Dynamic Programming: Mastering Tabulation and Memoization
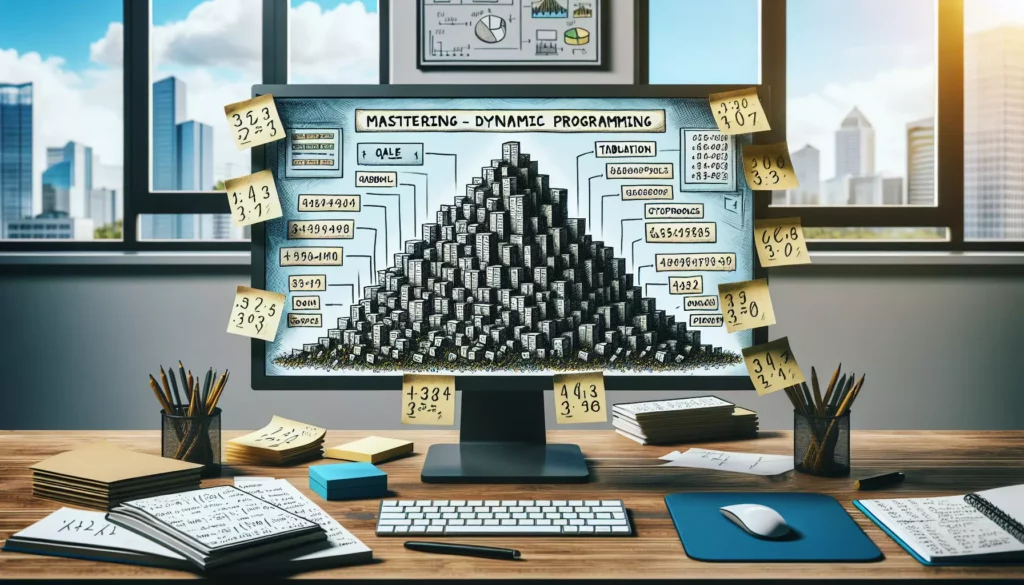
Dynamic Programming (DP) is a powerful algorithmic technique used to solve complex problems by breaking them down into simpler subproblems. It’s an essential skill for any programmer, especially those preparing for technical interviews at top tech companies. In this comprehensive guide, we’ll explore two fundamental approaches to dynamic programming: tabulation and memoization. By the end of this article, you’ll have a solid understanding of these techniques and be able to apply them to solve a wide range of programming challenges.
What is Dynamic Programming?
Before we dive into the specifics of tabulation and memoization, let’s first understand what dynamic programming is and why it’s so important in computer science and software engineering.
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It is applicable to problems that exhibit two key properties:
- Optimal Substructure: The optimal solution to the problem can be constructed from optimal solutions of its subproblems.
- Overlapping Subproblems: The problem can be broken down into subproblems which are reused several times.
By leveraging these properties, dynamic programming allows us to solve problems more efficiently than naive recursive approaches. It does this by storing the results of subproblems, so we don’t have to recompute them multiple times.
Tabulation: Bottom-Up Dynamic Programming
Tabulation is a bottom-up approach to dynamic programming where we start from the smallest subproblems and work our way up to the main problem. This method typically involves creating a table (hence the name “tabulation”) to store the results of subproblems.
Key Characteristics of Tabulation:
- Iterative approach
- Starts solving from the smallest subproblem
- Uses a table to store results
- Usually more space-efficient than memoization
Example: Fibonacci Sequence using Tabulation
Let’s implement the Fibonacci sequence using tabulation. The Fibonacci sequence is defined as follows: F(n) = F(n-1) + F(n-2), with F(0) = 0 and F(1) = 1.
def fibonacci_tabulation(n):
if n <= 1:
return n
# Initialize the table with 0s
dp = [0] * (n + 1)
# Base cases
dp[0] = 0
dp[1] = 1
# Fill the table
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Test the function
print(fibonacci_tabulation(10)) # Output: 55
In this example, we create a table (the dp
list) to store the Fibonacci numbers. We start by filling in the base cases (F(0) and F(1)), and then iteratively compute each subsequent Fibonacci number using the previously computed values.
Advantages of Tabulation:
- Space Efficiency: Tabulation often uses less space than memoization, especially for problems with a natural bottom-up structure.
- Ease of Analysis: The time and space complexity of tabulation algorithms are often easier to analyze than their recursive counterparts.
