Coding Challenges: Daily Exercises to Sharpen Your Skills
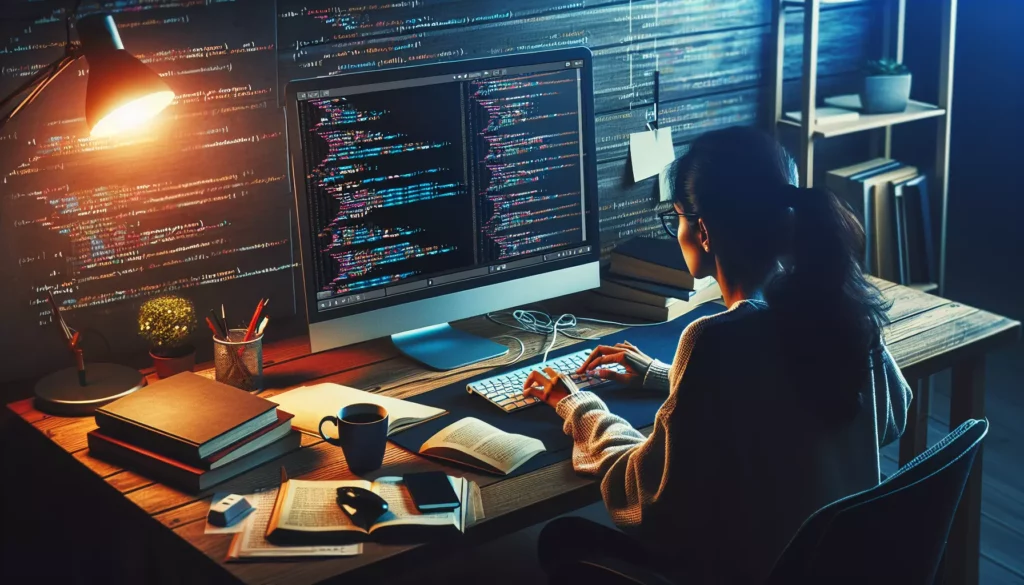
In the ever-evolving world of technology, staying sharp and continuously improving your coding skills is essential for any programmer, whether you’re a beginner or an experienced developer. One of the most effective ways to enhance your abilities is through daily coding challenges. These exercises not only help you practice your current skills but also expose you to new concepts and problem-solving techniques. In this comprehensive guide, we’ll explore the benefits of daily coding challenges, how to incorporate them into your routine, and provide you with a variety of resources to get started.
Why Daily Coding Challenges Matter
Before diving into the specifics of coding challenges, let’s understand why they are crucial for your growth as a programmer:
- Consistency in Learning: Daily practice helps reinforce concepts and keeps your skills fresh.
- Problem-Solving Skills: Regular exposure to diverse problems enhances your ability to approach and solve complex issues.
- Interview Preparation: Many challenges mimic the types of questions asked in technical interviews, especially for FAANG companies.
- Exposure to New Concepts: Challenges often introduce you to algorithms and data structures you might not encounter in your day-to-day work.
- Time Management: Working on timed challenges improves your ability to code efficiently under pressure.
- Community Engagement: Many platforms offer community discussions, allowing you to learn from others’ solutions.
Getting Started with Daily Coding Challenges
Embarking on a journey of daily coding challenges can seem daunting at first, but with the right approach, it can become an enjoyable and rewarding part of your routine. Here’s how to get started:
1. Choose the Right Platform
There are numerous platforms available for coding challenges. Some popular options include:
- LeetCode: Known for its extensive collection of algorithm and data structure problems, often used for interview preparation.
- HackerRank: Offers a wide range of challenges across multiple domains and skill levels.
- CodeWars: Provides challenges called “kata” in various programming languages, with a unique ranking system.
- Project Euler: Focuses on mathematical and computational problems that require programming solutions.
- AlgoCademy: Offers interactive coding tutorials and challenges with AI-powered assistance, ideal for beginners and those preparing for tech interviews.
2. Set Realistic Goals
Start small and build consistency. Aim for one challenge per day, even if it’s a simple one. As you progress, you can increase the difficulty or number of challenges.
3. Choose the Right Difficulty Level
Begin with challenges that match your current skill level. Most platforms categorize problems by difficulty, allowing you to progress gradually.
4. Allocate Dedicated Time
Set aside a specific time each day for your coding challenge. This could be early morning, during lunch break, or in the evening – whatever works best for your schedule.
5. Focus on Understanding, Not Just Solving
After solving a challenge, take time to understand different approaches and optimizations. Many platforms provide discussions or editorial sections where you can learn from others’ solutions.
Types of Coding Challenges
Coding challenges come in various forms, each focusing on different aspects of programming and problem-solving. Here are some common types you’ll encounter:
1. Algorithm Challenges
These focus on implementing and optimizing algorithms. Examples include:
- Sorting algorithms (e.g., QuickSort, MergeSort)
- Search algorithms (e.g., Binary Search, Depth-First Search)
- Dynamic Programming problems
- Greedy algorithms
2. Data Structure Challenges
These involve manipulating and utilizing various data structures efficiently. Common structures include:
- Arrays and Strings
- Linked Lists
- Trees and Graphs
- Stacks and Queues
- Hash Tables
3. Mathematical and Logic Puzzles
These challenges often require a combination of mathematical thinking and programming skills. They might involve:
- Number theory problems
- Probability and statistics
- Geometry algorithms
- Logic puzzles translated into code
4. System Design Challenges
While less common in daily challenges, these are crucial for interview preparation, especially for senior roles. They might involve:
- Designing a scalable web service
- Creating an efficient database schema
- Implementing a basic version of a complex system (e.g., a simple search engine)
5. Language-Specific Challenges
These focus on the nuances and features of specific programming languages. They help in mastering language-specific syntax and best practices.
