Mastering Prefix Sum: A Powerful Technique for Efficient Array Computations
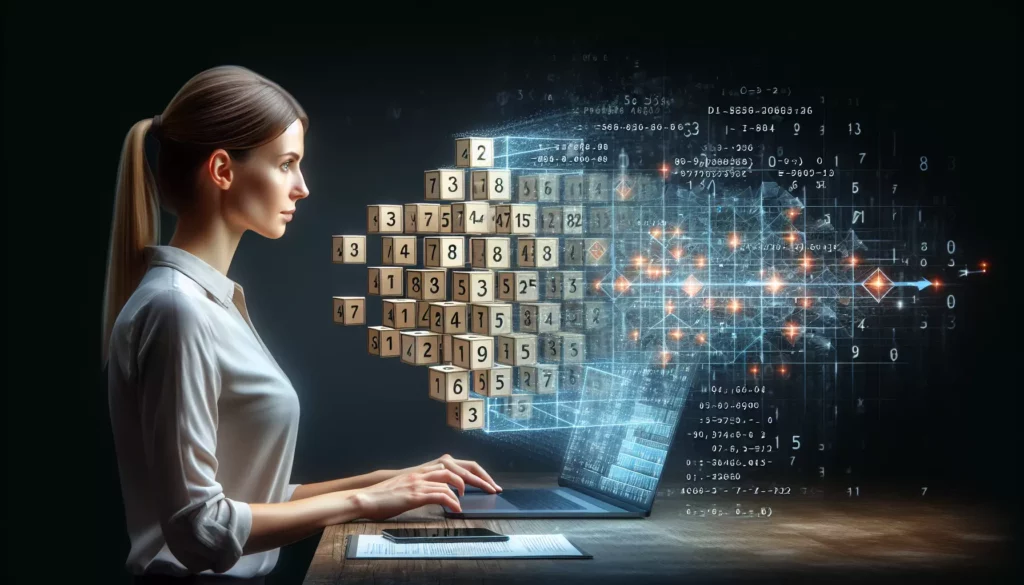
In the world of competitive programming and technical interviews, efficiency is key. One technique that consistently proves its worth is the Prefix Sum, also known as Cumulative Sum. This powerful algorithmic tool can significantly optimize certain types of array computations, making it an essential skill for any aspiring programmer or computer scientist. In this comprehensive guide, we’ll dive deep into the concept of Prefix Sum, explore its applications, and learn how to implement it effectively.
What is Prefix Sum?
Prefix Sum is a technique used to calculate the running total of a list of numbers. For any given array, the prefix sum at index i is the sum of all elements from index 0 to i, inclusive. This pre-computation allows for constant-time queries of sum ranges within the array, which can be incredibly useful in various problem-solving scenarios.
Mathematically, for an array A of n elements, the prefix sum array P is defined as:
P[i] = A[0] + A[1] + A[2] + ... + A[i]
Where P[i] represents the sum of all elements from index 0 to i in the original array A.
Why Use Prefix Sum?
The primary advantage of using Prefix Sum is its ability to reduce the time complexity of certain operations from O(n) to O(1). This is particularly useful when dealing with multiple queries on the same array. Instead of recalculating the sum for each query, we can pre-compute the prefix sum once and then use it to answer queries in constant time.
Implementing Prefix Sum
Let’s look at how to implement a basic Prefix Sum algorithm in Python:
def calculate_prefix_sum(arr):
n = len(arr)
prefix_sum = [0] * n
prefix_sum[0] = arr[0]
for i in range(1, n):
prefix_sum[i] = prefix_sum[i-1] + arr[i]
return prefix_sum
# Example usage
arr = [1, 2, 3, 4, 5]
prefix_sum = calculate_prefix_sum(arr)
print(prefix_sum) # Output: [1, 3, 6, 10, 15]
In this implementation, we create a new array prefix_sum
of the same length as the input array. We initialize the first element of prefix_sum
with the first element of the input array. Then, we iterate through the rest of the array, calculating each prefix sum by adding the current element to the previous prefix sum.
