Breaking Down Complex Problems into Simple Steps: A Comprehensive Guide for Programmers
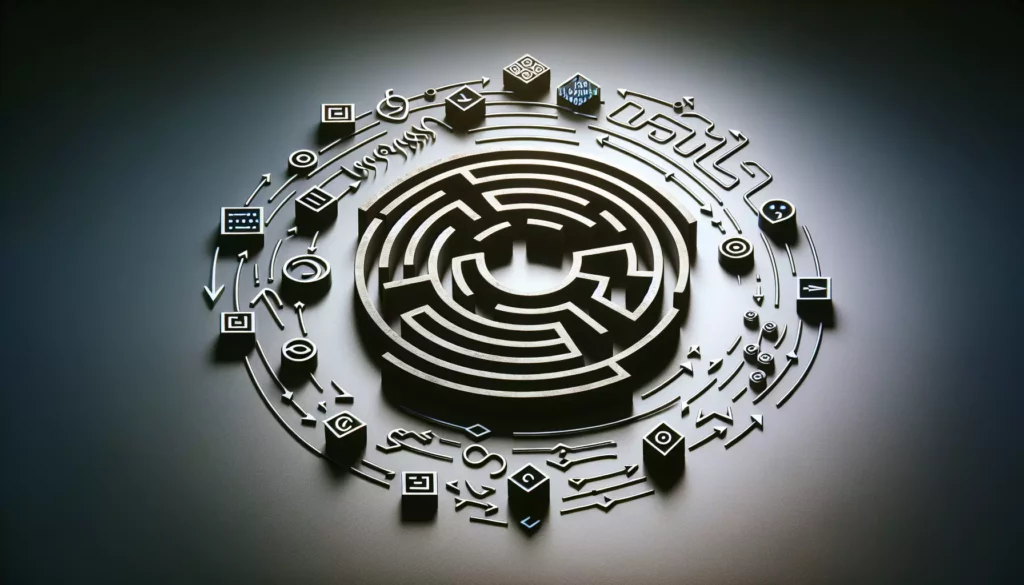
In the world of programming and software development, tackling complex problems is an everyday challenge. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, the ability to break down complex problems into manageable steps is a crucial skill. This comprehensive guide will explore the art of problem decomposition, providing you with practical strategies and techniques to approach even the most daunting coding challenges with confidence.
Why Breaking Down Problems Matters
Before we dive into the specific techniques, let’s understand why breaking down problems is so important:
- Manageability: Large, complex problems can be overwhelming. Breaking them down makes them more approachable and less intimidating.
- Clarity: Decomposing a problem helps you understand its components better, leading to clearer thinking and more effective solutions.
- Modularity: Breaking problems into smaller parts often results in more modular code, which is easier to maintain, test, and debug.
- Collaboration: When working in teams, breaking down problems allows for better task distribution and parallel development.
- Problem-solving skills: Regularly practicing this approach enhances your overall problem-solving abilities, a key skill for technical interviews and real-world programming challenges.
The Problem-Solving Framework
To effectively break down complex problems, it’s helpful to follow a structured approach. Here’s a framework you can use:
- Understand the problem
- Identify the inputs and outputs
- Break the problem into smaller subproblems
- Solve each subproblem
- Combine the solutions
- Optimize and refine
Let’s explore each step in detail.
1. Understand the Problem
Before you start coding or even breaking down the problem, it’s crucial to fully understand what you’re trying to solve. This step involves:
- Reading the problem statement carefully
- Identifying the key requirements and constraints
- Asking clarifying questions if anything is unclear
- Restating the problem in your own words to ensure comprehension
For example, let’s say you’re given this problem: “Implement a function to find the longest palindromic substring in a given string.”
You might restate it as: “I need to write a function that takes a string as input, examines all possible substrings within it, identifies which of these substrings are palindromes, and returns the longest one.”
2. Identify the Inputs and Outputs
Clearly defining what goes into your function and what should come out is a crucial step. For our palindrome example:
- Input: A string of characters
- Output: The longest palindromic substring within the input string
Understanding the input and output helps you focus on the transformation that needs to occur and can often suggest potential approaches or data structures to use.
3. Break the Problem into Smaller Subproblems
This is where the real decomposition happens. Look at the overall problem and identify smaller, more manageable tasks that, when solved, will contribute to the overall solution. For our palindrome problem, we might break it down like this:
- Generate all possible substrings of the input string
- Check if a given substring is a palindrome
- Keep track of the longest palindromic substring found so far
- Return the longest palindromic substring
Each of these subproblems is simpler than the original problem and can be tackled independently.