- Avoiding Stack Overflow: Since tabulation is iterative, it avoids the risk of stack overflow that can occur with deep recursion in memoization.
Memoization: Top-Down Dynamic Programming
Memoization is a top-down approach to dynamic programming where we start with the main problem and recursively break it down into smaller subproblems. The key idea is to store the results of expensive function calls and return the cached result when the same inputs occur again.
Key Characteristics of Memoization:
- Recursive approach
- Starts solving from the main problem
- Uses a cache (often a dictionary) to store results
- Can be easier to implement for some problems
Example: Fibonacci Sequence using Memoization
Let’s implement the same Fibonacci sequence using memoization:
def fibonacci_memoization(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memoization(n-1, memo) + fibonacci_memoization(n-2, memo)
return memo[n]
# Test the function
print(fibonacci_memoization(10)) # Output: 55
In this memoized version, we use a dictionary (memo
) to store the results of previously computed Fibonacci numbers. Before computing a Fibonacci number, we first check if it’s already in our memo. If it is, we return the cached result. Otherwise, we compute it recursively and store the result in the memo before returning it.
Advantages of Memoization:
- Intuitive Implementation: Memoization often follows the natural recursive structure of the problem, making it easier to implement for some problems.
- Lazy Evaluation: Memoization computes values only when they are needed, which can be more efficient for problems where not all subproblems are necessary.
- Adaptability: Memoization can be easily added to existing recursive solutions to improve their efficiency.
Comparing Tabulation and Memoization
While both tabulation and memoization are powerful techniques for implementing dynamic programming solutions, they each have their strengths and weaknesses. Let’s compare them across several dimensions:
1. Direction of Computation
- Tabulation: Bottom-up approach. Starts from the smallest subproblems and builds up to the main problem.
- Memoization: Top-down approach. Starts from the main problem and recursively breaks it down into smaller subproblems.
2. Space Efficiency
- Tabulation: Generally more space-efficient, as it only stores the necessary subproblems in a predefined table.
- Memoization: May use more space, especially if many unnecessary subproblems are computed and stored.
3. Time Efficiency
- Tabulation: Often slightly faster due to lack of recursive overhead and better cache performance.
- Memoization: May have some overhead due to recursion and dictionary lookups, but can be faster for problems where not all subproblems need to be solved.
4. Ease of Implementation
- Tabulation: Can be more challenging to implement, especially for problems that don’t have a natural bottom-up structure.
- Memoization: Often easier to implement, especially when starting from a recursive solution.
5. Subproblem Solving
- Tabulation: Solves all subproblems, even those that might not be necessary for the final solution.
- Memoization: Solves only the subproblems that are actually needed, which can be more efficient for some problems.
When to Use Tabulation vs. Memoization
Choosing between tabulation and memoization depends on the specific problem you’re trying to solve and the constraints of your system. Here are some guidelines to help you decide:
Use Tabulation When:
- You can identify a clear bottom-up approach to solving the problem.
- You need to solve all subproblems to reach the final solution.
- Space efficiency is a primary concern.
- You want to avoid the risk of stack overflow from deep recursion.
Use Memoization When:
- The problem has a natural recursive structure.
- Not all subproblems need to be solved to reach the final solution.
- You’re starting with a recursive solution and want to optimize it.
- You need a quick implementation and space efficiency is less of a concern.
Advanced Dynamic Programming Techniques
As you become more comfortable with basic tabulation and memoization, you can explore more advanced dynamic programming techniques:
1. Space Optimization
In many DP problems, you can optimize space usage by only keeping track of the last few states instead of the entire table. This is particularly useful in tabulation.
Example: Space-Optimized Fibonacci
def fibonacci_optimized(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
print(fibonacci_optimized(10)) # Output: 55
This implementation uses only O(1) space, regardless of the input size.
2. State Compression
For problems with multiple state variables, you can sometimes compress the state into a single variable (often using bitwise operations) to reduce memory usage and simplify the implementation.
3. Divide and Conquer DP
This technique combines the divide-and-conquer paradigm with dynamic programming. It’s useful for problems where the recurrence relation involves minimizing over all possible partition points.
4. Knuth’s Optimization
This optimization technique can be applied to certain types of dynamic programming problems to reduce the time complexity from O(n^3) to O(n^2).
