Mastering Cyclic Sort: A Powerful Algorithm for Efficient Array Manipulation
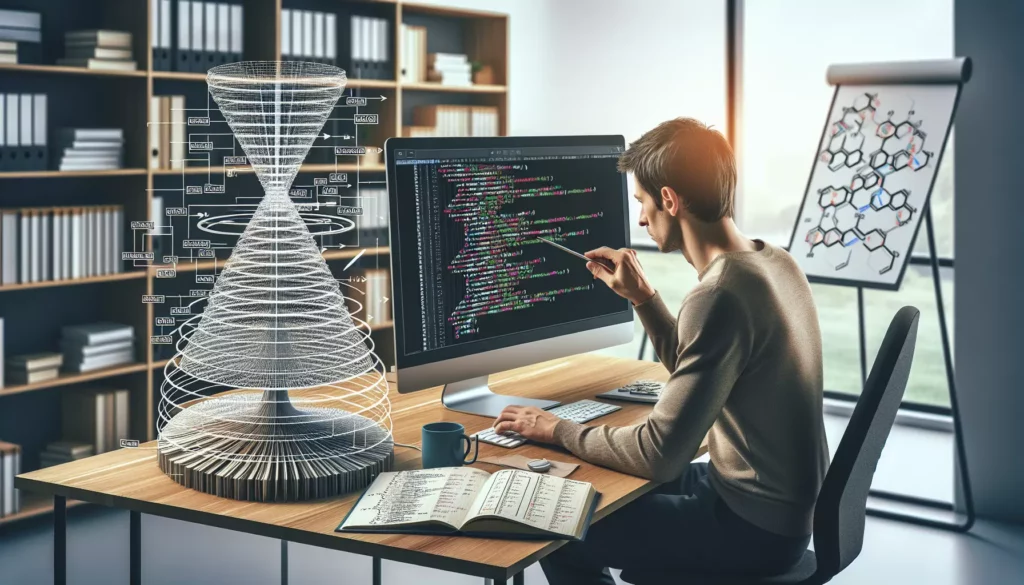
In the world of coding interviews and algorithmic problem-solving, certain patterns emerge that can give you a significant edge. One such pattern is the Cyclic Sort algorithm, a clever technique that can solve a variety of array-related problems with impressive efficiency. In this comprehensive guide, we’ll dive deep into Cyclic Sort, exploring its mechanics, applications, and why it’s a favorite among interviewers at top tech companies.
What is Cyclic Sort?
Cyclic Sort is an in-place sorting algorithm specifically designed for scenarios where you have an array containing numbers in a given range. It’s particularly useful when dealing with arrays where the elements are in the range of 1 to n, where n is the length of the array. The core idea behind Cyclic Sort is to place each number in its correct position in a single pass through the array.
The beauty of Cyclic Sort lies in its simplicity and efficiency. Unlike other sorting algorithms that might require multiple passes or additional space, Cyclic Sort achieves its goal with minimal operations and constant extra space.
How Cyclic Sort Works
Let’s break down the steps of the Cyclic Sort algorithm:
- Start with the first element of the array.
- Check if the current element is in its correct position (index = value – 1 for 1-based arrays).
- If it’s not in the correct position, swap it with the element at its correct position.
- If it is in the correct position, move to the next element.
- Repeat steps 2-4 until you’ve gone through all elements of the array.
Here’s a simple implementation of Cyclic Sort in Python:
def cyclic_sort(arr):
i = 0
while i < len(arr):
correct_position = arr[i] - 1
if arr[i] != arr[correct_position]:
arr[i], arr[correct_position] = arr[correct_position], arr[i]
else:
i += 1
return arr
# Example usage
arr = [3, 1, 5, 4, 2]
sorted_arr = cyclic_sort(arr)
print(sorted_arr) # Output: [1, 2, 3, 4, 5]
Time and Space Complexity
One of the main advantages of Cyclic Sort is its efficiency:
- Time Complexity: O(n), where n is the number of elements in the array. This is because we make at most n-1 swaps, and in each iteration, we are placing at least one element in its correct position.
- Space Complexity: O(1), as we are sorting the array in-place without using any extra space.
This combination of linear time complexity and constant space complexity makes Cyclic Sort an excellent choice for certain types of problems, especially in interview scenarios where efficiency is crucial.
When to Use Cyclic Sort
Cyclic Sort shines in specific scenarios, particularly when dealing with arrays that have the following characteristics:
- The array contains numbers from 1 to n (where n is the size of the array).
- The array might have duplicates or missing numbers within the range.
- You need to find missing numbers, duplicate numbers, or the smallest missing positive number in the array.
These conditions often appear in coding interview questions, making Cyclic Sort a valuable technique to master.