Strategies for Tackling Coding Challenges
To make the most of your daily coding challenges, consider the following strategies:
1. Read and Understand the Problem
Before diving into coding, ensure you fully understand the problem statement, input/output format, and any constraints.
2. Plan Your Approach
Sketch out your solution on paper or whiteboard before coding. This helps in organizing your thoughts and identifying potential issues early.
3. Start with a Brute Force Solution
If you’re stuck, begin with the simplest solution that comes to mind, even if it’s not optimal. You can optimize later.
4. Test Your Solution
Don’t rely solely on the platform’s test cases. Create your own test cases, including edge cases, to ensure your solution is robust.
5. Optimize and Refactor
Once you have a working solution, look for ways to improve its time and space complexity. Refactor your code for better readability and efficiency.
6. Learn from Others
After solving a challenge, review other solutions. This exposes you to different approaches and coding styles.
7. Revisit Challenging Problems
Keep a list of problems you found difficult. Revisit them after a few weeks to reinforce your learning and track your progress.
Sample Coding Challenge and Solution
Let’s walk through a simple coding challenge to illustrate the process:
Problem: Two Sum
Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
.
You may assume that each input would have exactly one solution, and you may not use the same element twice.
Example:
Input: nums = [2,7,11,15], target = 9
Output: [0,1]
Explanation: Because nums[0] + nums[1] == 9, we return [0, 1].
Solution in Python:
class Solution:
def twoSum(self, nums: List[int], target: int) -> List[int]:
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return [] # No solution found
Explanation:
This solution uses a hash table (dictionary in Python) to achieve O(n) time complexity:
- We iterate through the array once, using enumeration to keep track of both the index and value.
- For each number, we calculate its complement (target – num).
- If the complement exists in our dictionary, we’ve found our pair and return their indices.
- If not, we add the current number and its index to the dictionary.
- If we complete the loop without finding a solution, we return an empty list.
This approach is more efficient than the brute force method of checking every pair, which would have O(n^2) time complexity.
Advanced Topics in Coding Challenges
As you progress in your coding challenge journey, you’ll encounter more advanced topics. Here are some areas to focus on:
1. Time and Space Complexity Analysis
Understanding Big O notation and being able to analyze the efficiency of your algorithms is crucial. Practice identifying the time and space complexity of your solutions and look for ways to optimize them.
2. Advanced Data Structures
Familiarize yourself with more complex data structures such as:
- Heaps and Priority Queues
- Trie (Prefix Tree)
- Disjoint Set Union (Union-Find)
- Segment Trees
- Fenwick Trees (Binary Indexed Trees)
3. Advanced Algorithms
Delve into more sophisticated algorithmic techniques:
- Backtracking
- Divide and Conquer
- Sliding Window
- Two Pointers
- Topological Sorting
- Bit Manipulation
4. Concurrency and Multithreading
As you advance, you might encounter challenges related to concurrent programming. Understanding concepts like locks, semaphores, and thread synchronization becomes important.
5. System Design and Scalability
For those aiming for senior positions, especially in FAANG companies, system design challenges are crucial. These involve designing large-scale distributed systems and understanding concepts like:
- Load Balancing
- Caching
- Database Sharding
- Microservices Architecture
- CAP Theorem
Leveraging AI for Coding Challenges
With the advent of AI-powered coding assistants, there are new ways to enhance your coding challenge experience:
1. AI-Powered Hints and Explanations
Platforms like AlgoCademy use AI to provide contextual hints and explanations. This can be particularly helpful when you’re stuck on a problem or want to understand a concept better.
2. Code Analysis and Suggestions
Some AI tools can analyze your code and suggest improvements or point out potential bugs. This can help you learn best practices and coding standards.
3. Personalized Learning Paths
AI algorithms can track your progress and suggest challenges that are most relevant to your skill level and learning goals.
4. Natural Language Problem Solving
Advanced AI systems can help you break down complex problems by allowing you to describe your approach in natural language before translating it into code.
Maintaining Motivation and Tracking Progress
Consistency is key in daily coding challenges. Here are some tips to stay motivated:
1. Join a Community
Participate in coding challenge communities or find a coding buddy. Sharing experiences and competing friendly can be motivating.
2. Use Gamification Features
Many platforms offer badges, levels, or points systems. Use these to set personal goals and track your progress.
3. Keep a Coding Journal
Document your learnings, challenges faced, and breakthroughs. This can be rewarding to look back on and helpful for interview preparation.
4. Set Milestone Goals
Instead of focusing solely on daily challenges, set longer-term goals like mastering a specific topic or completing a certain number of hard-level problems.
5. Celebrate Small Wins
Acknowledge your progress, no matter how small. Solving a difficult problem or understanding a new concept is always worth celebrating.
Conclusion
Daily coding challenges are an invaluable tool for any programmer looking to improve their skills, prepare for technical interviews, or simply stay sharp in a rapidly evolving field. By incorporating these challenges into your routine, you’re not just practicing coding; you’re developing problem-solving skills, expanding your knowledge of algorithms and data structures, and preparing yourself for the challenges of a career in software development.
Remember, the key to success with coding challenges is consistency and a growth mindset. Every problem you tackle, whether you solve it immediately or struggle with it, is an opportunity to learn and grow. Embrace the challenges, learn from your mistakes, and celebrate your progress.
As you continue on this journey, consider leveraging platforms like AlgoCademy that offer AI-powered assistance and personalized learning paths. These tools can provide valuable guidance and help you make the most of your daily coding practice.
Happy coding, and may your daily challenges lead you to new heights in your programming journey!