The Connection Between Math and Programming: Unlocking the Power of Algorithmic Thinking
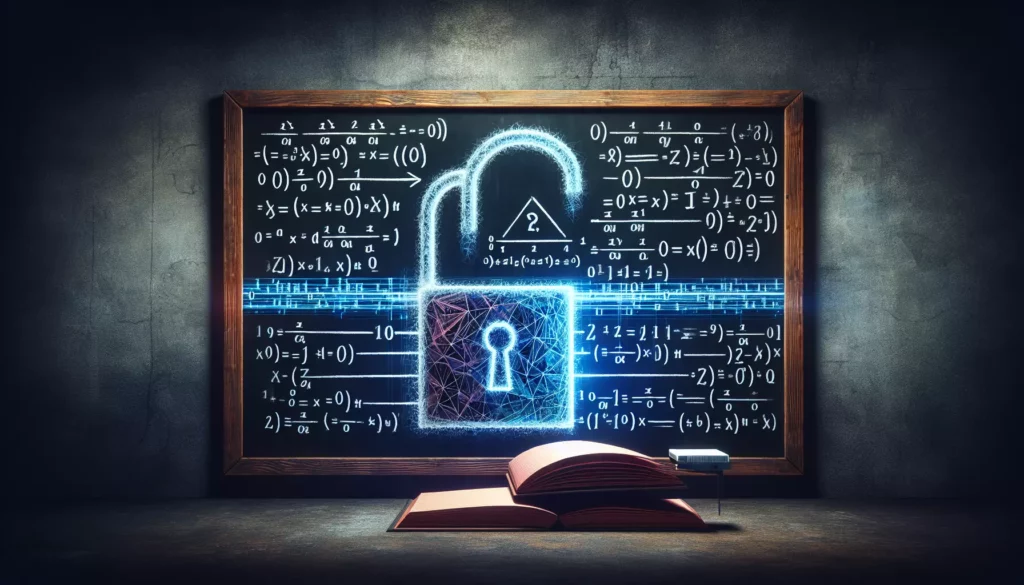
In the ever-evolving world of technology, the synergy between mathematics and programming has become increasingly apparent. As we delve deeper into the realm of coding and software development, it’s clear that a strong foundation in mathematical concepts can significantly enhance one’s programming skills. This intricate relationship between math and programming is not just a coincidence; it’s a fundamental connection that underpins much of computer science and software engineering.
In this comprehensive exploration, we’ll uncover the myriad ways in which mathematics and programming intersect, and how understanding this connection can propel aspiring developers to new heights in their coding journey. From basic arithmetic to complex algorithms, we’ll see how mathematical thinking shapes the way we approach problem-solving in programming.
The Foundations: Where Math Meets Code
At its core, programming is about solving problems through logical steps and processes. This fundamental aspect of coding shares a striking similarity with mathematical problem-solving. Both disciplines require a structured approach, breaking down complex problems into smaller, manageable parts.
1. Logical Thinking and Problem-Solving
Mathematics trains the mind to think logically and approach problems systematically. This same skill is crucial in programming, where developers must break down complex tasks into smaller, solvable components. The ability to analyze a problem, identify patterns, and develop step-by-step solutions is a cornerstone of both mathematical and programming prowess.
2. Abstraction and Generalization
In mathematics, we often deal with abstract concepts and generalize specific cases to broader principles. This skill translates directly to programming, where abstraction is a key concept in creating reusable and efficient code. Object-oriented programming, for instance, heavily relies on the ability to abstract common features and behaviors into classes and interfaces.
3. Precision and Attention to Detail
Mathematical calculations require precision and attention to detail. A single misplaced digit can lead to incorrect results. Similarly, in programming, a misplaced semicolon or an off-by-one error can cause entire systems to malfunction. The meticulous nature of mathematics instills a sense of precision that is invaluable in writing clean, bug-free code.
Mathematical Concepts in Programming
As we delve deeper into the world of programming, we encounter numerous mathematical concepts that play crucial roles in various aspects of software development. Let’s explore some of these key areas:
1. Algebra in Programming
Algebraic concepts are fundamental to many programming tasks. Variables in programming languages are essentially algebraic variables, storing and manipulating data. Understanding algebraic expressions and equations helps in writing efficient algorithms and solving complex coding problems.
For example, consider a simple algorithm to calculate the average of a list of numbers:
function calculateAverage(numbers) {
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum / numbers.length;
}
This function uses basic algebraic concepts like summation and division to compute the average.
2. Geometry and Graphics Programming
Geometric principles are essential in computer graphics, game development, and data visualization. Concepts like coordinates, vectors, and transformations are used extensively in these fields.
For instance, in game development, calculating the distance between two points often involves the Pythagorean theorem:
function calculateDistance(x1, y1, x2, y2) {
return Math.sqrt(Math.pow(x2 - x1, 2) + Math.pow(y2 - y1, 2));
}
3. Calculus in Optimization and Machine Learning
Calculus plays a significant role in advanced programming areas like optimization algorithms and machine learning. Concepts like derivatives and gradients are fundamental to many machine learning algorithms, especially in neural networks and deep learning.
