Binary Search: A Powerful Algorithm for Efficient Searching
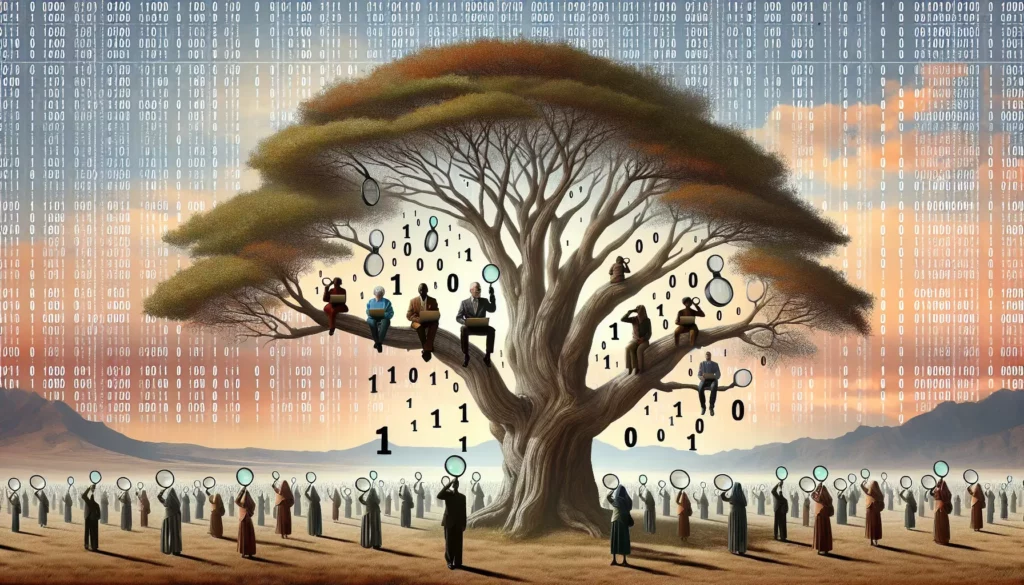
In the world of computer science and programming, efficiency is key. When it comes to searching through large datasets, one algorithm stands out for its simplicity and effectiveness: binary search. This powerful technique can dramatically reduce the time it takes to find a specific element in a sorted array, making it an essential tool in any programmer’s toolkit. In this comprehensive guide, we’ll dive deep into the world of binary search, exploring its concepts, implementation, and real-world applications.
What is Binary Search?
Binary search is a divide-and-conquer algorithm used to find a specific element in a sorted array. Unlike linear search, which checks each element one by one, binary search repeatedly divides the search interval in half. This approach allows it to quickly narrow down the possible locations of the target element, resulting in a much faster search process.
The key idea behind binary search is to take advantage of the array’s sorted nature. By comparing the target value with the middle element of the array, we can eliminate half of the remaining elements in each step. This process continues until the target element is found or it’s determined that the element doesn’t exist in the array.
How Binary Search Works
Let’s break down the binary search algorithm step by step:
- Start with the entire sorted array.
- Find the middle element of the current search range.
- Compare the middle element with the target value:
- If they’re equal, we’ve found the target element.
- If the target is less than the middle element, repeat the search on the left half of the array.
- If the target is greater than the middle element, repeat the search on the right half of the array.
This process allows binary search to eliminate half of the remaining elements in each iteration, resulting in a logarithmic time complexity of O(log n), where n is the number of elements in the array.
Implementing Binary Search
Now that we understand the concept, let’s implement binary search in various programming languages. We’ll start with a simple iterative approach and then explore a recursive implementation.
Iterative Binary Search
Here’s an implementation of binary search using an iterative approach in Python:
def binary_search(arr, target):
left = 0
right = len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Element not found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15, 17]
target = 7
result = binary_search(sorted_array, target)
print(f"Element {target} found at index: {result}")
In this implementation, we use two pointers, left
and right
, to keep track of the current search range. We calculate the middle index using integer division and compare the middle element with the target value. Based on the comparison, we update either the left
or right
pointer to narrow down the search range.
Recursive Binary Search
Now, let’s implement binary search using a recursive approach in Java:
public class BinarySearch {
public static int binarySearch(int[] arr, int target, int left, int right) {
if (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
}
if (arr[mid] > target) {
return binarySearch(arr, target, left, mid - 1);
}
return binarySearch(arr, target, mid + 1, right);
}
return -1; // Element not found
}
public static void main(String[] args) {
int[] sortedArray = {1, 3, 5, 7, 9, 11, 13, 15, 17};
int target = 7;
int result = binarySearch(sortedArray, target, 0, sortedArray.length - 1);
System.out.println("Element " + target + " found at index: " + result);
}
}
In this recursive implementation, we pass the current search range (left
and right
indices) as parameters to the function. The base case is when the search range is empty (left > right
). We calculate the middle index and make recursive calls on either the left or right half of the array based on the comparison with the target value.
