Depth First Search (DFS): A Comprehensive Guide for Programmers
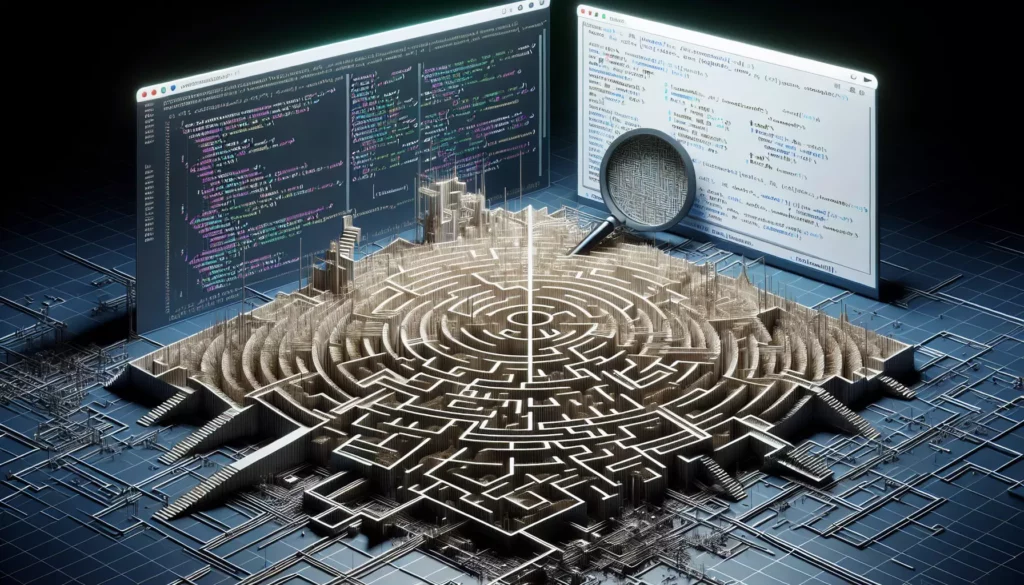
In the world of computer science and programming, algorithms play a crucial role in solving complex problems efficiently. One such fundamental algorithm that every programmer should be familiar with is Depth First Search (DFS). Whether you’re a beginner learning the basics of coding or an experienced developer preparing for technical interviews at major tech companies, understanding DFS is essential for developing strong algorithmic thinking and problem-solving skills.
In this comprehensive guide, we’ll dive deep into the world of Depth First Search, exploring its concepts, implementation, applications, and variations. By the end of this article, you’ll have a solid grasp of DFS and be well-equipped to tackle related coding challenges and interview questions.
Table of Contents
- What is Depth First Search?
- How DFS Works
- Implementing DFS
- Applications of DFS
- Variations of DFS
- Time and Space Complexity
- DFS vs. BFS: When to Use Each
- DFS in Technical Interviews
- Practice Problems
- Conclusion
1. What is Depth First Search?
Depth First Search (DFS) is a graph traversal algorithm that explores as far as possible along each branch before backtracking. It’s a systematic way to visit all the vertices of a graph or all the nodes of a tree. The key characteristic of DFS is that it goes deep into the structure before exploring other branches.
DFS can be visualized as exploring a maze by following one path until you hit a dead end, then backtracking to the last intersection and trying a different path. This process continues until all paths have been explored.
2. How DFS Works
The basic steps of the Depth First Search algorithm are as follows:
- Start at a chosen vertex (or node) in the graph.
- Mark the current vertex as visited.
- Explore an adjacent unvisited vertex (if there is one).
- Repeat steps 2 and 3 until there are no more unvisited adjacent vertices.
- Backtrack to the previous vertex.
- Repeat steps 3-5 until all vertices have been visited.
This process ensures that every vertex in the graph is visited exactly once, assuming the graph is connected. If the graph is not connected, you may need to run DFS multiple times, starting from different vertices, to cover all components of the graph.
3. Implementing DFS
There are two main ways to implement Depth First Search: recursively and iteratively. Let’s look at both approaches using Python.