4. Solve Each Subproblem
Now that you have smaller, more manageable tasks, you can focus on solving each one. Let’s look at how we might approach each subproblem for our palindrome example:
Generate all possible substrings:
def generate_substrings(s):
substrings = []
for i in range(len(s)):
for j in range(i + 1, len(s) + 1):
substrings.append(s[i:j])
return substrings
Check if a substring is a palindrome:
def is_palindrome(s):
return s == s[::-1]
Keep track of the longest palindrome:
longest_palindrome = ""
for substring in substrings:
if is_palindrome(substring) and len(substring) > len(longest_palindrome):
longest_palindrome = substring
5. Combine the Solutions
Once you’ve solved each subproblem, it’s time to bring everything together into a complete solution. Here’s how our palindrome function might look:
def longest_palindromic_substring(s):
def generate_substrings(s):
substrings = []
for i in range(len(s)):
for j in range(i + 1, len(s) + 1):
substrings.append(s[i:j])
return substrings
def is_palindrome(s):
return s == s[::-1]
substrings = generate_substrings(s)
longest_palindrome = ""
for substring in substrings:
if is_palindrome(substring) and len(substring) > len(longest_palindrome):
longest_palindrome = substring
return longest_palindrome
6. Optimize and Refine
The final step is to look for ways to improve your solution. This might involve:
- Optimizing for time or space complexity
- Simplifying the code
- Handling edge cases
- Adding error checking
In our palindrome example, we could optimize by avoiding the generation of all substrings and instead expanding around potential centers of palindromes:
def longest_palindromic_substring(s):
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return s[left + 1:right]
longest = ""
for i in range(len(s)):
# Odd length palindromes
palindrome = expand_around_center(i, i)
if len(palindrome) > len(longest):
longest = palindrome
# Even length palindromes
palindrome = expand_around_center(i, i + 1)
if len(palindrome) > len(longest):
longest = palindrome
return longest
This optimized version has a time complexity of O(n^2) instead of O(n^3), making it much more efficient for larger inputs.
Advanced Techniques for Breaking Down Problems
As you become more comfortable with breaking down problems, you can employ more advanced techniques to tackle even more complex challenges:
Divide and Conquer
This technique involves breaking a problem into two or more sub-problems of the same or related type, solving these sub-problems recursively, and then combining their results to solve the original problem. It’s particularly useful for problems that exhibit optimal substructure.
Example: Merge Sort algorithm
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It is applicable when the problem has overlapping subproblems and optimal substructure.
Example: Fibonacci sequence with memoization
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step with the hope of finding a global optimum. They don’t always yield the best solution, but when they do, they’re usually very efficient.
Example: Coin change problem (assuming coins of denominations that allow for a greedy approach)
def coin_change(amount, coins):
coins.sort(reverse=True)
change = []
for coin in coins:
while amount >= coin:
change.append(coin)
amount -= coin
return change
Problem-Solving Strategies for Technical Interviews
When preparing for technical interviews, especially for major tech companies, it’s crucial to not only know how to break down problems but also how to communicate your thought process effectively. Here are some strategies to keep in mind:
Think Aloud
As you work through the problem, verbalize your thoughts. This gives the interviewer insight into your problem-solving approach and allows them to provide hints if needed.
Start with a Brute Force Solution
Begin by describing or implementing the simplest solution that comes to mind, even if it’s not optimal. This demonstrates that you can at least solve the problem, and it provides a starting point for optimization.
Analyze Time and Space Complexity
After proposing a solution, discuss its time and space complexity. This shows that you’re thinking about efficiency and scalability.
Consider Edge Cases
Before finalizing your solution, think about potential edge cases and how your code would handle them. This demonstrates attention to detail and thoroughness.
Optimize Incrementally
If your initial solution isn’t optimal, discuss potential optimizations step by step. This shows your ability to iterate and improve on your solutions.
Tools and Resources for Problem Decomposition
To further enhance your problem-solving skills, consider using these tools and resources:
Pseudocode
Writing pseudocode before actual code can help you organize your thoughts and break down the problem without getting bogged down in syntax details.
Flowcharts and Diagrams
Visual representations of your algorithm can help you see the big picture and identify subproblems more easily. Tools like draw.io or Lucidchart can be useful for this.
Test-Driven Development (TDD)
Writing tests before implementing the solution can help you break down the problem into testable units and ensure each part of your solution works correctly.
Online Coding Platforms
Websites like LeetCode, HackerRank, and CodeSignal offer a wide range of coding problems to practice on, often with built-in testing and complexity analysis tools.
AI-Powered Coding Assistants
Tools like GitHub Copilot or AlgoCademy’s AI assistant can provide suggestions and help you break down problems, but remember to understand the solutions they propose rather than relying on them blindly.
Conclusion
Breaking down complex problems into simple steps is a fundamental skill for any programmer, whether you’re just starting out or preparing for technical interviews at top tech companies. By following a structured approach, employing advanced techniques when appropriate, and utilizing helpful tools and resources, you can tackle even the most challenging coding problems with confidence.
Remember, the key to mastering this skill is practice. Start with simpler problems and gradually work your way up to more complex ones. As you do, you’ll find that your ability to decompose and solve problems improves, making you a more effective and efficient programmer.
Whether you’re using AlgoCademy’s interactive tutorials, preparing for FAANG interviews, or working on your own projects, always take the time to break down the problem before diving into code. With patience and persistence, you’ll develop the problem-solving skills that are so highly valued in the world of software development.