Common Interview Problems Solvable with Cyclic Sort
Let’s explore some popular interview questions where Cyclic Sort can be applied effectively:
1. Find the Missing Number
Problem: Given an array containing n distinct numbers taken from 0 to n, find the one that is missing from the array.
Solution using Cyclic Sort:
def find_missing_number(nums):
i, n = 0, len(nums)
while i < n:
if nums[i] < n and nums[i] != i:
nums[nums[i]], nums[i] = nums[i], nums[nums[i]]
else:
i += 1
for i in range(n):
if nums[i] != i:
return i
return n
# Example usage
arr = [3, 0, 1]
missing = find_missing_number(arr)
print(f"The missing number is: {missing}") # Output: The missing number is: 2
2. Find All Duplicate Numbers
Problem: Given an array of integers containing n + 1 integers where each integer is in the range [1, n] inclusive, find all the duplicate numbers.
Solution using Cyclic Sort:
def find_all_duplicates(nums):
i = 0
duplicates = []
while i < len(nums):
correct_position = nums[i] - 1
if nums[i] != nums[correct_position]:
nums[i], nums[correct_position] = nums[correct_position], nums[i]
else:
i += 1
for i in range(len(nums)):
if nums[i] != i + 1:
duplicates.append(nums[i])
return duplicates
# Example usage
arr = [4, 3, 2, 7, 8, 2, 3, 1]
duplicates = find_all_duplicates(arr)
print(f"The duplicate numbers are: {duplicates}") # Output: The duplicate numbers are: [2, 3]
3. Find the Smallest Missing Positive Number
Problem: Given an unsorted integer array, find the smallest missing positive integer.
Solution using Cyclic Sort:
def first_missing_positive(nums):
i = 0
n = len(nums)
while i < n:
if 0 < nums[i] <= n and nums[i] != nums[nums[i] - 1]:
nums[nums[i] - 1], nums[i] = nums[i], nums[nums[i] - 1]
else:
i += 1
for i in range(n):
if nums[i] != i + 1:
return i + 1
return n + 1
# Example usage
arr = [3, 4, -1, 1]
smallest_missing = first_missing_positive(arr)
print(f"The smallest missing positive number is: {smallest_missing}") # Output: The smallest missing positive number is: 2
Advantages of Cyclic Sort
Cyclic Sort offers several advantages that make it a valuable addition to your algorithmic toolkit:
- Efficiency: With its O(n) time complexity and O(1) space complexity, Cyclic Sort is highly efficient for certain types of problems.
- In-place sorting: The algorithm sorts the array without requiring any additional space, which is beneficial when working with large datasets or in memory-constrained environments.
- Simplicity: The concept behind Cyclic Sort is straightforward and easy to understand, making it a good choice for explaining your thought process during interviews.
- Versatility: While primarily used for arrays with elements in a specific range, the concept can be adapted to solve various related problems.
Limitations of Cyclic Sort
While Cyclic Sort is powerful in certain scenarios, it’s important to understand its limitations:
- Specific range requirement: Cyclic Sort is most effective when dealing with arrays containing numbers in a specific range (usually 1 to n). It’s not suitable for general-purpose sorting.
- Not stable: Cyclic Sort is not a stable sorting algorithm, meaning it doesn’t preserve the relative order of equal elements.
- Limited applicability: While excellent for certain types of problems, Cyclic Sort is not as widely applicable as more general sorting algorithms like QuickSort or MergeSort.
Comparing Cyclic Sort to Other Sorting Algorithms
To better understand where Cyclic Sort fits in the landscape of sorting algorithms, let’s compare it to some other common sorting techniques:
Algorithm | Time Complexity | Space Complexity | Stability | In-Place |
---|---|---|---|---|
Cyclic Sort | O(n) | O(1) | No | Yes |
QuickSort | O(n log n) average, O(n^2) worst | O(log n) | No | Yes |
MergeSort | O(n log n) | O(n) | Yes | No |
HeapSort | O(n log n) | O(1) | No | Yes |
BubbleSort | O(n^2) | O(1) | Yes | Yes |
As we can see, Cyclic Sort stands out with its linear time complexity and constant space complexity, making it highly efficient for its specific use cases.
Advanced Applications of Cyclic Sort
While we’ve covered some common applications of Cyclic Sort, let’s explore some more advanced problems where this algorithm can be creatively applied:
1. Find the Corrupt Pair
Problem: You are given an unsorted array containing ‘n’ numbers taken from the range 1 to ‘n’. The array originally contained all the numbers from 1 to ‘n’, but due to a data error, one of the numbers got duplicated which also resulted in one number going missing. Find both these numbers.
