Mastering Whiteboard Interviews: A Comprehensive Guide to Success
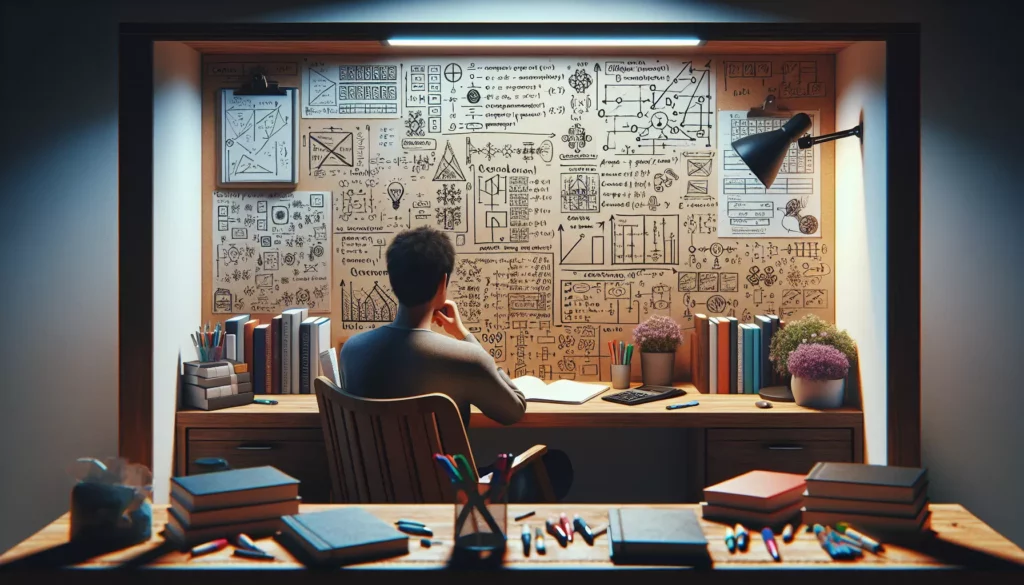
In the competitive world of tech hiring, whiteboard interviews have become a staple of the recruitment process, especially for software engineering positions. These interviews, which often involve solving complex coding problems on a whiteboard in real-time, can be daunting for even the most experienced programmers. However, with the right preparation and mindset, you can transform this challenge into an opportunity to showcase your skills and land your dream job. In this comprehensive guide, we’ll explore everything you need to know about preparing for and excelling in whiteboard interviews.
Understanding Whiteboard Interviews
Before diving into preparation strategies, it’s crucial to understand what whiteboard interviews are and why companies use them. A whiteboard interview typically involves the candidate solving a coding problem or algorithm on a whiteboard (or sometimes a shared online document) while explaining their thought process to the interviewer.
The primary goals of whiteboard interviews are to:
- Assess problem-solving skills
- Evaluate coding ability
- Gauge communication skills
- Observe how candidates handle pressure
- Test algorithmic thinking and optimization skills
While controversial in some circles, many tech giants, including FAANG companies (Facebook, Amazon, Apple, Netflix, and Google), continue to use this interview format as part of their hiring process.
Essential Preparation Strategies
1. Master the Fundamentals
Before you can tackle complex problems, ensure you have a solid grasp of computer science fundamentals. This includes:
- Data Structures (arrays, linked lists, trees, graphs, hash tables)
- Algorithms (sorting, searching, traversal, dynamic programming)
- Time and Space Complexity Analysis
- Object-Oriented Programming concepts
Platforms like AlgoCademy offer structured courses and interactive tutorials to help you brush up on these fundamentals.
2. Practice, Practice, Practice
Consistent practice is key to improving your problem-solving skills and building confidence. Here are some ways to practice:
- Solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal
- Participate in coding competitions
- Implement data structures and algorithms from scratch
- Work through problems in coding interview books
AlgoCademy’s AI-powered assistance can provide personalized problem recommendations based on your skill level and areas for improvement.
3. Mock Interviews
Simulating the interview environment is crucial for success. Consider the following approaches:
- Practice with a friend or colleague
- Use online mock interview platforms
- Record yourself solving problems and review your performance
- Participate in coding bootcamps or interview prep programs
These mock interviews will help you get comfortable with explaining your thought process out loud and working under time pressure.
4. Learn to Communicate Effectively
Clear communication is as important as your coding skills during a whiteboard interview. Practice the following:
- Explaining your approach before starting to code
- Thinking out loud as you work through the problem
- Asking clarifying questions about the problem statement
- Discussing trade-offs between different solutions
5. Study Common Interview Topics
While you can’t predict exactly what you’ll be asked, certain topics come up frequently in whiteboard interviews. Focus on:
- String manipulation
- Array and matrix operations
- Tree and graph algorithms
- Dynamic programming
- Bit manipulation
- System design (for more senior positions)
Whiteboard Interview Best Practices
1. Start with Clarifying Questions
Before diving into the solution, make sure you fully understand the problem. Ask questions about:
- Input format and constraints
- Expected output
- Edge cases to consider
- Any assumptions you can make
This demonstrates your analytical skills and helps you avoid solving the wrong problem.
