Breadth First Search (BFS): A Comprehensive Guide for Algorithmic Problem Solving
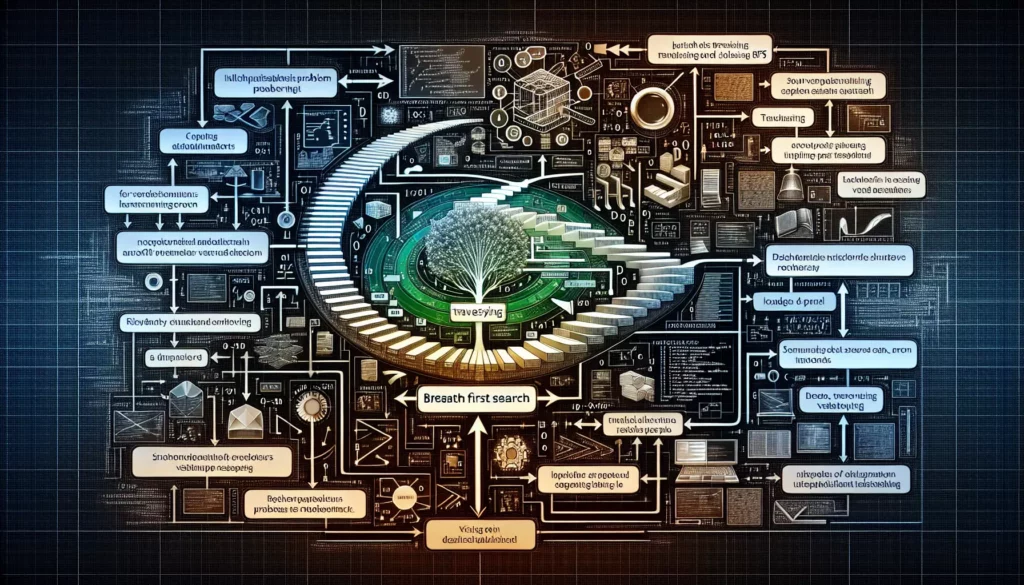
In the world of computer science and algorithmic problem-solving, Breadth First Search (BFS) stands as a fundamental and powerful technique. Whether you’re a beginner coder looking to expand your skills or an experienced developer preparing for technical interviews at top tech companies, understanding BFS is crucial. This comprehensive guide will delve deep into the intricacies of Breadth First Search, its applications, implementation, and its significance in coding interviews.
What is Breadth First Search?
Breadth First Search is a graph traversal algorithm that explores all the vertices of a graph or all the nodes of a tree at the present depth before moving on to the nodes at the next depth level. In simpler terms, it visits neighbors at the current depth before moving to nodes at the next depth level.
The key characteristics of BFS include:
- It visits nodes in order of their distance from the starting node
- It uses a queue data structure to keep track of nodes to visit
- It’s optimal for finding the shortest path on unweighted graphs
- It has a time complexity of O(V + E) where V is the number of vertices and E is the number of edges
How Does BFS Work?
To understand how BFS works, let’s break it down into steps:
- Start by choosing an initial node in the graph.
- Add this node to a queue and mark it as visited.
- While the queue is not empty:
- Remove the first node from the queue
- Add all of its unvisited neighbors to the queue and mark them as visited
- Repeat step 3 until the queue is empty
This process ensures that we explore all nodes at the current depth before moving deeper into the graph or tree.
Implementing BFS in Python
Let’s look at a basic implementation of BFS in Python:
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("BFS starting from vertex 'A':")
bfs(graph, 'A')
This implementation uses Python’s deque
from the collections
module, which provides an efficient queue data structure. The function takes a graph (represented as an adjacency list) and a starting node as input, then performs BFS and prints the nodes in the order they are visited.
Applications of Breadth First Search
BFS has numerous practical applications in computer science and beyond:
1. Shortest Path Problems
In unweighted graphs, BFS can find the shortest path between two nodes. This is particularly useful in scenarios like:
- GPS navigation systems for finding the route with the least number of turns
- Social networking sites to find the shortest connection between two users
- Puzzle solving, like finding the minimum number of moves in a sliding puzzle
2. Web Crawlers
Search engines use BFS to index web pages. Starting from a seed page, the crawler uses BFS to explore links, ensuring it covers a wide range of connected pages efficiently.
3. Network Broadcasting
In computer networks, BFS can be used to broadcast messages. It ensures that all nodes in the network receive the message in the minimum number of hops.
4. Social Network Analysis
BFS can help analyze social networks, finding degrees of separation between individuals or identifying clusters of connected users.
5. AI and Machine Learning
In artificial intelligence, BFS is used in various algorithms, including:
- Solving puzzles and games
- Finding optimal moves in game trees
- Exploring state spaces in planning problems
BFS vs DFS: When to Use Which?