For example, the gradient descent algorithm, widely used in machine learning, relies on calculus to minimize error functions:
function gradientDescent(initialGuess, learningRate, numIterations) {
let x = initialGuess;
for (let i = 0; i < numIterations; i++) {
let gradient = calculateGradient(x);
x = x - learningRate * gradient;
}
return x;
}
4. Discrete Mathematics in Algorithms and Data Structures
Discrete mathematics, including topics like set theory, graph theory, and combinatorics, is crucial in designing efficient algorithms and data structures. These concepts help in solving complex computational problems and optimizing code performance.
For instance, graph theory is extensively used in network algorithms:
class Graph {
constructor() {
this.adjacencyList = {};
}
addVertex(vertex) {
if (!this.adjacencyList[vertex]) {
this.adjacencyList[vertex] = [];
}
}
addEdge(vertex1, vertex2) {
this.adjacencyList[vertex1].push(vertex2);
this.adjacencyList[vertex2].push(vertex1);
}
}
Mathematical Thinking in Algorithm Design
The process of designing efficient algorithms often relies heavily on mathematical thinking. Let’s explore how mathematical concepts influence algorithm design and optimization:
1. Time and Space Complexity Analysis
Understanding the efficiency of algorithms is crucial in computer science. This analysis often involves mathematical concepts like Big O notation, which describes the upper bound of an algorithm’s growth rate. For example, an algorithm with O(n) time complexity grows linearly with the input size, while an O(n^2) algorithm grows quadratically.
Consider the difference between a linear search and a binary search:
// Linear search: O(n) time complexity
function linearSearch(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) return i;
}
return -1;
}
// Binary search: O(log n) time complexity
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
The binary search algorithm, with its logarithmic time complexity, demonstrates how mathematical thinking can lead to more efficient solutions.
2. Recursion and Mathematical Induction
Recursion, a powerful programming technique, is closely related to mathematical induction. Both involve solving a problem by breaking it down into smaller instances of the same problem. Understanding mathematical induction can greatly enhance a programmer’s ability to design and implement recursive algorithms.
A classic example is the recursive implementation of the Fibonacci sequence:
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
3. Optimization Problems
Many real-world programming challenges involve optimization problems, where the goal is to find the best solution among many possibilities. These problems often require mathematical techniques like linear programming, dynamic programming, or heuristic algorithms.
For instance, the knapsack problem, a classic optimization challenge, can be solved using dynamic programming:
function knapsack(values, weights, capacity) {
let n = values.length;
let dp = Array(n + 1).fill().map(() => Array(capacity + 1).fill(0));
for (let i = 1; i <= n; i++) {
for (let w = 1; w <= capacity; w++) {
if (weights[i - 1] <= w) {
dp[i][w] = Math.max(
values[i - 1] + dp[i - 1][w - weights[i - 1]],
dp[i - 1][w]
);
} else {
dp[i][w] = dp[i - 1][w];
}
}
}
return dp[n][capacity];
}
Mathematics in Specific Programming Domains
The influence of mathematics extends into various specialized areas of programming. Let’s explore how mathematical concepts are applied in different programming domains:
1. Cryptography and Security
Cryptography, the backbone of cybersecurity, relies heavily on mathematical principles. Number theory, particularly prime numbers and modular arithmetic, forms the basis of many encryption algorithms. For instance, the RSA algorithm, widely used in secure data transmission, is based on the mathematical difficulty of factoring large numbers.
Here’s a simplified example of modular exponentiation, a key concept in cryptography:
function modularExponentiation(base, exponent, modulus) {
if (modulus === 1) return 0;
let result = 1;
base = base % modulus;
while (exponent > 0) {
if (exponent % 2 === 1)
result = (result * base) % modulus;
exponent = Math.floor(exponent / 2);
base = (base * base) % modulus;
}
return result;
}
2. Computer Graphics and Game Development
Computer graphics and game development rely heavily on linear algebra and trigonometry. Concepts like matrices, vectors, and transformations are used to create and manipulate 3D objects, implement lighting models, and handle physics simulations.
For example, rotating a 2D point around the origin uses trigonometric functions:
function rotatePoint(x, y, angle) {
let radians = angle * (Math.PI / 180);
let cos = Math.cos(radians);
let sin = Math.sin(radians);
return {
x: x * cos - y * sin,
y: x * sin + y * cos
};
}
3. Data Science and Machine Learning
Data science and machine learning are fields where mathematics and programming intersect most prominently. Statistical concepts, linear algebra, and calculus form the foundation of many machine learning algorithms. Understanding these mathematical principles is crucial for implementing and optimizing machine learning models.