Recursive Implementation
The recursive implementation of DFS is elegant and closely mirrors the conceptual understanding of the algorithm:
def dfs_recursive(graph, vertex, visited=None):
if visited is None:
visited = set()
visited.add(vertex)
print(vertex, end=' ') # Process the vertex
for neighbor in graph[vertex]:
if neighbor not in visited:
dfs_recursive(graph, neighbor, visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
dfs_recursive(graph, 'A')
In this implementation, we use a set to keep track of visited vertices. The function recursively calls itself for each unvisited neighbor of the current vertex.
Iterative Implementation
The iterative implementation uses a stack to keep track of vertices to visit:
def dfs_iterative(graph, start_vertex):
visited = set()
stack = [start_vertex]
while stack:
vertex = stack.pop()
if vertex not in visited:
visited.add(vertex)
print(vertex, end=' ') # Process the vertex
# Add unvisited neighbors to the stack
stack.extend(neighbor for neighbor in graph[vertex] if neighbor not in visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
dfs_iterative(graph, 'A')
This implementation uses a stack to simulate the recursive calls. It’s worth noting that the order of exploration might differ slightly from the recursive version due to the order in which neighbors are pushed onto the stack.
4. Applications of DFS
Depth First Search has numerous practical applications in computer science and beyond. Some common use cases include:
- Topological Sorting: Used in scheduling tasks with dependencies.
- Cycle Detection: Identifying cycles in graphs, useful in deadlock detection.
- Pathfinding: Finding paths between two vertices in a graph.
- Solving Puzzles: Such as maze solving or generating mazes.
- Connected Components: Finding strongly connected components in a graph.
- Backtracking Algorithms: Used in solving problems like N-Queens or Sudoku.
Let’s look at an example of using DFS for cycle detection in a graph:
def has_cycle(graph):
visited = set()
rec_stack = set()
def dfs_cycle(vertex):
visited.add(vertex)
rec_stack.add(vertex)
for neighbor in graph[vertex]:
if neighbor not in visited:
if dfs_cycle(neighbor):
return True
elif neighbor in rec_stack:
return True
rec_stack.remove(vertex)
return False
for vertex in graph:
if vertex not in visited:
if dfs_cycle(vertex):
return True
return False
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['D'],
'D': ['E'],
'E': ['B'] # This edge creates a cycle
}
print("Graph has cycle:", has_cycle(graph))
This implementation uses DFS to detect cycles in a directed graph. It keeps track of vertices in the current recursion stack to identify back edges, which indicate cycles.
5. Variations of DFS
There are several variations and extensions of the basic DFS algorithm, each tailored to solve specific problems or optimize certain aspects of the search. Some notable variations include:
Iterative Deepening Depth-First Search (IDDFS)
IDDFS combines the space-efficiency of DFS with the level-wise exploration of Breadth-First Search (BFS). It repeatedly performs DFS with increasing depth limits until the goal is found.
def iddfs(graph, start, goal, max_depth):
for depth in range(max_depth):
if dfs_limited(graph, start, goal, depth):
return True
return False
def dfs_limited(graph, node, goal, depth):
if depth == 0 and node == goal:
return True
if depth > 0:
for neighbor in graph[node]:
if dfs_limited(graph, neighbor, goal, depth - 1):
return True
return False
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []
}
print("Path exists:", iddfs(graph, 'A', 'F', 3))
Bidirectional Search
Bidirectional search runs two simultaneous searches: one forward from the initial state and one backward from the goal state, stopping when the two meet in the middle.
Informed DFS
Informed DFS uses heuristic information to guide the search, potentially finding solutions more quickly in certain problem domains.
6. Time and Space Complexity
Understanding the time and space complexity of DFS is crucial for assessing its efficiency in different scenarios:
Time Complexity
The time complexity of DFS is O(V + E), where V is the number of vertices and E is the number of edges in the graph. This is because:
- Each vertex is processed once: O(V)
- Each edge is considered once: O(E)
In the worst case, when the graph is complete (every vertex is connected to every other vertex), the time complexity becomes O(V^2).
Space Complexity
The space complexity of DFS depends on the implementation:
- For the recursive implementation, the space complexity is O(h), where h is the maximum depth of the recursion stack. In the worst case (a linear graph), this can be O(V).
- For the iterative implementation using a stack, the space complexity is also O(V) in the worst case.
It’s worth noting that DFS is generally more space-efficient than Breadth-First Search (BFS) for deep graphs, as it only needs to store a single path from the root to a leaf node at a time.