Applications of Prefix Sum
Prefix Sum has numerous applications in various problem-solving scenarios. Let’s explore some of the most common ones:
1. Range Sum Queries
One of the most straightforward applications of Prefix Sum is in answering range sum queries efficiently. Given a range [L, R], we can calculate the sum of elements from index L to R using the following formula:
sum(L, R) = prefix_sum[R] - prefix_sum[L-1] (if L > 0)
sum(L, R) = prefix_sum[R] (if L == 0)
Here’s a Python implementation:
def range_sum(prefix_sum, L, R):
if L == 0:
return prefix_sum[R]
return prefix_sum[R] - prefix_sum[L-1]
# Example usage
arr = [1, 2, 3, 4, 5]
prefix_sum = calculate_prefix_sum(arr)
print(range_sum(prefix_sum, 1, 3)) # Output: 9 (2 + 3 + 4)
2. Finding Equilibrium Index
An equilibrium index of an array is an index where the sum of all elements to the left is equal to the sum of all elements to the right. Prefix Sum can be used to efficiently find such an index:
def find_equilibrium_index(arr):
total_sum = sum(arr)
left_sum = 0
for i in range(len(arr)):
if left_sum == total_sum - left_sum - arr[i]:
return i
left_sum += arr[i]
return -1 # No equilibrium index found
# Example usage
arr = [-7, 1, 5, 2, -4, 3, 0]
print(find_equilibrium_index(arr)) # Output: 3
3. Count of Subarrays with Given Sum
Prefix Sum can be used to efficiently count the number of subarrays with a given sum. Here’s an implementation:
from collections import defaultdict
def count_subarrays_with_sum(arr, target_sum):
count = 0
prefix_sum = 0
sum_count = defaultdict(int)
sum_count[0] = 1
for num in arr:
prefix_sum += num
count += sum_count[prefix_sum - target_sum]
sum_count[prefix_sum] += 1
return count
# Example usage
arr = [3, 4, 7, 2, -3, 1, 4, 2]
target_sum = 7
print(count_subarrays_with_sum(arr, target_sum)) # Output: 4
Advanced Applications of Prefix Sum
While the basic concept of Prefix Sum is straightforward, it can be extended and combined with other techniques to solve more complex problems. Let’s explore some advanced applications:
1. 2D Prefix Sum
The concept of Prefix Sum can be extended to 2D arrays, which is particularly useful for efficient range sum queries on matrices. Here’s how to implement a 2D Prefix Sum:
def calculate_2d_prefix_sum(matrix):
m, n = len(matrix), len(matrix[0])
prefix_sum = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
prefix_sum[i][j] = (prefix_sum[i-1][j] + prefix_sum[i][j-1]
- prefix_sum[i-1][j-1] + matrix[i-1][j-1])
return prefix_sum
def range_sum_2d(prefix_sum, r1, c1, r2, c2):
return (prefix_sum[r2+1][c2+1] - prefix_sum[r1][c2+1]
- prefix_sum[r2+1][c1] + prefix_sum[r1][c1])
# Example usage
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
prefix_sum_2d = calculate_2d_prefix_sum(matrix)
print(range_sum_2d(prefix_sum_2d, 0, 0, 1, 1)) # Output: 12 (1 + 2 + 4 + 5)
2. Difference Array
The Difference Array is a technique closely related to Prefix Sum. It’s particularly useful when dealing with range update operations. The idea is to maintain an array of differences between consecutive elements. Here’s an implementation:
def create_difference_array(arr):
n = len(arr)
diff = [0] * (n + 1)
diff[0] = arr[0]
for i in range(1, n):
diff[i] = arr[i] - arr[i-1]
return diff
def range_update(diff, l, r, value):
diff[l] += value
diff[r + 1] -= value
def reconstruct_array(diff):
n = len(diff)
arr = [0] * n
arr[0] = diff[0]
for i in range(1, n):
arr[i] = arr[i-1] + diff[i]
return arr
# Example usage
arr = [1, 2, 3, 4, 5]
diff = create_difference_array(arr)
range_update(diff, 1, 3, 2) # Add 2 to elements at indices 1, 2, and 3