2. Plan Before You Code
Resist the urge to start coding immediately. Instead:
- Discuss your high-level approach
- Consider multiple solutions and their trade-offs
- Sketch out a rough algorithm or flowchart
This planning phase shows your ability to think strategically and consider different angles.
3. Write Clean, Readable Code
Even on a whiteboard, code quality matters. Focus on:
- Using meaningful variable and function names
- Maintaining consistent indentation
- Breaking down complex logic into smaller functions
- Adding comments for clarity (but don’t overdo it)
4. Test Your Solution
Once you’ve written your code, don’t forget to test it. This involves:
- Walking through the code with a simple example
- Considering edge cases and how your code handles them
- Discussing the time and space complexity of your solution
This step demonstrates your attention to detail and commitment to producing reliable code.
5. Be Open to Feedback
If the interviewer provides hints or suggests improvements:
- Listen actively and show appreciation for the input
- Incorporate the feedback into your solution
- Use it as an opportunity to demonstrate your ability to collaborate and learn quickly
Common Whiteboard Interview Mistakes to Avoid
1. Jumping into Coding Too Quickly
Take the time to understand the problem and plan your approach. Rushing can lead to errors and inefficient solutions.
2. Staying Silent
Remember to communicate throughout the process. If you’re stuck, verbalize your thoughts and ask for clarification if needed.
3. Ignoring Time and Space Complexity
Always consider the efficiency of your solution. Be prepared to discuss Big O notation and potential optimizations.
4. Not Managing Time Effectively
Keep an eye on the clock. If you’re spending too much time on one part of the problem, consider moving on and coming back if time allows.
5. Giving Up Too Easily
If you’re struggling, don’t lose hope. Show perseverance and problem-solving skills by breaking down the problem into smaller parts or considering alternative approaches.
Advanced Techniques for Whiteboard Interviews
1. Pattern Recognition
As you practice more problems, you’ll start to recognize common patterns. Some key patterns to look out for include:
- Two-pointer technique
- Sliding window
- Divide and conquer
- Backtracking
- Dynamic programming
Being able to quickly identify these patterns can help you solve problems more efficiently.
2. Space-Time Tradeoffs
Often, there’s a tradeoff between time complexity and space complexity. Be prepared to discuss these tradeoffs and choose the most appropriate solution based on the constraints of the problem.
3. Handling Ambiguity
Sometimes, interviewers intentionally leave parts of the problem ambiguous. This is an opportunity to demonstrate your ability to:
- Ask insightful questions
- Make and articulate reasonable assumptions
- Adapt your solution as new information is provided
4. Optimizing Your Solution
After implementing a working solution, consider how you might optimize it. This could involve:
- Using more efficient data structures
- Eliminating unnecessary operations
- Applying specific algorithmic techniques (e.g., memoization in dynamic programming)
5. System Design Considerations
For more senior positions, you might be asked about system design. Be prepared to discuss:
- Scalability
- Load balancing
- Database design
- Caching strategies
- Microservices architecture
Sample Whiteboard Interview Problem and Solution
Let’s walk through a common whiteboard interview problem and how you might approach it:
Problem: Reverse a Linked List
Given the head of a singly linked list, reverse the list and return the reversed list.
Step 1: Clarify the Problem
Ask questions like:
- Is it a singly linked list or doubly linked list?
- Should I reverse it in-place or create a new list?
- What should I do if the list is empty or has only one node?
Step 2: Plan the Approach
Explain your strategy:
“I’ll use three pointers to reverse the list in-place: one for the previous node, one for the current node, and one for the next node. I’ll iterate through the list, reversing the links as I go.”
Step 3: Implement the Solution
Write the code on the whiteboard:
public ListNode reverseList(ListNode head) {
ListNode prev = null;
ListNode current = head;
ListNode next = null;
while (current != null) {
next = current.next;
current.next = prev;
prev = current;
current = next;
}
return prev;
}
Step 4: Explain Your Code
Walk through your code, explaining each step:
“We start with ‘prev’ as null and ‘current’ as the head. In each iteration, we store the next node, reverse the current node’s pointer, move prev and current one step forward, and continue until we reach the end of the list.”
Step 5: Analyze Time and Space Complexity
Discuss the efficiency of your solution:
“This solution has a time complexity of O(n) where n is the number of nodes in the list, as we traverse the list once. The space complexity is O(1) as we only use a constant amount of extra space regardless of the input size.”
Step 6: Test Your Solution
Walk through an example:
“Let’s test this with a simple list: 1 -> 2 -> 3 -> null. After running our function, it should become 3 -> 2 -> 1 -> null.”
Conclusion
Whiteboard interviews can be challenging, but with proper preparation and practice, you can approach them with confidence. Remember that the process is as important as the final solution – interviewers want to see how you think, communicate, and handle pressure.
Platforms like AlgoCademy offer valuable resources for honing your skills, from interactive coding tutorials to AI-powered problem recommendations. By mastering the fundamentals, practicing regularly, and following the strategies outlined in this guide, you’ll be well-equipped to tackle any whiteboard interview that comes your way.
Remember, the key to success lies not just in solving the problem, but in demonstrating your problem-solving approach, communication skills, and ability to write clean, efficient code. With dedication and the right mindset, you can turn the whiteboard interview from a daunting challenge into an opportunity to showcase your talents and land your dream job in the tech industry.