Hash Map and Hash Set: Powerful Data Structures for Efficient Coding
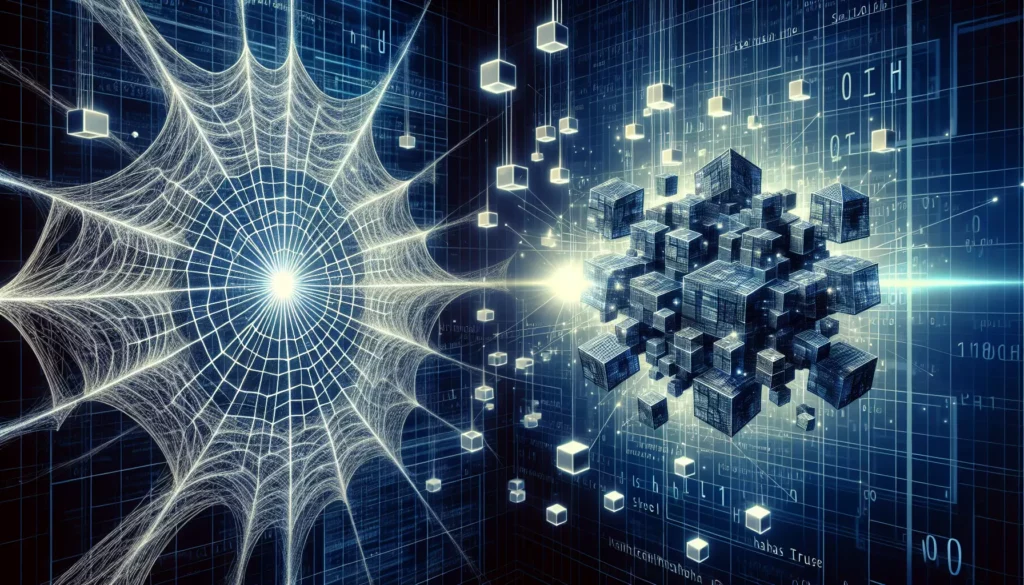
In the world of computer programming and algorithm design, efficient data structures play a crucial role in optimizing performance and solving complex problems. Two such powerful data structures that every programmer should be familiar with are Hash Maps and Hash Sets. These structures are fundamental to many algorithms and are frequently used in technical interviews, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google).
In this comprehensive guide, we’ll dive deep into Hash Maps and Hash Sets, exploring their concepts, implementations, use cases, and how they can be leveraged to solve various coding problems efficiently. Whether you’re a beginner looking to strengthen your programming foundation or an experienced developer preparing for technical interviews, this article will provide valuable insights and practical examples to enhance your understanding of these essential data structures.
Table of Contents
- Understanding Hash Maps
- Implementing Hash Maps
- Hash Map Operations
- Hash Map Use Cases
- Understanding Hash Sets
- Implementing Hash Sets
- Hash Set Operations
- Hash Set Use Cases
- Comparing Hash Maps and Hash Sets
- Performance Considerations
- Common Interview Questions
- Best Practices and Tips
- Conclusion
1. Understanding Hash Maps
A Hash Map, also known as a Hash Table or Dictionary in some programming languages, is a data structure that implements an associative array abstract data type. It allows you to store key-value pairs and provides efficient lookup, insertion, and deletion operations.
The core idea behind a Hash Map is the use of a hash function to compute an index into an array of buckets or slots, from which the desired value can be found. This process, known as hashing, allows for constant-time average complexity for basic operations, making Hash Maps extremely efficient for many tasks.
Key Characteristics of Hash Maps:
- Key-Value Pairs: Each element in a Hash Map consists of a key and its associated value.
- Unique Keys: Keys in a Hash Map must be unique, but values can be duplicated.
- Fast Access: Provides constant-time average complexity for basic operations (O(1)).
- Dynamic Size: Can grow or shrink as needed, depending on the number of elements.
- Unordered: Elements are not stored in any particular order.