Time and Space Complexity
One of the main advantages of binary search is its efficiency in terms of time complexity. Let’s analyze the time and space complexity of the algorithm:
Time Complexity
The time complexity of binary search is O(log n), where n is the number of elements in the sorted array. This logarithmic time complexity makes binary search extremely efficient for large datasets. To understand why, consider the following:
- In each iteration, we eliminate half of the remaining elements.
- The number of iterations required to find an element or determine its absence is at most logâ‚‚(n) + 1.
- This logarithmic growth means that doubling the size of the input array only increases the number of iterations by 1.
For example, in an array of 1 million elements, binary search would require at most 20 iterations to find any element or conclude that it doesn’t exist.
Space Complexity
The space complexity of binary search depends on the implementation:
- Iterative implementation: O(1) – We only use a constant amount of extra space for variables like
left
,right
, andmid
. - Recursive implementation: O(log n) – The space complexity is determined by the maximum depth of the recursion stack, which is logarithmic in the size of the input array.
In most cases, the iterative implementation is preferred due to its constant space complexity and slightly better performance.
Common Variations and Edge Cases
While the basic binary search algorithm is straightforward, there are several variations and edge cases to consider when implementing it in practice:
1. Finding the First or Last Occurrence
When dealing with arrays that contain duplicate elements, you might want to find the first or last occurrence of a specific value. Here’s an example of finding the first occurrence:
def binary_search_first_occurrence(arr, target):
left, right = 0, len(arr) - 1
result = -1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
result = mid
right = mid - 1 # Continue searching in the left half
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return result
# Example usage
sorted_array = [1, 2, 2, 2, 3, 4, 4, 5]
target = 2
result = binary_search_first_occurrence(sorted_array, target)
print(f"First occurrence of {target} found at index: {result}")
This variation continues the search even after finding a match, ensuring that we find the leftmost occurrence of the target value.
2. Finding the Insertion Point
Sometimes, you might want to find the index where an element should be inserted to maintain the sorted order of the array. This is useful for implementing algorithms like insertion sort or maintaining sorted data structures.
def binary_search_insertion_point(arr, target):
left, right = 0, len(arr)
while left < right:
mid = (left + right) // 2
if arr[mid] < target:
left = mid + 1
else:
right = mid
return left
# Example usage
sorted_array = [1, 3, 5, 7, 9]
target = 6
insertion_point = binary_search_insertion_point(sorted_array, target)
print(f"Insertion point for {target}: {insertion_point}")
This variation returns the index where the target should be inserted, even if it’s not present in the array.
3. Handling Floating-Point Numbers
When working with floating-point numbers, you need to be careful about comparing values due to precision issues. Here’s an example of binary search for floating-point numbers:
def binary_search_float(arr, target, epsilon=1e-9):
left, right = 0, len(arr) - 1
while right - left > 1:
mid = (left + right) // 2
if abs(arr[mid] - target) < epsilon:
return mid
elif arr[mid] < target:
left = mid
else:
right = mid
if abs(arr[left] - target) < epsilon:
return left
if abs(arr[right] - target) < epsilon:
return right
return -1
# Example usage
sorted_float_array = [1.1, 2.2, 3.3, 4.4, 5.5]
target = 3.3
result = binary_search_float(sorted_float_array, target)
print(f"Element {target} found at index: {result}")
In this variation, we use an epsilon
value to determine if two floating-point numbers are close enough to be considered equal.
Real-World Applications of Binary Search
Binary search is not just a theoretical concept; it has numerous practical applications in various domains of computer science and software development. Let’s explore some real-world scenarios where binary search proves to be invaluable:
1. Database Systems
Binary search is extensively used in database systems for efficient data retrieval. When data is stored in sorted order (e.g., in B-trees or other balanced search trees), binary search can quickly locate specific records or ranges of data. This is crucial for optimizing query performance in large-scale databases.
2. Compression Algorithms
Many compression algorithms, such as Huffman coding, use binary search trees to efficiently encode and decode data. The logarithmic time complexity of binary search helps in quickly traversing these trees, improving the overall performance of compression and decompression operations.
3. Computer Graphics
In computer graphics and game development, binary search is often used for collision detection and ray tracing. By organizing objects in space using data structures like bounding volume hierarchies, binary search can quickly determine intersections between rays and objects in a scene.
4. Machine Learning
Binary search plays a role in various machine learning algorithms, particularly in optimization problems. For example, in gradient descent algorithms, binary search can be used to find optimal learning rates or to implement line search methods for determining step sizes.
