp Algorithm Works
Let’s break down the steps of the Rabin-Karp algorithm:
- Calculate the hash value of the pattern.
- Calculate the hash value of the first substring of the text with the same length as the pattern.
- Compare the hash values. If they match, perform a character-by-character comparison to confirm the match.
- If no match is found, slide the window by one character and update the hash value using the rolling hash technique.
- Repeat steps 3-4 until the end of the text is reached or all occurrences of the pattern are found.
Implementing the Rabin-Karp Algorithm
Now, let’s implement the Rabin-Karp algorithm in Python. We’ll start with a basic implementation and then optimize it for better performance.
Basic Implementation
def rabin_karp(text, pattern):
n = len(text)
m = len(pattern)
prime = 101 # A prime number for modular arithmetic
d = 256 # Number of characters in the input alphabet
# Calculate hash value for pattern and first window of text
pattern_hash = 0
text_hash = 0
h = 1
# The value of h would be "pow(d, m-1) % prime"
for i in range(m - 1):
h = (h * d) % prime
# Calculate the hash value of pattern and first window of text
for i in range(m):
pattern_hash = (d * pattern_hash + ord(pattern[i])) % prime
text_hash = (d * text_hash + ord(text[i])) % prime
# Slide the pattern over text one by one
for i in range(n - m + 1):
# Check the hash values of current window of text and pattern
if pattern_hash == text_hash:
# If the hash values match, check for characters one by one
if text[i:i+m] == pattern:
print(f"Pattern found at index {i}")
# Calculate hash value for next window of text
if i < n - m:
text_hash = (d * (text_hash - ord(text[i]) * h) + ord(text[i + m])) % prime
# We might get negative value of text_hash, converting it to positive
if text_hash < 0:
text_hash += prime
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
rabin_karp(text, pattern)
This implementation demonstrates the core principles of the Rabin-Karp algorithm. It calculates hash values for the pattern and text windows, compares them, and performs character-by-character verification when hash values match.
Optimized Implementation
We can optimize the algorithm further by using a more efficient rolling hash function and handling multiple pattern searches. Here’s an improved version:
class RabinKarp:
def __init__(self, patterns):
self.patterns = patterns
self.prime = 101
self.d = 256
self.pattern_hashes = {}
self.calculate_pattern_hashes()
def calculate_pattern_hashes(self):
for pattern in self.patterns:
m = len(pattern)
pattern_hash = 0
for i in range(m):
pattern_hash = (self.d * pattern_hash + ord(pattern[i])) % self.prime
self.pattern_hashes[pattern_hash] = pattern
def search(self, text):
n = len(text)
m = max(len(pattern) for pattern in self.patterns)
h = pow(self.d, m - 1, self.prime)
text_hash = 0
results = []
# Calculate initial hash for text window
for i in range(m):
text_hash = (self.d * text_hash + ord(text[i])) % self.prime
# Slide the pattern over text one by one
for i in range(n - m + 1):
if text_hash in self.pattern_hashes:
pattern = self.pattern_hashes[text_hash]
if text[i:i+len(pattern)] == pattern:
results.append((pattern, i))
# Calculate hash value for next window of text
if i < n - m:
text_hash = (self.d * (text_hash - ord(text[i]) * h) + ord(text[i + m])) % self.prime
return results
# Example usage
text = "ABABDABACDABABCABAB"
patterns = ["ABABCABAB", "ABAB", "BAB"]
rk = RabinKarp(patterns)
matches = rk.search(text)
for pattern, index in matches:
print(f"Pattern '{pattern}' found at index {index}")
This optimized version introduces several improvements:
- It handles multiple patterns simultaneously, making it more efficient for scenarios where you need to search for multiple strings in the same text.
- The rolling hash function is implemented more efficiently, reducing the number of modulo operations.
- The code is structured as a class, making it easier to reuse and extend.
Time and Space Complexity
Understanding the time and space complexity of the Rabin-Karp algorithm is crucial for assessing its performance in different scenarios.
Time Complexity
- Average and Best Case: O(n + m), where n is the length of the text and m is the length of the pattern. This occurs when there are few matches or when the hash function distributes values uniformly.