Common Dynamic Programming Problems
To help you practice and reinforce your understanding of dynamic programming, here are some classic problems that can be solved using either tabulation or memoization:
- Longest Common Subsequence (LCS): Find the longest subsequence present in given two sequences.
- 0/1 Knapsack Problem: Maximize the value of items in a knapsack without exceeding its weight capacity.
- Coin Change Problem: Find the minimum number of coins that make a given amount of money.
- Longest Increasing Subsequence (LIS): Find the length of the longest subsequence of a given sequence such that all elements of the subsequence are sorted in ascending order.
- Matrix Chain Multiplication: Determine the most efficient way to multiply a chain of matrices.
- Edit Distance: Find the minimum number of operations required to convert one string into another.
Let’s solve one of these problems using both tabulation and memoization to further illustrate the differences between these approaches.
Example: Longest Common Subsequence (LCS)
The Longest Common Subsequence problem asks us to find the length of the longest subsequence present in given two sequences. A subsequence is a sequence that appears in the same relative order, but not necessarily contiguous.
Tabulation Approach:
def lcs_tabulation(X, Y):
m, n = len(X), len(Y)
# Create a table to store LCS lengths
dp = [[0] * (n + 1) for _ in range(m + 1)]
# Build the dp table
for i in range(1, m + 1):
for j in range(1, n + 1):
if X[i-1] == Y[j-1]:
dp[i][j] = dp[i-1][j-1] + 1
else:
dp[i][j] = max(dp[i-1][j], dp[i][j-1])
return dp[m][n]
# Test the function
X = "ABCDGH"
Y = "AEDFHR"
print(lcs_tabulation(X, Y)) # Output: 3
In this tabulation approach, we build a 2D table bottom-up. The entry dp[i][j]
represents the length of the LCS of the prefixes X[0:i] and Y[0:j].
Memoization Approach:
def lcs_memoization(X, Y, m, n, memo={}):
if (m, n) in memo:
return memo[(m, n)]
if m == 0 or n == 0:
result = 0
elif X[m-1] == Y[n-1]:
result = 1 + lcs_memoization(X, Y, m-1, n-1, memo)
else:
result = max(lcs_memoization(X, Y, m-1, n, memo), lcs_memoization(X, Y, m, n-1, memo))
memo[(m, n)] = result
return result
# Test the function
X = "ABCDGH"
Y = "AEDFHR"
print(lcs_memoization(X, Y, len(X), len(Y))) # Output: 3
In this memoization approach, we use a dictionary to store the results of subproblems. We start from the main problem and recursively break it down, storing results to avoid redundant computations.
Tips for Mastering Dynamic Programming
Becoming proficient in dynamic programming takes practice and patience. Here are some tips to help you master this powerful technique:
- Identify the Problem Structure: Look for overlapping subproblems and optimal substructure in the problem statement.
- Define the State: Clearly define what each state in your DP solution represents.
- Establish the Recurrence Relation: Figure out how the solution to a problem relates to the solutions of its subproblems.
- Determine the Base Cases: Identify the simplest subproblems that can be solved directly.
- Choose the Right Approach: Decide whether tabulation or memoization is more suitable for the problem at hand.
- Optimize: Look for ways to optimize your solution, such as reducing the space complexity.
- Practice Regularly: Solve a variety of DP problems to build your intuition and problem-solving skills.
- Analyze Time and Space Complexity: Always consider the time and space complexity of your solutions.
- Visualize the Process: Use diagrams or tables to visualize how your DP solution builds up the final result.
- Learn from Solutions: Study well-written solutions to complex DP problems to learn new techniques and approaches.
Conclusion
Dynamic programming is a powerful technique that can significantly improve the efficiency of algorithms for a wide range of problems. By mastering both tabulation and memoization, you’ll be well-equipped to tackle complex coding challenges and excel in technical interviews.
Remember that the key to becoming proficient in dynamic programming is practice. Start with simple problems and gradually work your way up to more complex ones. As you gain experience, you’ll develop an intuition for identifying DP problems and choosing the most appropriate approach to solve them.
Whether you’re preparing for coding interviews at top tech companies or simply looking to improve your problem-solving skills, a strong foundation in dynamic programming will serve you well throughout your programming career. Keep practicing, stay curious, and don’t be afraid to tackle challenging problems – with time and effort, you’ll master the art of dynamic programming!