5. Version Control Systems
Git and other version control systems use binary search algorithms to efficiently find specific commits in a repository’s history. The “git bisect” command, which helps developers locate the commit that introduced a bug, relies on binary search to quickly narrow down the search space.
6. Network Routing
In computer networks, binary search is used in routing algorithms to efficiently look up routing table entries. This helps in quickly determining the next hop for a packet based on its destination IP address.
Optimizing Binary Search
While binary search is already highly efficient, there are some techniques to further optimize its performance in certain scenarios:
1. Interpolation Search
For uniformly distributed data, interpolation search can improve upon binary search by making educated guesses about the position of the target value. Instead of always choosing the middle element, it estimates the likely position based on the values at the ends of the search range.
def interpolation_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right and arr[left] <= target <= arr[right]:
if left == right:
if arr[left] == target:
return left
return -1
pos = left + ((target - arr[left]) * (right - left)) // (arr[right] - arr[left])
if arr[pos] == target:
return pos
if arr[pos] < target:
left = pos + 1
else:
right = pos - 1
return -1
# Example usage
sorted_array = [1, 2, 4, 8, 16, 32, 64, 128, 256, 512]
target = 64
result = interpolation_search(sorted_array, target)
print(f"Element {target} found at index: {result}")
Interpolation search can achieve O(log log n) time complexity for uniformly distributed data, but it may perform worse than binary search for other distributions.
2. Exponential Search
Exponential search combines a quick range-finding step with binary search. It’s particularly useful when searching unbounded or infinite lists, or when the target is likely to be near the beginning of the array.
def exponential_search(arr, target):
if arr[0] == target:
return 0
i = 1
while i < len(arr) and arr[i] <= target:
i *= 2
return binary_search(arr, target, i // 2, min(i, len(arr) - 1))
def binary_search(arr, target, left, right):
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
target = 10
result = exponential_search(sorted_array, target)
print(f"Element {target} found at index: {result}")
Exponential search has a time complexity of O(log i), where i is the index of the target element. This can be more efficient than binary search when the target is near the beginning of the array.
3. Jump Search
Jump search is a compromise between linear search and binary search. It works by skipping a fixed number of elements and then performing a linear search.
import math
def jump_search(arr, target):
n = len(arr)
step = int(math.sqrt(n))
prev = 0
while arr[min(step, n) - 1] < target:
prev = step
step += int(math.sqrt(n))
if prev >= n:
return -1
while arr[prev] < target:
prev += 1
if prev == min(step, n):
return -1
if arr[prev] == target:
return prev
return -1
# Example usage
sorted_array = [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377]
target = 55
result = jump_search(sorted_array, target)
print(f"Element {target} found at index: {result}")
Jump search has a time complexity of O(√n), which is between linear search O(n) and binary search O(log n). It can be useful in systems where jumping back is costly (e.g., in some types of memory or storage systems).
Common Mistakes and Pitfalls
When implementing binary search, there are several common mistakes and pitfalls to watch out for:
1. Off-by-One Errors
One of the most common mistakes in binary search implementations is off-by-one errors. These can occur when calculating the middle index or updating the search boundaries. Always double-check your index calculations and make sure you’re not accidentally excluding valid elements from the search range.
2. Integer Overflow
When calculating the middle index, the naive approach of (left + right) / 2
can lead to integer overflow for large arrays. A safer way to calculate the middle index is:
mid = left + (right - left) // 2
This approach avoids potential overflow issues while still correctly calculating the middle index.
3. Infinite Loops
Incorrect handling of the search boundaries can lead to infinite loops. Make sure your termination condition is correct and that you’re properly updating the left
and right
pointers in each iteration.
4. Assuming Unique Elements
If your array contains duplicate elements, a basic binary search implementation might not behave as expected when searching for the first or last occurrence of a value. Make sure to handle duplicates correctly if they’re possible in your data.
5. Not Handling Empty Arrays
Always check if the input array is empty before starting the binary search. Failing to do so can lead to index out of bounds errors.
6. Incorrect Comparisons for Floating-Point Numbers
When working with floating-point numbers, be cautious about using exact equality comparisons. Due to precision issues, it’s often better to use an epsilon value to determine if two floating-point numbers are close enough to be considered equal.
Conclusion
Binary search is a fundamental algorithm that every programmer should master. Its efficiency and versatility make it an invaluable tool in various domains of computer science and software development. By understanding the core concepts, implementations, and optimizations of binary search, you’ll be well-equipped to tackle a wide range of searching problems efficiently.
As you continue your journey in algorithm design and problem-solving, remember that binary search is just one piece of the puzzle. Combine it with other algorithms and data structures to create powerful and efficient solutions to complex problems. Practice implementing binary search in different scenarios, and always be on the lookout for opportunities to apply this elegant algorithm in your projects.
Keep exploring, keep coding, and never stop searching for ways to optimize your algorithms!