updated_arr = reconstruct_array(diff)
print(updated_arr) # Output: [1, 4, 5, 6, 5]
3. Prefix XOR
The concept of Prefix Sum can be extended to other operations, such as XOR. This is particularly useful in problems involving XOR operations over ranges. Here’s an implementation of Prefix XOR:
def calculate_prefix_xor(arr):
n = len(arr)
prefix_xor = [0] * n
prefix_xor[0] = arr[0]
for i in range(1, n):
prefix_xor[i] = prefix_xor[i-1] ^ arr[i]
return prefix_xor
def range_xor(prefix_xor, L, R):
if L == 0:
return prefix_xor[R]
return prefix_xor[R] ^ prefix_xor[L-1]
# Example usage
arr = [1, 3, 4, 8]
prefix_xor = calculate_prefix_xor(arr)
print(range_xor(prefix_xor, 1, 3)) # Output: 7 (3 ^ 4 ^ 8)
Time and Space Complexity Analysis
Understanding the time and space complexity of Prefix Sum operations is crucial for effectively using this technique:
Time Complexity:
- Calculating Prefix Sum: O(n), where n is the length of the array
- Range Sum Query: O(1)
- 2D Prefix Sum Calculation: O(m * n), where m and n are the dimensions of the matrix
- 2D Range Sum Query: O(1)
Space Complexity:
- 1D Prefix Sum: O(n)
- 2D Prefix Sum: O(m * n)
The trade-off in using Prefix Sum is clear: we invest in additional space and preprocessing time to achieve constant-time query operations. This makes Prefix Sum particularly effective when dealing with multiple queries on the same array or matrix.
Common Pitfalls and How to Avoid Them
While Prefix Sum is a powerful technique, there are some common mistakes that programmers might encounter:
1. Off-by-One Errors
When calculating range sums, it’s easy to make off-by-one errors. Always double-check your index calculations, especially when dealing with zero-based indexing.
2. Integer Overflow
For large arrays or long sequences of operations, integer overflow can occur. Consider using long integers or implementing modular arithmetic if necessary.
3. Not Considering Negative Numbers
Some Prefix Sum applications assume all numbers are positive. Be cautious when dealing with arrays that might contain negative numbers, as it can affect certain algorithms (e.g., finding subarrays with a given sum).
4. Forgetting to Handle Edge Cases
Always consider edge cases, such as empty arrays or queries that start from index 0.
Practice Problems
To solidify your understanding of Prefix Sum, here are some practice problems you can try:
- LeetCode: Range Sum Query – Immutable
- LeetCode: Subarray Sum Equals K
- SPOJ: Cumulative Sum Query
- Codeforces: Good Subarrays
- CodeChef: Blondie
Conclusion
Prefix Sum is a versatile and powerful technique that can significantly optimize certain types of array computations. By pre-computing cumulative sums, we can achieve constant-time range sum queries and solve a variety of problems more efficiently. As you’ve seen, the basic concept can be extended to 2D arrays, difference arrays, and even other operations like XOR.
Mastering Prefix Sum and understanding its applications will not only help you in competitive programming and technical interviews but also in real-world scenarios where efficient array manipulations are required. As with any algorithmic technique, practice is key to fully grasping and applying Prefix Sum effectively.
Remember to always consider the trade-offs between time and space complexity when deciding to use Prefix Sum, and be mindful of common pitfalls like off-by-one errors and integer overflow. With continued practice and application, Prefix Sum will become a valuable tool in your problem-solving arsenal.