Solution using Cyclic Sort:
def find_corrupt_pair(nums):
i = 0
while i < len(nums):
correct_position = nums[i] - 1
if nums[i] != nums[correct_position]:
nums[i], nums[correct_position] = nums[correct_position], nums[i]
else:
i += 1
for i in range(len(nums)):
if nums[i] != i + 1:
return [nums[i], i + 1]
return [-1, -1] # No corrupt pair found
# Example usage
arr = [3, 1, 2, 5, 2]
corrupt_pair = find_corrupt_pair(arr)
print(f"The corrupt pair is: {corrupt_pair}") # Output: The corrupt pair is: [2, 4]
2. Find the First K Missing Positive Numbers
Problem: Given an unsorted array containing numbers and a number ‘k’, find the first ‘k’ missing positive numbers in the array.
Solution using Cyclic Sort:
def find_first_k_missing_positive(nums, k):
i, n = 0, len(nums)
while i < n:
correct_position = nums[i] - 1
if 0 < nums[i] <= n and nums[i] != nums[correct_position]:
nums[i], nums[correct_position] = nums[correct_position], nums[i]
else:
i += 1
missing_numbers = []
extra_numbers = set()
for i in range(n):
if len(missing_numbers) < k:
if nums[i] != i + 1:
missing_numbers.append(i + 1)
extra_numbers.add(nums[i])
i = 1
while len(missing_numbers) < k:
candidate = i + n
if candidate not in extra_numbers:
missing_numbers.append(candidate)
i += 1
return missing_numbers
# Example usage
arr = [3, -1, 4, 5, 5]
k = 3
missing_numbers = find_first_k_missing_positive(arr, k)
print(f"The first {k} missing positive numbers are: {missing_numbers}")
# Output: The first 3 missing positive numbers are: [1, 2, 6]
Cyclic Sort in Real-World Scenarios
While Cyclic Sort is often associated with coding interviews and algorithmic problem-solving, its principles can be applied in various real-world scenarios:
- Data Deduplication: In scenarios where you need to identify and remove duplicate entries from a dataset within a known range, Cyclic Sort can be an efficient approach.
- Error Detection in Sequential Data: When dealing with sequential data (like ID numbers or timestamps) where you expect values to be in a certain range, Cyclic Sort can help identify missing or duplicate entries quickly.
- Network Packet Sequencing: In networking, packets often come with sequence numbers. A variation of Cyclic Sort could be used to efficiently reorder out-of-sequence packets.
- Memory Management: In systems where memory blocks are numbered sequentially, Cyclic Sort concepts could be applied to efficiently manage and allocate memory blocks.
Tips for Mastering Cyclic Sort
To become proficient with Cyclic Sort and apply it effectively in coding interviews or real-world problems, consider the following tips:
- Practice Pattern Recognition: Learn to identify problems where Cyclic Sort can be applied. Look for keywords like “array contains numbers from 1 to n” or “find missing/duplicate numbers.”
- Understand the Core Concept: Focus on grasping the fundamental idea of placing each element in its correct position. Once you understand this, applying it to various problems becomes easier.
- Implement from Scratch: Try implementing Cyclic Sort from memory multiple times. This will help solidify your understanding and make it easier to adapt the algorithm to different problem scenarios.
- Solve Variations: After mastering the basic implementation, challenge yourself with different variations of the problem. This will help you develop the ability to adapt the algorithm to unique situations.
- Analyze Time and Space Complexity: Always be prepared to discuss the efficiency of your solution. Understanding why Cyclic Sort is O(n) time and O(1) space will give you confidence in explaining your approach.
- Compare with Other Methods: For each problem you solve using Cyclic Sort, consider how you might solve it using other techniques. This will help you appreciate the efficiency of Cyclic Sort and understand when it’s the best choice.
Conclusion
Cyclic Sort is a powerful and efficient algorithm that can be a game-changer in certain types of coding problems, particularly those involving arrays with elements in a specific range. Its ability to sort in-place with linear time complexity makes it an attractive choice for both interview scenarios and real-world applications where efficiency is crucial.
By mastering Cyclic Sort, you add a valuable tool to your problem-solving toolkit. It’s not just about memorizing an algorithm; it’s about understanding a pattern that can be applied creatively to solve a variety of problems. As you practice and become more familiar with Cyclic Sort, you’ll likely find yourself recognizing opportunities to use it in diverse scenarios, both in coding challenges and practical applications.
Remember, while Cyclic Sort is powerful in its niche, it’s just one of many algorithmic techniques you should be familiar with. A well-rounded understanding of various algorithms and data structures, combined with the ability to recognize when to apply each one, is key to excelling in coding interviews and tackling complex programming challenges.
Keep practicing, stay curious, and don’t hesitate to explore how Cyclic Sort can be adapted or combined with other techniques to solve even more complex problems. Happy coding!