7. DFS vs. BFS: When to Use Each
While both DFS and BFS are graph traversal algorithms, they have different characteristics that make them suitable for different scenarios:
Use DFS when:
- You need to search deeper into the graph before exploring other branches.
- Memory is a constraint (DFS generally uses less memory than BFS).
- You’re solving problems like maze generation or topological sorting.
- You want to find a path to a goal, but not necessarily the shortest path.
Use BFS when:
- You need to find the shortest path in an unweighted graph.
- You want to explore all neighbors at the present depth before moving to nodes at the next depth level.
- The solution is not far from the root of the tree.
- You’re working with a graph that may have cycles (DFS can get stuck in an infinite loop without proper cycle detection).
Here’s a quick comparison of DFS and BFS implementations:
from collections import deque
def dfs(graph, start):
visited = set()
stack = [start]
while stack:
vertex = stack.pop()
if vertex not in visited:
visited.add(vertex)
print(vertex, end=' ')
stack.extend(reversed(graph[vertex]))
def bfs(graph, start):
visited = set()
queue = deque([start])
while queue:
vertex = queue.popleft()
if vertex not in visited:
visited.add(vertex)
print(vertex, end=' ')
queue.extend(graph[vertex])
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []
}
print("DFS:")
dfs(graph, 'A')
print("\nBFS:")
bfs(graph, 'A')
8. DFS in Technical Interviews
Depth First Search is a common topic in technical interviews, especially at major tech companies. Here are some tips to help you excel in DFS-related interview questions:
- Understand the basics: Make sure you can implement both recursive and iterative versions of DFS from memory.
- Recognize DFS patterns: Many problems involving trees, graphs, or exploring possibilities can be solved using DFS.
- Practice common applications: Familiarize yourself with problems like cycle detection, topological sorting, and connected components.
- Analyze time and space complexity: Be prepared to discuss the efficiency of your DFS implementation.
- Consider edge cases: Think about how your algorithm handles empty graphs, disconnected graphs, or graphs with cycles.
- Optimize when possible: Look for opportunities to prune the search or use problem-specific heuristics.
- Explain your thought process: Clearly communicate your approach, including why you chose DFS over other algorithms.
9. Practice Problems
To reinforce your understanding of DFS, here are some practice problems you can try:
- Number of Islands (LeetCode 200): Count the number of islands in a 2D grid.
- Course Schedule (LeetCode 207): Determine if it’s possible to finish all courses given prerequisites (cycle detection).
- Binary Tree Inorder Traversal (LeetCode 94): Implement inorder traversal of a binary tree using DFS.
- Word Search (LeetCode 79): Find if a word exists in a 2D board of characters.
- Clone Graph (LeetCode 133): Deep copy a graph using DFS.
Here’s a sample solution for the “Number of Islands” problem using DFS:
class Solution:
def numIslands(self, grid: List[List[str]]) -> int:
if not grid:
return 0
count = 0
for i in range(len(grid)):
for j in range(len(grid[0])):
if grid[i][j] == '1':
self.dfs(grid, i, j)
count += 1
return count
def dfs(self, grid, i, j):
if i < 0 or j < 0 or i >= len(grid) or j >= len(grid[0]) or grid[i][j] != '1':
return
grid[i][j] = '0' # Mark as visited
# Check all adjacent cells
self.dfs(grid, i+1, j)
self.dfs(grid, i-1, j)
self.dfs(grid, i, j+1)
self.dfs(grid, i, j-1)
This solution uses DFS to explore each island and mark its cells as visited. The main function counts the number of times DFS is initiated, which corresponds to the number of islands.
10. Conclusion
Depth First Search is a powerful and versatile algorithm that forms the backbone of many advanced graph algorithms and problem-solving techniques. By mastering DFS, you’ll not only improve your coding skills but also enhance your ability to tackle complex problems in computer science and software development.
Remember that understanding the theory is just the first step. The key to truly mastering DFS is practice. Implement the algorithm in different scenarios, solve various problems, and analyze its behavior in different types of graphs. As you gain more experience, you’ll develop an intuition for when and how to apply DFS effectively.
Whether you’re preparing for technical interviews at top tech companies or simply aiming to become a better programmer, a solid grasp of Depth First Search will serve you well throughout your coding journey. Keep exploring, keep practicing, and don’t hesitate to dive deep into the fascinating world of algorithms!