Here’s a simple implementation of linear regression using gradient descent:
function linearRegression(X, y, learningRate, iterations) {
let m = X.length;
let theta = [0, 0]; // Initial parameters
for (let i = 0; i < iterations; i++) {
let h = X.map(x => theta[0] + theta[1] * x);
let cost = h.reduce((sum, h_i, i) => sum + Math.pow(h_i - y[i], 2), 0) / (2 * m);
let temp0 = theta[0] - learningRate * h.reduce((sum, h_i, i) => sum + (h_i - y[i]), 0) / m;
let temp1 = theta[1] - learningRate * h.reduce((sum, h_i, i) => sum + (h_i - y[i]) * X[i], 0) / m;
theta = [temp0, temp1];
}
return theta;
}
Developing Mathematical Thinking for Programming
Given the strong connection between mathematics and programming, aspiring developers can greatly benefit from cultivating their mathematical thinking skills. Here are some strategies to enhance mathematical abilities in the context of programming:
1. Practice Problem-Solving
Engage in mathematical problem-solving exercises regularly. Platforms like Project Euler offer a blend of mathematical and programming challenges that can sharpen both skills simultaneously. These problems often require a combination of mathematical insight and efficient coding to solve.
2. Study Algorithms and Data Structures
Delve deep into the study of algorithms and data structures. Many classic algorithms are based on mathematical principles. Understanding the mathematical foundations of these algorithms can provide insights into their efficiency and applicability.
3. Explore Discrete Mathematics
Discrete mathematics is particularly relevant to computer science. Topics like set theory, graph theory, and combinatorics have direct applications in programming. Studying these areas can enhance your ability to model and solve complex computational problems.
4. Learn Linear Algebra and Statistics
For those interested in data science, machine learning, or computer graphics, a solid understanding of linear algebra and statistics is invaluable. These mathematical fields provide the tools to work with large datasets, implement machine learning algorithms, and perform complex data manipulations.
5. Practice Formal Logic
Studying formal logic can improve your ability to construct and analyze logical arguments, a skill directly applicable to programming. Boolean algebra, in particular, is fundamental to understanding conditional statements and logical operations in programming.
Bridging the Gap: From Math to Code
Transitioning from mathematical concepts to practical coding implementations can be challenging. Here are some tips to help bridge this gap:
1. Implement Mathematical Concepts in Code
Try to implement mathematical algorithms and concepts in your preferred programming language. This practice helps solidify your understanding and shows how mathematical ideas translate into code.
For example, implementing the Sieve of Eratosthenes algorithm for finding prime numbers:
function sieveOfEratosthenes(n) {
let primes = new Array(n + 1).fill(true);
primes[0] = primes[1] = false;
for (let i = 2; i * i <= n; i++) {
if (primes[i]) {
for (let j = i * i; j <= n; j += i) {
primes[j] = false;
}
}
}
return primes.reduce((acc, isPrime, num) => isPrime ? [...acc, num] : acc, []);
}
2. Analyze Algorithmic Complexity
Practice analyzing the time and space complexity of algorithms. This exercise combines mathematical thinking with practical programming considerations, helping you understand the efficiency of your code.
3. Use Visualization Tools
Utilize visualization tools to understand complex mathematical concepts and algorithms. Many online platforms offer interactive visualizations of algorithms, helping bridge the gap between abstract mathematical ideas and their concrete implementations.
4. Participate in Coding Challenges
Engage in coding challenges that require mathematical problem-solving. Platforms like LeetCode, HackerRank, and CodeForces offer a variety of problems that blend mathematical concepts with programming skills.
The Future: Mathematics and Emerging Technologies
As we look to the future of technology, the role of mathematics in programming is set to become even more prominent. Emerging fields like quantum computing, artificial intelligence, and blockchain technology all have deep mathematical foundations.
1. Quantum Computing
Quantum computing relies heavily on complex mathematical concepts from quantum mechanics and linear algebra. As quantum computers become more prevalent, programmers with strong mathematical backgrounds will be in high demand to develop quantum algorithms and applications.
2. Advanced AI and Machine Learning
As AI systems become more sophisticated, the mathematical complexity underlying these systems increases. Deep learning, reinforcement learning, and other advanced AI techniques require a profound understanding of mathematics, particularly in areas like optimization theory and probability.
3. Blockchain and Cryptography
The growing field of blockchain technology and cryptocurrency relies on advanced cryptographic principles. As these technologies evolve, the demand for programmers who can implement and innovate in cryptographic algorithms will continue to grow.
Conclusion: Embracing the Mathematical Mindset
The connection between mathematics and programming is deep and multifaceted. From the logical thinking and problem-solving skills fostered by mathematical study to the direct application of mathematical concepts in various programming domains, the synergy between these two fields is undeniable.
For aspiring programmers and seasoned developers alike, cultivating a strong mathematical foundation can significantly enhance coding abilities and open doors to advanced areas of computer science. By embracing the mathematical mindset, programmers can approach problems with greater insight, design more efficient algorithms, and push the boundaries of what’s possible in software development.
As we stand on the brink of new technological frontiers, the interplay between mathematics and programming will continue to drive innovation. Those who can effectively bridge these two disciplines will be well-positioned to lead in the ever-evolving world of technology.
Remember, every line of code you write is, in essence, a mathematical expression. By honing your mathematical thinking skills alongside your programming abilities, you’re not just becoming a better coder – you’re becoming a more complete and versatile problem solver in the digital age.