- Worst Case: O(nm), which happens when all hash values match, but the strings are different, requiring a character-by-character comparison each time.
Space Complexity
The space complexity of the Rabin-Karp algorithm is O(1) for single pattern search, as it only needs to store a constant amount of data regardless of the input size. For multiple pattern search, as implemented in our optimized version, the space complexity becomes O(k), where k is the number of patterns, due to the storage of pattern hashes.
Advantages and Disadvantages
Advantages
- Efficient for multiple pattern search: The Rabin-Karp algorithm shines when searching for multiple patterns simultaneously.
- Good average-case performance: In practice, the algorithm often performs well, especially with a good hash function.
- Suitable for string matching in streaming data: The rolling hash allows for efficient updates as new characters arrive.
Disadvantages
- Worst-case performance: In scenarios where hash collisions are frequent, the algorithm can degrade to O(nm) time complexity.
- Sensitivity to hash function quality: The effectiveness of the algorithm heavily depends on the choice of hash function.
- Not the best for single pattern search: For single pattern searches, algorithms like KMP or Boyer-Moore might be more efficient.
Practical Applications
The Rabin-Karp algorithm finds applications in various domains:
1. Plagiarism Detection
Rabin-Karp is often used in plagiarism detection systems to identify matching text segments across multiple documents efficiently.
2. Bioinformatics
In DNA sequence analysis, Rabin-Karp can be employed to find specific patterns or motifs within long genetic sequences.
3. Network Security
Intrusion detection systems may use Rabin-Karp to scan network packets for known malicious patterns or signatures.
4. Data Deduplication
The algorithm can be adapted for finding duplicate data chunks in storage systems, aiding in data compression and efficient storage utilization.
Optimizing Rabin-Karp for Real-World Scenarios
When implementing Rabin-Karp for real-world applications, consider the following optimizations:
1. Choose an Appropriate Hash Function
The choice of hash function significantly impacts the algorithm’s performance. Consider using cryptographic hash functions for added security in sensitive applications.
2. Implement Parallel Processing
For large-scale text processing, implement parallel versions of Rabin-Karp to leverage multi-core processors or distributed systems.
3. Optimize for Memory Usage
In memory-constrained environments, consider streaming implementations that process the text in chunks rather than loading it entirely into memory.
4. Combine with Other Algorithms
For complex string matching tasks, consider hybrid approaches that combine Rabin-Karp with other algorithms like Aho-Corasick for multi-pattern matching or suffix trees for repeated pattern searches.
Rabin-Karp in Coding Interviews
Understanding and implementing the Rabin-Karp algorithm can be valuable in coding interviews, especially for positions that involve text processing or pattern matching. Here are some tips for discussing Rabin-Karp in an interview setting:
- Explain the core concept: Be prepared to explain the fundamental idea of using hashing for efficient string matching.
- Discuss time and space complexity: Demonstrate your understanding of the algorithm’s performance characteristics in different scenarios.
- Compare with other algorithms: Be ready to compare Rabin-Karp with other string matching algorithms like KMP or Boyer-Moore.
- Implement the algorithm: Practice implementing Rabin-Karp from scratch, as you might be asked to code it during the interview.
- Discuss optimizations: Show your problem-solving skills by suggesting potential optimizations for specific use cases.
Conclusion
The Rabin-Karp algorithm is a powerful tool in the string matching arsenal, offering efficient solutions for various text processing challenges. Its clever use of hashing and rolling computation makes it particularly suited for multiple pattern searches and scenarios where pattern matching needs to be performed on streaming data.
As we’ve explored in this comprehensive guide, mastering Rabin-Karp involves understanding its core principles, implementing it efficiently, and recognizing its strengths and limitations. By grasping these concepts and practicing their application, you’ll be well-equipped to tackle complex string matching problems in both real-world applications and coding interviews.
Remember, the key to truly mastering algorithms like Rabin-Karp lies not just in memorizing their implementation, but in understanding their underlying principles and being able to adapt them to various problem contexts. As you continue your journey in algorithm design and coding education, keep exploring, practicing, and challenging yourself with diverse string matching scenarios to solidify your skills and intuition.
Happy coding, and may your string matching adventures be efficient and collision-free!