How to Learn from Failed Interviews: Turning Setbacks into Success
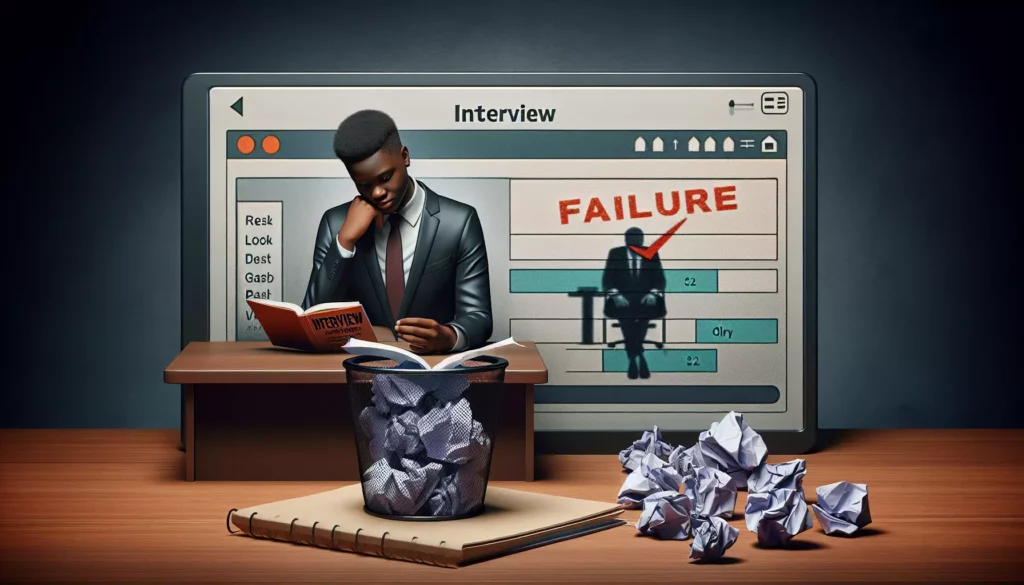
In the competitive world of tech, interviews can be daunting experiences, especially when you’re aiming for positions at major companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). While success is always the goal, the reality is that not every interview will end with a job offer. However, failed interviews don’t have to be just disappointing experiences; they can be invaluable learning opportunities that propel you forward in your career journey. In this comprehensive guide, we’ll explore how to effectively learn from failed interviews, turning these setbacks into stepping stones for future success.
Understanding the Value of Failed Interviews
Before diving into the specifics of learning from failed interviews, it’s crucial to understand why these experiences are so valuable:
- Real-world feedback: Interviews provide direct insight into what companies are looking for and where you might need improvement.
- Exposure to industry standards: Each interview gives you a glimpse into the current expectations and practices in the tech industry.
- Practice under pressure: Interviews are high-stakes situations that help you build resilience and improve your performance in stressful scenarios.
- Identifying knowledge gaps: Failed interviews can highlight areas where your skills or knowledge may be lacking, giving you clear targets for improvement.
Immediate Post-Interview Reflection
The learning process begins immediately after the interview. Here’s what you should do:
1. Document Your Experience
As soon as possible after the interview, write down everything you can remember:
- Questions asked
- Your responses
- Coding problems presented
- Your approach to solving them
- Any feedback provided by the interviewer
This documentation will be invaluable for later analysis and preparation.
2. Assess Your Performance
Be honest with yourself about how you performed:
- Which questions were you confident about?
- Where did you struggle?
- How was your communication throughout the interview?
- Did you manage your time effectively?
3. Identify Emotional Responses
Recognize any emotional reactions you had during the interview:
- Did you feel anxious at any point?
- Were there moments of confusion or frustration?
- How did these emotions affect your performance?
Understanding your emotional responses can help you better prepare for future interviews.
Analyzing Technical Challenges
A significant part of tech interviews, especially for FAANG companies, involves solving coding problems and discussing algorithms. Here’s how to learn from the technical aspects of a failed interview:
1. Review Coding Problems
For each coding problem you encountered:
- Try to solve it again without time pressure
- Research optimal solutions online
- Analyze the time and space complexity of different approaches
Here’s an example of how you might approach this:
// Problem: Reverse a linked list
// Your interview solution (suboptimal):
public ListNode reverseList(ListNode head) {
if (head == null || head.next == null) return head;
ListNode prev = null;
ListNode current = head;
while (current != null) {
ListNode nextTemp = current.next;
current.next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
// Optimal recursive solution:
public ListNode reverseList(ListNode head) {
if (head == null || head.next == null) return head;
ListNode p = reverseList(head.next);
head.next.next = head;
head.next = null;
return p;
}
By comparing your solution to the optimal one, you can identify areas for improvement in your problem-solving approach.
2. Identify Knowledge Gaps
Make a list of concepts or algorithms you weren’t familiar with during the interview. This might include:
- Data structures you struggled with (e.g., trees, graphs, heaps)
- Algorithmic concepts you found challenging (e.g., dynamic programming, backtracking)
- System design principles you weren’t confident about
3. Analyze Your Problem-Solving Approach
Reflect on how you tackled the problems presented:
- Did you ask clarifying questions before starting to code?
- Did you consider edge cases?
- How well did you explain your thought process?
- Did you optimize your initial solution?
Learning from Behavioral Questions
Technical skills are crucial, but so are soft skills. Many candidates underestimate the importance of behavioral questions. Here’s how to improve in this area:
1. Review Common Behavioral Questions
Make a list of behavioral questions you encountered or expect to encounter, such as:
- “Tell me about a time you faced a significant challenge in a project.”