While Breadth First Search and Depth First Search (DFS) are both graph traversal algorithms, they have different characteristics that make them suitable for different scenarios:
Use BFS when:
- You need to find the shortest path on an unweighted graph
- You’re working with a graph that might have cycles
- The solution is not far from the root of the tree
- You want to visit nodes in order of their distance from the start
Use DFS when:
- You need to search the entire graph
- You’re working with a very deep graph where solutions are likely at the bottom
- You’re implementing a topological sort
- You’re checking for graph properties like connectivity
BFS in Coding Interviews
Breadth First Search is a favorite topic in coding interviews, especially at major tech companies. Here are some types of problems where BFS is commonly used:
1. Shortest Path Problems
Questions involving finding the shortest path in a maze or grid are common. For example:
def shortest_path(maze, start, end):
rows, cols = len(maze), len(maze[0])
queue = deque([(start[0], start[1], 0)]) # (row, col, distance)
visited = set([(start[0], start[1])])
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)] # right, down, left, up
while queue:
r, c, dist = queue.popleft()
if (r, c) == end:
return dist
for dr, dc in directions:
nr, nc = r + dr, c + dc
if 0 <= nr < rows and 0 <= nc < cols and maze[nr][nc] == 0 and (nr, nc) not in visited:
queue.append((nr, nc, dist + 1))
visited.add((nr, nc))
return -1 # No path found
# Example usage
maze = [
[0, 0, 0, 0],
[1, 1, 0, 1],
[0, 0, 0, 0],
[0, 1, 1, 0]
]
start = (0, 0)
end = (3, 3)
print(f"Shortest path length: {shortest_path(maze, start, end)}")
2. Word Ladder Problems
These problems involve transforming one word to another by changing one letter at a time. BFS is ideal for finding the shortest transformation sequence.
from collections import deque
def word_ladder(begin_word, end_word, word_list):
word_set = set(word_list)
queue = deque([(begin_word, 1)])
visited = set([begin_word])
while queue:
word, length = queue.popleft()
if word == end_word:
return length
for i in range(len(word)):
for c in 'abcdefghijklmnopqrstuvwxyz':
next_word = word[:i] + c + word[i+1:]
if next_word in word_set and next_word not in visited:
queue.append((next_word, length + 1))
visited.add(next_word)
return 0 # No transformation sequence found
# Example usage
begin_word = "hit"
end_word = "cog"
word_list = ["hot","dot","dog","lot","log","cog"]
print(f"Shortest transformation sequence length: {word_ladder(begin_word, end_word, word_list)}")
3. Graph Connectivity Problems
BFS can be used to determine if all nodes in a graph are connected or to find connected components.
from collections import deque
def connected_components(graph):
visited = set()
components = []
for node in graph:
if node not in visited:
component = bfs_component(graph, node)
components.append(component)
visited.update(component)
return components
def bfs_component(graph, start):
visited = set([start])
queue = deque([start])
component = []
while queue:
node = queue.popleft()
component.append(node)
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
return component
# Example usage
graph = {
0: [1, 2],
1: [0, 2],
2: [0, 1],
3: [4],
4: [3],
5: []
}
components = connected_components(graph)
print(f"Number of connected components: {len(components)}")
print("Components:", components)
Advanced BFS Techniques
As you progress in your coding journey, you’ll encounter more advanced applications of BFS:
1. Bidirectional BFS
This technique runs two simultaneous BFS, one from the start node and one from the end node. It can be significantly faster than standard BFS for finding the shortest path between two nodes.
2. Multi-Source BFS
This variant starts BFS from multiple source nodes simultaneously. It’s useful in scenarios like finding the nearest hospital from multiple locations.
3. BFS on Implicit Graphs
Sometimes, the graph is not explicitly given but is implied by the problem statement. For example, in the Knight’s Tour problem, the graph of possible knight moves is implicit.
Time and Space Complexity of BFS
Understanding the complexity of BFS is crucial for optimizing your solutions:
Time Complexity
The time complexity of BFS is O(V + E), where V is the number of vertices and E is the number of edges in the graph. In the worst case, where every vertex is connected to every other vertex, this becomes O(V^2).
Space Complexity
The space complexity is O(V), as in the worst case, all vertices might be in the queue simultaneously.
Common Pitfalls and How to Avoid Them
When implementing BFS, be aware of these common mistakes:
- Forgetting to mark nodes as visited: This can lead to infinite loops in graphs with cycles. Always mark nodes as visited when you add them to the queue.
- Not handling disconnected graphs: If the graph is not fully connected, make sure your implementation can handle multiple components.
- Inefficient data structures: Using a list as a queue can be inefficient. Prefer
deque
in Python for O(1) append and pop operations. - Modifying the graph during traversal: This can lead to unexpected results. If you need to modify the graph, consider creating a copy first.
Practicing BFS: Resources and Problem Sets
To master BFS, consistent practice is key. Here are some resources to help you improve:
- LeetCode: Offers a wide range of BFS problems, from easy to hard.
- HackerRank: Has a dedicated graph theory track with BFS problems.
- Codeforces: Provides more challenging BFS problems for advanced practice.
- AlgoExpert: Offers curated BFS problems with video explanations.
Start with simpler problems and gradually move to more complex ones. Some classic BFS problems to tackle include:
- Binary Tree Level Order Traversal
- Word Ladder
- Number of Islands
- Rotting Oranges
- Shortest Bridge
Conclusion
Breadth First Search is a powerful algorithm that forms the backbone of many complex problem-solving techniques in computer science. Its ability to find shortest paths and explore graphs level by level makes it indispensable in various applications, from social network analysis to AI pathfinding.
As you continue your journey in coding and prepare for technical interviews, especially at top tech companies, mastering BFS will give you a significant advantage. Remember, the key to mastery is consistent practice and application to diverse problem sets.
Keep exploring, keep coding, and let the breadth of your knowledge grow with every BFS implementation you write!