2. Implementing Hash Maps
While most programming languages provide built-in implementations of Hash Maps, understanding how to implement one from scratch is crucial for mastering the concept and excelling in technical interviews. Let’s look at a basic implementation of a Hash Map in Python:
class HashMap:
def __init__(self, size=100):
self.size = size
self.map = [[] for _ in range(self.size)]
def _get_hash(self, key):
return hash(key) % self.size
def add(self, key, value):
key_hash = self._get_hash(key)
key_value = [key, value]
if self.map[key_hash] is None:
self.map[key_hash] = list([key_value])
return True
else:
for pair in self.map[key_hash]:
if pair[0] == key:
pair[1] = value
return True
self.map[key_hash].append(key_value)
return True
def get(self, key):
key_hash = self._get_hash(key)
if self.map[key_hash] is not None:
for pair in self.map[key_hash]:
if pair[0] == key:
return pair[1]
return None
def delete(self, key):
key_hash = self._get_hash(key)
if self.map[key_hash] is None:
return False
for i in range(len(self.map[key_hash])):
if self.map[key_hash][i][0] == key:
self.map[key_hash].pop(i)
return True
return False
This implementation uses a simple hash function and handles collisions using chaining (each bucket is a list of key-value pairs). While this is a basic version, it demonstrates the core concepts of how a Hash Map works.
3. Hash Map Operations
Hash Maps support several fundamental operations. Let’s explore each of these in detail:
3.1 Insertion (add or put)
Insertion involves adding a new key-value pair to the Hash Map. If the key already exists, the value is typically updated.
3.2 Retrieval (get)
Retrieval allows you to access the value associated with a given key. If the key doesn’t exist, it usually returns null or raises an exception, depending on the implementation.
3.3 Deletion (remove or delete)
Deletion removes a key-value pair from the Hash Map based on the provided key.
3.4 Containment Check (contains or has)
This operation checks whether a specific key exists in the Hash Map.
3.5 Size
Returns the number of key-value pairs currently stored in the Hash Map.
3.6 Clear
Removes all key-value pairs from the Hash Map, resetting it to an empty state.
4. Hash Map Use Cases
Hash Maps are versatile data structures with numerous applications in software development. Here are some common use cases:
4.1 Caching
Hash Maps are excellent for implementing caches, where you need to store and quickly retrieve computed results or fetched data.
4.2 Counting Occurrences
When you need to count the frequency of items in a collection, a Hash Map can efficiently keep track of counts for each unique item.
4.3 De-duplication
Hash Maps can be used to remove duplicates from a collection by using keys as unique identifiers.
4.4 Symbol Tables
In compilers and interpreters, Hash Maps are often used to implement symbol tables for storing variable names and their associated information.
4.5 Database Indexing
Many database systems use Hash Map-like structures for indexing to provide fast data retrieval.
5. Understanding Hash Sets
A Hash Set is a data structure that implements a mathematical set using a hash table. It allows you to store unique elements and provides efficient operations for adding, removing, and checking for the existence of elements.
Key Characteristics of Hash Sets:
- Unique Elements: Each element in a Hash Set must be unique.
- No Key-Value Pairs: Unlike Hash Maps, Hash Sets only store elements, not key-value pairs.
- Fast Operations: Provides constant-time average complexity for basic operations (O(1)).
- Dynamic Size: Can grow or shrink as needed, depending on the number of elements.
- Unordered: Elements are not stored in any particular order.
6. Implementing Hash Sets
While Hash Sets are often implemented using Hash Maps internally, let’s create a simple Hash Set implementation in Python to understand its core concepts:
class HashSet:
def __init__(self, size=100):
self.size = size
self.set = [[] for _ in range(self.size)]
def _get_hash(self, key):
return hash(key) % self.size
def add(self, key):
key_hash = self._get_hash(key)
if not self.contains(key):
self.set[key_hash].append(key)
return True
return False
def remove(self, key):
key_hash = self._get_hash(key)
if self.contains(key):
self.set[key_hash].remove(key)
return True
return False
def contains(self, key):
key_hash = self._get_hash(key)
return key in self.set[key_hash]
def clear(self):
self.set = [[] for _ in range(self.size)]
This implementation uses a similar approach to our Hash Map example, but instead of storing key-value pairs, it stores only unique elements.
7. Hash Set Operations
Hash Sets support several fundamental operations. Let’s explore each of these in detail:
7.1 Addition (add)
Adds a new element to the Hash Set. If the element already exists, it is typically not added again.
7.2 Removal (remove)
Removes an element from the Hash Set. If the element doesn’t exist, the operation usually has no effect.
7.3 Containment Check (contains)
Checks whether a specific element exists in the Hash Set.
7.4 Size
Returns the number of elements currently stored in the Hash Set.
7.5 Clear
Removes all elements from the Hash Set, resetting it to an empty state.