- “How do you handle conflicts in a team?”
- “Describe a situation where you had to meet a tight deadline.”
2. Prepare Structured Responses
Use the STAR method (Situation, Task, Action, Result) to structure your answers:
Situation: Describe the context
Task: Explain your responsibility
Action: Detail the steps you took
Result: Share the outcome and what you learned
3. Practice Storytelling
Effective answers to behavioral questions often involve storytelling. Practice telling your professional stories concisely and engagingly.
Seeking and Utilizing Feedback
Feedback is gold when it comes to learning from failed interviews. Here’s how to make the most of it:
1. Request Feedback from the Interviewer
If you haven’t received detailed feedback, don’t hesitate to ask for it. Send a polite email thanking them for their time and requesting specific feedback on areas for improvement.
2. Analyze the Feedback Objectively
When you receive feedback:
- Look for patterns across multiple interviews
- Identify both technical and soft skill areas mentioned
- Consider how the feedback aligns with your self-assessment
3. Create an Improvement Plan
Based on the feedback and your analysis:
- Set specific, measurable goals for improvement
- Create a timeline for addressing each area
- Identify resources (books, courses, mentors) that can help you improve
Leveraging Online Resources and Communities
The internet is a treasure trove of resources for improving your interview skills. Here’s how to use it effectively:
1. Coding Platforms
Utilize platforms like LeetCode, HackerRank, or AlgoCademy to practice coding problems. These platforms often have problems similar to those used in tech interviews.
2. Online Courses
Consider taking courses on algorithms, data structures, and system design. Platforms like Coursera, edX, and Udacity offer courses from top universities and tech companies.
3. Tech Interview Preparation Websites
Websites like InterviewBit and Pramp offer mock interviews and curated preparation paths.
4. Community Forums
Engage with communities on platforms like Reddit (/r/cscareerquestions) or Stack Overflow to learn from others’ experiences and get advice.
Improving Your Coding Skills
Continuous improvement of your coding skills is crucial. Here are some strategies:
1. Regular Coding Practice
Set aside time each day for coding practice. Consistency is key to improving your skills.
2. Implement Data Structures from Scratch
Understanding the internals of data structures will help you use them more effectively. Try implementing common data structures in your preferred language:
// Example: Implementing a basic Binary Search Tree in Java
public class BinarySearchTree {
class Node {
int key;
Node left, right;
public Node(int item) {
key = item;
left = right = null;
}
}
Node root;
BinarySearchTree() {
root = null;
}
void insert(int key) {
root = insertRec(root, key);
}
Node insertRec(Node root, int key) {
if (root == null) {
root = new Node(key);
return root;
}
if (key < root.key)
root.left = insertRec(root.left, key);
else if (key > root.key)
root.right = insertRec(root.right, key);
return root;
}
// Other methods like delete, search, etc.
}
3. Study Time and Space Complexity
Understanding the efficiency of your solutions is crucial. Practice analyzing the time and space complexity of different algorithms and data structures.
4. Read and Write Clean Code
Study clean coding practices and apply them in your solutions. This includes proper naming conventions, code organization, and commenting.
Preparing for System Design Questions
System design questions are common in interviews for more senior positions. Here’s how to improve:
1. Study Scalable Systems
Learn about distributed systems, load balancing, caching, and database sharding.
2. Practice Designing Real-World Systems
Try designing systems like:
- A URL shortener
- A social media news feed
- A ride-sharing application
3. Understand Trade-offs
Practice explaining the pros and cons of different design decisions.
Improving Communication Skills
Effective communication is crucial in interviews. Here’s how to enhance your communication skills:
1. Practice Explaining Complex Concepts
Try explaining technical concepts to non-technical friends or family members.
2. Record Mock Interviews
Record yourself in mock interviews and review your communication style.
3. Join a Public Speaking Group
Consider joining groups like Toastmasters to improve your public speaking skills.
Managing Interview Anxiety
Interview anxiety can significantly impact your performance. Here are some strategies to manage it:
1. Preparation and Practice
Thorough preparation can boost your confidence and reduce anxiety.
2. Mindfulness and Relaxation Techniques
Practice deep breathing or meditation before interviews to calm your nerves.
3. Positive Self-talk
Replace negative thoughts with positive affirmations.
Creating a Personal Brand
Building a strong personal brand can make you stand out in interviews:
1. Develop an Online Presence
Maintain an active GitHub profile and contribute to open-source projects.
2. Blog about Tech
Start a technical blog to showcase your knowledge and communication skills.
3. Networking
Attend tech meetups and conferences to build your professional network.
Conclusion: Embracing the Learning Process
Failed interviews, while disappointing, are not the end of your journey. They are valuable stepping stones on your path to success. By thoroughly analyzing your experiences, seeking feedback, and consistently working on improving your skills, you can transform these setbacks into powerful learning opportunities.
Remember, even the most successful tech professionals have faced rejection at some point in their careers. What sets them apart is their ability to learn, adapt, and persist. Embrace each interview as a chance to grow, and approach your preparation with renewed focus and determination.
As you continue on your journey, consider leveraging platforms like AlgoCademy, which offer structured learning paths, interactive coding tutorials, and AI-powered assistance to help you master the skills needed for technical interviews. With the right mindset and resources, you can turn your interview experiences – both successful and unsuccessful – into valuable tools for career growth and ultimate success in the tech industry.