7.6 Union
Combines two Hash Sets, resulting in a new set containing all unique elements from both sets.
7.7 Intersection
Creates a new Hash Set containing only the elements that are common to both input sets.
7.8 Difference
Creates a new Hash Set containing elements that are in one set but not in the other.
8. Hash Set Use Cases
Hash Sets are powerful data structures with various applications in software development. Here are some common use cases:
8.1 Removing Duplicates
Hash Sets are excellent for efficiently removing duplicate elements from a collection.
8.2 Membership Testing
When you need to quickly check if an element exists in a collection, Hash Sets provide fast lookups.
8.3 Mathematical Set Operations
Hash Sets are ideal for performing set operations like union, intersection, and difference.
8.4 Tracking Unique Visitors
In web analytics, Hash Sets can be used to keep track of unique visitors to a website.
8.5 Spell Checking
Hash Sets can be used to store a dictionary of valid words for efficient spell-checking algorithms.
9. Comparing Hash Maps and Hash Sets
While Hash Maps and Hash Sets share many similarities, they have distinct use cases and characteristics:
Similarities:
- Both use hash functions for efficient element access
- Both provide constant-time average complexity for basic operations
- Both can dynamically resize to accommodate more elements
Differences:
- Hash Maps store key-value pairs, while Hash Sets store only elements
- Hash Maps allow duplicate values (but not keys), while Hash Sets only store unique elements
- Hash Maps are used when you need to associate data with keys, while Hash Sets are used when you only need to track unique elements
10. Performance Considerations
Both Hash Maps and Hash Sets offer excellent performance characteristics, but there are some factors to consider:
10.1 Load Factor
The load factor is the ratio of the number of elements to the size of the underlying array. As the load factor increases, the likelihood of collisions also increases, potentially degrading performance.
10.2 Collision Resolution
Different collision resolution strategies (e.g., chaining, open addressing) can affect performance in various scenarios.
10.3 Hash Function Quality
The efficiency of the hash function in distributing elements evenly across buckets is crucial for maintaining good performance.
10.4 Initial Capacity
Choosing an appropriate initial capacity can help reduce the number of rehashing operations required as the collection grows.
11. Common Interview Questions
Here are some common interview questions related to Hash Maps and Hash Sets that you might encounter in technical interviews:
11.1 Two Sum Problem
Given an array of integers and a target sum, find two numbers in the array that add up to the target.
11.2 LRU Cache
Implement a Least Recently Used (LRU) cache with O(1) time complexity for both get and put operations.
11.3 First Non-Repeating Character
Find the first non-repeating character in a string.
11.4 Group Anagrams
Given an array of strings, group anagrams together.
11.5 Implement HashMap
Implement a HashMap class from scratch with basic operations like put, get, and remove.
12. Best Practices and Tips
To effectively use Hash Maps and Hash Sets in your code and ace technical interviews, consider these best practices and tips:
12.1 Choose the Right Tool
Understand the differences between Hash Maps and Hash Sets, and choose the appropriate data structure for your specific use case.
12.2 Consider Time Complexity
Always analyze the time complexity of your solutions and consider how Hash Maps or Hash Sets can help optimize performance.
12.3 Handle Edge Cases
When implementing or using these data structures, always consider edge cases like null keys, empty collections, or maximum capacity scenarios.
12.4 Understand Language-Specific Implementations
Familiarize yourself with the built-in Hash Map and Hash Set implementations in your preferred programming language, including any specific methods or behaviors.
12.5 Practice, Practice, Practice
Solve various coding problems that involve Hash Maps and Hash Sets to build your problem-solving skills and intuition for when to use these data structures.
13. Conclusion
Hash Maps and Hash Sets are powerful data structures that play a crucial role in efficient algorithm design and problem-solving. By understanding their concepts, implementations, and use cases, you’ll be better equipped to tackle complex coding challenges and excel in technical interviews.
Remember that mastering these data structures requires practice and application. As you continue your journey in programming and prepare for technical interviews, make sure to incorporate Hash Maps and Hash Sets into your problem-solving toolkit. With their ability to provide fast lookups, insertions, and deletions, these data structures will undoubtedly prove invaluable in your coding endeavors.
Keep practicing, stay curious, and don’t hesitate to explore more advanced topics related to hashing and data structures. Your dedication to understanding these fundamental concepts will set you apart as a skilled programmer and make you well-prepared for the challenges of technical interviews at top tech companies.